Snake Game Using C++ With Source Code
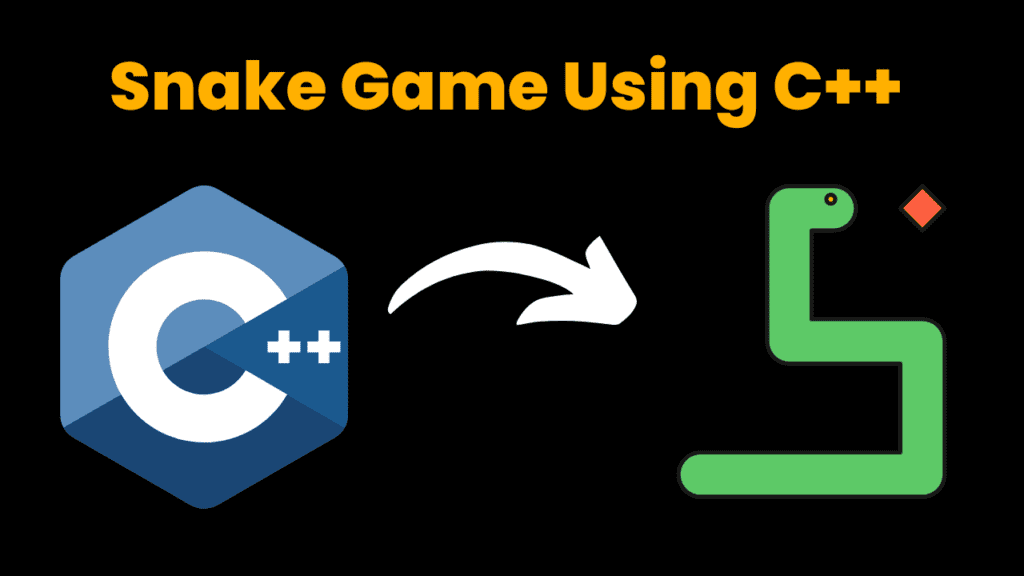
Introduction :
The Snake Game is one of the most well-known arcade games, having its roots in the late 1970s and 1980s. It gained immense popularity with its release on early mobile phones, particularly the Nokia models, where it became a staple game for millions of users worldwide. The premise of the Snake Game is simple yet highly engaging: the player controls a snake that moves around the screen, consuming food items that appear randomly. Each time the snake consumes a piece of food, it grows longer, and the player’s score increases. The game continues until the snake either collides with the screen’s boundaries or with itself, at which point the game is over.
The Snake Game has been a classic example of how minimalistic gameplay, simple controls, and an intuitive interface can lead to addictive gameplay. It not only provides a fun way to pass time but also enhances the player’s reflexes and strategic thinking. As the snake grows longer, maneuvering it without crashing becomes more challenging, requiring quick decision-making and hand-eye coordination.
This project involves implementing the Snake Game using C++, a powerful and widely-used programming language known for its performance and control over system resources. The game is created using the graphics.h
library, which provides a simple way to create graphical interfaces, making it suitable for beginners who are learning C++ and want to venture into game development. In this version of the Snake Game, the snake and the food are visually represented as blocks—a white block for the snake and a red block for the food. Players control the snake’s movement using the keyboard: ‘W’ for up, ‘A’ for left, ‘S’ for down, and ‘D’ for right.
The game integrates basic collision detection to check whether the snake has hit the walls or collided with itself. Additionally, the game dynamically updates the score based on the number of fruits eaten by the snake, providing a clear indication of the player’s progress. This project serves as an excellent introduction to game development fundamentals, such as handling user input, managing game state, and rendering graphics on the screen.
By creating this game, you will gain experience in using basic C++ concepts like loops, conditionals, and functions while also learning about graphics programming, memory management, and real-time processing. Additionally, you will become familiar with common game development concepts such as collision detection, dynamic object management, and scorekeeping, all of which are crucial for building more complex games in the future. This project is an ideal starting point for aspiring game developers looking to develop their skills and build a portfolio.
Modules or Packages required:
graphics.h:
Responsible for graphical output. It allows us to draw various geometric shapes and text on a window, which is essential for rendering game elements and the interface.
conio.h:
Provides useful keyboard input functions, such as kbhit()
and getch()
, which are used to detect key presses in real-time to control the snake.
stdlib.h:
Standard library for various general-purpose functions like dynamic memory allocation and random number generation.
stdio.h:
Standard I/O functions for handling input and output operations.
time.h:
Used for time manipulation and generating random numbers, which is crucial for placing the fruits at random positions.
How to run the code :-
Install a compatible C++ compiler and the graphics library.
Download or clone the project repository from GitHub.
Open a terminal or command prompt and navigate to the project directory.
Compile the code using a C++ compiler. For example:
g++ -o snake_game snake_game.cpp -lgraph
Run the executable file to start the game:
./snake_game
Explanation of Code :
The code is structured into several main functions:
start()
: Initializes the game window and sets up the initial game state, including drawing the boundary and placing the first fruit.update()
: Called in every frame to update the game’s state, move the snake, check for collisions, and refresh the graphics.move()
: Handles the snake’s movements based on user input, modifying the direction. It also prevents the snake from turning back directly on itself.graphic()
: Redraws the game window with the snake, fruit, and score to refresh the game display.fruit()
: Manages the generation and placement of the fruit. It also checks if the snake has eaten the fruit and updates its length and score accordingly.
Below is the key part of the move()
function that handles the snake’s movement:
void move() {
if (kbhit()) {
direc = getch();
}
// Prevent the snake from moving directly back onto itself
if ((direc == 'w' && last == 's') || (direc == 's' && last == 'w') ||
(direc == 'a' && last == 'd') || (direc == 'd' && last == 'a')) {
direc = last;
}
last = direc;
// Update snake position based on the direction
if (direc == 's') {
y1 += 10;
} else if (direc == 'a') {
x1 -= 10;
} else if (direc == 'w') {
y1 -= 10;
} else if (direc == 'd') {
x1 += 10;
}
}
This function reads keyboard input to change the direction of snakes and prevents the snake from moving in reverse directly.
Source Code :
snake_game.cpp
#include
#include
#include
#include
#include
#include
using namespace std;
int length = 2;
int lengthmax = 2; // Controls snake's length
int x1 = 30;
int y1 = 170; // Snake's location
char direc = '-';
char last = '-'; // Direction
bool playing = true; // Player status
int fruitx;
int fruity;
bool eaten = false; // Fruit status
vector snakex;
vector snakey; // Snake's tail coordinates
int i = 1;
int imax = 0; // Loop control
char score[50];
int score1 = 0; // Player's score
void fruit();
void graphic();
void move();
void start();
void update(); // Function declarations
int main() {
start();
while (playing) {
update();
if (x1 >= 340 || x1 < 0 || y1 >= 340 || y1 < 0) {
playing = false; // Check collision with walls
}
for (i = lengthmax; i > 0; i--) {
if (x1 == snakex[i] && y1 == snakey[i]) {
playing = false; // Check collision with itself
}
}
}
if (!playing) {
cleardevice();
outtextxy(70, 170, "YOU LOST, YOUR ");
outtextxy(188, 170, score);
getch();
return 0; // End game
}
}
void start() {
sprintf(score, "SCORE : %d ", score1);
srand(time(NULL));
initwindow(350, 354, "SnakeGame");
setcolor(GREEN);
setfillstyle(SOLID_FILL, GREEN);
rectangle(0, 0, 340, 10);
floodfill(5, 5, GREEN);
setcolor(WHITE);
outtextxy(10, 0, score);
fruitx = (rand() % 34) * 10;
fruity = (rand() % 34) * 10;
if (fruity == 0) {
fruity += 10;
}
setcolor(RED);
setfillstyle(SOLID_FILL, RED);
rectangle(fruitx, fruity, fruitx + 10, fruity + 10);
floodfill(fruitx + 5, fruity + 5, RED);
}
void update() {
sprintf(score, "SCORE : %d ", score1);
delay(150);
snakex.push_back(x1);
snakey.push_back(y1); // Update tail positions
fruit();
move();
graphic();
}
void move() {
if (kbhit()) {
direc = getch();
}
if ((direc == 'w' && last == 's') || (direc == 's' && last == 'w') ||
(direc == 'a' && last == 'd') || (direc == 'd' && last == 'a')) {
direc = last;
}
last = direc;
if (direc == 's') {
y1 += 10;
} else if (direc == 'a') {
x1 -= 10;
} else if (direc == 'w') {
y1 -= 10;
} else if (direc == 'd') {
x1 += 10;
}
}
void graphic() {
cleardevice();
setcolor(GREEN);
setfillstyle(SOLID_FILL, GREEN);
rectangle(0, 0, 350, 10);
floodfill(5, 5, GREEN);
setcolor(WHITE);
outtextxy(10, 0, score);
setcolor(RED);
setfillstyle(SOLID_FILL, RED);
rectangle(fruitx, fruity, fruitx + 10, fruity + 10);
floodfill(fruitx + 5, fruity + 5, RED);
setcolor(WHITE);
setfillstyle(SOLID_FILL, WHITE);
rectangle(x1, y1, x1 + 10, y1 + 10);
floodfill(x1 + 5, y1 + 5, WHITE);
for (int i = lengthmax; i > 0; i--) {
setcolor(WHITE);
setfillstyle(SOLID_FILL, WHITE);
rectangle(snakex[i], snakey[i], snakex[i] + 10, snakey[i] + 10);
floodfill(snakex[i] + 5, snakey[i] + 5, WHITE);
}
imax++;
i = imax;
}
void fruit() {
if (x1 == fruitx && y1 == fruity) {
eaten = true;
}
if (eaten) {
eaten = false;
lengthmax++;
score1++;
fruitx = (rand() % 34) * 10;
fruity = (rand() % 34) * 10;
for (int i = lengthmax; i > 0; i--) {
if (fruitx == snakex[i] && fruity == snakey[i]) {
fruitx = (rand() % 34) * 10;
fruity = (rand() % 34) * 10;
}
}
if (fruity == 0) {
fruity = 10;
}
setcolor(RED);
setfillstyle(SOLID_FILL, RED);
rectangle(fruitx, fruity, fruitx + 10, fruity + 10);
floodfill(fruitx + 5, fruity + 5, RED);
}
}
Output :
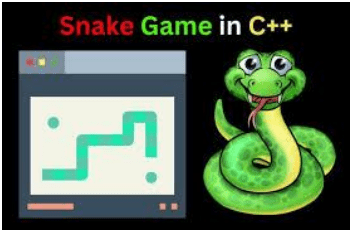
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …