Car rental management system Using C++ With Source Code
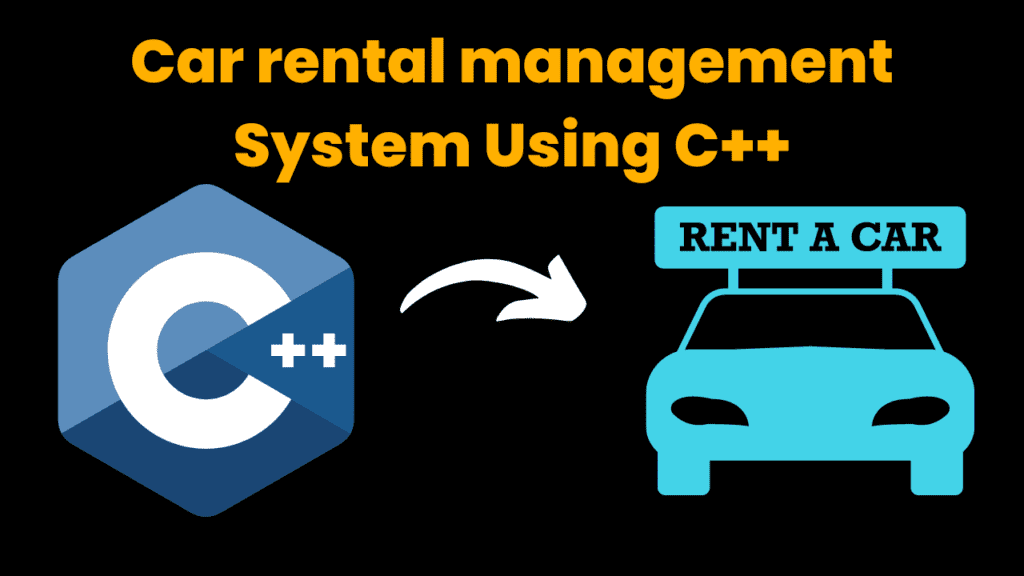
Introduction :
In this project, we have implemented a Car Rental System using C++ programming language. This system allows users to manage various aspects of a car rental service, such as adding, updating, and removing cars from the rental records. Additionally, it handles renting activities, including listing available cars, checking car details, renting cars, and modifying rental records. The system stores the data in JSON format, utilizing the JsonCpp library for manipulating these values.
Whether you’re looking to automate basic operations or store large amounts of data, this system can help streamline the day-to-day activities of a car rental business.
Features
Here are the key features of the Car Rental System:
Add Car: This function allows users to add new cars to the system. Each car requires the input of several details, including the car model, brand, and unique car ID.
Update Car Details: This feature enables users to update existing car information. If any changes are made to a car’s details, such as its availability or specifications, these updates are reflected in the system.
Remove Car: Users can remove a car from the records. Since this operation clears data permanently, caution is advised when performing this task.
List All Cars: This function displays all available cars in the car rental system, allowing users to browse through the entire inventory.
Check Car: Provides details about a specific car, including its availability status, ID, and associated rent records.
Rent a Car: Users can rent a car, which records the renter’s name, car number, rental ID, and rent price.
Modify Rent Records: This feature allows users to update existing rental records. For instance, if the rent price changes, this can be modified in the system.
System Design
The Car Rental System uses a modular design to manage car and rent records. Data is stored in JSON format in two files:
cars.json
- Stores details of the available cars.rents.json
- Stores details of the rental records.
This ensures that all car and rental data are well-organized and easily accessible. The JsonCpp library facilitates seamless interaction with these JSON files.
How to Run the Code:
- Download the Project: Extract the files from the provided source code zip file.
- Install Dependencies: Ensure that the JsonCpp library is installed on your system.
- Compile the Code: Use the following command to compile the main code along with the JsonCpp library.
g++ main.cpp jsoncpp.cpp -o car_rental_system
- Run the Executable: After successful compilation, run the program using the following command:
./car_rental_system
Code Explanation :
Here’s a breakdown of the core parts of the code:
main.cpp: This is the main driver of the program, which handles user input and controls the flow of the program. It interacts with both the
cars.json
andrents.json
files to manage car and rent records.jsoncpp.cpp: This file handles all interactions with the JSON data. It uses the JsonCpp library to read from and write to the JSON files.
