Interior Design Website using HTML CSS and JavaScript
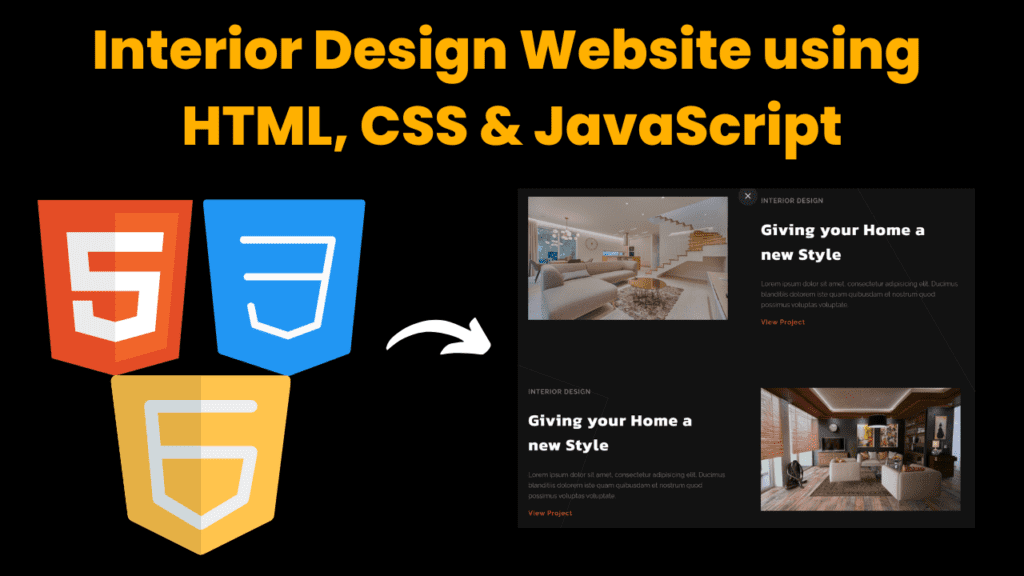
Introduction :
This project is an interior design website, created for showing the services, portfolio, and personal brand of an interior designer named “Ann Smith.” It is built using HTML for structure, CSS for styling, and JavaScript to add dynamic content. The design of the website focus on delivering a clean, professional, and engaging user experience.
The website includes sections such as a landing page, navigation menu, an introduction to the designer, and service offerings. Interactive elements like a hamburger menu for mobile navigation and smooth animations are incorporated for better usability. This website can be used in your business to grow your online presence by showing your designs
Structure :
Main Sections:
Container and Rectangles: The main container holds the content with decorative rectangles for an aesthetically pleasing background. These elements don’t affect the usability directly but contribute to the visual appeal.
Navigation Bar: Includes the designer’s name, profile image, and a responsive hamburger menu for navigation, leading to various sections of the website.
Landing Section: Displays an introductory banner with a smooth sliding animation to welcome users.
About Section: Introduces the designer with a brief bio and social media links.
Services Section: Showcases the various services offered by the designer.
HTML Structure
The HTML is structured with semantic tags to clearly differentiate between different parts of the website:
Container Div: This <div>
wraps all the content, ensuring that everything on the page stays within the layout.
Navigation: The <nav>
element defines the navigation bar that includes the designer’s information and a hamburger menu icon.
Sections:
- Landing: The
<section>
tag here defines the landing area, which serves as the hero section with an introduction and title. - About: Contains information about the designer along with social media and contact details.
- Services: Another section displaying the services the designer offers.
- Landing: The
Key CSS Components:
Rectangle Decoration: The rectangular elements are purely decorative, positioned at angles to give a unique, dynamic background without obstructing content.
Navigation Bar: The navigation is styled to be sleek with a designer profile image and a responsive hamburger menu. The navigation menu slides in from the right side of the screen when opened.
Hover Effects: The hamburger menu icon scales when hovered to give a subtle indication that it’s clickable.
Animations: Smooth transitions for the navigation menu and section changes during scroll enhance the user experience.
JavaScript Logic :
Purpose of JavaScript Functions
menuIcon Event Listener: This function adds a class navigate
when the hamburger menu is clicked, making the navigation menu visible.
xBtn Event Listener: This function removes the navigate
class when the “close” button (x-btn
) is clicked, hiding the navigation menu.
Scroll Event Listener: This function monitors how far the user has scrolled down the page. Based on the scroll position, it triggers animations for different sections like about
, services
, and portfolio
by adding a class that changes their appearance.
startCount Function: This function is responsible for creating a counting animation for elements with a data-val
attribute. As the user scrolls down to the statistics section, the function increments the displayed number from 0 to the specified value (data-val
) at an interval of 5 milliseconds, providing a smooth transition effect.
Get Discount on Top Educational Courses
Source Code :
HTML (index.html)
Interior Designer Website
Hello I am
Ann Smith
I'm from London
An Interior Designer
About
Hi! I'm Ann Smith
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Quos
enim nesciunt magnam deserunt in necessitatibus id quisquam,
voluptate repellat vero!
Contact me here:
Email: annsmith@design.com
Phone: 000 (123) 456 7890
What I do
My Services
Space Planning
Furniture Layout
Surface Selection
Lighting Design
Interior Detailing
Installation
Color Selection
Window Treatments
Constructor Documentations
Portfolio
Checkout My Work
Interior Design
Giving your Home a new Style
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Ducimus blanditiis dolorem iste quam quibusdam et nostrum quod
possimus voluptas voluptate.
View Project
Interior Design
Giving your Home a new Style
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Ducimus blanditiis dolorem iste quam quibusdam et nostrum quod
possimus voluptas voluptate.
View Project
Interior Design
Giving your Home a new Style
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Ducimus blanditiis dolorem iste quam quibusdam et nostrum quod
possimus voluptas voluptate.
View Project
Interior Design
Giving your Home a new Style
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Ducimus blanditiis dolorem iste quam quibusdam et nostrum quod
possimus voluptas voluptate.
View Project
Achievments
Let's Share My Achievments
Clients
0
Projects Done
0
Cups of Coffee
0
CSS (style.css)
/* Default Styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
text-decoration: none;
outline: none;
font-family: "Raleway", sans-serif;
}
html {
font-size: 62.5%;
}
/* End of Default Styles */
/* Container */
.container {
width: 100%;
height: 100%;
background-color: #121212;
position: relative;
/* overflow: hidden; */
}
.rectangle {
width: 70rem;
height: 40rem;
border: 0.1rem solid rgba(255, 255, 255, 0.1);
position: fixed;
}
.rectangle-1 {
top: 48%;
left: -21%;
transform: rotateZ(20deg);
}
.rectangle-2 {
top: -8%;
right: -10%;
transform: rotateZ(60deg);
}
/* Navigation */
.navbar {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 10rem;
z-index: 100;
}
.designer {
position: absolute;
top: 2rem;
left: 30rem;
display: flex;
align-items: center;
}
.designer div {
display: flex;
flex-direction: column;
text-align: center;
margin-left: 2rem;
}
.designer img {
width: 6rem;
height: 6rem;
object-fit: cover;
border-radius: 50%;
}
.designer div span:nth-child(1) {
font-size: 1.8rem;
font-weight: bold;
text-transform: uppercase;
color: #fff;
letter-spacing: 0.2rem;
}
.designer div span:nth-child(2) {
font-size: 1.4rem;
font-weight: bold;
text-transform: uppercase;
color: #999;
letter-spacing: 0.2rem;
}
.menu-icon {
position: absolute;
top: 3rem;
right: 30rem;
width: 5rem;
height: 3rem;
display: flex;
flex-direction: column;
justify-content: space-evenly;
cursor: pointer;
transition: transform 0.3s;
/* z-index: 100; */
}
.menu-icon:hover {
transform: scaleY(1.4);
}
.line {
width: 100%;
height: 0.2rem;
background-color: #fff;
}
.navigation {
width: 100%;
height: 100vh;
background-color: #121212;
position: fixed;
top: 0;
left: 0;
z-index: 100;
display: flex;
opacity: 0;
visibility: hidden;
transition: all 0.3s;
}
.navigation.navigate {
opacity: 1;
visibility: visible;
}
.nav-left {
width: 30%;
height: 100%;
}
.nav-left img {
width: 100%;
height: 100%;
object-fit: cover;
opacity: 0.5;
}
.nav-right {
width: 70%;
height: 100%;
}
.x-btn {
width: 4rem;
height: 4rem;
position: fixed;
top: 5rem;
right: 5rem;
cursor: pointer;
}
.x-line {
width: 4rem;
height: 0.2rem;
background-color: #fff;
}
.x-line-1 {
transform: rotateZ(-45deg);
}
.x-line-2 {
transform: rotateZ(45deg) translate(-0.2rem, -0.1rem);
}
.nav-right h1 {
font-family: "Kanit", sans-serif;
font-size: 5rem;
font-weight: 300;
text-transform: uppercase;
color: #fff;
position: absolute;
top: 20%;
left: 50%;
}
.nav-list {
display: flex;
flex-direction: column;
position: absolute;
top: 30%;
left: 50%;
}
.nav-list a {
font-family: "Kanit", sans-serif;
font-size: 4rem;
color: #ccc;
margin: 0.5rem 0;
transition: color 0.3s;
}
.nav-list a:nth-child(1) {
color: #c03740;
}
.nav-list a:hover {
color: #c03740;
}
/* End of Navigation */
/* Landing */
.landing {
width: 100%;
height: 100vh;
position: relative;
}
.banner {
position: absolute;
top: 35%;
left: 50%;
transform: translateX(-50%);
width: 100%;
height: 40rem;
text-align: center;
}
.heading-slide {
position: absolute;
width: 100%;
transform: translateZ(-8rem);
opacity: 0;
visibility: hidden;
}
.heading-slide-1 {
animation: animateHeading 8s infinite linear;
}
.heading-slide-2 {
animation: animateHeading 8s 4s infinite linear;
}
.heading-slide h3 {
font-size: 2rem;
text-transform: uppercase;
color: #999;
letter-spacing: 0.3rem;
margin-bottom: 2rem;
}
.heading-slide h1 {
font-size: 6rem;
font-weight: bold;
text-transform: uppercase;
color: #fff;
letter-spacing: 0.5rem;
}
@keyframes animateHeading {
0% {
transform: translateZ(-8rem);
opacity: 0;
visibility: hidden;
}
8% {
transform: translateZ(0);
opacity: 1;
visibility: visible;
}
48% {
transform: translateZ(0);
opacity: 1;
visibility: visible;
}
56% {
transform: translateZ(-8rem);
opacity: 0;
visibility: hidden;
}
100% {
transform: translateZ(-8rem);
opacity: 0;
visibility: hidden;
}
}
/* End of Landing */
/* About */
.about {
width: 100%;
height: 100vh;
display: flex;
justify-content: center;
padding-top: 10rem;
}
.about-left {
width: 40%;
position: relative;
}
.about-left img {
width: 33rem;
height: 55rem;
object-fit: cover;
position: absolute;
top: -10rem;
right: 0;
opacity: 0;
visibility: hidden;
transform: translateY(20rem);
transition: all 1s 0.5s;
}
.change .about-left img {
opacity: 0.8;
