Crypto Currency Tracker Using HTML, CSS, JavaScript and ReactJs With Source Code
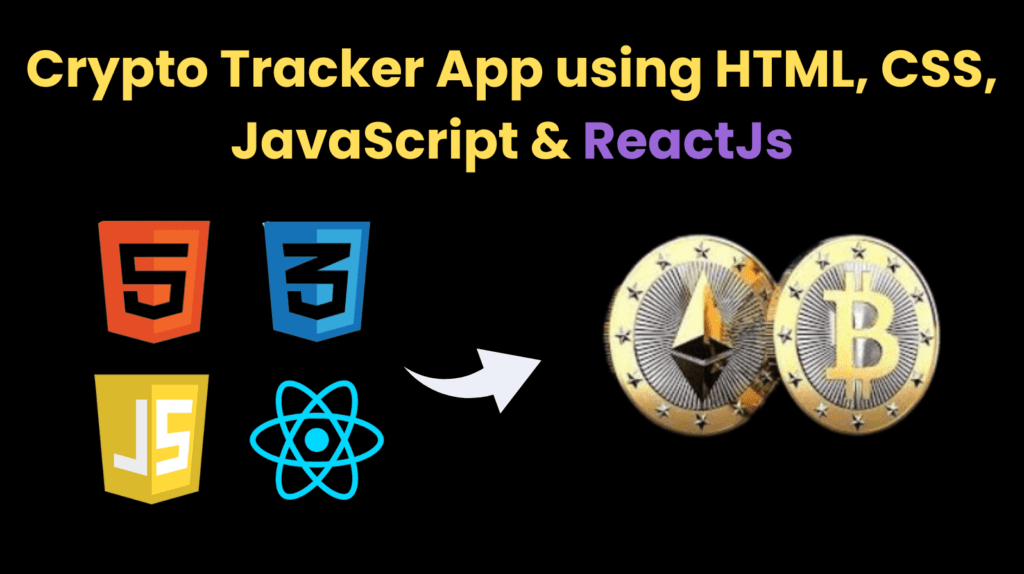
Introduction :
This cryptocurrency app is a web application built using React.js that allows users to track various cryptocurrencies, view detailed information about them, stay updated with the latest news, and explore different cryptocurrency exchanges. The app uses Redux for state management and several external libraries like Ant Design for UI components and Chart.js for visualizing data.
Explanation :
Structure :
App Component:
- Layout and Routing: The
App.js
component is the main entry point of the application. It sets up the overall layout using Ant Design’sLayout
component and manages navigation usingreact-router-dom
. - Components Integration: It includes the
Navbar
component for navigation, and dynamically renders different pages (likeHomepage
,Exchanges
,Cryptocurrencies
,CryptoDetails
, andNews
) based on the URL path.
- Layout and Routing: The
Index File:
- Application Bootstrapping: The
index.js
file initializes the app. It wraps theApp
component withProvider
fromreact-redux
to connect the Redux store andRouter
fromreact-router-dom
for routing. - Strict Mode: It also uses
React.StrictMode
to highlight potential problems in an application, ensuring best practices.
- Application Bootstrapping: The
Styles:
- Global Styles:
App.css
defines the global CSS styles, including variables for theming, layout rules, and responsive design to ensure the app looks good on various screen sizes.
- Global Styles:
State Management:
- Redux Store: The
store.js
file sets up the Redux store usingconfigureStore
from@reduxjs/toolkit
. It combines reducers from thecryptoApi
andcryptoNewsApi
services, enabling the app to manage state effectively and make API requests.
- Redux Store: The
CryptoDetails Component:
- Fetching Data:
CryptoDetails.js
fetches detailed information about a specific cryptocurrency using theuseGetCryptoDetailsQuery
anduseGetCryptoHistoryQuery
hooks fromcryptoApi
. - State and UI: It manages local state (e.g., selected time period for historical data) using React’s
useState
and displays the data using Ant Design’sTypography
,Select
, and other components. It shows various statistics, historical data in a line chart, and links related to the cryptocurrency.
- Fetching Data:
Cryptocurrencies Component:
- Listing and Searching:
Cryptocurrencies.js
lists cryptocurrencies and includes a search functionality. It fetches the data usinguseGetCryptosQuery
, manages local state for the search term and filtered cryptocurrencies, and renders the list using Ant Design’sCard
andRow
components.
- Listing and Searching:
JavaScript Logic :
Routing Logic:
- React Router: The
App
component usesSwitch
andRoute
fromreact-router-dom
to define routes for different parts of the app. Each route corresponds to a specific component (e.g.,Homepage
,Exchanges
), ensuring users can navigate seamlessly.
- React Router: The
State Management:
- Redux Toolkit: State management is handled using Redux Toolkit. The store integrates API slices from
cryptoApi
andcryptoNewsApi
, which are automatically generated bycreateApi
from@reduxjs/toolkit/query/react
. This setup simplifies data fetching and caching.
- Redux Toolkit: State management is handled using Redux Toolkit. The store integrates API slices from
Fetching Data:
- RTK Query: The app uses
useGetCryptoDetailsQuery
,useGetCryptoHistoryQuery
, anduseGetCryptosQuery
hooks to fetch data from the crypto API. These hooks handle the API requests and provide loading and error states, making data fetching and state management straightforward.
- RTK Query: The app uses
Component Logic:
- CryptoDetails: Manages the selected time period for historical data using
useState
. It updates the displayed data based on user selection and renders statistics and charts. - Cryptocurrencies: Filters the list of cryptocurrencies based on the search term. It updates the filtered list whenever the search term changes, ensuring the UI reflects the user’s input in real-time.
- CryptoDetails: Manages the selected time period for historical data using
Purpose of Functions:
App Component:
- Rendering Layout: The primary function is to render the overall layout of the app, including the navbar, main content, and footer.
- Routing: Manages routing to different parts of the app based on the URL.
Store Configuration:
- Combining Reducers: Integrates different reducers (crypto and news) to manage state centrally.
- Middleware: Automatically adds middleware for caching, invalidation, polling, and other features of RTK Query.
CryptoDetails Component:
- Fetching and Displaying Data: Fetches detailed cryptocurrency data and historical price data. Displays this data using various UI components.
- Handling User Input: Manages user input for selecting time periods and updates the displayed data accordingly.
Cryptocurrencies Component:
- Data Fetching: Fetches a list of cryptocurrencies and handles loading and error states.
- Search Functionality: Filters the list of cryptocurrencies based on user input, updating the UI in real-time to show matching results.
By combining these components and logic, the cryptocurrency app provides a comprehensive and interactive user experience, allowing users to explore and stay updated on various cryptocurrencies.
Get Discount on Top Educational Courses
Source Code :
Output :
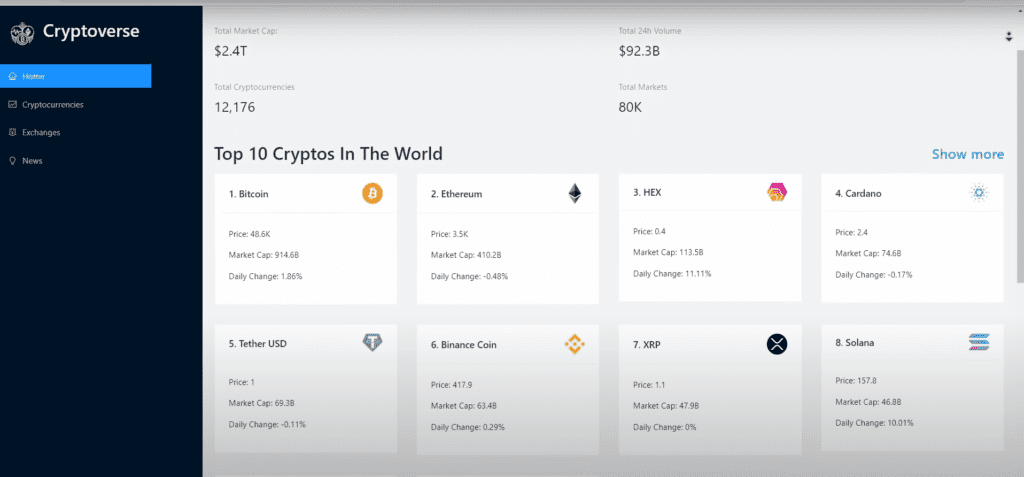
Find More Projects
how to create a video background with html CSS JavaScript Introduction Hello friends, you all are welcome to today’s new blog post. …
Auto Text Effect Animation Using Html CSS & JavaScript Introduction Hello friends, welcome to today’s new blog post. Today we have created …
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …