Simple Banking Application Using Java GUI
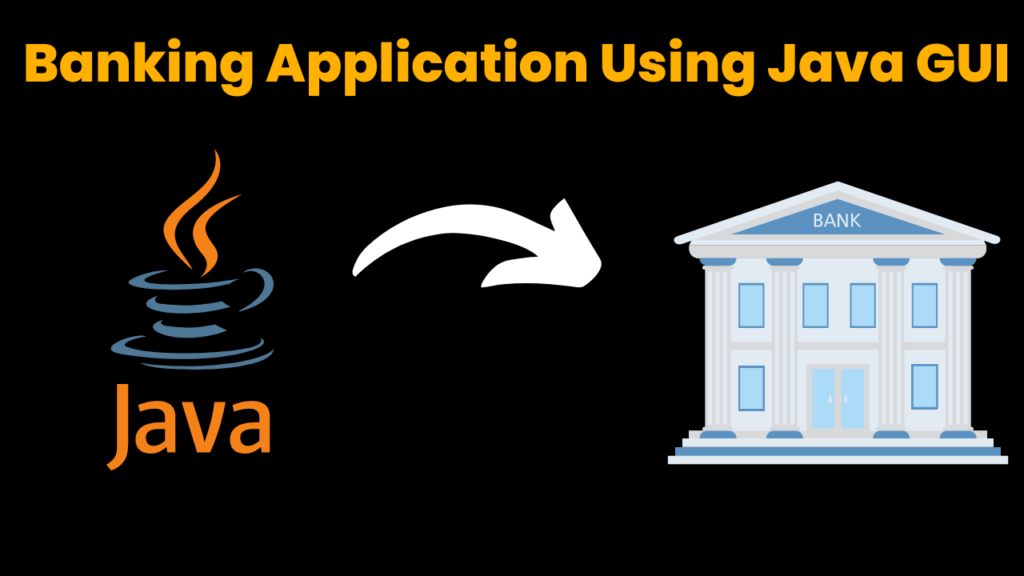
Introduction:
Welcome to the Banking Swing Application! This banking application’s graphical user interface (GUI) provides a simple and understandable method for performing deposit and withdrawal operations. It is created using Java’s Swing library, which provides a flexible and powerful set of components for creating graphical user interfaces. The application has a simple design, with easy-to-use buttons for performing deposit and withdrawal operations, also it updates a current balance after each operation. This application is the ideal tool for everyone who wants to quickly calculate deposits and withdrawals. So, relax, pour yourself a cup of coffee, and begin using the banking application to crunch numbers.
Explanation:
This banking application’s graphical user interface (GUI) provides a simple and understandable method for performing deposit and withdrawal operations. The application has a simple design, with easy-to-use buttons for performing deposit and withdrawal operations, also it updates a current balance after each operation.
Simply enter the desired amount and choose the deposit or withdrawal operation to utilize the banking application. The application will update the balance as per the selected operation, enabling you to quickly and easily estimate multiple deposits and withdrawals.
This application is built using Swing components like JLabel, JButton, and JTextField. To arrange the components, we used the flow Layout. To handle button events, the ActionListener interface is used. The amount will be added to the balance if the “Deposit” button is clicked, and the balance will be reduced if the “Withdraw” button is clicked.
Source Code:
Get Discount on Top Educational Courses
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Banking implements ActionListener {
private JFrame mainFrame;
private JTextField inputField;
private JLabel balanceLabel;
private int balance = 0;
public Banking() {
mainFrame = new JFrame("Simple Bank Application");
mainFrame.setVisible(true);
mainFrame.setSize(400, 400);
mainFrame.setFont(new Font("Arial",Font.BOLD,18));
mainFrame.setLayout(new FlowLayout());
inputField = new JTextField(10);
balanceLabel = new JLabel("Current balance: " + balance);
JButton depositButton = new JButton("Deposit");
JButton withdrawButton = new JButton("Withdraw");
mainFrame.add(inputField);
mainFrame.add(depositButton);
mainFrame.add(withdrawButton);
mainFrame.add(balanceLabel);
depositButton.addActionListener(this);
withdrawButton.addActionListener(this);
mainFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
@Override
public void actionPerformed(ActionEvent e) {
int amount = Integer.parseInt(inputField.getText());
if (e.getActionCommand().equals("Deposit")) {
balance += amount;
} else {
balance -= amount;
}
balanceLabel.setText("Current balance: " + balance);
inputField.setText("");
}
public static void main(String[] args) {
new Banking();
}
}
Find More Projects
resume screener in python using python introduction The hiring process often begins with reviewing numerous resumes to filter out the most suitable …
expense tracer in python using GUI introduction Tracking daily expenses is a vital part of personal financial management. Whether you’re a student …
my personal diary in python using GUI introduction Keeping a personal diary in python is one of the oldest and most effective …
interview question app in python using GUI introduction In today’s rapidly evolving tech landscape, landing a job often requires more than just …
sudoko solver in python using GUI introduction Sudoku, a classic combinatorial number-placement puzzle, offers an engaging challenge that blends logic, pattern recognition, …
handwritten digit recognizer in python introduction In an era where artificial intelligence and deep learning are transforming industries, real-time visual recognition has …