visibility: visible;
transform: translateY(0);
}
.about-right {
width: 60%;
position: relative;
opacity: 0;
visibility: hidden;
transform: translateY(15rem);
transition: all 1.5s;
}
.change .about-right {
opacity: 1;
visibility: visible;
transform: translateY(0);
}
.about-right h1 {
font-family: "Kanit", sans-serif;
font-size: 20rem;
font-weight: bold;
text-transform: uppercase;
letter-spacing: 0.3rem;
color: #222;
position: absolute;
top: -20rem;
left: 15rem;
}
.about-right h3 {
font-family: "Kanit", sans-serif;
font-size: 4rem;
font-weight: 300;
color: #fff;
position: absolute;
top: -7rem;
left: 25rem;
}
.info {
position: absolute;
top: 5rem;
left: 25rem;
}
.info p {
font-family: "Kanit", sans-serif;
font-size: 1.8rem;
width: 45rem;
color: #777;
line-height: 1.8;
margin-bottom: 5rem;
}
.social-media {
display: flex;
margin-bottom: 5rem;
}
.social-media i {
width: 6rem;
height: 6rem;
border-radius: 50%;
display: flex;
justify-content: center;
align-items: center;
font-size: 2.5rem;
background-color: #202020;
margin-right: 2rem;
color: #c03740;
cursor: pointer;
}
.info h4 {
font-size: 1.8rem;
color: #fff;
}
.info span {
font-size: 1.6rem;
font-weight: bold;
color: #777;
display: block;
margin: 2rem 0;
}
.info a {
color: #c03740;
font-family: "Kanit", sans-serif;
}
/* End of About */
/* Services */
.services {
width: 100%;
height: 70vh;
display: flex;
flex-direction: column;
align-items: center;
}
.services-header {
text-align: center;
opacity: 0;
visibility: hidden;
transform: translateY(5rem);
transition: all 1s;
}
.change .services-header {
opacity: 1;
visibility: visible;
transform: translateY(0);
}
.services-header h3 {
font-size: 2rem;
text-transform: uppercase;
color: #777;
letter-spacing: 0.3rem;
margin-bottom: 2rem;
}
.services-header h1 {
font-size: 5rem;
color: #fff;
letter-spacing: 0.3rem;
margin-bottom: 12rem;
}
.services-list {
width: 70%;
display: flex;
justify-content: space-evenly;
}
.list-item {
width: 30rem;
display: flex;
flex-direction: column;
align-items: center;
opacity: 0;
visibility: hidden;
transform: translateY(5rem);
}
.list-item:nth-child(1) {
transition: all 1s 0.5s;
}
.list-item:nth-child(2) {
transition: all 1s 0.7s;
}
.list-item:nth-child(3) {
transition: all 1s 0.9s;
}
.change .list-item {
opacity: 1;
visibility: visible;
transform: translateY(0);
}
.list-item i {
font-size: 4rem;
color: #c03740;
margin-bottom: 3rem;
}
.list-item span {
font-family: "Kanit", sans-serif;
font-size: 2rem;
color: #fff;
margin: 0.7rem 0;
}
/* End of Services */
/* Portfolio */
.portfolio {
width: 100%;
height: 100%;
padding: 20rem 0 5rem 0;
}
.portfolio-header {
text-align: center;
margin-bottom: 20rem;
opacity: 0;
visibility: hidden;
transform: translateY(5rem);
transition: all 1s;
}
.change .portfolio-header {
opacity: 1;
visibility: visible;
transform: translateY(0);
}
.portfolio-header h3 {
font-size: 2rem;
text-transform: uppercase;
color: #777;
letter-spacing: 0.3rem;
margin-bottom: 2rem;
}
.portfolio-header h1 {
font-size: 5rem;
color: #fff;
letter-spacing: 0.3rem;
margin-bottom: 12rem;
}
.works {
width: 80%;
margin: auto;
}
.work {
display: flex;
justify-content: space-evenly;
margin: 15rem 0;
opacity: 0;
visibility: hidden;
transform: translateY(10rem);
}
.work:nth-child(1) {
transition: all 1s 0.5s;
}
.work:nth-child(2) {
transition: all 1s 1s;
}
.work:nth-child(3) {
transition: all 1s 2s;
}
.work:nth-child(4) {
transition: all 1s 3s;
}
.change .work {
opacity: 1;
visibility: visible;
transform: translateY(0);
}
.work img {
width: 40%;
height: 30rem;
object-fit: cover;
opacity: 0.8;
}
.work-info {
width: 40%;
}
.work-info h3 {
font-size: 1.6rem;
color: #777;
text-transform: uppercase;
letter-spacing: 0.1rem;
}
.work-info h1 {
font-family: "Kanit", sans-serif;
font-size: 4rem;
font-weight: bold;
color: #fff;
letter-spacing: 0.2rem;
margin: 3rem 0;
}
.work-info p {
font-size: 1.6rem;
color: #777;
line-height: 1.6;
margin-bottom: 2rem;
}
.work-info a {
font-size: 1.6rem;
font-weight: bold;
color: #c03740;
letter-spacing: 0.1rem;
}
/* End of Portfolio */
/* Data */
.data {
width: 100%;
height: 100%;
padding: 20rem 0;
}
.data-header {
text-align: center;
}
.data-header h3 {
font-size: 2rem;
text-transform: uppercase;
color: #777;
letter-spacing: 0.3rem;
margin-bottom: 2rem;
}
.data-header h1 {
font-size: 5rem;
color: #fff;
letter-spacing: 0.3rem;
margin-bottom: 12rem;
}
.data-list {
width: 60%;
height: 90rem;
margin: auto;
position: relative;
}
.data-item {
position: absolute;
display: flex;
flex-direction: column;
}
.data-item:nth-child(1) {
top: 0;
left: 0;
}
.data-item:nth-child(2) {
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
.data-item:nth-child(3) {
bottom: 0;
right: 0;
}
.data-item span:nth-child(1) {
font-size: 2rem;
text-transform: uppercase;
letter-spacing: 0.2rem;
color: #777;
}
.data-item span:nth-child(2) {
font-family: "Kanit", sans-serif;
font-size: 20rem;
color: #c03740;
line-height: 1;
}
/* End of Data */
/* Footer */
footer {
width: 100%;
height: 50vh;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.footer-social-media {
display: flex;
margin-bottom: 3rem;
}
.footer-social-media i {
width: 5rem;
height: 5rem;
background-color: #262626;
border-radius: 50%;
display: flex;
justify-content: center;
align-items: center;
margin: 0 1rem;
font-size: 2rem;
color: #777;
}
footer h1 {
font-size: 4rem;
color: #fff;
letter-spacing: 0.2rem;
margin-bottom: 2rem;
}
footer a {
font-size: 2rem;
color: #ddd;
margin-bottom: 10rem;
}
.copyright {
font-size: 1.6rem;
color: #ccc;
}
.copyright span {
font-family: "Kanit", sans-serif;
font-size: 2rem;
color: #c03740;
}
/* End of Footer */
/* End of Container */
/* Responsive */
@media (max-width: 1500px) {
html {
font-size: 55%;
}
.rectangle-2 {
right: -15%;
}
}
@media (max-width: 1300px) {
html {
font-size: 50%;
}
.about-left {
width: 35%;
}
.about-right {
width: 65%;
}
.works {
width: 90%;
}
.data-list {
width: 70%;
}
}
@media (max-width: 1100px) {
html {
font-size: 45%;
}
.about-right h1 {
font-size: 15rem;
top: -18rem;
}
.about-right h3 {
font-size: 3.5rem;
top: -9rem;
}
.data-list {
height: 80rem;
}
.data-item span:nth-child(2) {
font-size: 16rem;
}
}
@media (max-width: 900px) {
.rectangle-1 {
left: -40%;
}
.rectangle-2 {
right: -25%;
}
.designer {
left: 15rem;
}
.menu-icon {
right: 15rem;
}
.nav-left {
width: 50%;
}
.nav-right {
width: 50%;
position: relative;
}
.nav-right h1 {
transform: translateX(-50%);
width: 100%;
text-align: center;
}
.nav-list {
transform: translateX(-50%);
text-align: center;
}
.about-left {
display: none;
}
.about-right {
width: 100%;
}
.about-right h1 {
left: 50%;
transform: translateX(-50%);
}
.about-right h3 {
left: 35%;
}
.info {
left: 50%;
transform: translateX(-50%);
}
.services-list {
width: 90%;
}
.works {
width: 100%;
}
.work-info {
width: 50%;
}
}
@media (max-width: 700px) {
html {
font-size: 40%;
}
.designer {
left: 8rem;
}
.menu-icon {
right: 8rem;
}
.heading-slide h1 {
font-size: 5rem;
}
.services {
height: 90vh;
}
.services-list {
align-items: center;
flex-direction: column;
}
.list-item {
margin-bottom: 3rem;
}
}
@media (max-width: 500px) {
.rectangle-1 {
left: -70%;
}
.rectangle-2 {
right: -50%;
}
.nav-left {
display: none;
}
.nav-right {
width: 100%;
}
.work {
align-items: center;
}
.work img {
width: 60%;
}
.work-info {
display: none;
}
.data-item span:nth-child(2) {
font-size: 12rem;
}
}
/* End of Responsive */
JavaScript (script.js)
const menuIcon = document.querySelector(".menu-icon");
const xBtn = document.querySelector(".x-btn");
const navigation = document.querySelector(".navigation");
menuIcon.addEventListener("click", () => {
navigation.classList.add("navigate");
});
xBtn.addEventListener("click", () => {
navigation.classList.remove("navigate");
});
let start = false;
window.addEventListener("scroll", () => {
const about = document.querySelector(".about");
const services = document.querySelector(".services");
const portfolio = document.querySelector(".portfolio");
const data = document.querySelector(".data");
const nums = document.querySelectorAll(".num");
if (window.pageYOffset >= 200) {
about.classList.add("change");
} else {
about.classList.remove("change");
}
if (window.pageYOffset >= about.offsetTop + 200) {
services.classList.add("change");
} else {
services.classList.remove("change");
}
if (window.pageYOffset >= services.offsetTop) {
portfolio.classList.add("change");
} else {
portfolio.classList.remove("change");
}
if (window.scrollY >= data.offsetTop - 300) {
if (!start) {
nums.forEach((num) => {
startCount(num);
});
}
start = true;
}
});
const startCount = (el) => {
let max = el.dataset.val;
let count = setInterval(() => {
el.textContent++;
if (el.textContent === max) {
clearInterval(count);
}
}, 5);
};
Output :
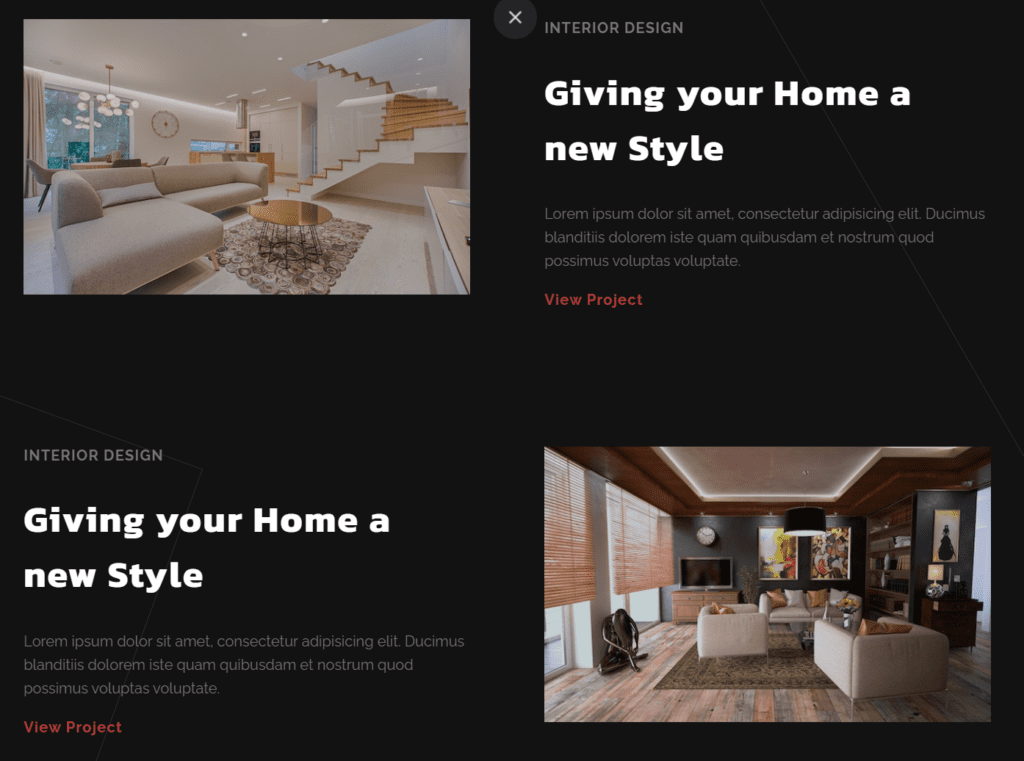
Disclaimer: The code provided in this post is sourced from GitHub and is distributed under the MIT License. All credits for the original code go to the respective owner. We have shared this code for educational purposes only. Please refer to the original repository for detailed information and any additional usage rights or restrictions.
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …
Typing Speed Test Game using Python Introduction: In this article, we will create a Typing Speed Test Game using Python and Tkinter. …
Inventory Management System Using Python Introduction The Inventory Management System is a Python-based project built using Tkinter, which provides a simple graphical …