Online Voting System Using Java With Source Code
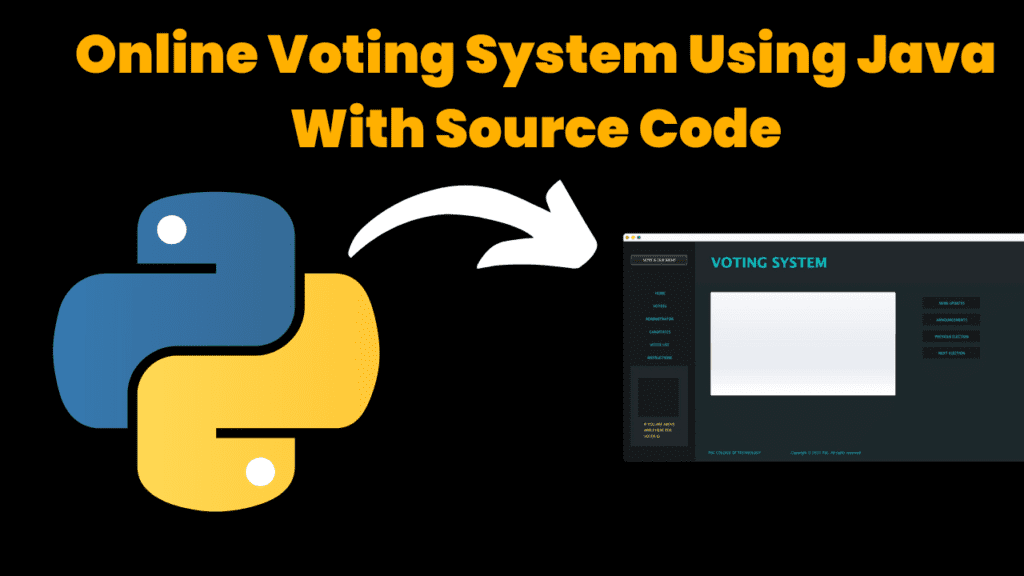
Introduction :
The Voting System is a Java-based desktop application developed using Java Swing for the graphical user interface (GUI) and MySQL for backend database management. This project is designed to simplify the election process by providing an easy-to-use platform for voters and administrators. The system is divided into two main panels: the Voters Panel and the Admin Panel.
In the Voters Panel, voters can apply for a Voter ID, log in once approved, and cast their votes. The Admin Panel allows administrators to log in, conduct elections by creating new elections, add candidates, view election statuses, see election results, and manage voters. This project is an excellent example of how programming can be used to create a practical and secure voting system for organizations, communities, or small-scale elections.
Key Features
- Two Panels (Voters and Admins): Separate interfaces for voters and administrators to perform their respective functions.
- User-Friendly Interface: Developed using Java Swing, the application provides a simple and intuitive GUI.
- SQL Database Integration: The backend uses MySQL to manage data related to voters, candidates, and election details.
- Voter Management: Voters can apply for Voter IDs, log in, and cast their votes securely.
- Admin Management: Admins can create elections, add candidates, manage voter data, view election results, and monitor election statuses.
- Security Features: Basic authentication for both voter and admin panels to ensure secure access.
Required Modules or Packages
This Java project utilizes the following modules and packages:
- Java Swing: Used to create the graphical user interface (GUI). Swing provides the necessary components like buttons, text fields, and panels to create an interactive application.
- Java AWT (Abstract Window Toolkit): Used for handling events, managing layout, and other GUI elements.
- Java JDBC (Java Database Connectivity): Used to connect the Java application with the MySQL database. JDBC allows the application to execute SQL queries, retrieve data, and manipulate the database.
- MySQL: Used as the backend database for storing information about voters, candidates, election details, and results.
To run this project, ensure you have Java and MySQL installed on your computer. You can use an IDE like NetBeans or Eclipse, which makes it easy to handle Java projects with GUI elements.
How to Run the Code:
Follow these steps to set up and run the Voting System project:
- Download and Install NetBeans or Eclipse IDE: Download and install your preferred Java IDE from their official websites.
- Clone or Download the Project: Download the project files from the repository or use a version control system to clone the repository to your local machine.
- Set Up the Database:
- Install MySQL and set up a new database.
- Import the SQL script (if provided) to create the necessary tables and insert initial data.
- Update the JDBC connection settings in the Java files to match your database credentials.
- Open the Project in NetBeans or Eclipse: Launch the IDE, click on
File > Open Project
, and select the downloaded project folder. - Build the Project: Once the project is open, click on
Run > Build Project
or pressF11
to compile the code and ensure there are no errors. - Run the Main Class: Find the
Home.java
file and run it by right-clicking and selectingRun File
. This will start the application, allowing you to use both the Voters Panel and the Admin Panel.
Explanation :
This section explains the key components and functionalities of the code.
1. Home Class (Home.java
)
The Home
class serves as the entry point for the application. It provides the main menu from which users can navigate to either the Voters Panel or the Admin Panel.
public class Home {
public static void main(String[] args) {
// Code to initialize the main menu and handle user input
}
}
2. Voters Login (VotersLogin.java
)
This class handles the login process for voters, ensuring that only approved voters can access the voting system.
public class VotersLogin {
// Methods to authenticate voters and redirect to the voting page
}
3. Admin Page (AdminPage.java
)
The AdminPage
class allows administrators to log in and manage various aspects of the election, such as creating new elections, adding candidates, and viewing results.
public class AdminPage {
// Methods for admin functionalities like creating elections and adding candidates
}
4. Voting Process (VotersVotingProcess.java
)
Manages the voting process, including displaying candidates and recording votes.
public class VotersVotingProcess {
// Code to display the list of candidates and handle vote casting
}
5. Election Management (CreateElection.java
and CreateElectionAddCanditates.java
)
Classes for creating new elections and adding candidates to those elections.
public class CreateElection {
// Code to create a new election
}
public class CreateElectionAddCanditates {
// Code to add candidates to the created election
}
Get Discount on Top Educational Courses
Source Code:
list of all the files from the “Voting System Using Java GUI” project:
Java Files and Their Uses
AdminLogin.java
- This file contains the code for the Admin Login functionality. It handles the authentication process for the admin, ensuring that only authorized users can access the admin panel.
AdminPage.java
- This file is responsible for managing the admin dashboard, where the admin can perform various operations like creating elections, adding candidates, managing voters, viewing election status, and checking results.
Canditates.java
- This file manages the candidates’ data. It contains methods and properties related to candidate information such as adding new candidates and displaying the list of candidates.
CreateElection.java
- This file contains the logic for creating new elections. It allows the admin to set up a new election event, including its name, date, and other relevant details.
CreateElectionAddCanditates.java
- This file allows the admin to add candidates to the newly created elections. It ensures that the candidates are linked correctly to the specific election event.
ElectionResults.java
- This file manages the process of calculating and displaying the results of the election. It retrieves data from the database, processes the votes, and shows the outcome to the admin.
ElectionStatus.java
- This file provides functionality to check the status of ongoing or completed elections. It helps the admin monitor the progress and update the election status as needed.
FirstTimeVotersDetails.java
- This file handles the details of voters applying for the first time. It collects and manages the data of new voter applications and sends it to the admin for approval.
Home.java
- This is the entry point of the application. It provides the main menu interface from which users can choose to navigate to either the Voters Panel or the Admin Panel.
Instructions.java
- This file contains the instructions or guidelines for using the application. It helps users understand how to interact with the system effectively.
ManageVoters.java
- This file manages the list of voters, including adding, removing, and updating voter information. It allows the admin to handle voter-related tasks efficiently.
NewVoterApplication.java
- This file allows new users to apply for a voter ID. It provides a form for entering necessary details and sends the application to the admin for approval.
VotersList.java
- This file displays a list of all registered voters. It allows the admin to view and manage voter details within the system.
VotersLogin.java
- This file handles the login process for voters. It authenticates voters based on their credentials and redirects them to the voting process if verified.
VotersVotingProcess.java
- This file manages the actual voting process. It allows authenticated voters to cast their votes by selecting a candidate from the list.
Output:
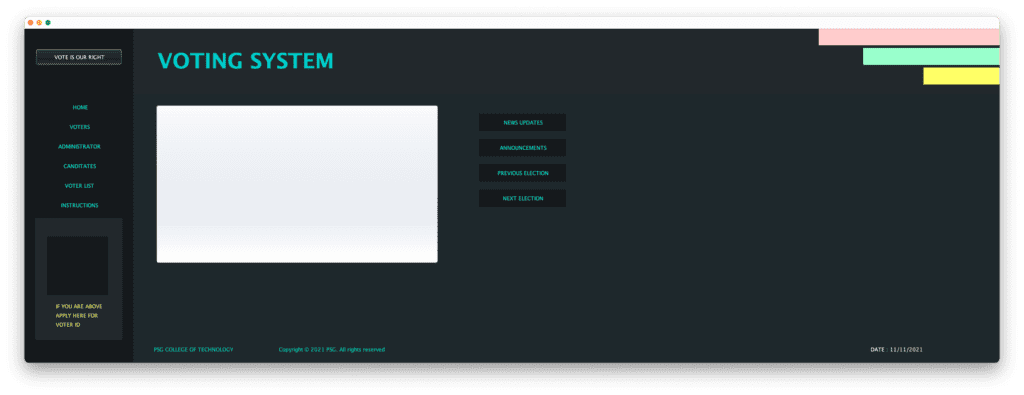
Conclusion:
Security, scalability and user interface are the issues to consider while creating a secure online voting system for the students using Java. Due to Spring Boot, Hibernate, and MySQL, you can develop sufficient architecture for meeting these requirements. The portability of Java, along with present day tools put it possible to build an efficient system that is secure.
Finally do check out the complete source code on GitHub and try to build your own version of an online voting system. Whatever the point is that, this project showcases the power and versatile platform independence of Java in creating modern web applications.
Find More Projects
how to create a video background with html CSS JavaScript Introduction Hello friends, you all are welcome to today’s new blog post. …
Auto Text Effect Animation Using Html CSS & JavaScript Introduction Hello friends, welcome to today’s new blog post. Today we have created …
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …