Hangman Game Using Java Swing With Source Code
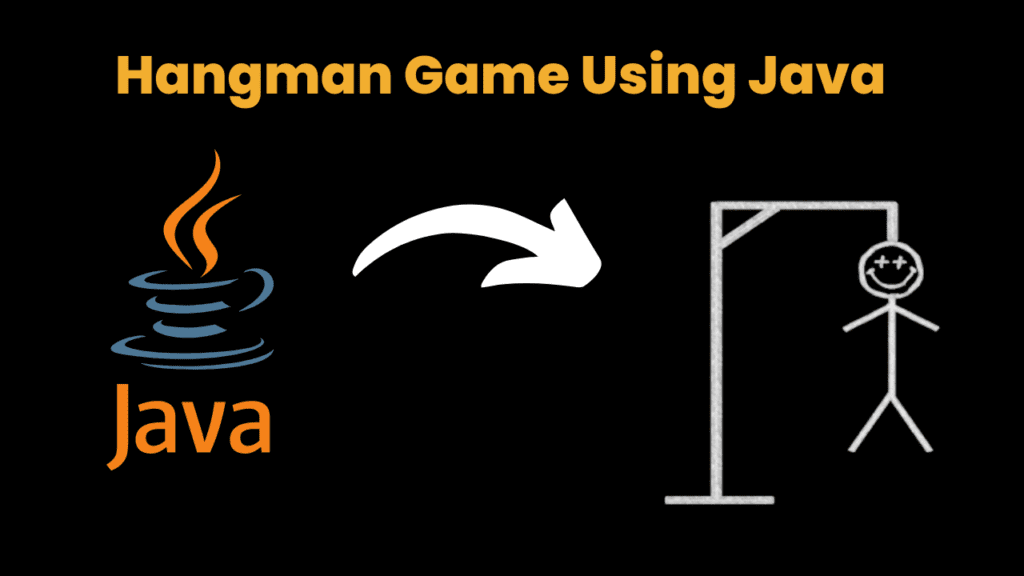
Introduction :
The hangman game has been a popular pastime for generations, challenging players to guess a hidden word by uncovering one letter at a time. If you’re a Java enthusiast looking to delve into game development, creating a hangman game using Java is an excellent project to showcase your skills.
To embark on this coding adventure, you’ll need to leverage the power of Java and its Swing framework, a versatile toolkit for building graphical user interfaces. Java Swing provides a wide range of components and features to design an engaging user interface for your hangman game. You can refer to the Java Swing documentation for detailed information on available classes and methods.
Designing the user interface is a crucial step in building your hangman game. With Java Swing, you can create a main game window, incorporate labels to display the hidden word and incorrect guesses, and style the interface with various components. The interactive nature of the game comes to life through buttons for letter selection and feedback mechanisms to guide the player.
Implementing the game logic involves generating a random word from a predefined list, tracking user guesses, and updating the game state accordingly. Java’s object-oriented programming capabilities enable you to encapsulate these functionalities within classes and methods, making the code modular and maintainable.
Handling user input is another vital aspect of the hangman game. With Java, you can capture user keystrokes, validate and process their guesses, and provide real-time feedback on the correctness of their input.
Once you’ve built the core components, you can enhance the user experience by adding sound effects, animations, and themes to make your hangman game visually appealing and immersive.
To take your Java swing game development skills further, consider testing and debugging your code, creating an executable JAR file, and exploring deployment options to share your creation with others.
Building a hangman game using Java is not only a fun project but also an opportunity to enhance your programming skills and gain a deeper understanding of Java’s capabilities. So, fire up your IDE, dive into the world of Java Swing, and embark on this exciting journey of game development.
Explanation :
Certainly! Here’s an explanation of the key components and concepts used in the provided Hangman game code:
JFrame: It represents the main window or frame of the game.
JLabel: It is used to display text or images in a GUI. In this code,
hiddenWordLabel
andguessesLeftLabel
are JLabel components used to display the hidden word and remaining guesses.JTextField: It is an input component that allows the user to enter their guesses. The
guessTextField
variable represents this component.JButton: It represents a button that triggers an action when clicked. The
guessButton
variable represents the guess submission button.ActionListener: It is an interface used to handle events, such as button clicks or text field submissions. The
HangmanGame
class implements ActionListener to handle user interactions.initializeGame(): This method initializes the game by selecting a random word from the
words
array, setting up the hidden word with underscores, and resetting the number of guesses left.updateHiddenWord(char guess): This method updates the hidden word based on the player’s guess. If the guess is correct, the corresponding letters in the hidden word are revealed. If the guess is incorrect, the number of remaining guesses decreases.
endGame(String message): This method is called when the game ends, either due to winning or losing. It disables the text field and guess button, displays a message dialog with the game result, and allows the player to restart the game.
actionPerformed(ActionEvent e): This method is called when the guess button is clicked or when the enter key is pressed in the text field. It retrieves the guess from the text field, updates the hidden word, and checks for win/loss conditions.
main(String[] args): It is the entry point of the program. The
SwingUtilities.invokeLater()
method is used to run the GUI initialization code on the event dispatch thread, ensuring proper UI rendering.
Source Code :
Get Discount on Top Educational Courses
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class HangmanGame extends JFrame implements ActionListener {
private String[] words = {"hangman", "java", "swing", "programming", "openai"};
private String wordToGuess;
private int guessesLeft = 6;
private StringBuilder hiddenWord;
private JLabel hiddenWordLabel;
private JLabel guessesLeftLabel;
private JTextField guessTextField;
private JButton guessButton;
public HangmanGame() {
setTitle("Hangman Game");
setDefaultCloseOperation(EXIT_ON_CLOSE);
setResizable(false);
hiddenWordLabel = new JLabel();
guessesLeftLabel = new JLabel("Guesses Left: " + guessesLeft);
guessTextField = new JTextField(10);
guessButton = new JButton("Guess");
guessButton.addActionListener(this);
guessTextField.addActionListener(this);
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new FlowLayout());
mainPanel.add(hiddenWordLabel);
mainPanel.add(guessesLeftLabel);
mainPanel.add(guessTextField);
mainPanel.add(guessButton);
getContentPane().add(mainPanel);
initializeGame();
pack();
setLocationRelativeTo(null);
setVisible(true);
}
private void initializeGame() {
wordToGuess = words[(int) (Math.random() * words.length)];
hiddenWord = new StringBuilder();
for (int i = 0; i < wordToGuess.length(); i++) {
hiddenWord.append("_");
}
hiddenWordLabel.setText(hiddenWord.toString());
guessesLeft = 6;
guessesLeftLabel.setText("Guesses Left: " + guessesLeft);
}
private void updateHiddenWord(char guess) {
boolean found = false;
for (int i = 0; i < wordToGuess.length(); i++) {
if (wordToGuess.charAt(i) == guess) {
hiddenWord.setCharAt(i, guess);
found = true;
}
}
hiddenWordLabel.setText(hiddenWord.toString());
if (!found) {
guessesLeft--;
guessesLeftLabel.setText("Guesses Left: " + guessesLeft);
if (guessesLeft == 0) {
endGame("You lose! The word was: " + wordToGuess);
}
} else if (hiddenWord.toString().equals(wordToGuess)) {
endGame("Congratulations! You won!");
}
}
private void endGame(String message) {
guessTextField.setEnabled(false);
guessButton.setEnabled(false);
JOptionPane.showMessageDialog(this, message, "Game Over", JOptionPane.INFORMATION_MESSAGE);
initializeGame();
guessTextField.setEnabled(true);
guessButton.setEnabled(true);
guessTextField.requestFocus();
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == guessButton || e.getSource() == guessTextField) {
String guessText = guessTextField.getText();
if (guessText.length() > 0) {
char guess = guessText.charAt(0);
updateHiddenWord(guess);
guessTextField.setText("");
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(HangmanGame::new);
}
}
Output :
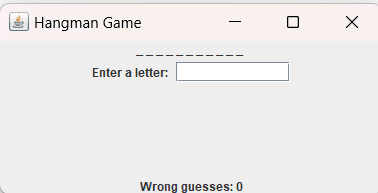
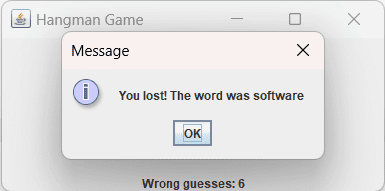
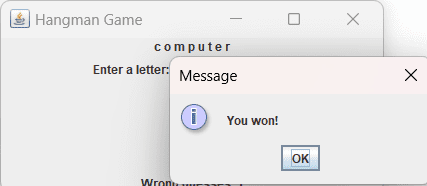
Find More Projects
Drawing Chhatrapati Shivaji Maharaj Using Python Chhatrapati Shivaji Maharaj, the legendary Maratha warrior and founder of the Maratha Empire, is an inspiration …
Resume Builder Application using Java With Source Code Graphical User Interface [GUI] Introduction: The Resume Builder Application is a powerful and user-friendly …
Encryption Tool using java with complete source Code GUI Introduction: The Encryption Tool is a Java-based GUI application designed to help users …
Movie Ticket Booking System using Java With Source Code Graphical User Interface [GUI] Introduction: The Movie Ticket Booking System is a Java …
Video Call Website Using HTML, CSS, and JavaScript (Source Code) Introduction Hello friends, welcome to today’s new blog post. Today we have …
promise day using html CSS and JavaScript Introduction Hello all my developers friends my name is Gautam and everyone is welcome to …