Attendance Management System Using Java With Source Code
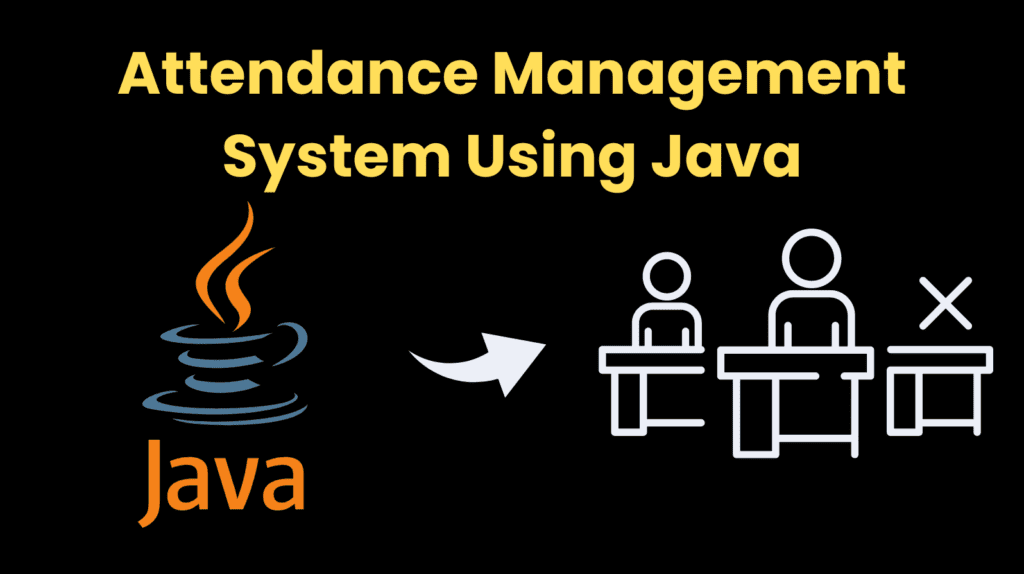
Introduction :
An AMS as the name suggests is an important tool that helps organizations manage their employees’ attendance data electronically. This blog will help you in developing an AMS from scratch using Advanced Java..
From this paper, we will discuss the language and its interface, crucial terminologies, necessary modules and packages, how to execute the code, actual code elaboration, as well as output.
Language and Interface :
Java is probably the most popular programming language at the present time and it has the advantages of operating on any platform, being secure and rather reliable. Java is basically extended and built on core Java with specific emphasis on web based applications.
In an AMS, a java interface is important in determining the characteristics of classes that are responsible for processing of operations in the system. In Java an interface is a kind of reference type which is like a class that can only have constants, method signatures, default methods, static methods and nested types. Interface cannot have instance variables or constructors as they are implemented only through the interface.
The key technologies used in this project include:
Servlets | Handle the basic logic of the application, processing requests and generating responses. |
JSP (Java Server Pages) | Create dynamic web content, combining Java with HTML to deliver rich user interfaces |
JDBC (Java Database Connectivity) | Connects and interact with the database, ensuring seamless connectivity and data manipulation. |
The interface is built using JSP for dynamic content and HTML/CSS for the layout and design, providing a user-friendly experience.
While developing the Attendance Management System, the project image or the overall structure of the system can be visualized as follows:
- Client-Side (Front-End):- It is a web-based interface that was developed using HTML, CSS and JSP hence the interaction with the system is done under a web browser.
- Server-Side (Back-End):– Manages the business logic level working with Servlets and controls the databases activity using JDBC.
- Database: – It has the documents of the attendance of every participant, users and any other clip that might be considered pertinent and stores them.
Here’s a simple diagram to illustrate the flow:
Some Important Terminologies:
Understanding some key terminologies is essential before diving into the code:
- Servlet: A Java class that processes requests from the client, performs operations on the server, and returns responses.
- JSP (JavaServer Pages): A technology that helps in developing dynamic web pages using Java embedded in HTML.
- JDBC (Java Database Connectivity): A standard API for connecting and executing queries with databases in Java.
- Session Management: A mechanism to maintain state across multiple client requests, typically used to track user sessions.
- DAO (Data Access Object): A design pattern that provides an abstract interface to the database, allowing for separation between business logic and data access.
Required Modules and Packages:
To build the Attendance Management System, the following modules and packages are required:
- Java Development Kit (JDK): The essential toolkit for Java development.
- Servlet API: For handling server-side business logic.
- JSP API: To develop the user interface with dynamic content.
- JDBC API: For database connectivity.
- Apache Tomcat: A web server to deploy and run your Java web applications.
- MySQL/PostgreSQL: A relational database to store attendance records.
How to Run the Code:
To run the Attendance Management System, follow these steps:
- Set Up the Environment:
- Install JDK, an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA, and Apache Tomcat.
- Set up the database (e.g., MySQL) and create tables for users, attendance records, etc.
- Configure the Project:
- Import the necessary libraries (Servlet, JSP, JDBC) into your IDE.
- Configure Tomcat in your IDE for deployment.
- Deploy the Application:
- Write the Java code (Servlets, JSP) and connect it with the database using JDBC.
- Deploy the project on Tomcat and start the server.
- Access the Application:
- Open a web browser and navigate to http://localhost:8080/AttendanceManagementSystem.
- Log in using the provided credentials and start managing attendance.
Code Explanation :
Here’s a brief overview of the key parts of the code:
- Servlet for Recording Attendance:
Java |
@WebServlet(“/RecordAttendanceServlet”) public class RecordAttendanceServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String empId = request.getParameter(“empId”); LocalDate date = LocalDate.now(); String status = “Present”; AttendanceService.recordAttendance(empId, date, status); response.sendRedirect(“attendanceSuccess.jsp”); } } |
Explanation: This Servlet processes the attendance form submitted by the user, records the attendance in the database, and redirects to a success page.
- JSP for User Interface:
Jsp |
<form action=”RecordAttendanceServlet” method=”post”> <input type=”text” name=”empId” placeholder=”Employee ID”> <input type=”submit” value=”Record Attendance”> </form> |
Explanation: This JSP file provides a simple form for recording attendance. The form data is sent to the RecordAttendanceServlet for processing.
- JDBC Connection:
Java |
Connection conn = DriverManager.getConnection(DB_URL, USER, PASS); String sql = “INSERT INTO attendance (empId, date, status) VALUES (?, ?, ?)”; PreparedStatement pstmt = conn.prepareStatement(sql); pstmt.setString(1, empId); pstmt.setDate(2, java.sql.Date.valueOf(date)); pstmt.setString(3, status); pstmt.executeUpdate(); |
Explanation: This code snippet connects to the database and inserts the attendance record. The use of PreparedStatement ensures secure database interactions.
Source Code :
Addstudent.java
import javax.swing.JOptionPane;
import javax.swing.GroupLayout.Alignment;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.GroupLayout;
import javax.swing.SwingConstants;
import java.awt.Component;
import java.awt.event.ActionListener;
import java.io.BufferedWriter;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.LineNumberReader;
import java.io.Writer;
import java.awt.event.ActionEvent;
@SuppressWarnings("unused")
public class Addstudent extends javax.swing.JFrame {
/**
*
*/
private static final long serialVersionUID = -9045446600138314933L;
public Addstudent() {
initComponents();
}
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jTextField1 = new javax.swing.JTextField();
jButton1 = new javax.swing.JButton();
jButton1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try{
String f ="src\\students.txt";
BufferedWriter output = new BufferedWriter(new FileWriter(f, true));
output.append(jTextField1.getText()+","+jTextField3.getText()+"\n");
output.newLine();
output.close();
dispose();
}
catch(Exception e1){
System.out.println(e1);
}
}
});
jButton2 = new javax.swing.JButton();
jLabel4 = new javax.swing.JLabel();
jTextField3 = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setText("Name");
jButton1.setText("OK");
jButton2.setText("Cancel");
jButton2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton2ActionPerformed(evt);
}
});
jLabel4.setFont(new java.awt.Font("Tahoma", 1, 15)); // NOI18N
jLabel4.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel4.setText("Add Student Info");
jLabel5.setText("Semester");
GroupLayout groupLayout = new GroupLayout(getContentPane());
groupLayout.setHorizontalGroup(
groupLayout.createParallelGroup(Alignment.TRAILING)
.addGroup(groupLayout.createSequentialGroup()
.addContainerGap(75, Short.MAX_VALUE)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addComponent(jButton1, GroupLayout.DEFAULT_SIZE, 67, Short.MAX_VALUE)
.addPreferredGap(ComponentPlacement.RELATED))
.addComponent(jLabel1))
.addGap(73))
.addGroup(groupLayout.createSequentialGroup()
.addComponent(jLabel5)
.addGap(88)))
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addComponent(jTextField3, GroupLayout.PREFERRED_SIZE, 136, GroupLayout.PREFERRED_SIZE)
.addComponent(jButton2, GroupLayout.PREFERRED_SIZE, 82, GroupLayout.PREFERRED_SIZE)
.addComponent(jTextField1, GroupLayout.PREFERRED_SIZE, 136, GroupLayout.PREFERRED_SIZE))
.addGap(25))
.addGroup(Alignment.LEADING, groupLayout.createSequentialGroup()
.addGap(92)
.addComponent(jLabel4, GroupLayout.PREFERRED_SIZE, 188, GroupLayout.PREFERRED_SIZE)
.addContainerGap(96, Short.MAX_VALUE))
);
groupLayout.setVerticalGroup(
groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel4)
.addGap(26)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(jLabel1)
.addComponent(jTextField1, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(18)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(jLabel5)
.addComponent(jTextField3, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(31)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(jButton1)
.addComponent(jButton2))
.addContainerGap())
);
getContentPane().setLayout(groupLayout);
pack();
}
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(1);
}
public static void main(String args[]) {
new Addstudent().setVisible(true);
}
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton2;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JTextField jTextField1;
private javax.swing.JTextField jTextField3;
}
Addteacher.java
import javax.swing.JOptionPane;
import javax.swing.GroupLayout.Alignment;
import javax.swing.GroupLayout;
import javax.swing.LayoutStyle.ComponentPlacement;
import java.awt.event.ActionListener;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.awt.event.ActionEvent;
@SuppressWarnings("unused")
public class Addteacher extends javax.swing.JFrame {
/**
*
*/
private static final long serialVersionUID = 2424801300343983088L;
public Addteacher() {
initComponents();
}
private void initComponents() {
jLabel2 = new javax.swing.JLabel();
jTextField2 = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
jTextField3 = new javax.swing.JTextField();
jLabel4 = new javax.swing.JLabel();
jTextField4 = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
