Simple Supermarket Billing System using Java With Source Code
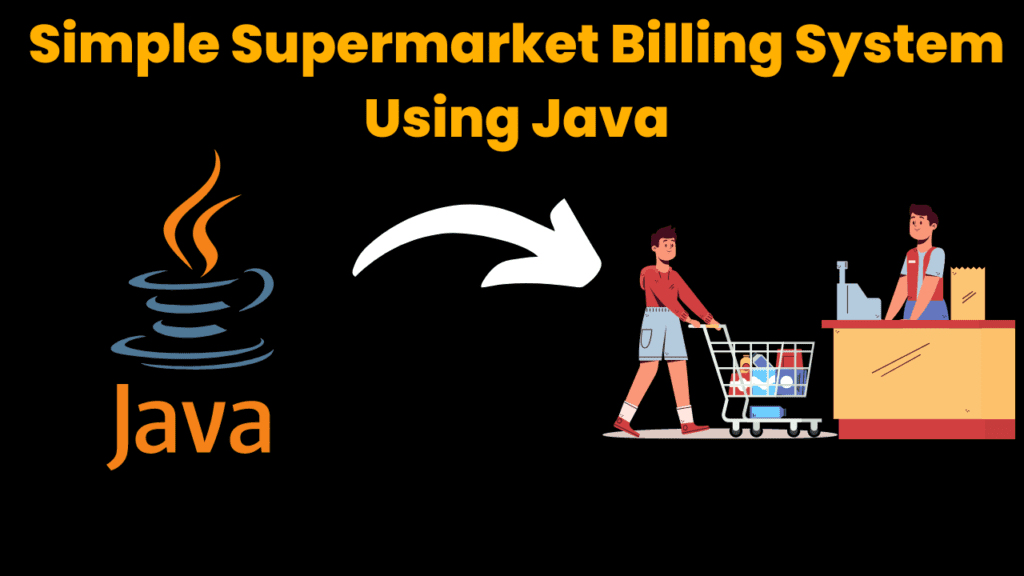
Abstract:
The java program for a supermarket billing system. The code prompts the user to enter details about each items they are purchasing, such as the item name, quantity, and price. It then stores this information in an ArrayList of Item objects, which is a custom class defined in the code. The program then enters a while loop, which continues to execute until the user enters “exit” as the item name. After the user exits the while loop, the program prompts the user to enter the tax rate and discount amount. It then calculates the total tax, the final total and displays the subtotal, tax, discount and final total. This program uses java’s ArrayList data structure and user defined class Item
to keep track of the items purchased and their details. The program can be easily extended and enhanced to include additional features such as bar code scanning, inventory management, and customer database.
Introduction:
Supermarket Billing System is an essential part of any retail store that sells items to customers. It is used to calculate the total cost of the items purchased by a customer, including taxes and discounts. In this article, we will be discussing a Java program that implements a basic Supermarket Billing System.
Explanation:
The program uses a while loop to repeatedly prompt the user to enter details of an item, such as the item name, quantity, and price. It stores this information in an ArrayList of Item objects, which is a custom class defined in the code. The ArrayList of Item objects is useful for creating an inventory system or for printing out a receipt of the items purchased.
The program then prompts the user to enter the tax rate and discount amount. It then calculates the total tax, the final total and displays the subtotal, tax, discount and final total.
The Item class defines a simple object that stores the name, quantity, and price of an item. It has three methods getName()
, getQuantity()
, getPrice()
which are used to retrieve the item’s name, quantity, and price respectively.
The program is relatively simple, but it can be easily extended and enhanced to include additional features such as barcode scanning, inventory management, and customer database.
One of the benefits of using Java for this program is its robustness and scalability. Java is a widely-used, high-level programming language that is suitable for a wide range of applications, including large-scale enterprise systems. It also has a large and active community of developers, making it easy to find solutions to any problems that may arise during the development of the program.
Source Code:
import java.util.ArrayList;
import java.util.Scanner;
public class SupermarketBilling {
public static void main(String[] args) {
ArrayList items = new ArrayList();
Scanner scanner = new Scanner(System.in);
double total = 0;
double subTotal = 0;
double tax = 0;
double discount = 0;
double finalTotal = 0;
System.out.println("Welcome to the Supermarket Billing System");
System.out.println("Enter the item details:");
while (true) {
System.out.print("Enter item name: ");
String itemName = scanner.next();
if (itemName.equalsIgnoreCase("exit")) {
break;
}
System.out.print("Enter item quantity: ");
int itemQuantity = scanner.nextInt();
System.out.print("Enter item price: ");
double itemPrice = scanner.nextDouble();
Item item = new Item(itemName, itemQuantity, itemPrice);
items.add(item);
subTotal += itemQuantity * itemPrice;
System.out.println("Item: " + itemName + ", Quantity: " + itemQuantity + ", Price: $" + itemPrice);
}
System.out.print("Enter the tax rate(%): ");
double taxRate = scanner.nextDouble();
tax = (taxRate * subTotal) / 100;
System.out.print("Enter the discount amount($): ");
discount = scanner.nextDouble();
finalTotal = subTotal + tax - discount;
System.out.println("Subtotal: $" + subTotal);
System.out.println("Tax: $" + tax);
System.out.println("Discount: $" + discount);
System.out.println("Total: $" + finalTotal);
}
}
class Item {
private String name;
private int quantity;
private double price;
public Item(String name, int quantity, double price) {
this.name = name;
this.quantity = quantity;
this.price = price;
}
public String getName() {
return name;
}
public int getQuantity() {
return quantity;
}
public double getPrice() {
return price;
}
}
In conclusion, the Supermarket Billing System is an essential part of any retail store that sells items to customers. The program discussed in this article is a basic implementation of such a system, but can be easily extended and enhanced to meet the specific needs of any retail store. Java is a suitable language for developing such a system due to its robustness, scalability, and the availability of a large and active community of developers.
Output:
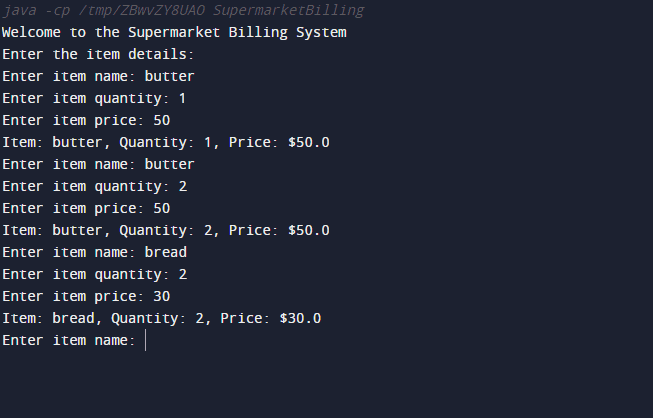
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …