Movie Ticket Booking System using Java With Source Code Graphical User Interface [GUI]
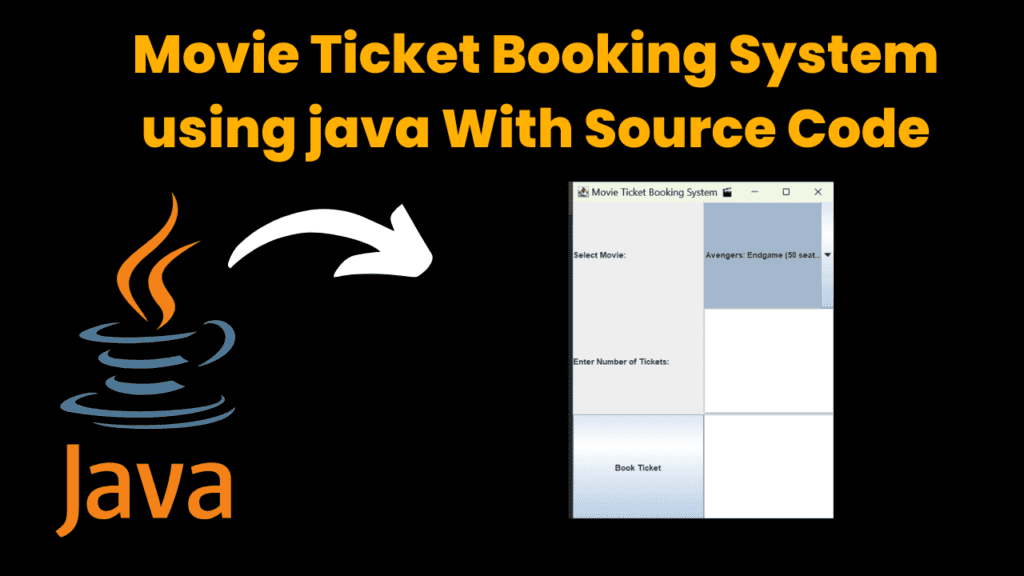
Introduction:
The Movie Ticket Booking System is a Java Swing-based application that allows users to browse movies, book tickets, and view their booking details in an easy-to-use graphical interface. It provides a seamless and interactive experience for users, ensuring that they can select their favorite movie, check seat availability, and book tickets with just a few clicks. This system allows real-time seat updates, preventing overbooking issues. It is designed with a clean and intuitive user interface, making it user-friendly for both beginners and advanced users. The application also implements error handling and input validation, ensuring a smooth booking process.
Features
- User-Friendly GUI
- Browse Available Movies
- Book Movie Tickets
- View Booking Details
- Error Handling & Validation
- Dynamic Seat Management
- Real-Time Updates
Required Modules:
javax.swing.*
: This import is necessary for creating all the graphical user interface components like frames, buttons, labels, text fields, combo boxes, etc.java.awt.*
: Provides basic drawing capabilities and layout management (like GridLayout for organizing components).java.awt.event.*
: Needed for handling events like button clicks, which trigger actions in the program.java.util.ArrayList
: Used to dynamically store and manage lists of Movies and Bookings.
How to Run the Code:
1. Install Java Development Kit (JDK)
Ensure you have the JDK installed on your computer. You can download the latest version of JDK from the official Oracle website or use OpenJDK.
To check if you have Java installed, open the terminal (Command Prompt on Windows) and run
java -version
If Java is installed, you’ll see a version number. If not, you’ll need to install it.
2. Save the Code
- Save the file as
MovieTicketBookingGUI.java
.
3. Compile the Code
Open a command prompt (Windows) or terminal (macOS/Linux).
Navigate to the folder where you saved the
MovieTicketBookingGUI.java
file.Use
cd
command to navigate. For example:cd C:\path\to\your\folder
Compile the Java code using the
javac
command:javac MovieTicketBookingGUI.java
- This will compile the Java file and create a
.class
file (bytecode), which is needed to run the program.
- This will compile the Java file and create a
4. Run the Program
Run the compiled code using the
java
command:java MovieTicketBookingGUI
If everything is set up correctly, this will launch the Movie Ticket Booking System with a graphical interface where you can browse movies and book tickets.
Code Explanation :
1. Movie Class
The Movie
class is responsible for holding information about a movie, such as the movie’s name and the number of available seats. It also includes methods to book seats.
class Movie {
private String name;
private int availableSeats;
// Constructor to initialize movie name and available seats
public Movie(String name, int seats) {
this.name = name;
this.availableSeats = seats;
}
// Getters
public String getName() {
return name;
}
public int getAvailableSeats() {
return availableSeats;
}
// Method to book seats by reducing available seats
public void bookSeats(int seats) {
if (seats <= availableSeats) {
availableSeats -= seats; // Reduce available seats
}
}
// Override toString to display movie info in the JComboBox
public String toString() {
return name + " (" + availableSeats + " seats)";
}
}
Purpose:
- The
Movie
class holds the movie’s name and the number of available seats. bookSeats(int seats)
: This method is used to update the available seats when a user books tickets.
2. Booking Class
The Booking
class is used to store the booking details like the movie name and the number of seats booked by the user.
class Booking {
private String movieName;
private int bookedSeats;
// Constructor to store booking details
public Booking(String movieName, int bookedSeats) {
this.movieName = movieName;
this.bookedSeats = bookedSeats;
}
// Method to get booking details
public String getDetails() {
return "š„ Movie: " + movieName + " | šļø Tickets: " + bookedSeats;
}
}
Purpose:
- The
Booking
class helps store the booking information for each user, which includes the movie name and the number of seats booked. getDetails()
: Displays the booking summary (movie name and number of seats).
3. MovieTicketBookingGUI Class (Main Class)
This is the core class that ties everything together and displays the GUI. It uses Java Swing components like JComboBox
, JTextField
, and JTextArea
to provide a user interface for the movie ticket booking system.
public class MovieTicketBookingGUI extends JFrame {
private JComboBox<Movie> movieDropdown; // Dropdown for selecting movies
private JTextField ticketField; // Text field to enter number of tickets
private JTextArea bookingDetails; // Text area to display booking details
private ArrayList<Movie> movies = new ArrayList<>(); // List of available movies
private ArrayList<Booking> bookings = new ArrayList<>(); // List of bookings made
public MovieTicketBookingGUI() {
setTitle("š¬ Movie Ticket Booking System");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(6, 1)); // Layout manager for arranging components
// Initialize movie list
movies.add(new Movie("Avengers: Endgame", 50));
movies.add(new Movie("Inception", 40));
movies.add(new Movie("The Dark Knight", 30));
movies.add(new Movie("Interstellar", 25));
movies.add(new Movie("Titanic", 20));
// Add the movie names to the dropdown menu
JLabel selectMovieLabel = new JLabel("Select Movie:");
movieDropdown = new JComboBox<>();
for (Movie movie : movies) {
movieDropdown.addItem(movie);
}
// Add ticket field
JLabel ticketLabel = new JLabel("Enter Number of Tickets:");
ticketField = new JTextField();
// Book ticket button
JButton bookButton = new JButton("Book Ticket");
bookButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
bookTicket(); // Trigger booking process when the button is clicked
}
});
// Text area to display booking details
bookingDetails = new JTextArea();
bookingDetails.setEditable(false);
// Add components to the window
add(selectMovieLabel);
add(movieDropdown);
add(ticketLabel);
add(ticketField);
add(bookButton);
add(new JScrollPane(bookingDetails));
setVisible(true); // Show the window
}
private void bookTicket() {
// Get the selected movie and number of tickets
int selectedIndex = movieDropdown.getSelectedIndex();
String ticketText = ticketField.getText();
try {
int tickets = Integer.parseInt(ticketText);
if (tickets <= 0 || tickets > movies.get(selectedIndex).getAvailableSeats()) {
// Show error message if tickets are invalid
JOptionPane.showMessageDialog(this, "Invalid number of tickets!", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
// Book the tickets and update available seats
movies.get(selectedIndex).bookSeats(tickets);
// Add booking details to the list
bookings.add(new Booking(movies.get(selectedIndex).getName(), tickets));
// Update the movie dropdown to reflect remaining seats
movieDropdown.insertItemAt(movies.get(selectedIndex), selectedIndex);
movieDropdown.removeItemAt(selectedIndex + 1);
// Display booking details
bookingDetails.append("š„ Movie: " + movies.get(selectedIndex).getName() + "\nšļø Tickets: " + tickets + "\n\n");
// Show success message
JOptionPane.showMessageDialog(this, "ā
Booking Successful!", "Success", JOptionPane.INFORMATION_MESSAGE);
ticketField.setText(""); // Clear ticket field
} catch (NumberFormatException ex) {
// Handle invalid number input
JOptionPane.showMessageDialog(this, "Please enter a valid number!", "Error", JOptionPane.ERROR_MESSAGE);
}
}
public static void main(String[] args) {
new MovieTicketBookingGUI(); // Launch the GUI
}
}
Purpose:
- Sets up the GUI for the movie ticket booking system.
- It manages the user interactions like:
- Selecting a movie from a dropdown.
- Entering the number of tickets.
- Booking the tickets and displaying booking details.
Source Code:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
class Movie {
String name;
int availableSeats;
Movie(String name, int seats) {
this.name = name;
this.availableSeats = seats;
}
}
public class MovieTicketBookingGUI extends JFrame {
private JComboBox movieDropdown;
private JTextField ticketField;
private JTextArea bookingDetails;
private ArrayList movies = new ArrayList();
public MovieTicketBookingGUI() {
setTitle("Movie Ticket Booking System š¬");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(5, 1));
// Add movies
movies.add(new Movie("Avengers: Endgame", 50));
movies.add(new Movie("Inception", 40));
movies.add(new Movie("The Dark Knight", 30));
// Movie Selection Dropdown
JLabel selectMovieLabel = new JLabel("Select Movie:");
movieDropdown = new JComboBox();
for (Movie movie : movies) {
movieDropdown.addItem(movie.name + " (" + movie.availableSeats + " seats)");
}
// Ticket Input
JLabel ticketLabel = new JLabel("Enter Number of Tickets:");
ticketField = new JTextField();
// Book Button
JButton bookButton = new JButton("Book Ticket");
bookButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
bookTicket();
}
});
// Booking Details Area
bookingDetails = new JTextArea();
bookingDetails.setEditable(false);
// Add components to frame
add(selectMovieLabel);
add(movieDropdown);
add(ticketLabel);
add(ticketField);
add(bookButton);
add(new JScrollPane(bookingDetails));
setVisible(true);
}
private void bookTicket() {
int selectedIndex = movieDropdown.getSelectedIndex();
String ticketText = ticketField.getText();
try {
int tickets = Integer.parseInt(ticketText);
if (tickets movies.get(selectedIndex).availableSeats) {
JOptionPane.showMessageDialog(this, "Invalid number of tickets!", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
// Update seat availability
movies.get(selectedIndex).availableSeats -= tickets;
movieDropdown.insertItemAt(movies.get(selectedIndex).name + " (" + movies.get(selectedIndex).availableSeats + " seats)", selectedIndex);
movieDropdown.removeItemAt(selectedIndex + 1);
// Display booking confirmation
bookingDetails.append("š„ Movie: " + movies.get(selectedIndex).name + "\nšļø Tickets: " + tickets + "\n\n");
JOptionPane.showMessageDialog(this, "ā
Booking Successful!", "Success", JOptionPane.INFORMATION_MESSAGE);
ticketField.setText("");
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "Please enter a valid number!", "Error", JOptionPane.ERROR_MESSAGE);
}
}
public static void main(String[] args) {
new MovieTicketBookingGUI();
}
}
Output:
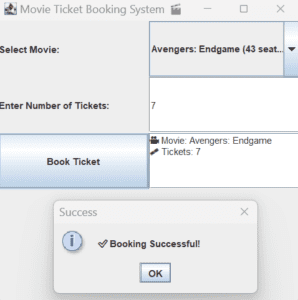
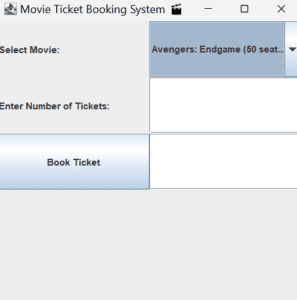