Source Code :
main.cpp
// made by https://github.com/Supsource
#include
#include
#include
#include
#include "json/json.h"
using std::string;
using std::cout;
using std::cin;
using std::endl;
Json::Value getCarRecords() {
// https://finbarr.ca/jsoncpp-example/
Json::Value root;
std::ifstream file("cars.json");
file >> root;
file.close();
return root;
}
void setCarRecords(Json::Value root) {
// Write the output to a file
std::ofstream outFile;
outFile.open("cars.json");
outFile << root;
outFile.close();
}
string getName() {
string str;
cout << "Enter Car's Name: ";
getline(cin, str);
if(str == "") {
cout << "Enter a name!" << endl;
getName();
}
return str;
}
int carOptions() {
cout << "1. Car Enquiry"<< endl;
cout << "2. New Car"<< endl;
cout << "3. Modify Car"<< endl;
cout << "4. Remove Car"<< endl;
cout << "5. Show All Cars"<< endl;
cout << "6. Rent a Car"<< endl;
cout << "7. Change Rent Records"<< endl;
cout << "8. Show Rent Records"<< endl;
cout << "0. Exit"<< endl;
cout << "--- Choose any one option ---" << endl;
cout << "Enter one option: ";
int selectedOption = 0;
cin >> selectedOption;
cin.ignore ( std::numeric_limits::max(), '\n' );
return selectedOption;
}
void checkCar() {
cout << "--- Check Car ---" << endl;
string str = getName(); // get account holder's name
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
Json::Value list = getCarRecords();
int len = list.size();
for ( int i = 0; i < len; i++ )
{
string name = list[i]["name"].asString();
string carName = name;
transform(name.begin(), name.end(), name.begin(), ::tolower);
if (name == str) {
string model = list[i]["model"].asString();
string carNum = list[i]["carNumber"].asString();
string rentPrice = list[i]["rentPrice"].asString();
cout << "Car Name: " << carName << endl;
cout << "Car Number: " << carNum << endl;
cout << "Model: " << model << endl;
cout << "Model: " << rentPrice << endl;
return;
}
}
cout << "Record Not Found." << endl;
}
void addCar() {
string carName, carNumber, id, model, rentPrice;
Json::Value records = getCarRecords();
int numOfCars = records.size();
id = std::to_string(numOfCars);
carNumber = "C100" + std::to_string(numOfCars);
cout << endl << "--- Provide Car Details ---" << endl;
cout << "Car Name: ";
getline(cin, carName);
cout << "Car Model: ";
getline(cin, model);
cout << "Rent Price: ";
std::getline(std::cin, rentPrice);
Json::Value record;
record["id"] = id;
record["name"] = carName;
record["carNumber"] = carNumber;
record["model"] = model;
record["rentPrice"] = rentPrice;
records.append(record);
setCarRecords(records);
cout << carName << " Car added. " << endl;
cout << "Car Number: " << carNumber << endl;
}
void updateCar() {
cout << endl << "--- Update Car ---" << endl;
string str = getName();
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
Json::Value records = getCarRecords();
int len = records.size();
bool found = false;
for ( int i = 0; i < len; i++ )
{
string value = records[i]["name"].asString();
// convert to lowercase for comparision;
std::transform(value.begin(), value.end(), value.begin(), ::tolower);
if(str == value) {
string name, model, rentPrice;
cout << "New Name: ";
std::getline(std::cin, name);
cout << "Car Model: ";
std::getline(std::cin, model);
cout << "Rent Price: ";
std::getline(std::cin, rentPrice);
records[i]["name"] = name;
records[i]["model"] = model;
records[i]["rentPrice"] = rentPrice;
cout << "Updated" << endl;
found = true;
break;
}
}
if (!found) {
cout << "Record Not Found." << endl;
} else setCarRecords(records);
}
void removeCar() {
string carNum;
cout << endl << "--- Remove Car ---" << endl;
cout << "Car's Number to remove " << endl;
getline(cin, carNum);
string& str = carNum;
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
Json::Value records = getCarRecords();
int len = records.size();
Json::Value newRecords;
bool carExists = false;
for ( int i = 0; i < len; i++ )
{
string value = records[i]["carNumber"].asString();
// convert to lowercase for comparision;
std::transform(value.begin(), value.end(), value.begin(), ::tolower);
if(str == value) {
carExists = true;
continue;
}
newRecords.append(records[i]);
}
if(carExists) {
setCarRecords(newRecords);
cout << "Car Removed";
} else {
cout << "Car does not exist";
}
}
void showCars() {
cout << "--- List of Cars ---" << endl;
Json::Value list = getCarRecords();
int len = list.size();
cout << "No. | Car Name | Car No. | Model | Rent Price (per day)" << endl;
for ( int i = 0; i < len; i++ )
{
string name = list[i]["name"].asString();
string num = list[i]["carNumber"].asString();
string model = list[i]["model"].asString();
string rentPrice = list[i]["rentPrice"].asString();
int padding = 12 - name.length();
if (padding > 0) {
for(int j = 0; j < padding; j++) {
name += " ";
}
}
cout << i+1 << ". " << name << " " << num << " " << model << " $" << rentPrice << endl;
}
}
// Rent
Json::Value getRentRecords() {
// https://finbarr.ca/jsoncpp-example/
Json::Value root;
std::ifstream file("rents.json");
file >> root;
file.close();
return root;
}
void setRentRecords(Json::Value root) {
// Write the output to a file
std::ofstream outFile;
outFile.open("rents.json");
outFile << root;
outFile.close();
}
struct renter {
string name, phone, address;
};
typedef struct renter Renter;
void addRentRecord() {
string carNumber, rentStartDate, rentEndDate, id;
Renter r;
Json::Value records = getRentRecords();
int numOfRents = records.size();
id = std::to_string(numOfRents);
cout << endl << "--- Provide Rent Details ---" << endl;
cout << "Car Number: ";
getline(cin, carNumber);
cout << "Renter's Name: ";
getline(cin, r.name);
cout << "Renter's Phone: ";
getline(cin, r.phone);
cout << "Renter's Address: ";
getline(cin, r.address);
// dd-mm-yy . format for date . eg: 01-03-2021
cout << "Rent Start Date: ";
getline(cin, rentStartDate);
cout << "Rent End Date: ";
getline(cin, rentEndDate);
Json::Value record;
record["id"] = id;
record["renter"]["name"] = r.name;
record["renter"]["phone"] = r.phone;
record["renter"]["address"] = r.address;
record["car"]["carNumber"] = carNumber;
record["rentStartDate"] = rentStartDate;
record["rentEndDate"] = rentEndDate;
records.append(record);
setRentRecords(records);
cout << endl << r.name << " rented a car. " << endl;
cout << "Car Number: " << carNumber << endl;
}
void showRentRecords() {
cout << "--- Rent Records ---" << endl;
Json::Value list = getRentRecords();
int len = list.size();
cout << "No. | " << "Renter's Name | " << "Car No. | " << "Rent End Date " << endl;
for ( int i = 0; i < len; i++ )
{
string name = list[i]["renter"]["name"].asString();
string num = list[i]["car"]["carNumber"].asString();
string rentEndDate = list[i]["rentEndDate"].asString();
int padding = 18 - name.length();
if (padding > 0) {
for(int j = 0; j < padding; j++) {
name += " ";
}
}
cout << i+1 << ". " << name << " " << num << " " << rentEndDate << endl;
}
}
void changeRentRecords() {
string carNumber, rentStartDate, rentEndDate;
Renter r;
bool found = false;
cout << endl << "--- Update Rent Details ---" << endl;
string str;
cout << "Rent Id: ";
getline(cin, str);
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
Json::Value records = getRentRecords();
int len = records.size();
for ( int i = 0; i < len; i++ )
{
string value = records[i]["id"].asString();
// convert to lowercase for comparision;
std::transform(value.begin(), value.end(), value.begin(), ::tolower);
if(str == value) {
cout << endl << "--- Provide New Rent Details ---" << endl;
cout << "New Car Number: ";
getline(cin, carNumber);
cout << "New Renter's Name: ";
getline(cin, r.name);
cout << "New Renter's Phone: ";
getline(cin, r.phone);
cout << "New Renter's Address: ";
getline(cin, r.address);
// dd-mm-yy . format for date . eg: 01-03-2021
cout << "Rent Start Date: ";
getline(cin, rentStartDate);
cout << "Rent End Date: ";
getline(cin, rentEndDate);
records[i]["renter"]["name"] = r.name;
records[i]["renter"]["phone"] = r.phone;
records[i]["renter"]["address"] = r.address;
records[i]["car"]["carNumber"] = carNumber;
records[i]["rentStartDate"] = rentStartDate;
records[i]["rentEndDate"] = rentEndDate;
setRentRecords(records);
cout << "Update Successful " << endl;
return;
}
}
cout << "Rent not found." << endl;
}
void actions(int& option) {
switch(option) {
case 1:
checkCar();
break;
case 2:
addCar();
break;
case 3:
updateCar();
break;
case 4:
removeCar();
break;
case 5:
showCars();
break;
case 6:
addRentRecord();
break;
case 7:
changeRentRecords();
break;
case 8:
showRentRecords();
break;
}
}
void home () {
int option = carOptions();
if (option != 0 && option <= 8) {
actions(option);
} else if (option > 8) {
cout << endl << "!!! Enter Valid Option !!!" << endl;
option = carOptions();
} else {
exit(0);
}
}
int main () {
cout << "*** WELCOME ***";
string yesOrNo;
while(true) {
cout << endl << "--- Car Rental System ---" << endl;
home();
cout << endl << "Continue? (y/n) :";
cin >> yesOrNo;
if(yesOrNo != "y") break;
}
cout << "Good Bye!" << endl;
}
Output :
After running the program, you will see outputs like “CUSTOMER CREATED SUCCESSFULLY!” or “VEHICLE DELETED” based on the actions you perform. The display functions will show detailed lists of customers or vehicles in a formatted table, making it easy to view and manage records.
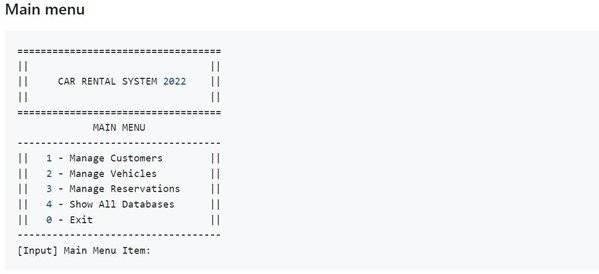
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …