YouTube Clone Using HTML, CSS, JavaScript and ReactJs With Source Code
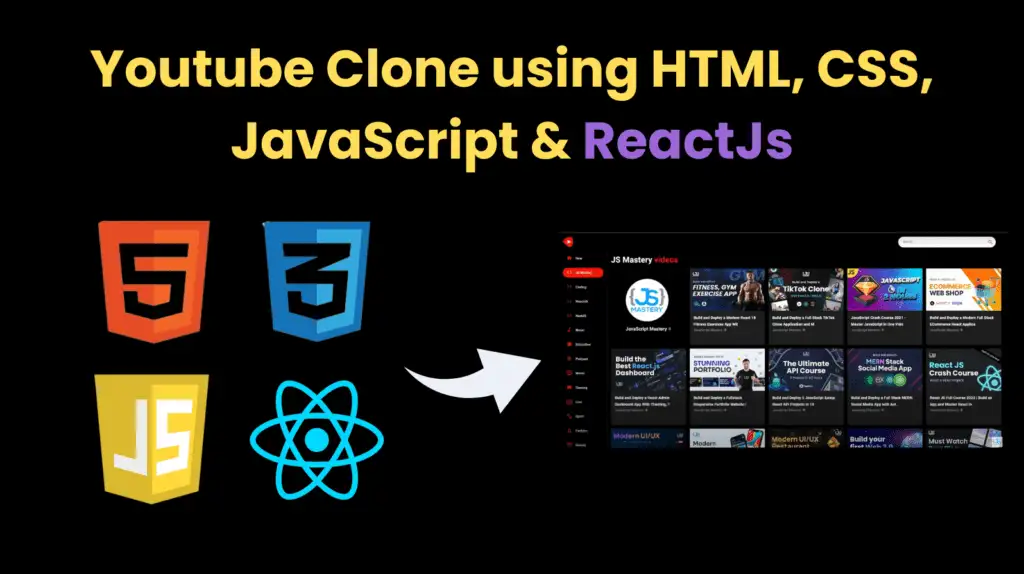
Introduction :
This project is a YouTube clone built using React.js, a popular JavaScript library for building user interfaces, especially single-page applications. The clone mimics the core functionalities of YouTube, including video playback, channel viewing, searching for videos, and a dynamic feed. It utilizes the react-router-dom
library for client-side routing and axios
for making HTTP requests to the YouTube API.
Explanation :
Project Structure
- App Component: The core component that sets up the routing structure of the application.
- Global Styles: Defined in
index.css
to ensure consistent styling across the application. - Entry Point:
index.js
serves as the entry point for the React application. - Utility Constants: Defined in
utils/constants.js
to store static data such as categories and demo URLs. - API Fetching: Handled in
fetchFromApi.js
usingaxios
to interact with the YouTube API.
App Component:
- BrowserRouter: This component is used to wrap the entire application, enabling the use of React Router for navigation.
- Box: A Material UI component used to style the container. In this case, it sets the background color.
- Routes: Defines the various routes in the application and the corresponding components that should be rendered for each route.
- Navbar: The navigation bar component, which is always displayed.
- Feed: Displays the main feed of videos on the home page (
/
). - VideoDetail: Shows the details of a specific video when the user navigates to
/video/:id
. - ChannelDetail: Displays details of a specific channel when the user navigates to
/channel/:id
. - SearchFeed: Shows search results when the user performs a search (
/search/:searchTerm
).
Global Styles (index.css) :
- Basic Styling: Resets default margins and paddings and applies a box-sizing rule to ensure padding and borders are included in the element’s total width and height.
- Link Styling: Removes underline and sets the default color for links.
- Scrollbar Styling: Customizes the appearance of the scrollbar.
- Category Button Styling: Defines styles for category buttons, including hover effects and responsive behavior for different screen sizes.
- Responsive Design: Ensures the application is responsive and adjusts elements like the search bar and video player based on the screen size.
Entry Point (index.js)
- ReactDOM: Renders the
App
component into the root DOM element. - CSS Import: Imports the global CSS styles.
Utility Constants (utils/constants.js):
- Icons: Imports various Material UI icons used throughout the application.
- Logo: URL of the application’s logo.
- Categories: An array of category objects, each with a name and an icon. This data is used to display different categories of videos.
- Demo Data: URLs and titles for demo videos and channels, used as placeholders or defaults within the application.
API Fetching (fetchFromApi.js):
- axios: A promise-based HTTP client used to make requests to the YouTube API.
- BASE_URL: The base URL for the YouTube API endpoints.
- Options: Configuration for the API request, including query parameters and headers with the API key.
- fetchFromAPI Function: An asynchronous function that makes a GET request to a specified endpoint of the YouTube API and returns the fetched data.
Purpose of Functions:
- App Component: Manages the overall structure of the application and the routing between different components.
- fetchFromAPI: Centralizes the logic for fetching data from the YouTube API, ensuring that API requests are made consistently throughout the application.
Additional Components:
- Navbar: Contains the navigation elements and possibly a search bar.
- Feed: Displays a list or grid of videos, likely fetched from the API.
- VideoDetail: Shows detailed information about a selected video, including the video player, description, and related videos.
- ChannelDetail: Displays information about a specific channel, including channel videos and possibly subscriber information.
- SearchFeed: Displays search results based on the user’s query.
Instructions to Run this Project :
Step 01 : Download the zip file of source code (given below) and extract it.
Step 02 : Navigate to the Project Directory:
- Once the repository is cloned, navigate into the project directory using the
cd
command: cd <project_directory> - Replace
<project_directory>
with the name of the directory where the project was cloned.
Step 03 : Install Dependencies:
- Inside the project directory, run the following command to install project dependencies using Yarn : npm install
- This command will read the
package.json
file and install all required dependencies listed in it.
Step 04 : Run the Project:
- After installing dependencies, you can start the development server using the following command: npm start
- This command will start the development server and automatically open the project in your default web browser.
- Once the development server is up and running, you can access the project by opening your web browser and navigating to the specified address (usually
http://localhost:3000
).
Conclusion:
This YouTube clone project showcases the use of React.js for building a single-page application with dynamic routing and API interaction. It leverages modern web development practices and tools like react-router-dom
for navigation, axios
for HTTP requests, and Material UI for styling and icons. By organizing the application into well-defined components and utilities, it maintains a clean and scalable codebase.
Get Discount on Top Educational Courses
Source Code :
Output :
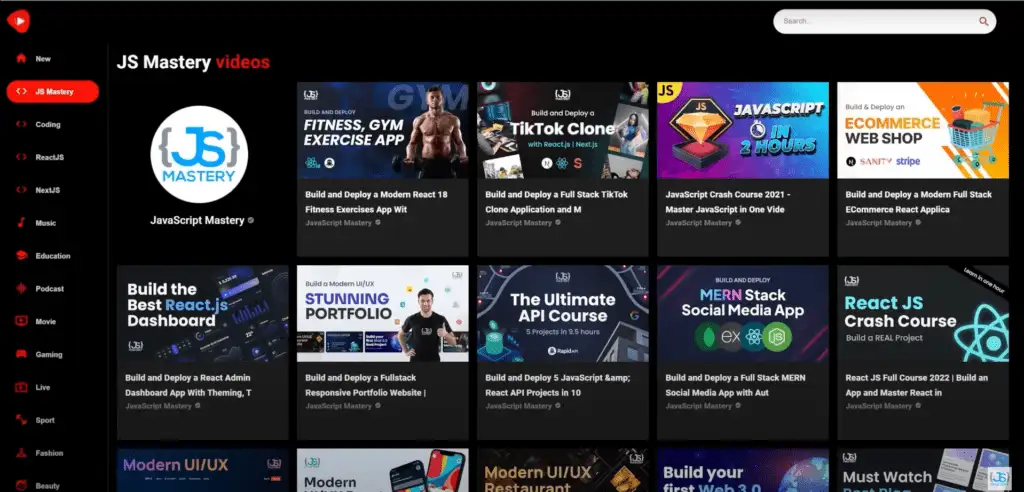
Find More Projects
MathGenius Pro – AI-Powered Math Solver Using Python Introduction: From simple arithmetic to more complicated college-level subjects like integration, differentiation, algebra, matrices, …
CipherMaze: The Ultimate Code Cracking Quest Game Using Python Introduction: You can make CipherMaze, a fun and brain-boosting puzzle game, with Python …
Warp Perspective Using Open CV Python Introduction: In this article, we are going to see how to Create a Warp Perspective System …
Custom AI Story Generator With Emotion Control Using Python Introduction: With the help of this AI-powered story generator, users can compose stories …
AI Powered PDF Summarizer Using Python Introduction: AI-Powered PDF Summarizer is a tool that extracts and summarizes research papers from ArXiv PDFs using Ollama (Gemma 3 LLM). The …
AI Based Career Path Recommender Using Python Introduction: One of the most significant and frequently perplexing decisions in a person’s life is …