Online Voting System Using Java With Source Code
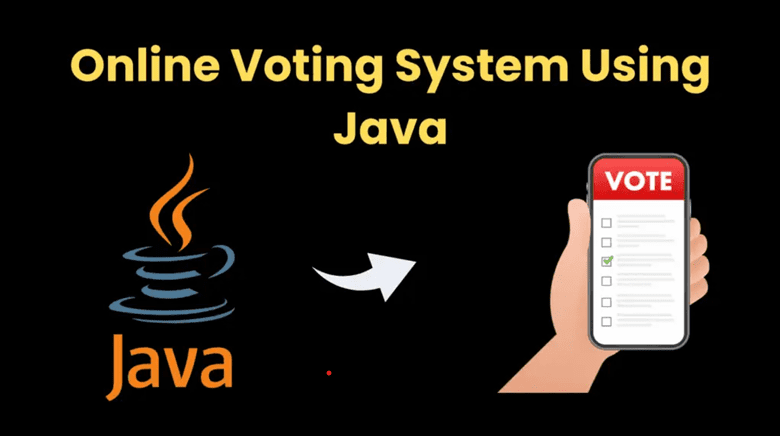
Introduction :
In this project, we build an online voting system using Java, focusing on simplicity and functionality. The system allows voters to cast their votes for different political parties, and provides administrative functionalities to check voting statistics and manage the voting process. This system demonstrates essential concepts in Java, including file handling, inheritance, and user authentication. It provides a practical example of how to manage and record votes, ensuring that each voter can vote only once and that the system accurately tracks and displays results.
Required Modules or Packages :
This project requires no additional modules or packages beyond the Java standard library. The core functionalities are implemented using Java I/O classes and basic control structures.
How to Run the Code :
1. Clone the Repository:
Download or clone the source code from the repository. Compile the Code: Use the javac command to compile the Java files.
javac *.java
Run the Application:
Execute the compiled Test class to start the system.
java Test
Follow the Prompts:
The system will prompt you to log in as an admin or voter and guide you through the voting process.
Code Explanation :
The project is structured into several classes, each responsible for different aspects of the
voting system:
1. Voting machine:
An abstract class that defines the vote method, which is overridden by subclasses representing different political parties. BJP, INC, AAP, BSP, NCP, CPI: Concrete classes extending Voting_machine. Each class implements the vote method to record a vote in a specific file
class BJP extends Voting_machine {
void vote() {
try {
FileWriter fw = new FileWriter("BJP.txt", true);
fw.write("1");
fw.write("\n");
fw.close();
} catch (Exception e) {
System.out.println("Exception is: " + e);
}
}
}
voter_check:
Checks if a voter has already cast their vote. It reads from people_who_voted.txt to determine if the voter’s name and ID are listed.
class voter_check {
int check(String s1, String s2) throws IOException {
BufferedReader b = new BufferedReader(new
FileReader("people_who_voted.txt"));
String s;
while ((s = b.readLine()) != null) {
if (s.equals(s1)) {
s = b.readLine();
if (s.equals(s2)) {
return 1;
}
}
}
return 0;
}
}
admin:
Handles administrative login and verification. It reads from admin.txt to validate the administrator’s credentials.
class admin {
int admin_check(String s1, String s2) throws IOException {
BufferedReader b = new BufferedReader(new
FileReader("admin.txt"));
String s;
while ((s = b.readLine()) != null) {
if (s.equals(s1)) {
s = b.readLine();
if (s.equals(s2)) {
return 1;
}
}
}
return 0;
}
}
voter_count:
Counts the total number of voters by reading the people_who_voted.txt
file.
class voter_count {
int count() throws IOException {
int counter = 0;
BufferedReader b = new BufferedReader(new
FileReader("people_who_voted.txt"));
String s;
while ((s = b.readLine()) != null) {
counter++;
}
return counter / 2;
}
}
max:
Contains a method to determine the maximum value among the votes for different parties.
