WhatsApp Web UI Clone Using HTML, CSS & JavaScript With Source Code
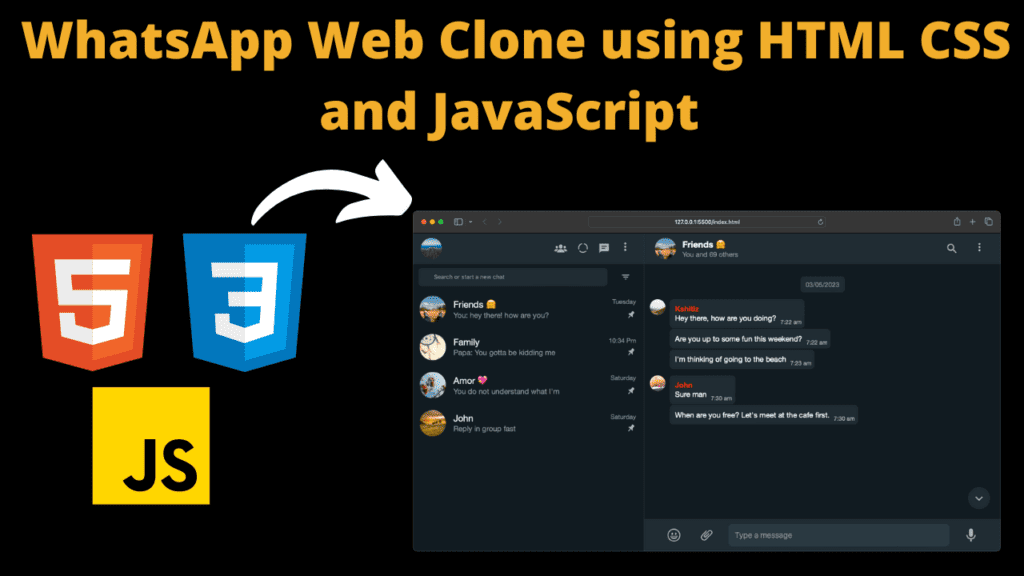
Introduction :
The WhatsApp Web UI clone project aims to replicate the user interface of the popular messaging platform, WhatsApp Web, using only HTML ,CSS and JavaScript. This project provides an opportunity to understand and implement various UI components such as sidebars, chat windows, and message elements that are commonly used in modern web applications. The primary focus is on achieving a visually similar layout and style, rather than functionality.
Explanation :
Project Structure :
The project is structured into two primary sections: the sidebar and the chat window. Each section has distinct roles and elements that contribute to the overall functionality and appearance of the interface.
Sidebar :
Overview: The sidebar is a critical component of the WhatsApp Web interface. It contains user-related elements and provides navigation capabilities. The sidebar allows users to view their profile, access various tools, search for chats, and navigate through a list of conversations.
Elements:
- Profile Image: The user’s profile picture is displayed at the top of the sidebar, providing a personalized touch.
- Toolbar: A set of icons for accessing communities, status updates, starting new chats, and a dropdown menu for additional options.
- Search Bar: Allows users to search for specific chats or contacts.
- Connectivity Notification: Displays a warning message if the computer is not connected to the internet, ensuring the user is aware of their connection status.
- Chats List: Displays a list of chat tiles, each representing a conversation with a contact or group.
Design Considerations:
- Flexbox Layout: The sidebar utilizes flexbox to align and distribute space among items.
- Hover Effects: Interactive elements like the chat tiles and toolbar icons have hover effects to enhance user experience.
- Color Scheme: The sidebar uses a consistent color scheme to match the overall theme of the application, with primary and secondary colors for different elements.
Chat Window :
Overview: The chat window is the primary area where conversations take place. It displays the messages of the active chat and provides tools for composing and sending new messages.
Elements:
- Chat Header: Displays the profile image and name of the active chat, along with additional options like search and a menu dropdown.
- Chat Contents: Contains the messages of the active chat, organized in message groups by sender.
- Message Group: Each message group includes messages from a single sender, displayed in chronological order.
- Footer: Contains input elements and icons for composing and sending new messages, as well as attaching files and sending voice messages.
Design Considerations:
- Scrollable Area: The chat contents area is designed to be scrollable, allowing users to navigate through long conversations easily.
- Flexbox Layout: The chat window uses flexbox for arranging elements, ensuring a responsive and flexible layout.
- Message Styling: Individual messages are styled with padding, background colors, and rounded corners to mimic the appearance of chat bubbles.
JavaScript Logic
Although the primary focus of this project is on HTML and CSS, JavaScript is used to add interactivity and enhance user experience. The provided scripts include:
Scroll-to-Top Functionality:
- A scroll-to-top button allows users to quickly scroll to the top of the chat window. JavaScript is used to handle the scroll events and show/hide the button based on the scroll position.
Connectivity Check:
- JavaScript is used to monitor the internet connection status. If the computer is not connected to the internet, a notification is displayed to the user. This is achieved using the
navigator.onLine
property and event listeners for connection changes.
- JavaScript is used to monitor the internet connection status. If the computer is not connected to the internet, a notification is displayed to the user. This is achieved using the
Purpose of Functions :
Scroll-to-Top Functionality:
- scrollToTop() Function: Smoothly scrolls the chat window to the top when the scroll-to-top button is clicked. Enhances navigation by allowing users to quickly access the beginning of the conversation.
- Event Listeners: Handle scroll events to determine when to show or hide the scroll-to-top button based on the current scroll position.
Connectivity Check:
- checkConnectivity() Function: Checks the internet connection status and updates the connectivity notification accordingly. Ensures that users are aware of their connection status and can take appropriate action if needed.
- Event Listeners: Monitor changes in the connection status and update the UI in real-time to reflect the current state.
Conclusion :
This WhatsApp Web UI clone project provides a detailed look at how a complex user interface can be constructed using HTML and CSS. By breaking down the project into its core components and understanding the design and functionality of each element, developers can gain valuable insights into modern web design principles. The use of JavaScript to add interactivity further enhances the user experience, making this project a comprehensive learning exercise in front-end development.
Source Code :
index.html
style.css
body {
background-color: var(--bg-color);
}
main {
display: flex;
align-items: stretch;
height: 100%;
}
.avatar {
border-radius: 50%;
}
.icon {
padding: 8px;
cursor: pointer;
filter: invert(79%) sepia(18%) saturate(148%) hue-rotate(158deg) brightness(91%) contrast(88%);
}
.pin {
filter: invert(79%) sepia(18%) saturate(148%) hue-rotate(158deg) brightness(91%) contrast(88%);
}
.hidden {
display: none !important;
}
.dropdown {
position: relative;
}
.dropdown-content {
display: none;
position: absolute;
padding: 10px 0px;
width: 195px;
background-color: var(--dropdown-color);
z-index: 10;
border-radius: 3px;
/* display: flex; */
flex-direction: column;
box-shadow: 0px 8px 16px 0px rgba(0, 0, 0, 0.2);
}
.dropdown-content a {
padding: 10px 16px;
color: var(--text-primary);
}
.dropdown-content a:hover {
background-color: var(--bg-color);
}
.dropdown:hover .dropdown-content {
display: flex;
}
chat-message.css
.chat-message-group {
display: flex;
color: white;
align-items: flex-start;
column-gap: 8px;
margin-bottom: 10px;
}
.emoji-toolbar {
/* visibility: none; */
/* opacity: 0; */
position: relative;
}
.reaction-emoji-selector {
position: absolute;
top: -40px;
height: 60px;
display: flex;
display: none;
justify-content: space-between;
align-items: center;
font-size: 24px;
z-index: 10;
border-radius: 50px;
background-color: var(--primary);
/* bottom: 10px; */
}
.chat-messages {
flex-grow: 1;
display: flex;
flex-direction: column;
align-items: flex-start;
}
.chat-message-container {
flex-grow: 1;
display: flex;
column-gap: 6px;
align-items: center;
}
.chat-message-container img {
display: none;
}
.chat-message-container:hover img {
display: flex;
}
.chat-message {
padding: 10px;
margin-bottom: 2px;
background-color: var(--primary);
border-radius: var(--border-radius);
/* position: relative; */
}
.chat-message-sender {
font-weight: 600;
}
.chat-message-sender:nth-of-type(2n + 1) {
color: red;
}
.chat-message-time {
font-size: 11px;
color: #ffffff99;
/* margin-top: ; */
position: relative;
bottom: -6px;
right: -4px;
}
.chat-message-avatar {
height: 30px;
border-radius: 50%;
}
chat-tile.css
.chat-tile {
display: flex;
color: var(--text-secondary);
height: 72px;
position: relative;
margin-bottom: 0px;
/* padding: 12px; */
column-gap: 10px;
align-items: center;
}
.chat-tile:hover {
background-color: var(--primary);
cursor: pointer;
}
.chat-tile>img {
height: 49px;
width: 49px;
border-radius: 50%;
margin: 0px 5px 0px 13px;
}
.chat-tile::after {
content: "";
position: absolute;
left: 0;
bottom: 0px;
width: 100%;
height: 0.1px;
background-color: var(--border-color);
}
.chat-tile-details {
flex-grow: 1;
padding-right: 15px;
}
.chat-tile-title {
display: flex;
justify-content: space-between;
}
.chat-tile-title> :first-child {
color: var(--text-primary-strong);
font-size: 17px;
}
.chat-tile-title> :nth-child(2) {
font-size: 12px;
}
.chat-tile-subtitle {
display: flex;
white-space: nowrap;
/* max-width: 100%; */
/* overflow: hidden; */
/* text-overflow: ellipsis; */
justify-content: space-between;
}
.chat-tile-menu {
/* display: none; */
}
.chat-tile:hover>.chat-tile-details>.chat-tile-subtitle~.chat-tile-menu {
display: inline-flexbox;
background-color: red;
}
chat-window.css
#chat-window {
flex-grow: 2;
display: flex;
flex-direction: column;
position: relative;
/* background: url(../images/chat-bg.png); */
}
#chat-window-header {
color: white;
display: flex;
align-items: center;
column-gap: 12px;
height: var(--header-height);
padding: 15px 20px;
background-color: var(--primary);
}
.contact-menu {
position: absolute;
right: 5px;
}
#active-chat-details {
flex-grow: 1;
}
#chat-window-contents {
flex-grow: 1;
position: relative;
z-index: 1;
overflow: auto;
padding: 10px;
}
/* #chat-window-contents::before {
content: "";
position: absolute;
background-color: rgba(0, 0, 0, 0.9);
width: 100%;
height: 100%;
z-index: -1;
} */
#compose-chat-box {
margin: 0px 10px;
background-color: var(--compose-bg-color);
padding-left: 12px;
font-size: 15px;
}
#chat-window-footer {
display: flex;
/* column-gap: 10px; */
height: 62px;
width: 100%;
padding: 10px 25px;
background-color: var(--primary);
}
#active-chat-details>.info {
color: var(--text-secondary);
font-size: 13px;
}
.datestamp-container {
display: flex;
justify-content: center;
align-items: center;
}
.datestamp {
color: var(--text-secondary);
font-size: 12.5px;
background-color: var(--primary);
border-radius: var(--border-radius);
padding: 8px 10px;
margin: 13px 0px;
}
.scroll-to-top-button {
border-radius: 50%;
height: 42px;
width: 42px;
background-color: var(--primary);
position: absolute;
bottom: 80px;
right: 20px;
transition: all 0.1s ease-in-out;
z-index: 3;
display: flex;
justify-content: center;
align-items: center;
}
.scroll-to-top-button-icon {
height: 35px;
width: 35px;
}
.shrink {
height: 0;
width: 0;
}
core.css
:root {
--bg-color: #111b21;
--color-1: #06cf9c;
--color-1: #53bdeb;
--color-1: #00a884;
--green: #005c4b;
--green-secondary: #025144;
--light-grey: #2a3942;
--primary: #202c33;
--color-1: #1d282f;
--bg-color-secondary: #182229;
--color-1: #111b21;
--color-1: #101a20;
--color-1: #0b141a;
--color-1: #0b141a59;
--color-1: #000000b3;
--text-primary: #d1d7db;
--text-primary-strong: #e9edef;
--text-secondary: #8696a0;
--search-input-background: var(--primary);
--border-color: var(--light-grey);
--compose-bg-color: var(--light-grey);
--input-placeholder-color: var(--text-secondary);
--dropdown-color: var(--primary);
--border-radius: 0.5rem;
--header-height: 59px;
--footer-height: 62px;
--searchbar-border-radius: var(--border-radius);
}
::-webkit-scrollbar {
width: 5px;
}
::-webkit-scrollbar-thumb {
background-color: var(--primary);
}
::-webkit-scrollbar-track {
background-color: transparent;
}
* {
margin: 0;
padding: 0;
box-sizing: border-box;
/* border: solid 0.2px pink; */
}
html,
body {
font-family: "Segoe UI", "Helvetica Neue", Helvetica, "Lucida Grande", Arial,
Ubuntu, "Cantarell", "Fira Sans", sans-serif;
font-size: 14px;
height: 100%;
}
input[type="search"] {
border: none;
outline: none;
flex-grow: 1;
color: var(--text-primary);
background-color: var(--primary);
border-radius: var(--searchbar-border-radius);
}
input[type="search"]::-webkit-input-placeholder,
input[type="search"]::placeholder {
color: var(--input-placeholder-color);
}
input[type="search"]::-webkit-search-cancel-button {
/* Remove default */
-webkit-appearance: none;
height: 10px;
width: 10px;
background: red;
cursor: pointer;
}
ul>li {
list-style: none;
}
a {
text-decoration: none;
color: inherit;
}
sidebar.css
#sidebar {
flex-grow: 1;
max-width: 40%;
display: flex;
flex-direction: column;
border-right: solid 1px var(--border-color);
}
#sidebar-header {
display: flex;
justify-content: space-between;
align-items: center;
height: var(--header-height);
background-color: var(--primary);
padding: 20px 15px;
}
#profile-image {
height: 40px;
}
.toolbar {
/* width: 190px; */
display: flex;
justify-content: space-between;
}
#search-toolbar {
display: flex;
column-gap: 10px;
height: 49px;
padding: 7px 10px;
}
#search-input {
padding-left: 30px;
}
#sidebar-contents {
flex-grow: 1;
overflow: auto;
display: flex;
flex-direction: column;
}
.connectivity-notification {
padding: 12px 15px;
background-color: var(--bg-color-secondary);
display: flex;
align-items: center;
column-gap: 15px;
color: var(--text-secondary);
}
.connectivity-notification-title {
color: var(--text-primary-strong);
font-size: 16px;
}
.connectivity-notification > img {
filter: invert(82%) sepia(49%) saturate(1077%) hue-rotate(328deg)
brightness(103%) contrast(103%);
}
check-connectivity.js
const connectivityNotification = document.getElementsByClassName(
"connectivity-notification"
)[0];
connectivityNotification.classList.add("hidden");
window.addEventListener("online", () => {
connectivityNotification.classList.add("hidden");
console.log(connectivityNotification.classList);
console.log("online");
});
window.addEventListener("offline", () => {
connectivityNotification.classList.remove("hidden");
console.log("offline");
});
scroll-to-top.js
const chatWindowContents = document.getElementById("chat-window-contents");
const scrollToTopButton = document.getElementsByClassName(
"scroll-to-top-button"
)[0];
const scrollToTopButtonIcon = document.getElementsByClassName(
"scroll-to-top-button-icon"
)[0];
chatWindowContents.addEventListener("scroll", (event) => {
const scrollPositionFromTop = chatWindowContents.scrollTop;
const scrollFromBottom =
chatWindowContents.scrollHeight -
scrollPositionFromTop -
chatWindowContents.clientHeight;
if (scrollFromBottom === 0) {
scrollToTopButton.classList.add("shrink");
scrollToTopButtonIcon.classList.add("shrink");
} else {
scrollToTopButton.classList.remove("shrink");
scrollToTopButtonIcon.classList.remove("shrink");
}
});
scrollToTopButton.addEventListener("click", (event) => {
event.preventDefault();
chatWindowContents.scrollTop =
chatWindowContents.scrollHeight - chatWindowContents.clientHeight;
});
Output :
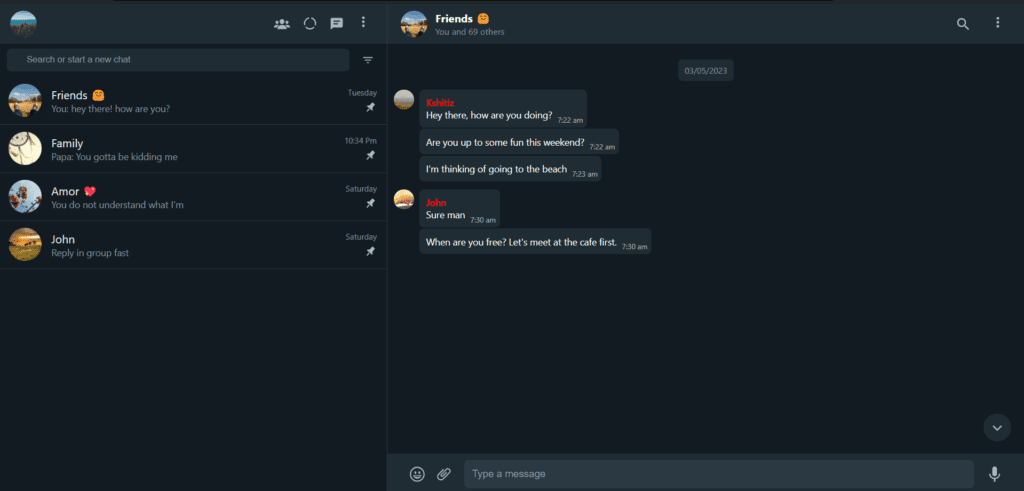
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Drawing Ganesha Using Python Turtle Graphics[Drawing Ganapati Using Python] Introduction In this blog post, we will learn how to draw Lord Ganesha …
Contact Management System in Python with a Graphical User Interface (GUI) Introduction: The Contact Management System is a Python-based application designed to …
KBC Game using Python with Source Code Introduction : Welcome to this blog post on building a “Kaun Banega Crorepati” (KBC) game …
Basic Logging System in C++ With Source Code Introduction : It is one of the most important practices in software development. Logging …
Snake Game Using C++ With Source Code Introduction : The Snake Game is one of the most well-known arcade games, having its …
C++ Hotel Management System With Source Code Introduction : It is very important to manage the hotels effectively to avail better customer …