BMI Calculator Using HTML , CSS & JavaScript
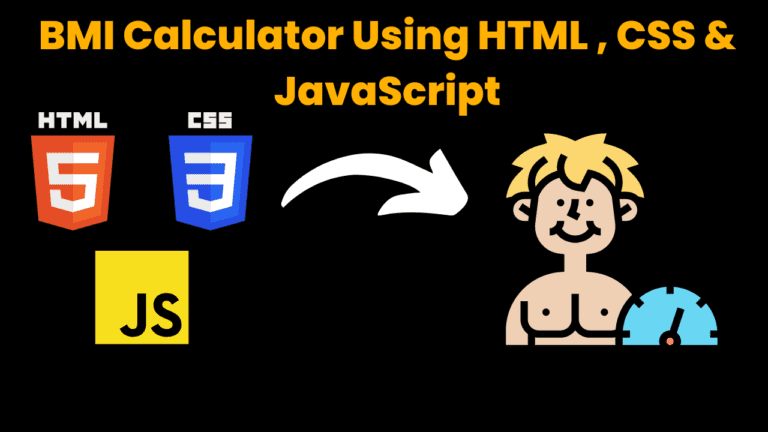
Introduction:
The Body Mass Index (BMI) calculator is a web application designed to help users calculate their BMI based on their height and weight. BMI is a measure of body fat based on an individual’s weight in relation to their height. This calculator provides a convenient way for users to assess their body composition and determine if they are underweight, normal weight, overweight, or obese according to standard BMI categories.
Key Features:
User Input: The calculator allows users to enter their height and weight in metric units (centimeters and kilograms) or imperial units (feet, inches, and pounds).
BMI Calculation: The application calculates the BMI using the formula: BMI = weight (kg) / (height (m))^2. The result is then displayed to the user.
BMI Categories: The calculated BMI is categorized into different ranges such as underweight, normal weight, overweight, and obese. These categories provide users with an understanding of their current body composition.
Responsive Design: The web application is designed to be responsive, adapting to different screen sizes and devices, ensuring optimal user experience across desktop, tablet, and mobile devices.
Attractive Layout Design: The user interface is visually appealing, featuring a modern and clean design to enhance the overall user experience.
Background Animation: The application incorporates a background animation to add an element of visual interest and engagement, making the user experience more enjoyable.
Source Code:
HTML:
BMI Calculator
BMI Calculator
CSS (style.css):
/* @codewithcurious.com */
body {
background-color: #f2f2f2;
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
.container {
max-width: 400px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
text-align: center;
}
h1 {
color: #333;
}
.input-container {
margin-bottom: 10px;
text-align: left;
}
input[type="number"] {
width: 100%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
}
button {
background-color: #4caf50;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
}
#result {
margin-top: 20px;
font-weight: bold;
}
/* @codewithcurious.com */
body {
background-color: #f2f2f2;
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
.container {
max-width: 400px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
text-align: center;
}
h1 {
color: #333;
}
.input-container {
margin-bottom: 10px;
text-align: left;
}
input[type="number"] {
width: 100%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
}
button {
background-color: #4caf50;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
}
#result {
margin-top: 20px;
font-weight: bold;
}
JavaScript (script.js):
//@codewithcurious.com
function calculateBMI() {
var heightInput = document.getElementById("height");
var weightInput = document.getElementById("weight");
var resultDiv = document.getElementById("result");
var height = parseFloat(heightInput.value);
var weight = parseFloat(weightInput.value);
if (isNaN(height) || isNaN(weight)) {
resultDiv.innerHTML = "Please enter valid height and weight.";
return;
}
var bmi = weight / ((height / 100) ** 2);
var category = "";
if (bmi < 18.5) {
category = "Underweight";
} else if (bmi < 25) {
category = "Normal weight";
} else if (bmi < 30) {
category = "Overweight";
} else {
category = "Obese";
}
resultDiv.innerHTML = "Your BMI is " + bmi.toFixed(2) + " (" + category + ")";
}
//@codewithcurious.com
function calculateBMI() {
var heightInput = document.getElementById("height");
var weightInput = document.getElementById("weight");
var resultDiv = document.getElementById("result");
var height = parseFloat(heightInput.value);
var weight = parseFloat(weightInput.value);
if (isNaN(height) || isNaN(weight)) {
resultDiv.innerHTML = "Please enter valid height and weight.";
return;
}
var bmi = weight / ((height / 100) ** 2);
var category = "";
if (bmi < 18.5) {
category = "Underweight";
} else if (bmi < 25) {
category = "Normal weight";
} else if (bmi < 30) {
category = "Overweight";
} else {
category = "Obese";
}
resultDiv.innerHTML = "Your BMI is " + bmi.toFixed(2) + " (" + category + ")";
}
Output:
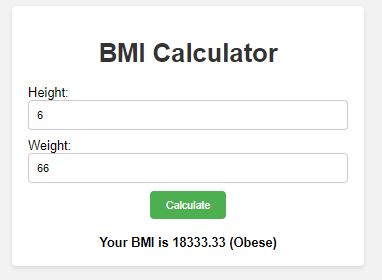
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …