Budget Tracker using Java With Source Code
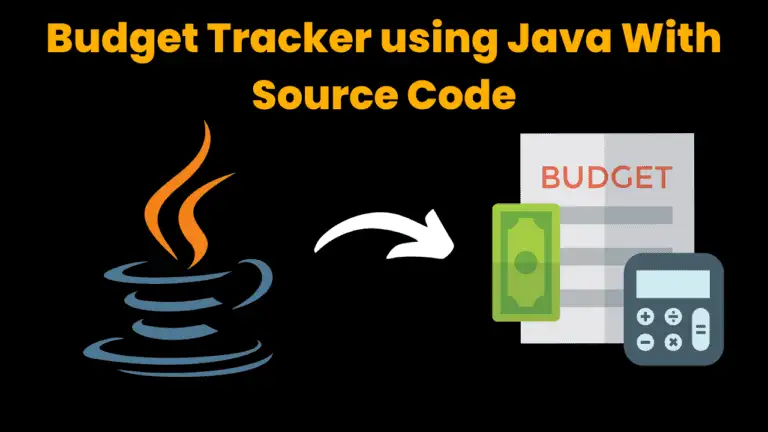
Introduction :
The above code is a sample implementation of a budget tracker in Java. The BudgetTracker class keeps track of a user’s expenses and incomes, and calculates the current budget balance.
The class has a constructor BudgetTracker()
, which initializes two ArrayList
objects, one for expenses and one for incomes, as well as a double variable balance
which is set to zero.
The class has three methods:
addExpense(double expense)
: This method takes in a double value as an argument, representing an expense, and adds it to the expensesArrayList
. It also subtracts the expense from the balance.addIncome(double income)
: This method takes in a double value as an argument, representing an income, and adds it to the incomesArrayList
. It also adds the income to the balance.getBalance()
: This method returns the current balanceprintExpenses()
: This method prints out all the expenses stored in the expensesArrayList
printIncomes()
: This method prints out all the incomes stored in the incomesArrayList
It’s worth noting that this is just a basic example, and you might want to add more features such as handling categories, displaying the balance in a specific currency,
Source Code
import java.util.ArrayList;
public class BudgetTracker {
private ArrayList expenses;
private ArrayList incomes;
private double balance;
public BudgetTracker() {
expenses = new ArrayList();
incomes = new ArrayList();
balance = 0;
}
public void addExpense(double expense) {
expenses.add(expense);
balance -= expense;
}
public void addIncome(double income) {
incomes.add(income);
balance += income;
}
public double getBalance() {
return balance;
}
public void printExpenses() {
System.out.println("Expenses: ");
for (double expense : expenses) {
System.out.println(expense);
}
}
public void printIncomes() {
System.out.println("Incomes: ");
for (double income : incomes) {
System.out.println(income);
}
}
}
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Output
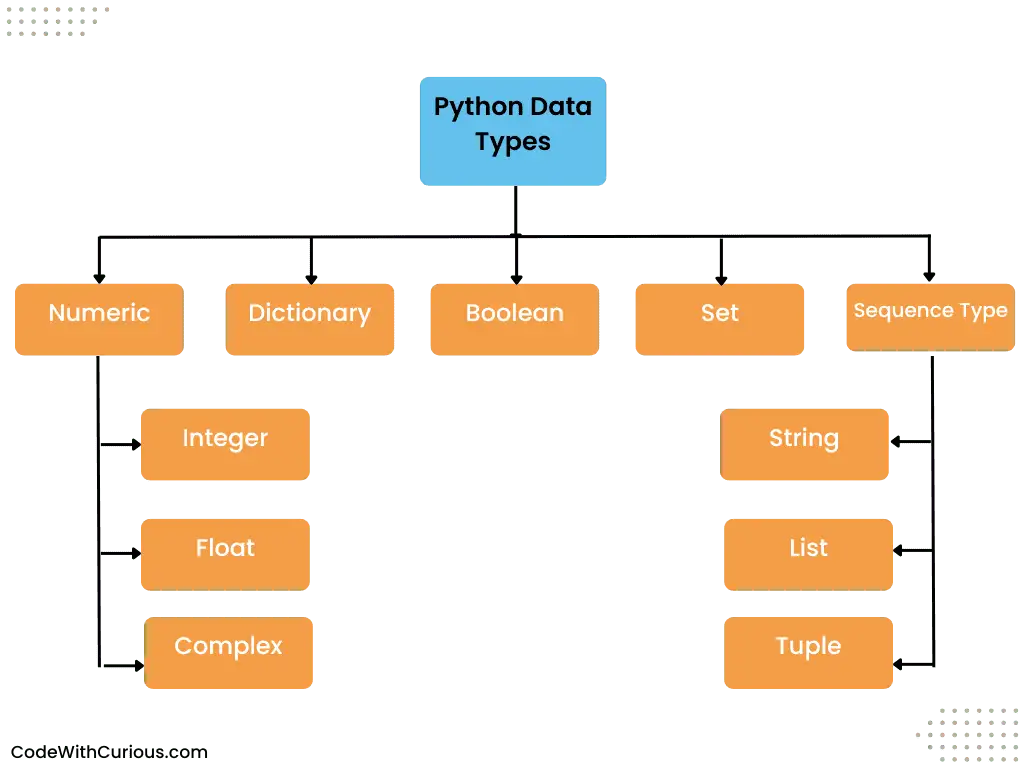
Find More Projects
Resume Builder Application using Java With Source Code Graphical User Interface [GUI] Introduction: The Resume Builder Application is a powerful and user-friendly …
Encryption Tool using java with complete source Code GUI Introduction: The Encryption Tool is a Java-based GUI application designed to help users …
Movie Ticket Booking System using Java With Source Code Graphical User Interface [GUI] Introduction: The Movie Ticket Booking System is a Java …
Video Call Website Using HTML, CSS, and JavaScript (Source Code) Introduction Hello friends, welcome to today’s new blog post. Today we have …
promise day using html CSS and JavaScript Introduction Hello all my developers friends my name is Gautam and everyone is welcome to …
Age Calculator Using HTML, CSS, and JavaScript Introduction Hello friends, my name is Gautam and you are all welcome to today’s new …