Car Rental System Using Java With Source Code
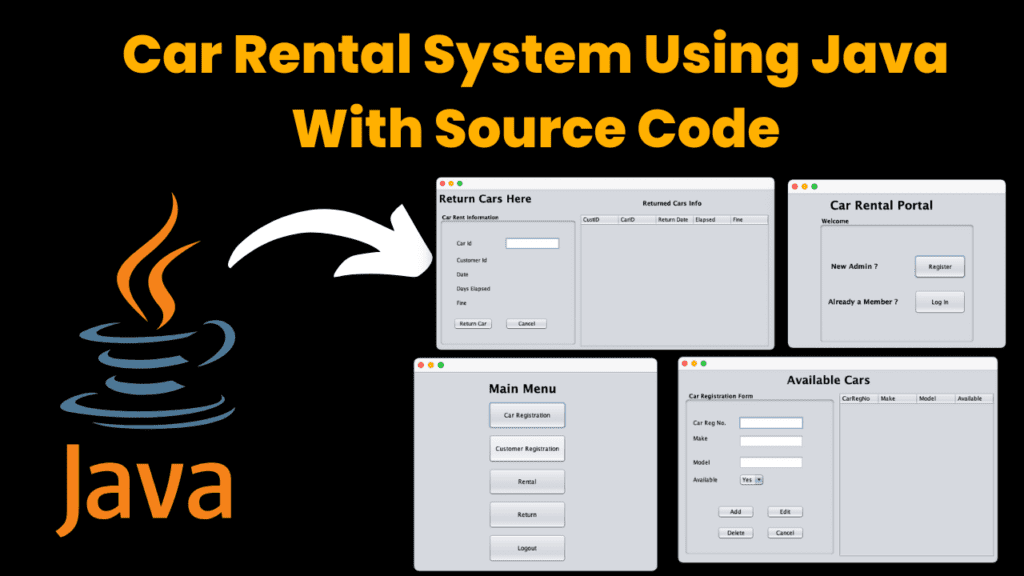
Introduction :
This Car Rental System project is developed to automate the process of renting cars, managing customer records, and handling rental transactions. Built using Java with MySQL as the database, this application aims to streamline car rental operations, making it easier for businesses to manage their fleet and customer data effectively.
The Car Rental System is a desktop application developed in Java using the Swing library for its graphical user interface (GUI) and MySQL for backend data management. This application is designed to streamline the process of renting cars, managing customer information, and handling transactions in a car rental business. Developed in NetBeans IDE, the project integrates multiple functionalities to ensure smooth and efficient management of car rental operations.
This blog post will guide you through the features, database structure, and core functionality of the Car Rental System, providing an overview of how it can be used to automate the workflow of a car rental business.
Features of the Car Rental System
- User Authentication: The system includes a secure login mechanism to authenticate users, ensuring that only authorized personnel can access and manage the rental operations.
- Car Registration Management: Allows for the addition, updating, and management of car details, including car number, make, model, and availability status.
- Customer Management: Facilitates adding new customers and maintaining their personal information, such as name, address, and contact details.
- Car Rental Management: Manages the rental process, including assigning cars to customers, calculating fees, and setting rental durations.
- Car Return Management: Handles the return process, including updating car status, calculating elapsed days, and applying fines if necessary.
- Dashboard: Provides an overview of all operations, including active rentals, available cars, and pending returns.
- Database Integration: Utilizes a MySQL database to store and manage data securely, ensuring data integrity and ease of access.
Tools Used
- Programming Language: Java (Swing)
- IDE: NetBeans
- Database: MySQL
Database Structure
The Car Rental System uses a MySQL database named “rentcar” to store all relevant data. The database consists of the following tables:
carregistration Table: Stores information about the cars available for rent.
- Fields:
id
(Primary Key): Unique identifier for each car.car_no
: The registration number of the car.make
: The car’s make (manufacturer).model
: The model of the car.available
: Status indicating whether the car is available for rent.
- Fields:
customer Table: Manages customer details.
- Fields:
id
(Primary Key): Unique identifier for each customer.cust_id
: Customer’s unique identification number.name
: Customer’s name.address
: Customer’s address.mobile
: Customer’s mobile number.
- Fields:
rental Table: Handles the rental transactions.
- Fields:
id
(Primary Key): Unique identifier for each rental transaction.car_id
: ID of the rented car.cust_id
: ID of the customer renting the car.fee
: Rental fee charged to the customer.date
: Start date of the rental.due
: Due date for the car return.
- Fields:
login Table: Stores login credentials for system users.
- Fields:
login_id
(Primary Key): Unique identifier for each login credential.username
: Username for the system user.password
: Password for the system user.
- Fields:
returncar Table: Manages car returns and fines.
- Fields:
id
(Primary Key): Unique identifier for each car return transaction.carid
: ID of the car being returned.custid
: ID of the customer returning the car.return_date
: The date on which the car was returned.elap
: The number of days elapsed since the rental.fine
: Any fine imposed for late returns.
- Fields:
Core Functionalities
1. Car Registration Management
The application allows users to register new cars into the system’s database. Details such as car number, make, model, and availability status can be added and updated. This functionality helps keep the car inventory up-to-date, ensuring that only available cars are listed for rental.
2. Customer Management
The system provides an interface for adding new customers and managing their details, including name, address, and contact number. Each customer is assigned a unique ID to distinguish their records in the database, making it easy to track customer information and rental history.
3. Car Rental Process
The rental management feature enables staff to assign cars to customers, calculate rental fees, and set the rental duration. The system stores all rental transactions in the rental
table, ensuring a comprehensive record of all rentals. It also updates the car’s availability status in real time.
4. Car Return and Fine Calculation
The application simplifies the car return process by allowing staff to update the status of returned cars. It calculates any fines for late returns based on the number of elapsed days since the due date and updates the records accordingly.
5. User Authentication and Security
The system includes a secure login mechanism, where user credentials are stored in the login
table. Only authorized users can access the application, protecting sensitive data from unauthorized access.
Required Modules and Packages:
This project requires the following modules and packages:
- Java Swing: Used for creating the graphical user interface (GUI).
- JDBC (Java Database Connectivity): Used to connect Java applications to the MySQL database.
- MySQL Connector/J: The official JDBC driver for MySQL, required to establish a connection between the Java application and the MySQL database.
To install these packages, use the following steps:
- Download and install the MySQL Connector/J for your Java version.
- Ensure that you have a working installation of NetBeans IDE.
- Make sure MySQL Server is installed and running on your system.
How to Run the Code:
To run this project on your local machine, follow these steps:
Download the Code from Below Link
Open the Project in NetBeans IDE:
- Open NetBeans and navigate to
File > Open Project
. - Select the cloned project directory.
- Open NetBeans and navigate to
Set Up the MySQL Database:
- Create a new database named
rentcar
. - Import the provided SQL script or manually create the tables:
carregistration
,customer
,rental
,login
,returncar
.
- Create a new database named
Configure the Database Connection:
- Modify the database connection parameters in the Java code to match your MySQL configuration (username, password, and database URL).
Build and Run the Project:
- Click on
Run > Run Project
or pressF6
in NetBeans to compile and execute the application.
- Click on
Code Explanation :
The code for this project is organized into several Java classes that handle different functionalities of the car rental system. Here are some key parts of the code:
1. Car Registration Module: The CarRegistration
class is responsible for adding new cars to the system’s database. It allows the admin to input car details such as car number, make, model, and availability status.
public void addCar(String carNo, String make, String model, boolean available) {
try {
String query = "INSERT INTO carregistration (car_no, make, model, available) VALUES (?, ?, ?, ?)";
PreparedStatement pst = connection.prepareStatement(query);
pst.setString(1, carNo);
pst.setString(2, make);
pst.setString(3, model);
pst.setBoolean(4, available);
pst.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
2. Customer Management Module: The Customer
class handles the addition and management of customer details.
public void addCustomer(String custId, String name, String address, String mobile) {
try {
String query = "INSERT INTO customer (cust_id, name, address, mobile) VALUES (?, ?, ?, ?)";
PreparedStatement pst = connection.prepareStatement(query);
pst.setString(1, custId);
pst.setString(2, name);
pst.setString(3, address);
pst.setString(4, mobile);
pst.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
3. Rental Management Module: The Rental
class manages the rental process, calculating fees and due dates.
public void rentCar(String carId, String custId, int fee, String date, String due) {
try {
String query = "INSERT INTO rental (car_id, cust_id, fee, date, due) VALUES (?, ?, ?, ?, ?)";
PreparedStatement pst = connection.prepareStatement(query);
pst.setString(1, carId);
pst.setString(2, custId);
pst.setInt(3, fee);
pst.setString(4, date);
pst.setString(5, due);
pst.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
Source Code:
Output :
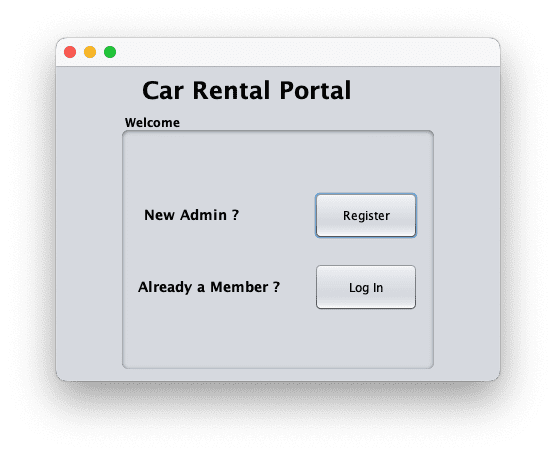
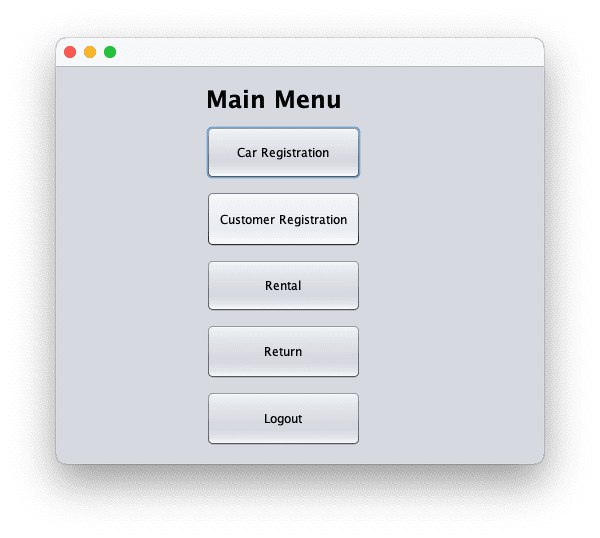
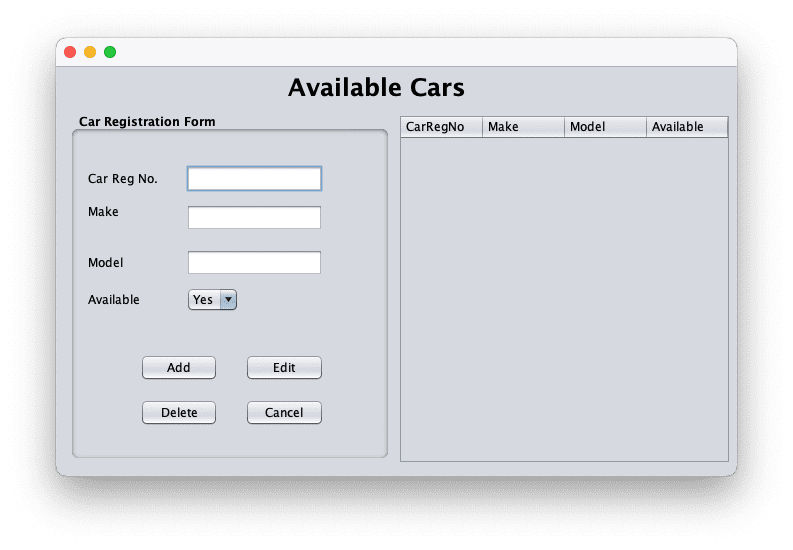
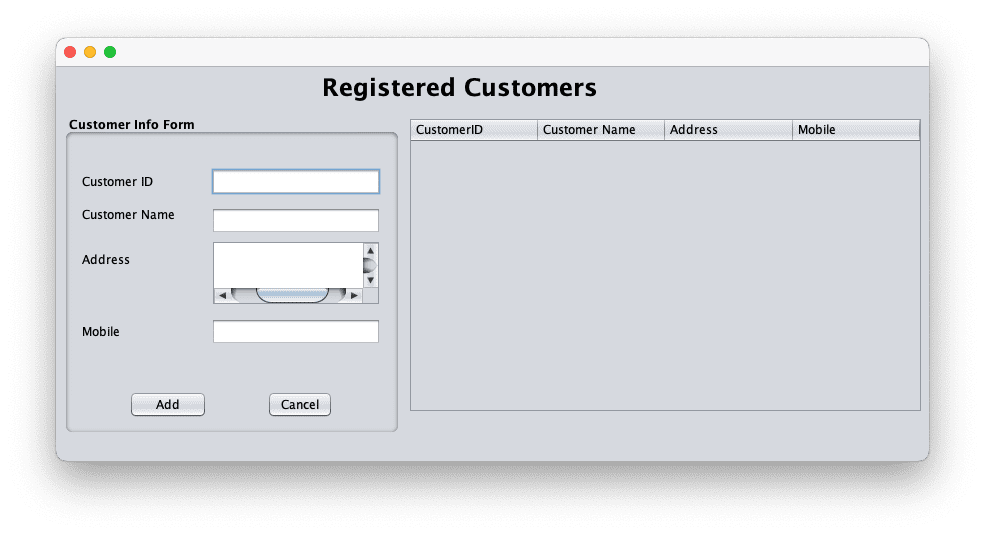
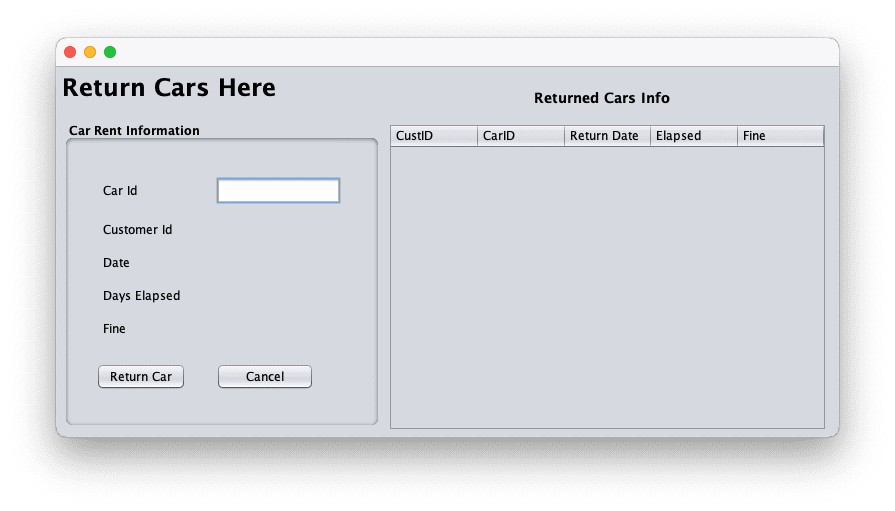
Conclusion :
The Car Rental System project is the solution for car renting companies where anyone can rent a car, and the administrators are able to organize and supervise the renting process. Due to the capabilities of Java, Swing, and the JDBC, the system maintains the automation of the rental process and the ultimate reliability of the rental business. Several optional features also can be added which may include procedures for online payment, car navigating by GPS, as well as providing more detailed reports to the administrators.
Therefore, the Car Rental System is a useful application for any car rental firm that needs to update its organization processes
Find More Projects
Drawing Ganesha Using Python Turtle Graphics[Drawing Ganapati Using Python] Introduction In this blog post, we will learn how to draw Lord Ganesha …
Contact Management System in Python with a Graphical User Interface (GUI) Introduction: The Contact Management System is a Python-based application designed to …
KBC Game using Python with Source Code Introduction : Welcome to this blog post on building a “Kaun Banega Crorepati” (KBC) game …
Basic Logging System in C++ With Source Code Introduction : It is one of the most important practices in software development. Logging …
Snake Game Using C++ With Source Code Introduction : The Snake Game is one of the most well-known arcade games, having its …
C++ Hotel Management System With Source Code Introduction : It is very important to manage the hotels effectively to avail better customer …