Basic Logging System in C++ With Source Code
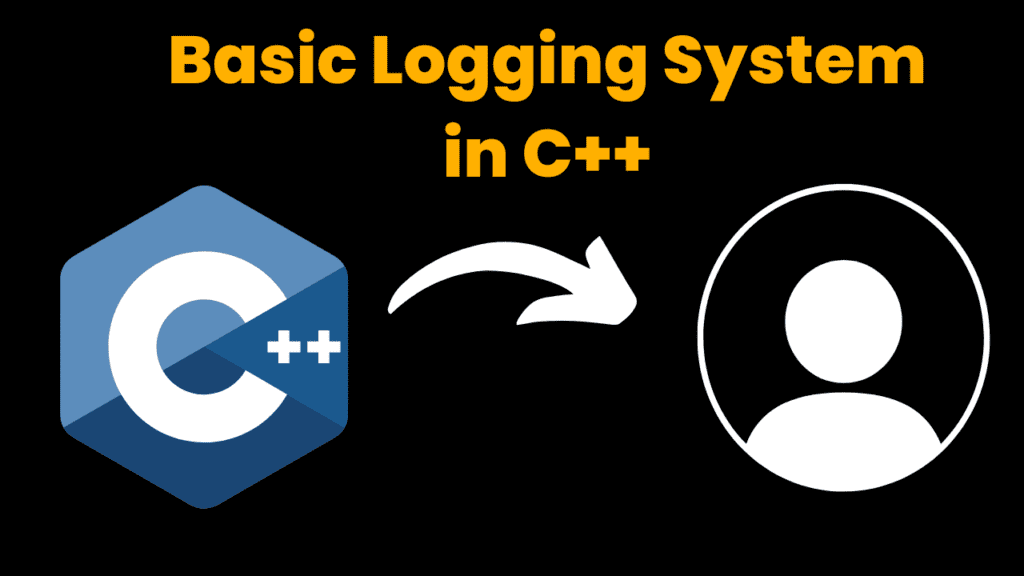
Introduction :
It is one of the most important practices in software development. Logging is immensely helpful for efficient monitoring of application behaviors or tracking issues. The Basic Logging System is a simple and powerful utility in C++, which, with its versatility, enables one to write log messages with a timestamp and variable severity levels to a file. Thisisvery useful for novices in learning C++ since the underlying programming involves verybasic concepts: file handling, enumeration, and time.
The Logging System is developed in C++. It works through the console-based interface. The system allows any developer to log various messages with specific severitylikeDEBUG, INFO, WARNING, ERROR, and CRITICAL directly into a log file. Thoughsimple, the system works pretty well for any small application or acts as an abstractionforfurther complex systems.
The system is mainly designed to log messages on the following levels: DEBUG, INFO, WARNING, ERROR, and CRITICAL. All of these messages will be tagged with a creationtime so that log files have events in chronological order. This project will help developerskeep their logs readable and organized, something very important while debuggingandtrying to maintain their code over time. This mechanism of logging can be integrated into almost any application that calls for event tracking and/or logging. It’s easy to extend, due to the simplicity of it, into more complex functionalities like logging to multiple files or interfacing with external monitoring applications.
Required Header Files:
To implement this project, you only need a C++ compiler. The project utilizes the C++ Standard Library, so there are no additional dependencies or packages required.
Requirements:
- C++ Compiler: The code can be compiled using any modern C++ compiler, such as GCC, Clang, or Microsoft Visual C++.
- Text Editor/IDE: You can use any text editor like Visual Studio Code, Sublime Text, or an IDE like Code::Blocks to write and edit the code.
How to Run Code :
Running the Basic Logging System in C++ is straightforward. Here’s how you can do it:
Clone the Repository: If the project is hosted on GitHub, clone it using the following command:
git clone <repository link>
Alternatively, you can copy the code snippet below into a
.cpp
file.Navigate to the Project Directory: If you cloned the repository, enter the project directory by executing:
cd logging_system
Compile the Code: Use any compiler that supports C++ compilation. For example, using GCC:
g++ -o logger logging_system.cpp
Run the Program: After compilation, run the program by executing:
./logger
The log entries will be printed on the console and written to a file named
logfile.txt
.
Explanation of Code:-
This C++ logging system is developed in the most lightweight and effective way. Thefollowing is the most important part of the code:
Enum for Log Levels
enum LogLevel { DEBUG, INFO, WARNING, ERROR, CRITICAL };
This enumeration defines the different log severity levels. Each log message will be associated with one of these levels, allowing easy filtering and categorization of messages.
Logger Class
The Logger
class contains all the functionality required for logging—such as opening and closing the log file, formatting log entries, and writing them to the file.
Constructor and Destructor :
Logger(const string& filename) {
logFile.open(filename, ios::app);
if (!logFile.is_open()) {
cerr << "Error opening log file." << endl;
}
}
~Logger() {
logFile.close();
}
- The constructor opens the log file in append mode to ensure that every new log is added at the end of the file.
- The destructor closes the file to release resources when it is no longer needed.
Logging Function :
void log(LogLevel level, const string& message) {
time_t now = time(0);
tm* timeinfo = localtime(&now);
char timestamp[20];
strftime(timestamp, sizeof(timestamp), "%Y-%m-%d %H:%M:%S", timeinfo);
ostringstream logEntry;
logEntry << "[" << timestamp << "] " << levelToString(level) << ": " << message << endl;
cout << logEntry.str();
if(logFile.is_open()) {
logFile << logEntry.str();
logFile.flush();
}
}
This function performs the following tasks:
- Generates a timestamp for each log entry.
- Formats the log message with the timestamp, log level, and message content.
- Outputs the formatted message to both the console and the log file.
Level to String Conversion :
string levelToString(LogLevel level) {
switch (level) {
case DEBUG: return "DEBUG";
case INFO: return "INFO";
case WARNING: return "WARNING";
case ERROR: return "ERROR";
case CRITICAL: return "CRITICAL";
default: return "UNKNOWN";
}
}
This helper function converts the LogLevel
enumeration into a human-readable string format, making it easier to identify the severity of each logged message.
Source Code :-
login.cpp
#include
#include
#include
#include
using namespace std;
// Enum to represent log levels
enum LogLevel { DEBUG, INFO, WARNING, ERROR, CRITICAL };
class Logger {
public:
// Constructor: Opens the log file in append mode
Logger(const string& filename) {
logFile.open(filename, ios::app);
if (!logFile.is_open()) {
cerr << "Error opening log file." << endl;
}
}
// Destructor: Closes the log file
~Logger() {
if (logFile.is_open()) {
logFile.close();
}
}
// Logs a message with a given log level
void log(LogLevel level, const string& message) {
// Get current timestamp
time_t now = time(0);
tm* timeinfo = localtime(&now);
char timestamp[20];
strftime(timestamp, sizeof(timestamp), "%Y-%m-%d %H:%M:%S", timeinfo);
// Create log entry
ostringstream logEntry;
logEntry << "[" << timestamp << "] "
<< levelToString(level) << ": " << message << endl;
// Output to console
cout << logEntry.str();
// Output to log file
if (logFile.is_open()) {
logFile << logEntry.str();
logFile.flush(); // Ensure immediate write to file
}
}
private:
ofstream logFile; // File stream for the log file
// Converts log level to a string for output
string levelToString(LogLevel level) {
switch (level) {
case DEBUG:
return "DEBUG";
case INFO:
return "INFO";
case WARNING:
return "WARNING";
case ERROR:
return "ERROR";
case CRITICAL:
return "CRITICAL";
default:
return "UNKNOWN";
}
}
};
int main() {
Logger logger("logfile.txt"); // Create logger instance
// Example usage of the logger
logger.log(INFO, "Program started.");
logger.log(DEBUG, "Debugging information.");
logger.log(ERROR, "An error occurred.");
return 0;
}
Output :
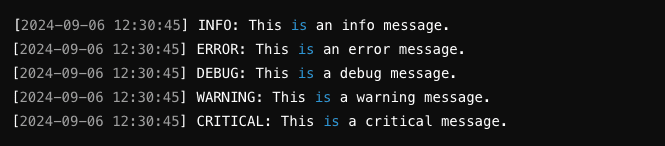
Find More Projects
Resume Builder Application using Java With Source Code Graphical User Interface [GUI] Introduction: The Resume Builder Application is a powerful and user-friendly …
Encryption Tool using java with complete source Code GUI Introduction: The Encryption Tool is a Java-based GUI application designed to help users …
Movie Ticket Booking System using Java With Source Code Graphical User Interface [GUI] Introduction: The Movie Ticket Booking System is a Java …
Video Call Website Using HTML, CSS, and JavaScript (Source Code) Introduction Hello friends, welcome to today’s new blog post. Today we have …
promise day using html CSS and JavaScript Introduction Hello all my developers friends my name is Gautam and everyone is welcome to …
Age Calculator Using HTML, CSS, and JavaScript Introduction Hello friends, my name is Gautam and you are all welcome to today’s new …