class max {
public int max_method(int i1, int i2, int i3, int i4, int i5, int
i6) {
return Math.max(i1, Math.max(i2, Math.max(i3, Math.max(i4,
Math.max(i5, i6)))));
}
}
Test:
The main class that manages user interactions, handles login, and processes votes. It
reads and writes to various files to manage voting and administrative operations
class Test {
public static void main(String args[]) throws IOException {
// Initialize counters for votes
int count1 = 0, count2 = 0, count3 = 0, count4 = 0, count5 =
0, count6 = 0;
BufferedReader b = new BufferedReader(new
FileReader("previous_votes.txt"));
String s;
int counter = 0;
while ((s = b.readLine()) != null) {
counter++;
switch (counter) {
case 1: count1 = Integer.parseInt(s); break;
case 2: count2 = Integer.parseInt(s); break;
case 3: count3 = Integer.parseInt(s); break;
case 4: count4 = Integer.parseInt(s); break;
case 5: count5 = Integer.parseInt(s); break;
case 6: count6 = Integer.parseInt(s); break;
}
}
// User interaction loop
Scanner sc = new Scanner(System.in);
String choice;
do {
System.out.println("Welcome to Online Voting System!");
System.out.println("Press 1 for admin login, 2 for voter
login and Exit: ");
choice = sc.next();
// Handle admin and voter login
// (Code omitted for brevity)
} while (!choice.equals("-1"));
// Save vote counts
FileWriter fw = new FileWriter("previous_votes.txt", false);
fw.write(count1 + "\n" + count2 + "\n" + count3 + "\n" +
count4 + "\n" + count5 + "\n" + count6);
fw.close();
}
}
Source Code :
import java.io.*;
import java.util.Scanner;
import java.util.ArrayList;
import java.lang.*;
abstract class Voting_machine{
abstract void vote();
}
class BJP extends Voting_machine{
void vote(){
try{
FileWriter fw=new FileWriter("BJP.txt",true);
fw.write("1");
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}
}
}
class INC extends Voting_machine{
void vote(){
try{
FileWriter fw=new FileWriter("INC.txt",true);
fw.write("1");
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}
}
}
class AAP extends Voting_machine{
void vote(){
try{
FileWriter fw=new FileWriter("AAP.txt",true);
fw.write("1");
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}
}
}
class BSP extends Voting_machine{
void vote(){
try{
FileWriter fw=new FileWriter("BSP.txt",true);
fw.write("1");
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}
}
}
class NCP extends Voting_machine{
void vote(){
try{
FileWriter fw=new FileWriter("NCP.txt",true);
fw.write("1");
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}
}
}
class CPI extends Voting_machine{
void vote(){
try{
FileWriter fw=new FileWriter("CPI.txt",true);
fw.write("1");
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}
}
}
//
To check if person has voted earlier or not
class voter_check {
String s="";
int check (String s1, String s2)throws IOException
{
}}
BufferedReader b=new BufferedReader(new FileReader("people_who_voted.txt"));
while((s=b.readLine())!=null) {
if (s.equals(s1))
{ s=b.readLine();
if (s.equals(s2))
{
return 1;}
}}
return 0;
////////
class admin{
String s="";
ADMIN ID check
int admin_check (String s1, String s2)throws IOException
{
BufferedReader b=new BufferedReader(new FileReader("admin.txt"));
while((s=b.readLine())!=null) {
if (s.equals(s1))
{ s=b.readLine();
if (s.equals(s2))
{
return 1;}
}}
return 0;
}
}
//
class voter_count {
String s="";
VOTER COUNT
int count ()throws IOException
{
int counter=0;
BufferedReader b=new BufferedReader(new FileReader("people_who_voted.txt"));
while((s=b.readLine())!=null)
{
}
BufferedReader b = new BufferedReader(new
FileReader("previous_votes.txt"));
String s;
int counter = 0;
while ((s = b.readLine()) != null) {
counter++;
switch (counter) {
case 1: count1 = Integer.parseInt(s); break;
case 2: count2 = Integer.parseInt(s); break;
case 3: count3 = Integer.parseInt(s); break;
case 4: count4 = Integer.parseInt(s); break;
case 5: count5 = Integer.parseInt(s); break;
case 6: count6 = Integer.parseInt(s); break;
}
}
// User interaction loop
Scanner sc = new Scanner(System.in);
String choice;
do {
System.out.println("Welcome to Online Voting System!");
System.out.println("Press 1 for admin login, 2 for voter
login and Exit: ");
choice = sc.next();
// Handle admin and voter login
// (Code omitted for brevity)
} while (!choice.equals("-1"));
// Save vote counts
FileWriter fw = new FileWriter("previous_votes.txt", false);
fw.write(count1 + "\n" + count2 + "\n" + count3 + "\n" +
count4 + "\n" + count5 + "\n" + count6);
fw.close();
}
}
counter = counter/2;
return counter;
}}
class max
{
}
public int max_method(int i1,int i2,int i3,int i4,int i5,int i6)
{
}
int max_result = Math.max(i1,Math.max(i2,Math.max(i3,Math.max(i4,Math.max(i5,i6)))));
return max_result;
class Test{
public static void main(String args[]) throws IOException{
///
INITIALIZING ALL COUNTERS TO PREVIOUS VOTES
int count1=0;
int count2=0;
int count3=0;
int count4=0;
int count5=0;
int count6=0;
String s="";
int counter=0;
BufferedReader b=new BufferedReader(new FileReader("previous_votes.txt"));
while((s=b.readLine())!=null)
{
counter++;
if(counter==1)
{
}
count1 = Integer.parseInt(s);
if(counter==2)
{
}
count2 = Integer.parseInt(s);
if(counter==3)
{
}
count3 = Integer.parseInt(s);
if(counter==4)
{
count4 = Integer.parseInt(s);
}
if(counter==5)
{
}
count5 = Integer.parseInt(s);
if(counter==6)
{
}
}
count6 = Integer.parseInt(s);
String choice;
do{
String voter_name;
String aadhar;
String login_var;
Scanner sc = new Scanner(System.in);
System.out.println("Welcome to Online Voting System!");
System.out.println("Press 1 for admin login, 2 for voter login and Exit: ");
login_var = sc.next();
if(login_var.equals("1"))
{
choice = "0";
String us,pass;
int ad_chk_res;
System.out.println("Enter your UserId :");
us = sc.next();
System.out.println("Enter your Password :");
pass = sc.next();
admin ad = new admin();
ad_chk_res = ad.admin_check(us,pass);
if(ad_chk_res==1)
{
String admin_log;
System.out.println("Admin Verified!");
System.out.println("\n");
System.out.println("Enter 1 to check on who's winning and 2 to check number of voters: ");
admin_log = sc.next();
if(admin_log.equals("1"))
{
int most=0;
max m=newmax();
most = m.max_method(count1,count2,count3,count4,count5,count6);
ArrayList ar = new ArrayList();
int size;
if (most==count1)
{
}
ar.add("BJP");
if (most==count2)
{
}
ar.add("INC");
if (most==count3)
{
}
ar.add("AAP");
if (most==count4)
{
}
ar.add("BSP");
if (most==count5)
{
}
ar.add("NCP");
if(most==count6)
{
}
ar.add("CPI");
if((ar.size())==1)
{
}
System.out.println("Winning party is: " +ar.get(0));
if((ar.size())==2)
{
}
System.out.println("There is a draw between "+ar.get(0)+" and "+ar.get(1));
if((ar.size())==3)
{
System.out.println("There is a draw between "+ar.get(0)+" and "+ar.get(1)+" and
"+ar.get(2));
}
if((ar.size())==4)
{
System.out.println("There is a draw between "+ar.get(0)+" and "+ar.get(1)+" and
"+ar.get(2)+" and "+ar.get(3));
}
if((ar.size())==5)
{
System.out.println("There is a draw between "+ar.get(0)+" and "+ar.get(1)+" and
"+ar.get(2)+" and "+ar.get(3)+" and "+ar.get(4));
}
if((ar.size())==6)
{
System.out.println("There is a draw between "+ar.get(0)+" and "+ar.get(1)+" and
"+ar.get(2)+" and "+ar.get(3)+" and "+ar.get(4)+" and "+ar.get(5));
}
choice = "0";
}
if(admin_log.equals("2")){
int voters;
voter_count sp = new voter_count();
voters = sp.count();
System.out.println("Number of Voters are: "+voters);
choice = "0";
}
}
}
else{
System.out.println("Enter valid UserId and Password!");
System.out.println("\n");
choice = "0";
}
else{
System.out.println("Enter your name: ");
voter_name = sc.next();
System.out.println("Enter your Voter Id number: ");
aadhar = sc.next();
//
Calling check method to check on voter
voter_check vc = new voter_check();
int result = vc.check(voter_name,aadhar);
if(result == 0)
{
System.out.println("Enter which party you want to vote for: ");
System.out.println("1 for BJP");
System.out.println("2 for INC");
System.out.println("3 for AAP");
System.out.println("4 for BSP");
System.out.println("5 for NCP");
System.out.println("6 for CPI");
System.out.println("-1 to EXIT:");
choice = sc.next();
switch(choice)
{
case "1":
{
BJP bjp=new BJP();
bjp.vote();
count1++;
//
try{
Writing voters name and aadhar to people_who_voted file
FileWriter fw=new FileWriter("people_who_voted.txt",true);
fw.write(voter_name);
fw.write("\n");
fw.write(aadhar);
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}//
}
case "2":
{
ENDOFAPPENDINGVOTERNAME
break;
INC inc=new INC();
inc.vote();
count2++;
//
try{
Writing voters name and aadhar to people_who_voted file
FileWriter fw=new FileWriter("people_who_voted.txt",true);
fw.write(voter_name);
fw.write("\n");
fw.write(aadhar);
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}//
}
case "3":
{
ENDOFAPPENDINGVOTERNAME
break;
AAP aap=new AAP();
aap.vote();
count3++;
//
try{
Writing voters name and aadhar to people_who_voted file
FileWriter fw=new FileWriter("people_who_voted.txt",true);
fw.write(voter_name);
fw.write("\n");
fw.write(aadhar);
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}//
}
case "4":
{
ENDOFAPPENDINGVOTERNAME
break;
BSP bsp=new BSP();
bsp.vote();
count4++;
//
try{
Writing voters name and aadhar to people_who_voted file
FileWriter fw=new FileWriter("people_who_voted.txt",true);
fw.write(voter_name);
fw.write("\n");
fw.write(aadhar);
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}//
break;
}
case "5":
{
ENDOFAPPENDINGVOTERNAME
NCPncp=new NCP();
ncp.vote();
count5++;
//
try{
Writing voters name and aadhar to people_who_voted file
FileWriter fw=new FileWriter("people_who_voted.txt",true);
fw.write(voter_name);
fw.write("\n");
fw.write(aadhar);
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}//
break;
}
case "6":
{
ENDOFAPPENDINGVOTERNAME
CPI cpi=new CPI();
cpi.vote();
count6++;
//
try{
Writing voters name and aadhar to people_who_voted file
FileWriter fw=new FileWriter("people_who_voted.txt",true);
fw.write(voter_name);
fw.write("\n");
fw.write(aadhar);
fw.write("\n");
fw.close();
}catch(Exception e){
System.out.println("Exception is: "+ e);
}//
}
}
}
else{
choice = "0";
ENDOFAPPENDINGVOTERNAME
break;
System.out.println("You can't vote more than once!");
}
}
}while(!(choice.equals("-1")));
String sa = String.valueOf(count1);
String sb = String.valueOf(count2);
String sc = String.valueOf(count3);
String sd = String.valueOf(count4);
String se = String.valueOf(count5);
String sf = String.valueOf(count6);
FileWriter fw=new FileWriter("previous_votes.txt",false);
fw.write(sa);
fw.write("\n");
fw.write(sb);
fw.write("\n");
fw.write(sc);
fw.write("\n");
fw.write(sd);
fw.write("\n");
fw.write(se);
fw.write("\n");
fw.write(sf);
fw.close();
}
}
Output :
When running the system:
1. Admin Login: Admins can check the leading party or count the number of voters.
2. Voter Login: Voters can cast their vote for a party, and the system ensures they cannot
vote more than once.
Reference
For further details or to view the complete code, visit: [Insert URL to code repository or documentation here
More java Pojects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
resume screener in python using python introduction The hiring process often begins with reviewing numerous resumes to filter out the most suitable …
expense tracer in python using GUI introduction Tracking daily expenses is a vital part of personal financial management. Whether you’re a student …
my personal diary in python using GUI introduction Keeping a personal diary in python is one of the oldest and most effective …
interview question app in python using GUI introduction In today’s rapidly evolving tech landscape, landing a job often requires more than just …
sudoko solver in python using GUI introduction Sudoku, a classic combinatorial number-placement puzzle, offers an engaging challenge that blends logic, pattern recognition, …
handwritten digit recognizer in python introduction In an era where artificial intelligence and deep learning are transforming industries, real-time visual recognition has …