Facebook Messenger Using HTML, CSS, JavaScript and ReactJs With Source Code
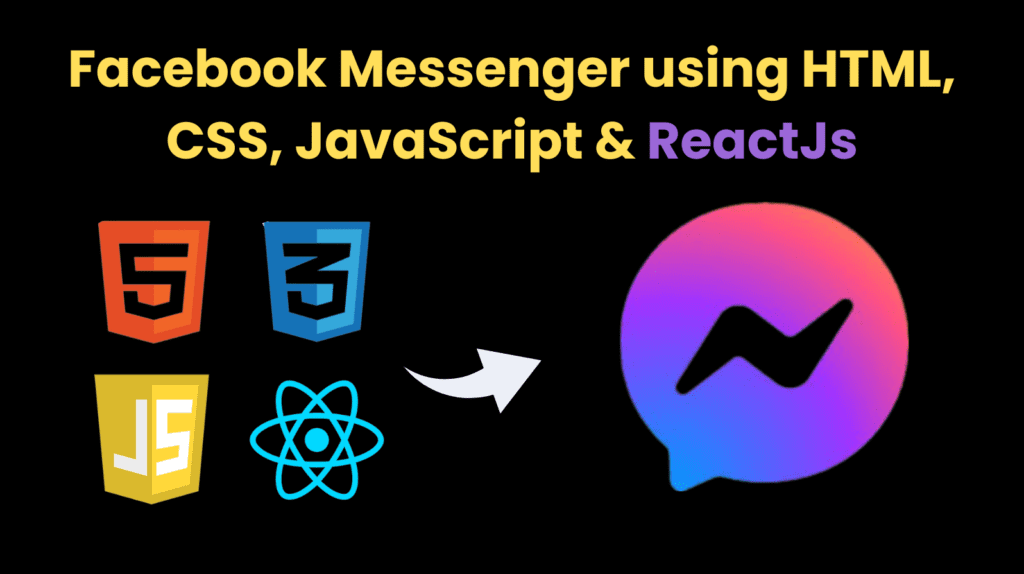
Introduction :
The project is a simplified clone of Facebook Messenger, built using React.js. The application includes basic functionalities such as login (via Facebook and Google), a chat interface, and navigation between different parts of the app. This clone is a good example of a single-page application (SPA) that leverages React’s component-based architecture to create a responsive and interactive user experience.
Explanation :
Project Structure
The project is organized into several key files and directories:
- index.js: The entry point of the application.
- index.css: Global styles and specific styles for various components.
- App.js: The main application component that sets up routing.
- components: A directory (not shown but implied) where other components like Chats, Login, and context providers reside.
index.js
This file serves as the entry point for the React application. It renders the root component (App
) into the DOM.
Key Points:
- Uses
ReactDOM.render
to mount theApp
component. - Wraps the
App
component inReact.StrictMode
for highlighting potential problems in the application.
index.css
This file contains global CSS styles and specific styles for different pages and components.
Key Points:
- Global styles ensure consistent font usage across the application.
- Specific styles are defined for the chat page and login page to handle layout and design.
- Custom scrollbar styles to improve the user experience.
App.js
This is the main component of the application. It sets up routing using react-router-dom
.
Key Points:
- Uses
BrowserRouter
for routing. - Contains a
Switch
component withRoute
components to define different routes (commented out in the provided code but typically includes paths for chats and login). - The commented-out
AuthProvider
suggests there is an authentication context to handle user authentication.
3. JavaScript Logic and Components
The project’s logic is divided into various components and context providers.
React Router
The react-router-dom
library is used to manage routing within the application. It allows for navigation between different components without refreshing the page.
Key Points:
BrowserRouter
is the router implementation for HTML5 browsers.Switch
renders the first child<Route>
or<Redirect>
that matches the location.Route
is used to define individual routes for different components.
AuthProvider (Commented Out)
The AuthProvider
(presumably defined in ../contexts/AuthContext
) would provide authentication context to the entire application.
Key Points:
- Manages user authentication state.
- Provides authentication functions and data to other components via React Context.
Chats and Login Components (Commented Out)
These components are not shown but are implied to exist in the components
directory.
Chats Component:
- Displays the chat interface.
- Fetches and displays messages.
- Allows users to send new messages.
Login Component:
- Provides a login interface with options for Facebook and Google authentication.
- Handles user authentication and redirects to the chat interface upon successful login.
4. CSS Styling
The index.css
file provides styling for the entire application, ensuring a cohesive and visually appealing design.
Global Styles:
- Applies a universal font family for consistency.
Chats Page Styles:
.chats-page
: Positions the chat page to cover the entire viewport..nav-bar
: Styles the navigation bar at the top of the chat page..logo-tab
and.logout-tab
: Position and style the logo and logout elements.
Login Page Styles:
#login-page
: Sets the background gradient for the login page.#login-card
: Centers the login card and styles it with padding, border-radius, and text alignment..login-button
: Styles the login buttons for Facebook and Google.
5. Purpose of Functions and Logic
The functions and logic in this application serve to manage routing, user authentication, and component rendering.
ReactDOM.render:
- Initializes the application by rendering the
App
component into the DOM.
App Component:
- Manages routing using
react-router-dom
. - Conditionally renders components based on the URL path.
Conclusion :
This Facebook Messenger clone project demonstrates the use of React.js for building a responsive, component-based single-page application. The structure and logic of the application emphasize best practices in React development, such as component reusability, context for state management, and efficient routing. The provided CSS ensures a consistent and attractive user interface, enhancing the overall user experience.
Source Code :
Output :
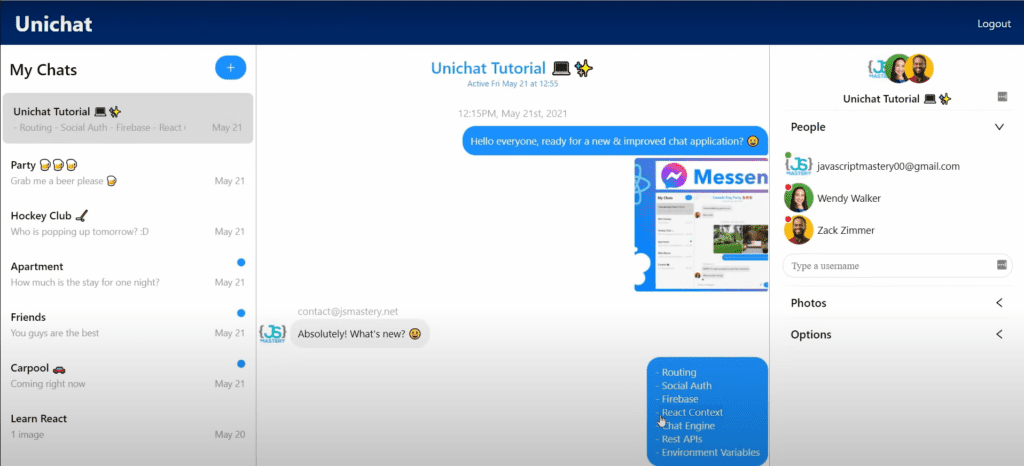
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello friends, welcome to today’s new blog. Today’s project is going to …
Number Guessing Game Using HTML CSS And JavaScript Introduction Hello coders, you might have played various games, but were you aware that …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …