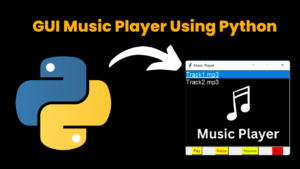
Introduction:
The GUI Music Player using Python is an interactive application where user can simply control it by clicking on the buttons. To build this project in python we need to create an interface first with the help of tkinter() package. Next, using mixer module in pygame package we are going to control the execution of song. Finally, to control the execution of any process we need interact with the operating system we are using os package in python and the code for this project is here…
Code:
# import all the required packages
from tkinter import *
from pygame import mixer
import os
# Creating interface or root window
root=Tk()
# To make the size of the window static
root.resizable(0,0)
# To Insert a title to the created root window
root.title('Music Player')
# Function to play the song
def play():
currentsong=playlist.get(ACTIVE)
mixer.music.load(currentsong)
mixer.music.play()
# Function to pause the song which is currently playing
def pause():
mixer.music.pause()
# Function to resume the song which has paused
def resume():
mixer.music.unpause()
# Function to stop the currently playing song
def stop():
mixer.music.stop()
# Intilaizing the mixer module
mixer.init()
# Creating a listbox where the list of songs are going to be displayed
playlist = Listbox(root, selectmode=SINGLE, bg="black", fg="white", font=('arial', 15), width=30)
playlist.grid(columnspan=4)
# Specifying the path from where the list of songs need to displayed on the root window
os.chdir = os.chdir(r"C:\\Users\Music")
songs = os.listdir()
for s in songs:
playlist.insert(END, s)
# Creating button which is used to control the play,pause,resume and stop the song
playbtn = Button(root, text="Play", command=play,bg='yellow',fg='blue')
playbtn.grid(row=1, column=0)
pausebtn = Button(root, text="Pause", command=pause,bg='yellow',fg='red')
pausebtn.grid(row=1, column=1)
Resumebtn = Button(root, text="Resume", command=resume,bg='yellow',fg='green')
Resumebtn.grid(row=1, column=2)
stopbtn = Button(root, text="Stop", command=stop,bg='red',fg='black')
stopbtn.grid(row=1, column=3)
# To execute the output window
mainloop()
Explanation:
1. Imported all the required libraries
import tkinter – to create the root window
import mixer from pygame – To control the flow of the song play
import os – As we need to stop, resume the play of music (in backend we are stopping the executing process and resuming it) so we need to interact with the os
2. Created root window or an interface with required geometric specifications using tkinter
3. Creating a lit box inside root window which is used to display list of songs from the given directory
4. Next we created functions play,resume,pause,stop to play, resume, pause and stop the song respectively when the buttons are pressed.
5. Finally the buttons are created to operate the GUI music plays just by clicking them
Note:
-> Make sure you first installed all the required packages on your computer using command
pip install package_name
-> Give correct directory in the following statement in the code when you want to run your audio files using the above program
os.chdir = os.chdir(r”C:\\Users\Music”)
-> When you click on play without selecting any audio file by default the first audio file will play.
Output :
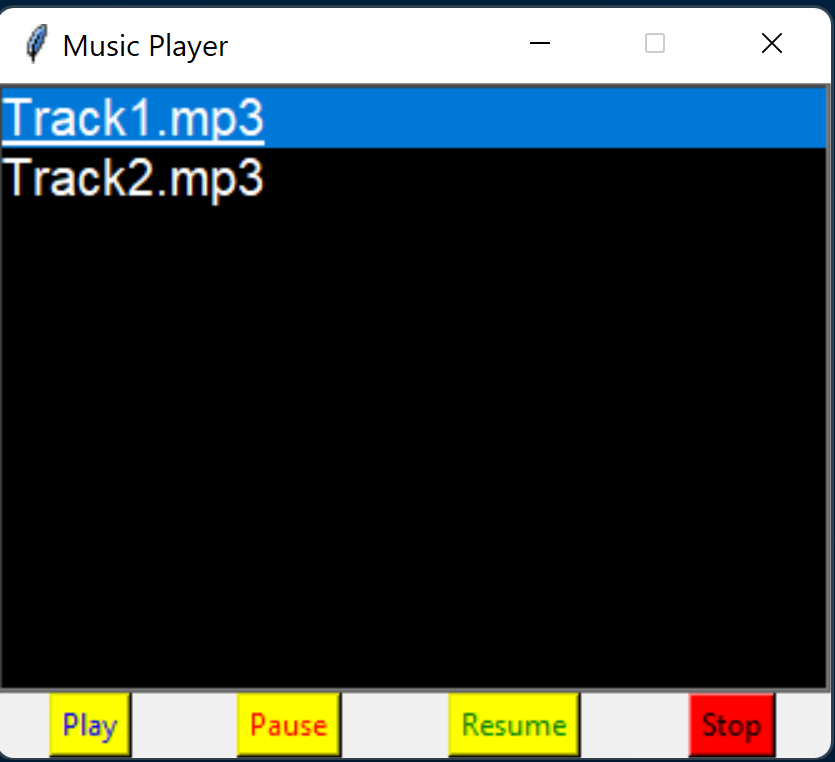
Find More Projects
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …
Chat Application Using HTML , CSS And Javascript Introduction Hello developers friends, all of you are welcome to this new and wonderful …
Travel Planner Website Using HTML , CSS And Javascript Introduction Hello friends, welcome to all of you developer friends, in this new …
Dictionary App using HTML, CSS, JavaScript & ReactJs Introduction : The React dictionary app is a web application designed to provide users …
Weather App using HTML, CSS, JavaScript & ReactJs Introduction : The React Weather App is a web application designed to provide users …