jButton1 = new javax.swing.JButton();
jButton2 = new javax.swing.JButton();
jButton2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try{
String f ="src\\users.txt";
BufferedWriter output = new BufferedWriter(new FileWriter(f, true));
output.newLine();
output.append("\n"+jTextField3.getText()+","+jTextField4.getText());
output.close();
dispose();
}
catch(Exception e1){
System.out.println(e1);
}
}
});
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel2.setText("Name");
jLabel3.setText("Username");
jLabel4.setText("Password");
jLabel5.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel5.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel5.setText("ADD TEACHER");
jButton1.setText("Cancel");
jButton2.setText("Add");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
layout.setHorizontalGroup(
layout.createParallelGroup(Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(109)
.addGroup(layout.createParallelGroup(Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addComponent(jButton2, GroupLayout.PREFERRED_SIZE, 66, GroupLayout.PREFERRED_SIZE)
.addGap(63)
.addComponent(jButton1)
.addGap(30))
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(121)
.addComponent(jLabel5, GroupLayout.PREFERRED_SIZE, 222, GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addGap(109)
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel3, GroupLayout.PREFERRED_SIZE, 62, GroupLayout.PREFERRED_SIZE)
.addGap(18)
.addComponent(jTextField3, GroupLayout.PREFERRED_SIZE, 137, GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel4, GroupLayout.PREFERRED_SIZE, 62, GroupLayout.PREFERRED_SIZE)
.addGap(18)
.addComponent(jTextField4, GroupLayout.PREFERRED_SIZE, 137, GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel2, GroupLayout.PREFERRED_SIZE, 62, GroupLayout.PREFERRED_SIZE)
.addGap(18)
.addComponent(jTextField2, GroupLayout.PREFERRED_SIZE, 137, GroupLayout.PREFERRED_SIZE))))))
.addContainerGap(157, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(27)
.addComponent(jLabel5, GroupLayout.PREFERRED_SIZE, 26, GroupLayout.PREFERRED_SIZE)
.addGap(46)
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addComponent(jTextField2, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel2))
.addPreferredGap(ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(Alignment.BASELINE)
.addComponent(jTextField3, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel3))
.addPreferredGap(ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(Alignment.BASELINE)
.addComponent(jTextField4, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel4))
.addGap(52)
.addGroup(layout.createParallelGroup(Alignment.BASELINE)
.addComponent(jButton1, GroupLayout.PREFERRED_SIZE, 36, GroupLayout.PREFERRED_SIZE)
.addComponent(jButton2, GroupLayout.PREFERRED_SIZE, 36, GroupLayout.PREFERRED_SIZE))
.addContainerGap(64, Short.MAX_VALUE))
);
getContentPane().setLayout(layout);
pack();
}
public static void main(String args[]) {
new Addteacher().setVisible(true);
}
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton2;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JTextField jTextField2;
private javax.swing.JTextField jTextField3;
private javax.swing.JTextField jTextField4;
}
Attendanceproj.java
public class Attendanceproj {
public static void main(String[] args) {
Entryportal f1 = new Entryportal();
f1.setVisible(true);
}
}
Chemistry.txt
Student,Present/Absent
1,Present
3,Absent
Entryportal.java
import javax.swing.JOptionPane;
import javax.swing.GroupLayout.Alignment;
import javax.swing.GroupLayout;
import java.awt.Font;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.JTextField;
import java.io.*;
import java.util.*;
@SuppressWarnings("unused")
public class Entryportal extends javax.swing.JFrame {
/**
*
*/
private static final long serialVersionUID = 7522526792628754248L;
public Entryportal() {
initComponents();
}
@SuppressWarnings({ "rawtypes", "unchecked" })
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel1.setFont(new Font("Tahoma", Font.PLAIN, 16));
jLabel2 = new javax.swing.JLabel();
jLabel2.setFont(new Font("Tahoma", Font.PLAIN, 16));
jLabel3 = new javax.swing.JLabel();
jLabel3.setFont(new Font("Tahoma", Font.PLAIN, 16));
userid = new javax.swing.JTextField();
mode = new javax.swing.JComboBox();
loginb = new javax.swing.JButton();
cancelb = new javax.swing.JButton();
jLabel4 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Login Information");
setName("Entryportal");
jLabel1.setText("Username");
jLabel2.setText("Password");
jLabel3.setText("User Type");
mode.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Administrator", "Teacher" }));
loginb.setText("Login");
loginb.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
cancelb.setText("Cancel");
cancelb.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton2ActionPerformed(evt);
}
});
jLabel4.setFont(new java.awt.Font("Tahoma", 0, 18)); // NOI18N
jLabel4.setText("ATTENDANCE MANAGEMENT SYSTEM");
pwd = new JTextField();
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
layout.setHorizontalGroup(
layout.createParallelGroup(Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(87)
.addComponent(jLabel4, GroupLayout.PREFERRED_SIZE, 324, GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(68)
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addComponent(jLabel2)
.addGroup(layout.createSequentialGroup()
.addPreferredGap(ComponentPlacement.RELATED)
.addComponent(jLabel3, GroupLayout.PREFERRED_SIZE, 88, GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel1)))
.addGroup(layout.createSequentialGroup()
.addGap(76)
.addComponent(loginb, GroupLayout.PREFERRED_SIZE, 108, GroupLayout.PREFERRED_SIZE)))
.addGap(79)
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addComponent(cancelb, GroupLayout.PREFERRED_SIZE, 106, GroupLayout.PREFERRED_SIZE)
.addGroup(layout.createParallelGroup(Alignment.TRAILING)
.addComponent(mode, GroupLayout.PREFERRED_SIZE, 150, GroupLayout.PREFERRED_SIZE)
.addComponent(userid, GroupLayout.PREFERRED_SIZE, 150, GroupLayout.PREFERRED_SIZE)
.addComponent(pwd, GroupLayout.PREFERRED_SIZE, 150, GroupLayout.PREFERRED_SIZE)))))
.addContainerGap(89, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel4, GroupLayout.PREFERRED_SIZE, 63, GroupLayout.PREFERRED_SIZE)
.addGap(44)
.addGroup(layout.createParallelGroup(Alignment.BASELINE)
.addComponent(jLabel1)
.addComponent(userid, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(36)
.addGroup(layout.createParallelGroup(Alignment.LEADING)
.addComponent(jLabel2)
.addComponent(pwd, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(31)
.addGroup(layout.createParallelGroup(Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(mode, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(58)
.addGroup(layout.createParallelGroup(Alignment.LEADING, false)
.addComponent(cancelb, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(loginb, GroupLayout.DEFAULT_SIZE, 45, Short.MAX_VALUE))
.addGap(56))
);
getContentPane().setLayout(layout);
pack();
}
@SuppressWarnings("resource")
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
try {
String username = userid.getText();
String password = pwd.getText();
String file = "src\\users.txt";
BufferedReader br=new BufferedReader(new FileReader(file));
String line, user, pass;
boolean isLoginSuccess = false;
while ((line = br.readLine()) != null) {
user = line.split(",")[0];
pass = line.split(",")[1];
if (user.equals(username) && pass.equals(password)) {
isLoginSuccess = true;
}
}
if (isLoginSuccess) {
JOptionPane.showMessageDialog(null, "Succesfully logged in");
if( mode.getSelectedItem()=="Administrator")
{
admin f2 = new admin(userid.getText());
f2.setVisible(true);
dispose();
}
else
if( mode.getSelectedItem()=="Teacher")
{
Teacher1 f2 = new Teacher1(userid.getText());
f2.setVisible(true);
dispose();
}
} else {
JOptionPane.showMessageDialog(null, "Invalid username or paswword");
}
} catch (Exception e) {
System.out.println("Somethig is wrong");
JOptionPane.showMessageDialog(null, e);
}
}
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(1);
}
public static void main(String args[]) {
new Entryportal().setVisible(true);
}
private javax.swing.JTextField userid;
@SuppressWarnings("rawtypes")
private javax.swing.JComboBox mode;
private javax.swing.JButton loginb;
private javax.swing.JButton cancelb;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private JTextField pwd;
}
Takeattendance.java
import javax.swing.JOptionPane;
import javax.swing.GroupLayout.Alignment;
import javax.swing.GroupLayout;
import javax.swing.JComboBox;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.JLabel;
import java.awt.Font;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
@SuppressWarnings("unused")
public class Takeattendence extends javax.swing.JFrame {
/**
*
*/
private static final long serialVersionUID = 3502024109032056383L;
public Takeattendence(String k) {
initComponents(k);
}
public void UpdateJTable(){
}
@SuppressWarnings("unchecked")
private void initComponents(String s) {
String sub=s;
jLabel1 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel1.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel1.setText("ATTENDANCE REPORT FOR STUDENTS STUDYING");
@SuppressWarnings("rawtypes")
JComboBox stucb = new JComboBox();
JLabel lblStudent = new JLabel("Student");
lblStudent.setFont(new Font("Tahoma", Font.PLAIN, 16));
JButton btnAbsent = new JButton("Absent");
btnAbsent.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String stu = (String) stucb.getSelectedItem();
try{
String f ="src\\"+sub+".txt";
BufferedWriter output = new BufferedWriter(new FileWriter(f, true));
output.append(stu+",Absent"+"\n");
output.newLine();
output.close();
}
catch(Exception e1){
System.out.println(e1);
}
}
});
JButton btnPresent = new JButton("Present");
btnPresent.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String stu = (String) stucb.getSelectedItem();
try{
String f ="src\\"+sub+".txt";
BufferedWriter output = new BufferedWriter(new FileWriter(f, true));
output.append(stu+",Present"+"\n");
output.newLine();
output.close();
}
catch(Exception e1){
System.out.println(e1);
}
}
});
JButton btnSubmit = new JButton("Submit");
btnSubmit.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit(1);
}
});
try {
String file = "src\\students.txt";
@SuppressWarnings("resource")
BufferedReader br=new BufferedReader(new FileReader(file));
String line, stu;
while ((line = br.readLine()) != null) {
stu = line.split(",")[0];
stucb.addItem(stu);
}
} catch (Exception e) {
System.out.println("Somethig is wrong");
JOptionPane.showMessageDialog(null, e);
}
GroupLayout groupLayout = new GroupLayout(getContentPane());
groupLayout.setHorizontalGroup(
groupLayout.createParallelGroup(Alignment.TRAILING)
.addGroup(groupLayout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel1, GroupLayout.PREFERRED_SIZE, 433, GroupLayout.PREFERRED_SIZE)
.addContainerGap(35, Short.MAX_VALUE))
.addGroup(groupLayout.createSequentialGroup()
.addContainerGap(86, Short.MAX_VALUE)
.addComponent(lblStudent)
.addGap(58)
.addComponent(stucb, GroupLayout.PREFERRED_SIZE, 172, GroupLayout.PREFERRED_SIZE)
.addGap(108))
.addGroup(groupLayout.createSequentialGroup()
.addGap(107)
.addComponent(btnAbsent)
.addGap(90)
.addComponent(btnPresent)
.addContainerGap(145, Short.MAX_VALUE))
.addGroup(Alignment.LEADING, groupLayout.createSequentialGroup()
.addGap(184)
.addComponent(btnSubmit, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGap(227))
);
groupLayout.setVerticalGroup(
groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel1)
.addGap(18)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(stucb, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(lblStudent, GroupLayout.PREFERRED_SIZE, 14, GroupLayout.PREFERRED_SIZE))
.addGap(18)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(btnPresent)
.addComponent(btnAbsent))
.addGap(18)
.addComponent(btnSubmit)
.addContainerGap(35, Short.MAX_VALUE))
);
getContentPane().setLayout(groupLayout);
pack();
}
public static void main(String args[]) {
new Takeattendence("Maths").setVisible(true);
}
private javax.swing.JLabel jLabel1;
}
Teacher1.java
import javax.swing.JOptionPane;
import javax.swing.DefaultComboBoxModel;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.FileReader;
import java.awt.event.ActionEvent;
@SuppressWarnings("unused")
public class Teacher1 extends javax.swing.JFrame {
/**
*
*/
private static final long serialVersionUID = -5925756146593045815L;
public Teacher1(String s) {
initComponents(s);
}
String iddd = null;
@SuppressWarnings({ "rawtypes", "unchecked" })
private void initComponents(String s) {
String name = s;
jLabel1 = new javax.swing.JLabel();
sub = new javax.swing.JComboBox();
sub.setModel(new DefaultComboBoxModel(new String[] {"Maths", "Physics", "Chemistry"}));
jLabel2 = new javax.swing.JLabel();
jButton1 = new javax.swing.JButton();
jButton1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
Takeattendence t = new Takeattendence((String) sub.getSelectedItem());
t.setVisible(true);
dispose();
}
});
jButton2 = new javax.swing.JButton();
jButton2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Report t = new Report((String) sub.getSelectedItem());
t.setVisible(true);
dispose();
}
});
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Tahoma", 1, 18));
jLabel1.setText("WELCOME "+name);
sub.setEditable(true);
jLabel2.setText("Choose Subject");
jButton1.setText("Take Attendance");
jButton2.setText("Generate Report");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(72, 72, 72)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton1, javax.swing.GroupLayout.DEFAULT_SIZE, 136, Short.MAX_VALUE)
.addComponent(jLabel2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(102, 102, 102)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(sub, 0, 133, Short.MAX_VALUE))
.addContainerGap(97, Short.MAX_VALUE))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 222, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(158, 158, 158))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(30, 30, 30)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(72, 72, 72)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(sub, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel2))
.addGap(71, 71, 71)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButton1)
.addComponent(jButton2))
.addContainerGap(83, Short.MAX_VALUE))
);
pack();
}
public static void main(String args[]) {
new Teacher1("Maths").setVisible(true);
}
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton2;
@SuppressWarnings("rawtypes")
private javax.swing.JComboBox sub;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
}
Report.java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
@SuppressWarnings("unused")
public class Report extends javax.swing.JFrame {
/**
*
*/
private static final long serialVersionUID = 3502024109032056383L;
public Report(String k) {
initComponents(k);
}
private void initComponents(String sub) {
jLabel1 = new javax.swing.JLabel();
jScrollPane2 = new javax.swing.JScrollPane();
jTable2 = new javax.swing.JTable();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel1.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel1.setText("ATTENDANCE REPORT FOR STUDENTS STUDYING");
jTable2.setAutoCreateRowSorter(true);
jScrollPane2.setViewportView(jTable2);
String filePath = "src\\"+sub+".txt";
File file = new File(filePath);
try {
@SuppressWarnings("resource")
BufferedReader br = new BufferedReader(new FileReader(file));
String firstLine = br.readLine().trim();
String[] columnsName = firstLine.split(",");
DefaultTableModel model = (DefaultTableModel)jTable2.getModel();
model.setColumnIdentifiers(columnsName);
Object[] tableLines = br.lines().toArray();
for(int i = 0; i < tableLines.length; i++)
{
String line = tableLines[i].toString().trim();
String[] dataRow = line.split(",");
model.addRow(dataRow);
}
} catch (Exception ex) {
}
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(29, 29, 29)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 433, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(28, Short.MAX_VALUE))
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(40, 40, 40)
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 401, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(49, Short.MAX_VALUE)))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(30, 30, 30)
.addComponent(jLabel1)
.addContainerGap(420, Short.MAX_VALUE))
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(101, 101, 101)
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 310, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(56, Short.MAX_VALUE)))
);
pack();
}
// public static void main(String args[]) {
// new Report("Maths").setVisible(true);
// }
private javax.swing.JLabel jLabel1;
private javax.swing.JScrollPane jScrollPane2;
private javax.swing.JTable jTable2;
}
Maths.txt
Student,Present/Absent
1,Present
1,Absent
1,Present
1,Absent
1,Present
null,Absent
Students.txt
1,2
3,4
Users.txt
teacher1,tkey1
teacher2,tkey2
teacher3,tkey3
teacher4,tkey4
student1,skey1
student2,skey2
student3,skey3
student4,skey4
student5,skey5
1,2
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …