Restaurant Management System Java With Source Code Graphical User Interface [GUI]
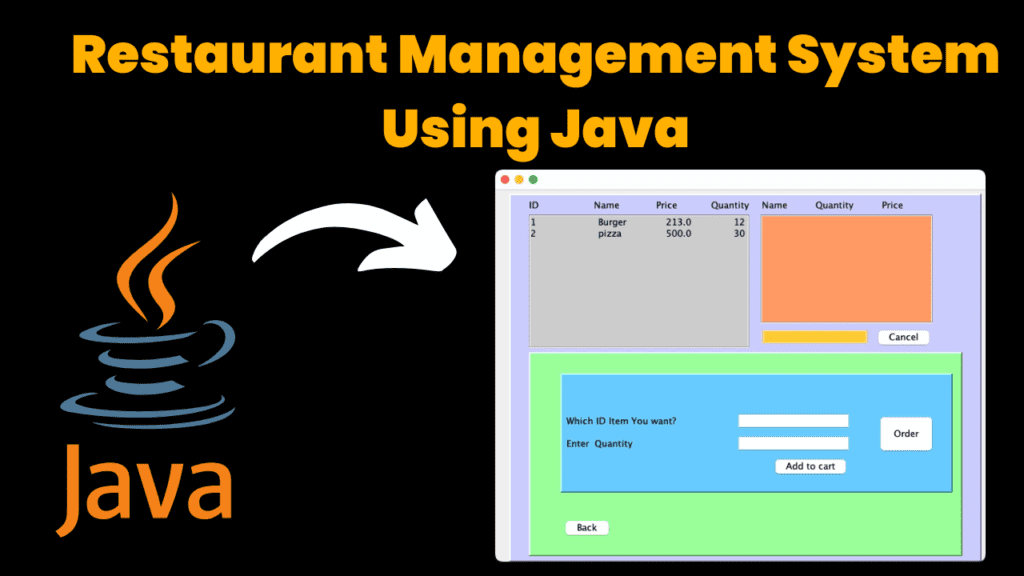
Introduction :
The Restaurant Management System is a desktop application developed for a university assignment in the Programming 1 course. This software aims to assist restaurant managers in efficiently handling daily operations, such as order processing, item management, labor management, and billing. It features a user-friendly interface, basic authentication, and file-based data storage. This project serves as a foundational system that can be expanded or modified according to specific requirements, making it ideal for both educational purposes and practical applications in smaller restaurants.
The system provides a range of modules, including:
- Intuitive User Interface: The application is designed to be easy to use, with a simple layout that facilitates quick navigation between different functionalities.
- Desktop Application: This Java-based software is intended for use on desktop computers, providing a standalone solution that does not require an internet connection.
- File-Based Data Storage: Data is stored in text files, making it easy to manage, view, and modify without needing a complex database system.
- Easy to Modify Design: Developed using the NetBeans IDE, the project allows for easy modifications and design changes through drag-and-drop functionality.
- Multiple Modules: The system includes several modules, such as item management, order management, labor management, and billing, to cover various aspects of restaurant operations.
- Basic Authentication: The application currently uses a hardcoded username and password for authentication.
Required Modules or Packages
This Java project utilizes the following modules and packages:
- Java Swing: Used for creating the graphical user interface (GUI) of the application. Swing provides a range of lightweight components such as buttons, text fields, and tables, which are essential for creating an interactive interface.
- Java AWT (Abstract Window Toolkit): Provides the tools necessary for managing the application’s GUI elements, such as event handling, layout managers, and other components.
- Java I/O (Input/Output): Utilized for reading and writing data to text files, which serve as the project’s storage mechanism. This includes reading from and writing to files like
menu.txt
andEmployee.txt
. - Java Util: Provides various utility classes, such as collections, which are essential for managing data structures like lists and maps used in the application.
To run this project, ensure you have Java installed on your computer. You can use an IDE like NetBeans, which makes it easy to handle Java projects.
To install Java, visit Oracle’s Java Download Page and follow the installation instructions for your operating system.
How to Run the Code:
Follow these steps to set up and run the Restaurant Management System project:
- Download and Install NetBeans IDE: This project is best opened and edited using NetBeans IDE. Download NetBeans from here.
- Clone or Download the Project: Download the project files from the repository or use a version control system to clone the repository to your local machine.
- Open the Project in NetBeans: Launch NetBeans IDE, click on
File > Open Project
, and select the downloaded project folder. - Build the Project: Once the project is open, click on
Run > Build Project
or pressF11
to compile the code and ensure there are no errors. - Run the Main Class: Find the
Main.java
file in theJava Files
directory and run it by right-clicking and selectingRun File
. The application will start, and you can begin using the Restaurant Management System. Login to the System:
- Enter the hardcoded credentials:
Username: shahin
Password: shahin
- Enter the hardcoded credentials:
Navigate Through the Menu:
- Use the on-screen prompts to navigate through various options such as adding orders, managing menu items, handling employee details, and generating bills.
Code Explanation :
This section explains the key components and functionalities of the code.
1. Main Class (Main.java
)
The Main
class is the entry point of the application. It initializes the user interface and handles the main application flow.
public class Main {
public static void main(String[] args) {
// Initialize the manager
Manager manager = new Manager();
manager.displayMenu();
}
}
2. Manager Class (Manager.java
)
The Manager
class is responsible for handling different operations such as managing items, orders, employees, and billing.
public class Manager {
// Display the main menu and manage user choices
public void displayMenu() {
// Logic to display menu options and handle user input
}
}
3. Employee Class (Employee.java
)
This class manages employee-related data, including adding, removing, and updating employee information.
public class Employee {
private String name;
private String role;
// Constructor, getters, setters, and other methods for managing employee data
}
4. Data Handling (DataGeneration.java
)
Handles file-based data storage and retrieval. This includes methods for reading and writing to menu.txt
and Employee.txt
.
public class DataGeneration {
public void loadData() {
// Code to read data from text files
}
public void saveData() {
// Code to write data to text files
}
}
5. Authentication Handling (User.java
)
Handles basic authentication for the system. Currently, the username and password are hardcoded.
public class User {
private final String username = "shahin";
private final String password = "shahin";
public boolean authenticate(String inputUsername, String inputPassword) {
return username.equals(inputUsername) && password.equals(inputPassword);
}
}
Source Code:
The Source Code is Have Multiple Files, Kindly download the source code from below link:
Source Code Contains:
Java Files:
- Bill.java: Likely handles billing operations.
- DataGeneration.java: Probably used for generating or managing data.
- Employee.java: Manages employee-related information and functionalities.
- Main.java: The main entry point of the application.
- Manager.java: Possibly manages different management-related functionalities.
- Menu.java: Manages menu-related data and operations.
- User.java: Handles user-related functionalities, such as authentication.
Text Files:
- Employee.txt: Appears to store employee data.
- menu.txt: Likely stores menu data.
Output :
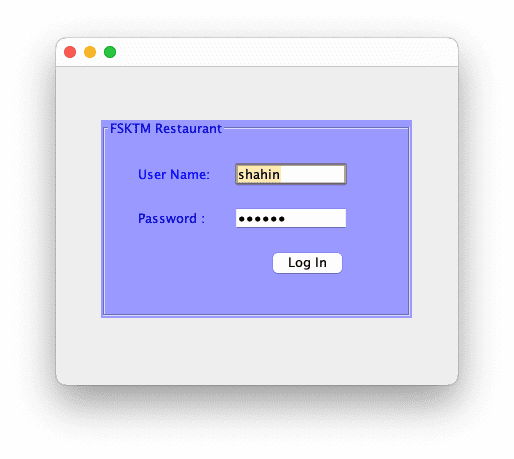
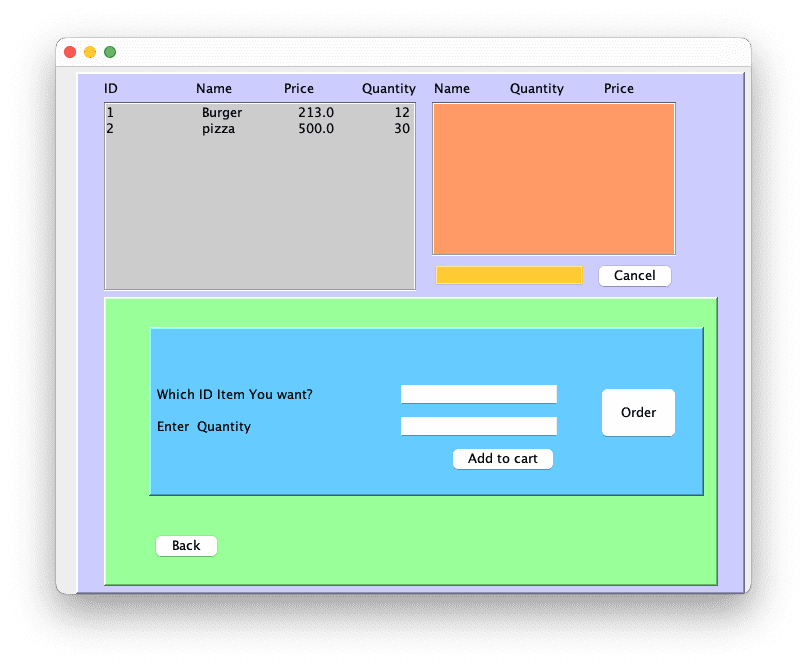
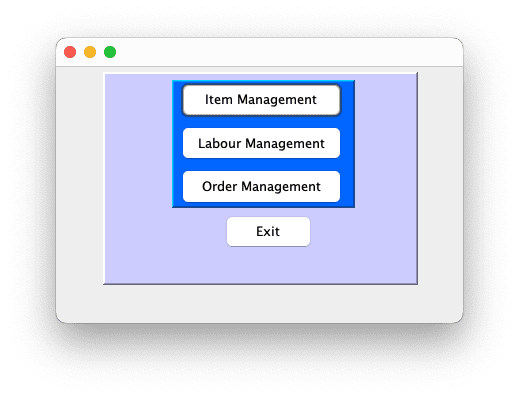
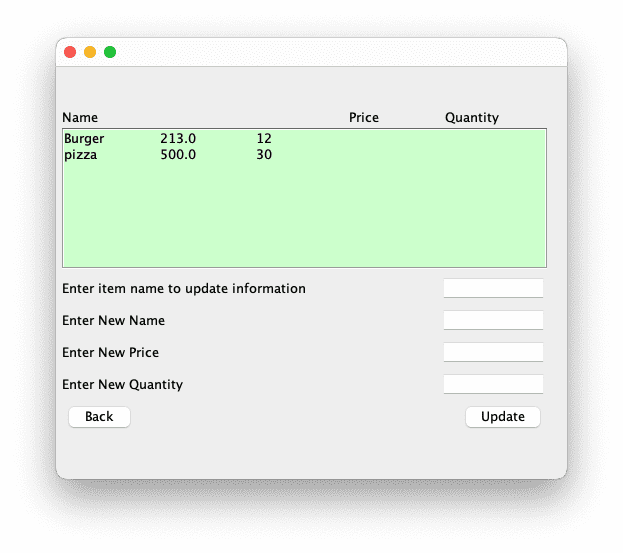
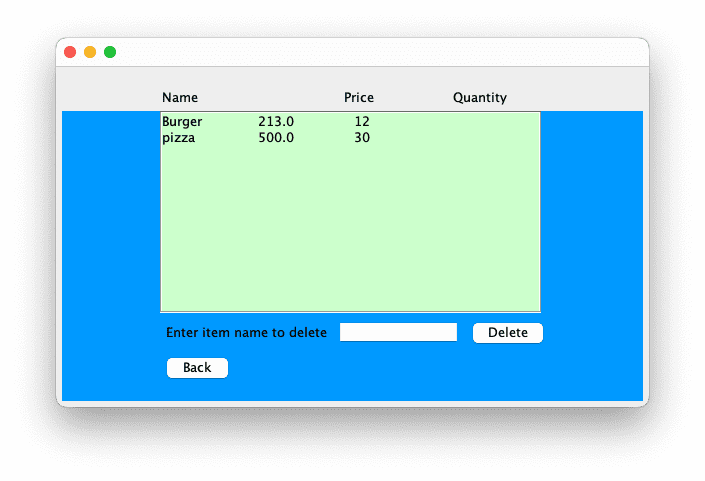
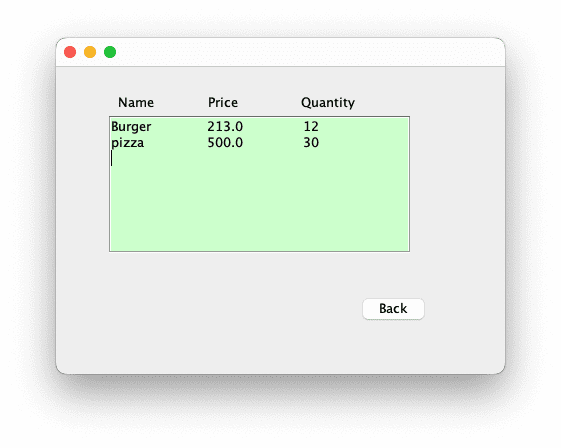
Find More Projects
Resume Builder Application using Java With Source Code Graphical User Interface [GUI] Introduction: The Resume Builder Application is a powerful and user-friendly …
Encryption Tool using java with complete source Code GUI Introduction: The Encryption Tool is a Java-based GUI application designed to help users …
Movie Ticket Booking System using Java With Source Code Graphical User Interface [GUI] Introduction: The Movie Ticket Booking System is a Java …
Video Call Website Using HTML, CSS, and JavaScript (Source Code) Introduction Hello friends, welcome to today’s new blog post. Today we have …
promise day using html CSS and JavaScript Introduction Hello all my developers friends my name is Gautam and everyone is welcome to …
Age Calculator Using HTML, CSS, and JavaScript Introduction Hello friends, my name is Gautam and you are all welcome to today’s new …