Personal Finance Manager using java swing with complete source code
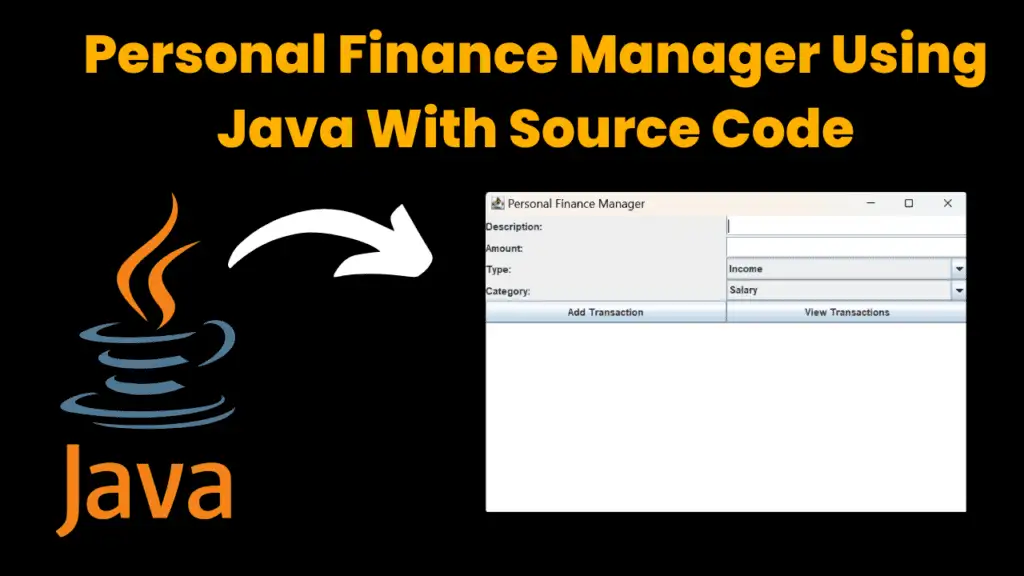
Introduction:
The Personal Finance Manager is a Java-based application designed to help users track their income and expenses efficiently. It allows users to record financial transactions and categorize them for better budget management. The application saves transaction data in a text file to ensure persistence across sessions. With a simple console-based interface, it provides an easy way to monitor financial health. Users can review their income, expenses, and overall balance at any time. Users can categorize expenses under predefined sections like Salary, Food, and Entertainment. The tool also helps prevent overspending by validating expenses against total income. Being Java-based, it is platform-independent and requires minimal setup.
Key Features:
- Add Transaction: Users can add income or expense transactions with a description, amount, and category (e.g., Salary, Food, Entertainment).
- View Transactions: Displays a list of all transactions, total income, total expenses, and net balance.
- Transaction Persistence: Saves all transactions in a file (
transactions.txt
) to retain data across application restarts. - Expense Validation: Ensures that total expenses do not exceed total income to prevent overspending.
- Categorization: Transactions can be sorted into predefined categories for better financial tracking.
- Simple UI: A console-based interface that is easy to use.
- Java-Based: Runs on Java, making it platform-independent.
- Budget Tracking: Helps users keep track of their savings and spending trends.
Required Packages:
The Personal Finance Manager is a Java-based application, so it primarily relies on core Java packages. Based on the project files, here are the required Java packages:
java.util.Scanner
– Used for user input handling.java.io.File
– Used for file handling to save transaction data.java.io.FileWriter
– Used for writing transaction data to a file.java.io.BufferedWriter
– Used for efficient writing of data to a file.java.io.FileReader
– Used for reading transaction data from a file.java.io.BufferedReader
– Used for efficient reading of data from a file.java.io.IOException
– Handles exceptions related to file operations.java.util.ArrayList
– Used to store and manage transactions in memory.java.util.List
– Interface for working with lists of transactions.
How to Run the Code:
Prerequisites
- Install Java Development Kit (JDK 8 or above).
- Check if Java is installed:
java -version
- If not installed, download from:
Navigate to the Project Folder
- Open Command Prompt (Windows) or Terminal (Mac/Linux).
- Move to the project directory:
cd path/to/Personal-Finance-Manager-main
Compile the Java Files
- Run:
javac *.java
- This will generate
.class
files.
Run the Application
- Execute the main class (e.g.,
FinanceManager
):java FinanceManager
- The program starts, allowing you to add, view, and manage transactions.
Running in an IDE (Optional)
- Open the project in IntelliJ IDEA, Eclipse, or VS Code.
- Ensure the main file (e.g.,
FinanceManager.java
) has amain()
method. - Click Run or Execute the file.
Managing Transactions
- Follow on-screen prompts to add, view, or manage transactions.
- Transactions are saved in
transactions.txt
for future use.
Code Explanation :
Transaction.java
(Transaction Class)
- This class likely defines a Transaction object with fields like:
amount
(double)description
(String)type
(Income or Expense)category
(Salary, Food, Entertainment, etc.)
- It includes:
- A constructor to initialize transaction objects.
- Getters/Setters to retrieve or modify transaction details.
- A
toString()
method to display transaction details.
FinanceManager.java
(Main Class)
- This file runs the program and interacts with users.
- It likely includes:
- Scanner object for user input.
- Methods like:
addTransaction()
→ Adds a new transaction.viewTransactions()
→ Displays all transactions.saveToFile()
→ Stores transactions intransactions.txt
.loadFromFile()
→ Reads saved transactions.
- A loop-based menu for user options (e.g., 1: Add, 2: View, 3: Exit).
Source Code:
FinanceManager.java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
public class FinanceManager extends JFrame {
private static final String FILE_NAME = "transactions.txt";
private List transactions;
private JTextField descriptionField, amountField;
private JComboBox typeComboBox, categoryComboBox;
private JTextArea displayArea;
public FinanceManager() {
setTitle("Personal Finance Manager");
setSize(600, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
transactions = new ArrayList();
loadTransactions();
// GUI Components
JPanel inputPanel = new JPanel();
inputPanel.setLayout(new GridLayout(5, 2));
inputPanel.add(new JLabel("Description:"));
descriptionField = new JTextField();
inputPanel.add(descriptionField);
inputPanel.add(new JLabel("Amount:"));
amountField = new JTextField();
inputPanel.add(amountField);
inputPanel.add(new JLabel("Type:"));
typeComboBox = new JComboBox(new String[] { "Income", "Expense" });
inputPanel.add(typeComboBox);
inputPanel.add(new JLabel("Category:"));
categoryComboBox = new JComboBox(new String[] { "Salary", "Food", "Entertainment", "Utilities", "Others" });
inputPanel.add(categoryComboBox);
JButton addButton = new JButton("Add Transaction");
inputPanel.add(addButton);
addButton.addActionListener(e -> addTransaction());
JButton viewButton = new JButton("View Transactions");
inputPanel.add(viewButton);
viewButton.addActionListener(e -> viewTransactions());
displayArea = new JTextArea();
displayArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(displayArea);
add(inputPanel, BorderLayout.NORTH);
add(scrollPane, BorderLayout.CENTER);
}
private void addTransaction() {
String description = descriptionField.getText();
double amount;
try {
amount = Double.parseDouble(amountField.getText());
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(this, "Invalid amount.", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
String type = (String) typeComboBox.getSelectedItem();
String category = (String) categoryComboBox.getSelectedItem();
Transaction transaction = new Transaction(description, amount, type, category);
transactions.add(transaction);
// Check if total expenses are greater than total income
double totalIncome = 0;
double totalExpense = 0;
for (Transaction t : transactions) {
if (t.getType().equals("Income")) {
totalIncome += t.getAmount();
} else {
totalExpense += t.getAmount();
}
}
if (totalExpense > totalIncome) {
transactions.remove(transaction); // Remove the added transaction to avoid imbalance
JOptionPane.showMessageDialog(this, "Error: Total expenses cannot exceed total income!", "Error",
JOptionPane.ERROR_MESSAGE);
} else {
saveTransactions();
clearFields();
}
}
private void clearFields() {
descriptionField.setText("");
amountField.setText("");
typeComboBox.setSelectedIndex(0);
categoryComboBox.setSelectedIndex(0);
}
private void viewTransactions() {
StringBuilder sb = new StringBuilder();
double totalIncome = 0;
double totalExpense = 0;
for (Transaction transaction : transactions) {
sb.append(transaction).append("\n");
if (transaction.getType().equals("Income")) {
totalIncome += transaction.getAmount();
} else {
totalExpense += transaction.getAmount();
}
}
sb.append("\nTotal Income: ").append(totalIncome);
sb.append("\nTotal Expense: ").append(totalExpense);
sb.append("\nNet Balance: ").append(totalIncome - totalExpense);
displayArea.setText(sb.toString());
}
private void loadTransactions() {
try (BufferedReader reader = new BufferedReader(new FileReader(FILE_NAME))) {
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(";", 4);
if (parts.length == 4) {
String description = parts[0].trim();
double amount = Double.parseDouble(parts[1].trim());
String type = parts[2].trim();
String category = parts[3].trim();
transactions.add(new Transaction(description, amount, type, category));
}
}
} catch (IOException e) {
System.out.println("No previous transactions found.");
}
}
private void saveTransactions() {
try (PrintWriter writer = new PrintWriter(new FileWriter(FILE_NAME))) {
for (Transaction transaction : transactions) {
writer.println(transaction.toFileString());
}
} catch (IOException e) {
System.out.println("Failed to save transactions.");
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new FinanceManager().setVisible(true));
}
}
Transaction.java
public class Transaction {
//private cause of encapsulation
private String description;
private double amount;
private String type;
private String category;
public Transaction(String description, double amount, String type, String category) {
this.description = description;
this.amount = amount;
this.type = type;
this.category = category;
}
//getter methods
public String getDescription() {
return description;
}
public double getAmount() {
return amount;
}
public String getType() {
return type;
}
public String getCategory() {
return category;
}
@Override
public String toString() {
return type + ": " + description + " - " + amount + " (" + category + ")";
}
public String toFileString() {
return description + ";" + amount + ";" + type + ";" + category;
}
}
transactions.txt
Create a text file name “Transaction.txt” where all the data will be stored.
For example:Stipend;20000.0;Income;Salary
movie;1000.0;Expense;Entertainment
food;500.0;Expense;Food
Utilities;800.0;Expense;Utilities
Output :
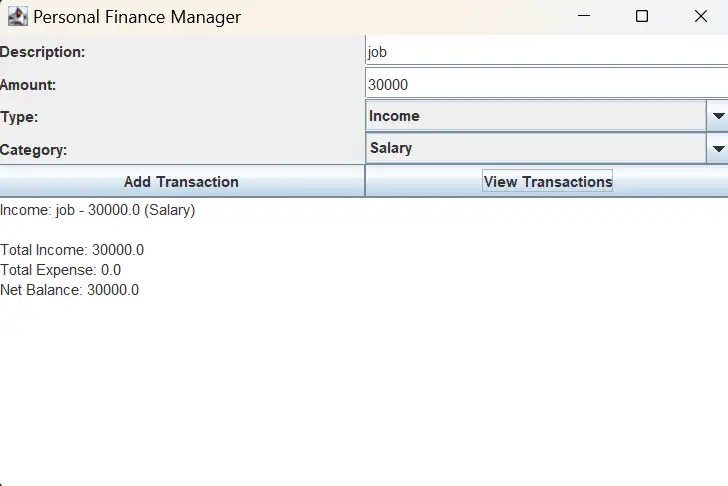
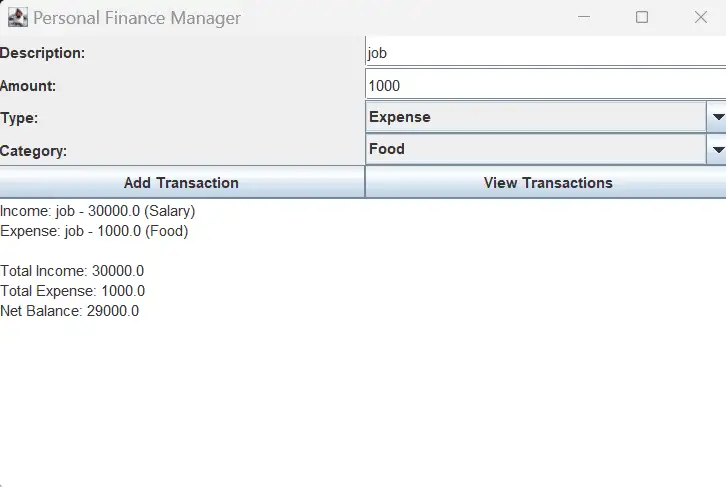