Typing Game Using Python With Source Code
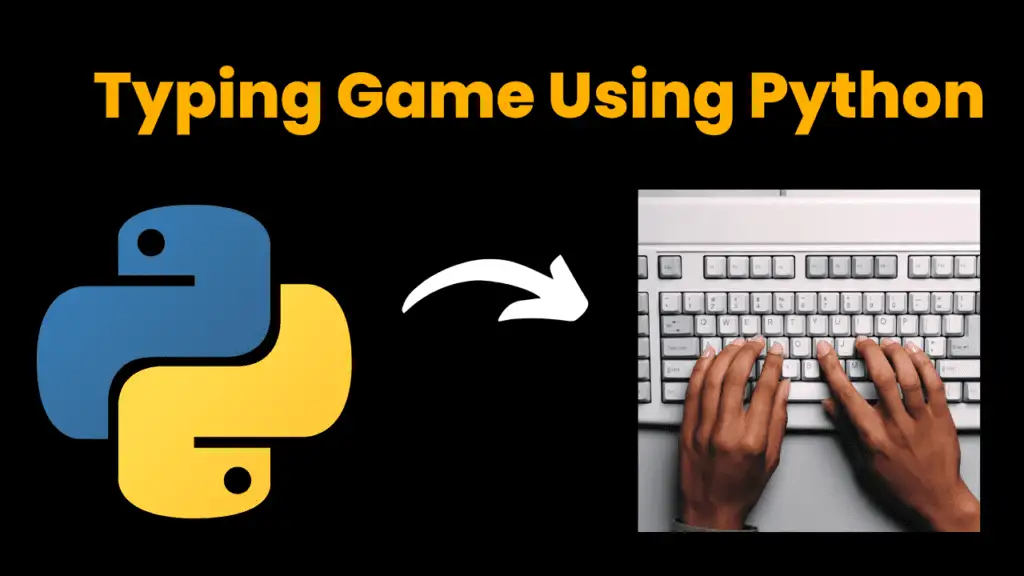
Introduction :
In this tutorial, we’ll build a simple typing game using Python’s turtle graphics module. This game will challenge players to type letters falling from the top of the screen, and their score will increase based on their accuracy. It’s a great way to practice both programming and typing skills. This typing game is designed to be a fun and interactive way to improve typing skills. The game involves letters falling from the top of the screen, and players need to type the correct letters to score points. The game uses Python’s turtle graphics module for visualization and free games for managing vectors.
Required Modules :
To run this project, you’ll need the turtle and freegames modules. Install the freegames module if you haven’t already:
pip install freegames
How to Run the Code :
1. Install Dependencies:
○ Ensure Python is installed.
○ Install the freegames module using pip.
2. Run the Code:
○ Save the provided code in a file named typing_game.py.
Run the script using Python:
python typing_game.py
3. Play the Game:
○ Agraphical window will open where letters will fall from the top.
○ Typethe letters that appear to score points.
Code Explanation :
Let’s break down the code for creating this typing game:
1. Importing Modules
from random import choice, randrange
from string import ascii_lowercase
from turtle import *
from freegames import vector
● choiceandrandrange from random are used to select random letters and positions.
● ascii_lowercase from string provides a list of lowercase letters.
● turtleis used for graphical output.
● vectorfrom freegames is used for managing the position of letters.
2. Initializing Variables
targets = []
letters = []
score = 0
● targets: List to keep track of the falling letters’ positions.
● letters: List to keep track of the falling letters themselves.
● score:Variable to track the player’s score..
3. Defining Functions
def inside(point):
"""Return True if point on screen."""
return-200 < point.x < 200 and-200 < point.y < 200
● inside(point): Checks if a point is within the screen’s bounds. Drawing Letters on the Screen.
def draw():
"""Draw letters."""
clear()
for target, letter in zip(targets, letters):
goto(target.x, target.y)
write(letter, align='center', font=('Consolas', 20, 'normal'))
update()
● draw(): Clears the screen and redraws the letters at their current positions. Moving the Letters
def move():
"""Move letters."""
if randrange(20) == 0:
x = randrange(-150, 150)
target = vector(x, 200)
targets.append(target)
letter = choice(ascii_lowercase)
letters.append(letter)
for target in targets:
target.y-= 1
draw()
for target in targets:
if not inside(target):
return
ontimer(move, 100)
● move(): Adds new letters to the screen at random intervals, moves existing letters
downward, and redraws them. It sets up a timer to call itself repeatedly.
4. Handling Key Presses
def press(key):
"""Press key."""
global score
if key in letters:
score += 1
pos = letters.index(key)
del targets[pos]
del letters[pos]
else:
score-= 1
print('Score:', score)
● press(key): Increases the score if the correct letter is typed and removes it from the
screen. Decreases the score for incorrect key presses.
5. Setting Up the Game
setup(420, 420, 370, 0)
hideturtle()
up()
tracer(False)
listen()
for letter in ascii_lowercase:
onkey(lambda letter=letter: press(letter), letter)
move()
done()
● setup(): Initializes the graphics window with a width of 420 and height of 420.
● hideturtle(): Hides the turtle cursor for a cleaner look.
● up():Lifts the turtle to avoid drawing unnecessary lines.
● tracer(False): Disables automatic screen updates for better performance.
● listen(): Prepares the window to listen for key presses.
● onkey(): Binds each letter key to the press() function.
● move(): Starts the movement of letters.
● done(): Completes the setup and starts the game loop.
Get Discount on Top Educational Courses
Source Code :
from random import choice, randrange
from string import ascii_lowercase
from turtle import *
from freegames import vector
targets = []
letters = []
score = 0
def inside(point):
"""Return True if point on screen."""
return -200 < point.x < 200 and -200 < point.y < 200
def draw():
"""Draw letters."""
clear()
for target, letter in zip(targets, letters):
goto(target.x, target.y)
write(letter, align='center', font=('Consolas', 20, 'normal'))
update()
def move():
"""Move letters."""
if randrange(20) == 0:
x = randrange(-150, 150)
target = vector(x, 200)
targets.append(target)
letter = choice(ascii_lowercase)
letters.append(letter)
for target in targets:
target.y -= 1
draw()
for target in targets:
if not inside(target):
return
ontimer(move, 100)
def press(key):
"""Press key."""
global score
if key in letters:
score += 1
pos = letters.index(key)
del targets[pos]
del letters[pos]
else:
score -= 1
print('Score:', score)
setup(420, 420, 370, 0)
hideturtle()
up()
tracer(False)
listen()
for letter in ascii_lowercase:
onkey(lambda letter=letter: press(letter), letter)
move()
done()
Output :
Running the code will open a graphical window where letters fall from the top. Players need to type the correct letters to score points. The game provides real-time feedback on the score and demonstrates a simple yet effective way to combine graphics and input handling in Python.
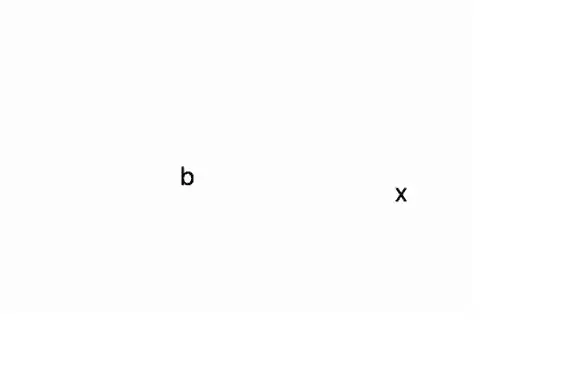
Reference
For more information on the turtle and freegames modules, check out their documentation:
● Turtle Graphics Documentation
Find More Projects
ludo game in python using gui and thinkter introduction The “ludo game in python“ project is a simplified digital version of the …
hotel management system in python introduction The Hotel Management System is a simple, interactive GUI-based desktop application developed using Python’s Tkinter library. …
cryptography app in python using gui introduction In the digital age, data security and privacy are more important than ever. From messaging …
portfolio generator tool in python using GUI introduction In today’s digital-first world, having a personal portfolio is essential for students, job seekers, …
interactive story game in python using gui introduction The Interactive story game in python using gUI is a visually rich, dynamic, and engaging …
maze generator using Python with Pygame Module GUI introduction In this maze generator using Python, realm of logic, problem-solving, and computer science, …