Library Management System Using Java
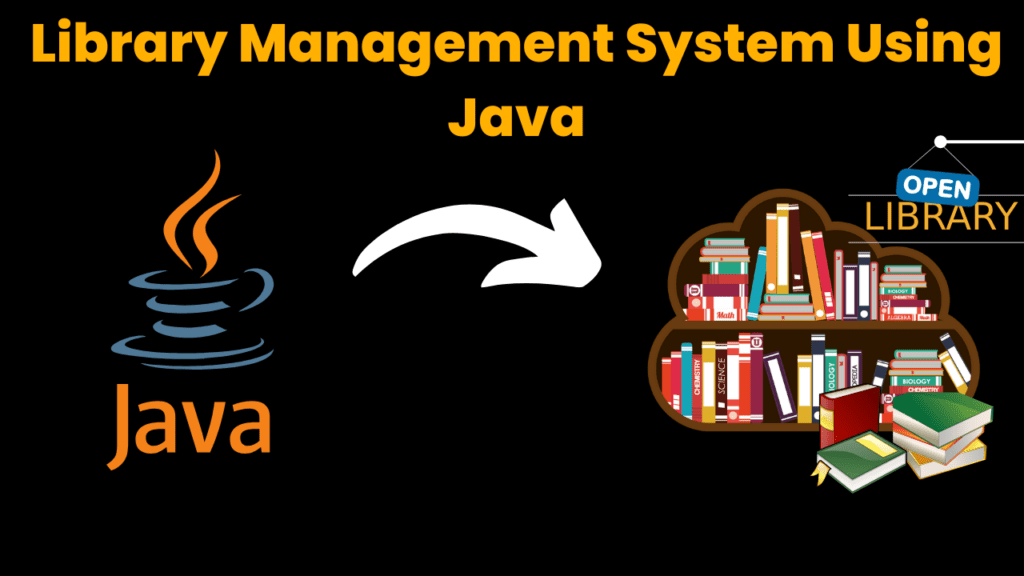
Abstract:
library management system would be to create a separate class for handling the catalog of books, and another class for handling the individual books. This would allow for better organization and separation of concerns, as well as making the code more reusable. The Library class holds a list of all the books in the library and has methods for adding and removing books, as well as displaying all the books in the library. The LibraryManagementSystem
class handles the user interface and communication between the Book
and Library
classes, using a Scanner
object to get user input and controlling the overall flow of the program
Introduction:
Explanation:
A Library Management System is a software application that helps to manage and organize the daily operations of a library.
Before we begin, let’s first define the main components of our LMS. We will need to create a Book class that will hold information about each book in the library, including the title, author, ISBN, and availability status. Finally, you will create a LibraryManagementSystem
class that will handle the user interface and communication between the Book
and Library
classes.
Book class will have four instance variables: Title
, Author
, ISBN, and Availability
. These variables will hold the respective information about each book. We will also include a constructor that will take in the values for each variable and set them to the corresponding instance variables.
Source Code:
import java.util.ArrayList;
import java.util.Scanner;
class Book {
String title;
String author;
String ISBN;
boolean availability;
public Book(String title, String author, String ISBN, boolean availability) {
this.title = title;
this.author = author;
this.ISBN = ISBN;
this.availability = availability;
}
}
class Library {
ArrayList books;
public Library() {
books = new ArrayList();
}
public void addBook(Book book) {
books.add(book);
}
public void removeBook(String ISBN) {
for (Book book : books) {
if (book.ISBN.equals(ISBN)) {
books.remove(book);
break;
}
}
}
public void displayBooks() {
for (Book book : books) {
System.out.println("Title: " + book.title);
System.out.println("Author: " + book.author);
System.out.println("ISBN: " + book.ISBN);
System.out.println("Availability: " + book.availability);
System.out.println();
}
}
}
class LibraryManagementSystem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Library library = new Library();
while (true) {
System.out.println("1. Add Book");
System.out.println("2. Remove Book");
System.out.println("3. Display Books");
System.out.println("4. Exit");
int choice = scanner.nextInt();
if (choice == 1) {
scanner.nextLine(); // to consume the newline character
System.out.print("Enter title: ");
String title = scanner.nextLine();
System.out.print("Enter author: ");
String author = scanner.nextLine();
System.out.print("Enter ISBN: ");
String ISBN = scanner.nextLine();
System.out.print("Enter availability: ");
boolean availability = scanner.nextBoolean();
library.addBook(new Book(title, author, ISBN, availability));
} else if (choice == 2) {
scanner.nextLine(); // to consume the newline character
System.out.print("Enter ISBN: ");
String ISBN = scanner.nextLine();
library.removeBook(ISBN);
} else if (choice == 3) {
library.displayBooks();
} else if (choice == 4) {
break;
}
}
scanner.close();
}
}
Next, we will create the Library
class. This class will have an instance variable called book that will hold a list of all the books in the library. We will also include methods for adding and removing books from the list, as well as a method for displaying all the books in the library.
Finally, we will create the LibraryManagementSystem
class that will handle the user interface and communication between the Book
and Library
classes. This class will use a Scanner
object to get user input and will include a main method that will handle the overall flow of the program.
Output:
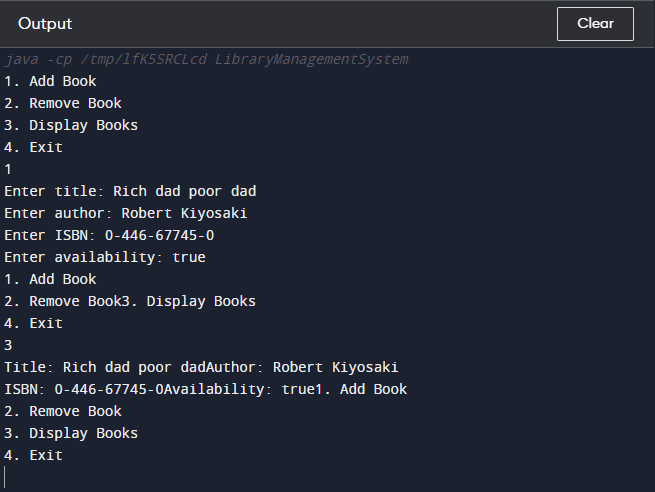
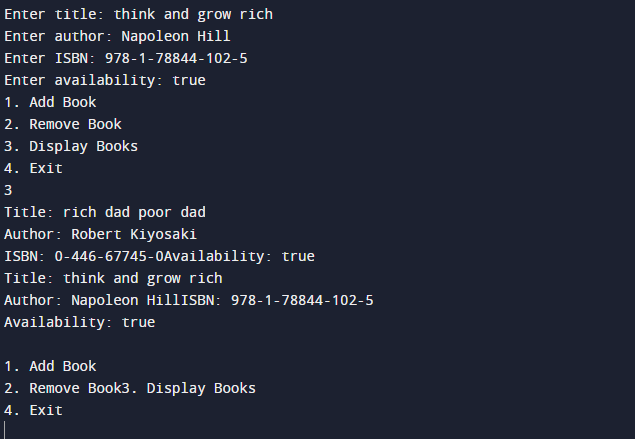
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …