Airline Reservation System Using Java With Source Code
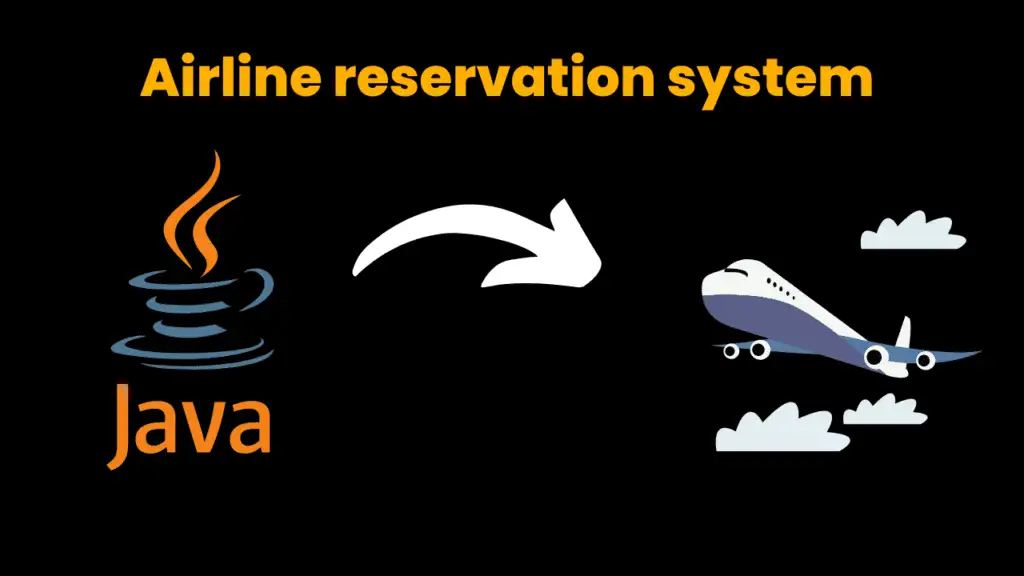
Introduction:
In this project, we’ll show you how to create an Airline reservation system using java. This is a command-line user interface-based airline reservation system that allows you to check seat availability and reserve a seat if it is available. The system is designed with a switch case that gives you three options: view available seats, and reserve a seat. So, get a cup of coffee and use the airline reservation system.
Explanation :
This Command-Line User Interface (CLI)-based Airline Reservation System provides a simple and easy way for checking seat availability and reserving a seat if it is available. The system includes a simple command line interface with a switch case for executing viewing available seats and reserving a seat.
Switch Case, while loop, and Scanner class are used to build this system. The system will first present the user with the available options. View available seats, book a seat, and EXIT is available as a choice. The user must choose a useful option. The operation will be carried out as per the input choice. The seats are stored in array seats, where 0 indicates an available seat and 1 represents a reserved seat.
Source Code :
Get Discount on Top Educational Courses
//importing required packages for program
import java.util.Scanner;
//creating class
public class airline_reservation {
//declaring static global variables
static int seats[] = new int[11];
static Scanner sc = new Scanner(System.in);
//creating main
public static void main(String[] args) {
System.out.println("Welcome to XYZ Airlines");
//while loop to display option till the exit is called
while (true) {
System.out.println("Please select an option: ");
System.out.println("1. View available seats");
System.out.println("2. Reserve a seat");
System.out.println("3. Exit");
int choice = sc.nextInt();
//use of switch case for performing operation of users's prefered choice
switch (choice) {
case 1://display seat
displaySeats();
break;
case 2://reserve seat
reserveSeat();
break;
case 3:
System.out.println("Thank you for using XYZ Airlines");
System.exit(0);
} //end of switch case
} //end of while loop
} //end of main method
//declaring method displaySeats() for display avaliable and reserved seats
public static void displaySeats() {
System.out.println("Seats:");
for (int i = 1; i <= 10; i++) {
//use of ternary operator to print if the seats are avalible or not
System.out.println("Seat " + i + ": " + (seats[i] == 0 ? "Available" : "Reserved"));
}
} //end of displayseats()
//declaring method reserveSeat()
public static void reserveSeat() {
System.out.println("Enter seat number to reserve: ");
int seat = sc.nextInt();
//if checks if the seat is avaliable or not and if the the seat is avaliable it reserves the seat else the seat will be not reserved
if (seats[seat] == 0) {
seats[seat] = 1;
System.out.println("Seat " + seat + " reserved successfully");
} else {
System.out.println("Seat " + seat + " is already reserved");
}
}
}//end of class
Output :
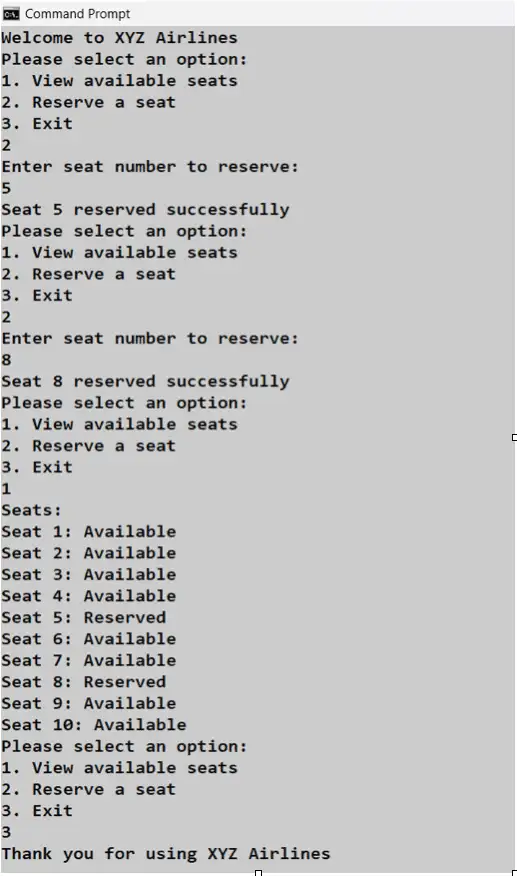
Find More Projects
how to create a video background with html CSS JavaScript Introduction Hello friends, you all are welcome to today’s new blog post. …
Auto Text Effect Animation Using Html CSS & JavaScript Introduction Hello friends, welcome to today’s new blog post. Today we have created …
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …