Stock Management System Using Java With Source Code
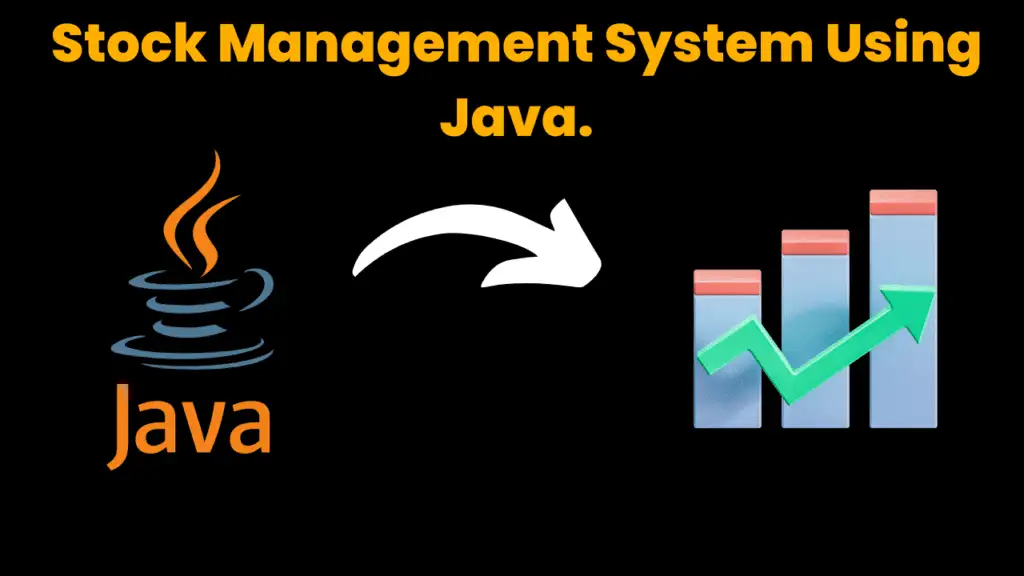
Introduction:
Welcome to Stock Management System using Java! A stock management system is a software application that helps businesses manage their inventory of goods. It allows businesses to track the flow of goods from supplier to warehouse to customer and provides real-time information on the quantity and availability of products. The system is designed to help businesses maintain optimal stock levels, reduce waste and stockouts, and improve efficiency. It makes the tasks of adding and removing of stocks in inventory simple. Stock manager can handle all these tasks on his fingertips. Overall, a stock management system is an essential tool for businesses of all sizes that need to manage their inventory effectively and efficiently. It can help businesses reduce costs, improve customer service, and increase profitability by optimizing their supply chain and inventory management processes.
Explanation:
The Stock Management System we will build will be a simple console-based application that allows users to add, remove and view all the available stocks and their amount. We have used inbuilt classes ArrayList and Scanner. ArrayList is for storing the stocks while Scanner class is for taking the output from user through keyboard. Our program consists of two classes named as StockManagementSystem and Stock. Stock class has all the getters and setters for stock name, quantity, and price. It also has method for removing stock with condition of quantity to remove is less than available quantity. Stock management system class has main method. It has switch case inside do while loop. There are four options will appear as – Add stock, Remove stock, View stock and Exit. For adding stock user need to give stock name, quantity, and price. ‘Add’ function of ArrayList has been used to add stocks in record. Removal of stock is taken place by its name. If stock is available in record then it will be removed on the given quantity provided by user. We have simply called the method to remove which we have coded in Stock class. For displaying stocks for each loop has been used which will print stock name with it’s quantity and price. Breaking the loop will make user exits from the system. Basic OOPS concepts are making this system easy to code and understand. Happy Coding!
Source Code:
import java.util.ArrayList;
import java.util.Scanner;
public class StockManagementSystem {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// create an empty arraylist to hold the stocks
ArrayList stocks = new ArrayList<>();
// display the menu and ask for user input
int choice;
do {
System.out.println("1. Add stock");
System.out.println("2. Remove stock");
System.out.println("3. View stock");
System.out.println("4. Exit");
System.out.print("Enter your choice: ");
choice = input.nextInt();
switch (choice) {
case 1:
// ask for stock details
System.out.print("Enter the stock name: ");
String name = input.next();
System.out.print("Enter the stock quantity: ");
int quantity = input.nextInt();
System.out.print("Enter the stock price: ");
double price = input.nextDouble();
// create a new Stock object and add it to the arraylist
Stock newStock = new Stock(name, quantity, price);
stocks.add(newStock);
System.out.println("Stock added successfully");
break;
case 2:
// ask for the stock name and quantity to remove
System.out.print("Enter the stock name: ");
String stockName = input.next();
System.out.print("Enter the quantity to remove: ");
int removeQuantity = input.nextInt();
// loop through the stocks to find the matching stock
boolean stockFound = false;
for (Stock stock : stocks) {
if (stock.getName().equals(stockName)) {
// if the stock is found, remove the specified quantity
stockFound = true;
stock.removeQuantity(removeQuantity);
System.out.println("Stock removed successfully");
break;
}
}
if (!stockFound) {
System.out.println("Stock not found");
}
break;
case 3:
// display the list of stocks
System.out.println("Current stocks:");
for (Stock stock : stocks) {
System.out.println(stock.toString());
}
break;
case 4:
System.out.println("Exiting the program...");
break;
default:
System.out.println("Invalid choice, try again.");
break;
}
} while (choice != 4);
input.close();
}
}
class Stock {
private String name;
private int quantity;
private double price;
public Stock(String name, int quantity, double price) {
this.name = name;
this.quantity = quantity;
this.price = price;
}
public String getName() {
return name;
}
public int getQuantity() {
return quantity;
}
public double getPrice() {
return price;
}
public void removeQuantity(int quantityToRemove) {
if (quantityToRemove <= quantity) {
quantity -= quantityToRemove;
} else {
System.out.println("Not enough quantity to remove");
}
}
public String toString() {
return name + ", quantity: " + quantity + ", price: $" + price;
}
}
Output:
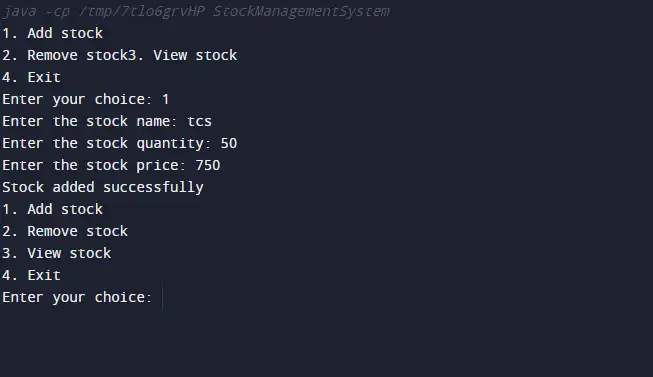
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …