Tiles– A Sliding Puzzle Game in Python With Source Code
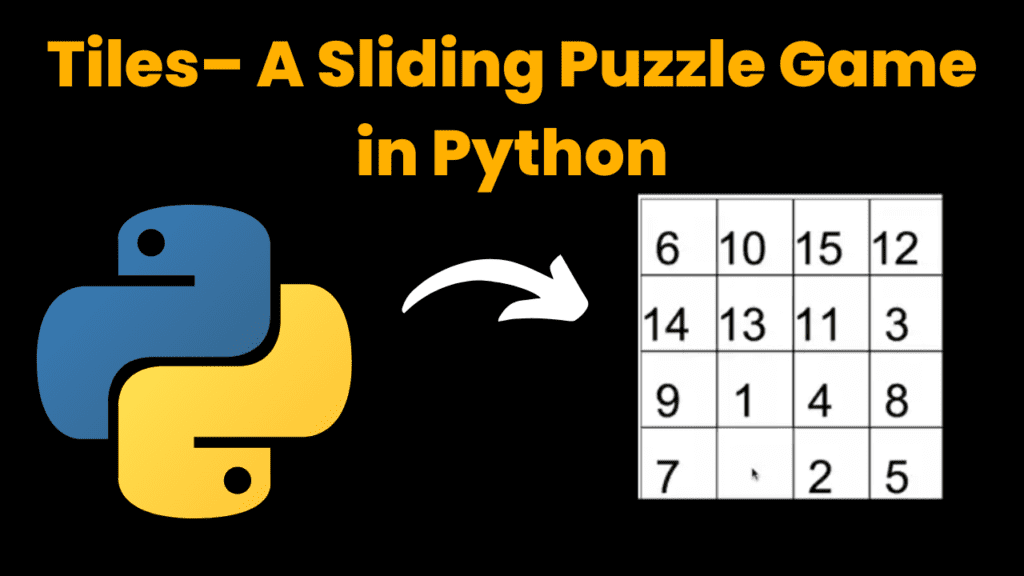
Introduction :
Tiles is a classic sliding puzzle game where the objective is to arrange numbered tiles in a specific order. The puzzle consists of a grid with one empty space, allowing adjacent tiles to be swapped. In this implementation, the goal is to arrange tiles numbered from one to fifteen in ascending order from left to right and top to bottom. In this blog post, we’ll walk through creating the Tiles puzzle game using Python and the turtle graphics module. This project is a fun way to practice programming and understand basic game mechanics Tiles is a sliding puzzle game where the player clicks on a tile adjacent to the empty space to swap their positions. The game starts with a scrambled arrangement of tiles, and the player must move tiles around until they achieve the goal state: a grid with numbers from 1 to 15 in ascending order
Required Modules and Packages :-
To run this project, you need the turtle and freegames modules. If you don’t have freegames installed, you can install it using:
pip install freegames
How to Run the Code :-
1. Install Dependencies:
○ Ensure Python is installed.
○ Install the freegames module using pip.
2. Run the Code:
○ Savetheprovided code in a file named tiles_puzzle.py.
Run the script using Python:
python tiles_puzzle.py
3. Play the Game:
○ Agraphical window will open displaying the Tiles puzzle.
○ Click on a tile adjacent to the empty space to move it.
○ Yourgoal is to arrange the tiles in numerical order.
Code Explanation :-
Let’s break down the code to understand how the Tiles puzzle game is implemented:
1. Importing Modules
from random import *
from turtle import *
from freegames import floor, vector
● random.choice: Used to randomly choose a neighboring tile to swap.
● turtle: Used for graphical output.
● freegames: Provides utilities for drawing and managing game elements.
2. Initializing Variables
tiles = {}
neighbors = [
vector(100, 0),
vector(-100, 0),
vector(0, 100),
vector(0,-100),
]
● tiles:Adictionary that maps tile positions to their numbers or None for the empty
space.
● neighbors: A list of vectors representing the possible moves (up, down, left, right) from
a given tile
3. Loading and Scrambling the Tiles
def load():
"""Load tiles and scramble."""
count = 1
for y in range(-200, 200, 100):
for x in range(-200, 200, 100):
mark = vector(x, y)
tiles[mark] = count
count += 1
tiles[mark] = None
for count in range(1000):
neighbor = choice(neighbors)
spot = mark + neighbor
if spot in tiles:
number = tiles[spot]
tiles[spot] = None
tiles[mark] = number
mark = spot
● load(): Initializes the tiles in a sequential order and then scrambles them by making random moves. The mark variable represents the current position of the empty space.
4. Drawing the Tiles
def square(mark, number):
"""Draw white square with black outline and number."""
up()
goto(mark.x, mark.y)
down()
color('black', 'white')
begin_fill()
for count in range(4):
forward(99)
left(90)
end_fill()
if number is None:
return
elif number < 10:
forward(20)
write(number, font=('Arial', 60, 'normal'))
● square(mark, number): Draws a tile at the specified position with a number. The tile has a white background with a black border. If the tile is empty, it just draws the border
without a number.
5. Handling Tile Moves
def tap(x, y):
"""Swap tile and empty square."""
x = floor(x, 100)
y = floor(y, 100)
mark = vector(x, y)
for neighbor in neighbors:
spot = mark + neighbor
if spot in tiles and tiles[spot] is None:
number = tiles[mark]
tiles[spot] = number
square(spot, number)
tiles[mark] = None
square(mark, None)
● tap(x, y):Handles mouse clicks. It converts the click position into the nearest tile position and swaps it with the empty space if it is adjacent.
6. Drawing All Tiles
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
load()
draw()
onscreenclick(tap)
done()
● setup(): Initializes the graphical window with a width and height of 420.
● hideturtle(): Hides the turtle cursor for a cleaner look.
● tracer(False): Disables automatic screen updates for better performance.
● load(): Initializes and scrambles the tiles.
● draw(): Draws the initial state of the puzzle.
● onscreenclick(tap): Sets up the tap() function to handle tile interactions.
● done(): Completes the setup and starts the game loop
Source Code :
from random import *
from turtle import *
from freegames import floor, vector
tiles = {}
neighbors = [
vector(100, 0),
vector(-100, 0),
vector(0, 100),
vector(0, -100),
]
def load():
"""Load tiles and scramble."""
count = 1
for y in range(-200, 200, 100):
for x in range(-200, 200, 100):
mark = vector(x, y)
tiles[mark] = count
count += 1
tiles[mark] = None
for count in range(1000):
neighbor = choice(neighbors)
spot = mark + neighbor
if spot in tiles:
number = tiles[spot]
tiles[spot] = None
tiles[mark] = number
mark = spot
def square(mark, number):
"""Draw white square with black outline and number."""
up()
goto(mark.x, mark.y)
down()
color('black', 'white')
begin_fill()
for count in range(4):
forward(99)
left(90)
end_fill()
if number is None:
return
elif number < 10:
forward(20)
write(number, font=('Arial', 60, 'normal'))
def tap(x, y):
"""Swap tile and empty square."""
x = floor(x, 100)
y = floor(y, 100)
mark = vector(x, y)
for neighbor in neighbors:
spot = mark + neighbor
if spot in tiles and tiles[spot] is None:
number = tiles[mark]
tiles[spot] = number
square(spot, number)
tiles[mark] = None
square(mark, None)
def draw():
"""Draw all tiles."""
for mark in tiles:
square(mark, tiles[mark])
update()
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
load()
draw()
onscreenclick(tap)
done()
Output :
Running the code will open a graphical window displaying the Tiles puzzle game. Click on a tile adjacent to the empty space to move it. Arrange the tiles in numerical order to complete the puzzle.
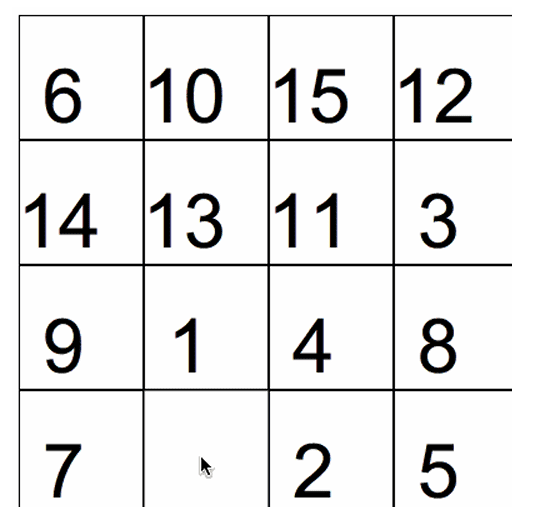
Reference
For more information on the turtle and freegames modules, check out their documentation:
● Turtle Graphics Documentation
Find More Projects
Drawing Ganesha Using Python Turtle Graphics[Drawing Ganapati Using Python] Introduction In this blog post, we will learn how to draw Lord Ganesha …
Contact Management System in Python with a Graphical User Interface (GUI) Introduction: The Contact Management System is a Python-based application designed to …
KBC Game using Python with Source Code Introduction : Welcome to this blog post on building a “Kaun Banega Crorepati” (KBC) game …
Basic Logging System in C++ With Source Code Introduction : It is one of the most important practices in software development. Logging …
Snake Game Using C++ With Source Code Introduction : The Snake Game is one of the most well-known arcade games, having its …
C++ Hotel Management System With Source Code Introduction : It is very important to manage the hotels effectively to avail better customer …