Hotel Management System using C++ With Source Code
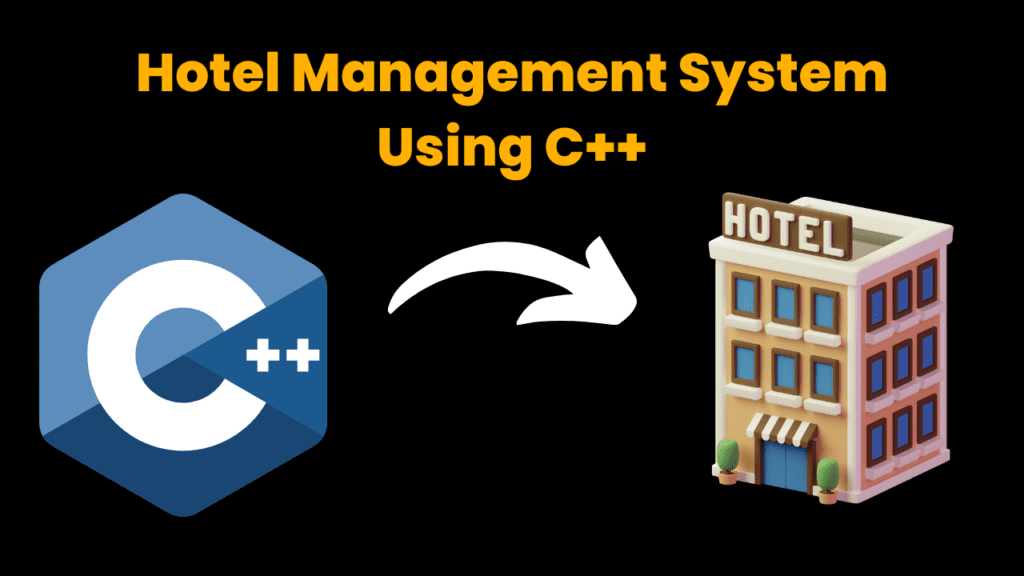
Introduction :
Managing a hotel effectively is key to ensuring great customer service and smooth business operations. The Hotel Management System in C++ is a console-based application designed to manage various aspects of hotel operations, including room booking, customer information maintenance, room allotment, customer checkout, and restaurant service management. This system uses the standard input and output streams in C++ to provide a user-friendly command-line interface for hotel management.
Project Overview
The system provides a user-friendly command-line interface where hotel staff can efficiently perform essential tasks like booking rooms, managing customer details, viewing allocated rooms, editing customer information, and handling restaurant orders. This project aims to provide a comprehensive solution for managing the daily operations of a hotel, ensuring smooth and efficient service delivery to customers.
The Hotel Management System in C++ enables hotel staff to easily perform all major tasks such as booking rooms, viewing customer information, listing allocated rooms, editing customer details, and ordering food from the restaurant.
The Hotel Management System offers the following key features:
Room Booking: The system allows staff to book rooms by entering customer details, such as name, address, phone number, and the number of days for the stay. Rooms are categorized into Deluxe, Executive, and Presidential, with different pricing options. This categorization helps efficiently allocate rooms according to the customer’s preferences and budget.
Customer Information Management: The system maintains a detailed customer database, allowing staff to quickly retrieve and display customer information. This feature is particularly useful for handling booking inquiries and managing customer data.
Room Allocation Overview: Staff can view a comprehensive overview of all allocated rooms, displayed in a tabular format with details like room number, guest name, address, room type, and contact number.
Editing Customer Details: The system provides an edit menu where staff can modify customer information such as name, address, phone number, and duration of stay. This ensures data consistency and accuracy by updating relevant records in the database.
Restaurant Service Management: Customers can order meals (breakfast, lunch, or dinner) from their rooms, and the system automatically adds the charges to the customer’s bill. This feature enhances convenience for customers and improves service delivery.
User-Friendly Interface: The console-based interface makes the system compatible with various platforms without requiring high-end graphical interfaces, making it a compact yet effective solution for hotel management.
This C++ project relies only on standard libraries; thus, no external modules or packages are dependent on successful compilation and execution. However, for compiling and running this program, the following components are necessary to be available and properlyconfigured in your environment:
1. C++ Compiler: The source code needs a generic C++ compiler, such as GCC, standing for GNU Compiler Collection, for compilation. GCC is very common and enjoys support
from most operating systems, which include Windows, Linux, and macOS.
2. Standard C++ Libraries: This program makes use of standard libraries such as, but not
limited to, iostream, fstream, stdio.h, string.h, cstring, and iomanip. These are usedforstandard input and output operations, file handling operations, string operations, andformatting outputs, respectively.
Required Modules or Packages:
Installation Guide :
Windows:
MinGW installation In your system’s environment variables, add the bin directory of MinGWin the variablenamed PATH.
Linux:
Fire up the terminal and type the following:
sudo apt-get update
sudo apt-get install build-essential
macOS Installation :
Run the following to install the Xcode Command Line Tools:
xcode-select --install
Install the g++ compiler and confirm that it is working, by running g++ –versioninyourterminal or command prompt. This should return the version of the compiler installed.
How to Run the Code :
Follow the following steps to setup and run the Hotel Management System on your local environment:
1.Download Source Code:
git clone https://github.com/yourusername/hotel-management-system.git
or clone the ZIP from the repository and extract it to the destination of your choice
2. Enter Project Directory:
cd hotel-management-system
3. Compile Source Code:
To compile the main.cpp file, you can use:
g++ main.cpp -o hotel_management
This command will compile the code into an executable called hotel_management. 4. Running Executable: For running the executable, use:
./hotel_management
The main menu pops out on your console to interact with the system.
5. Operating the Application:
- Select an option by typing the corresponding number from the main menu.
- Follow the on-screen instructions for room booking, customer information management, editing details, ordering food, and other functions.
Note: Ensure you have read and write permissions for the project directory, as the application reads from and writes to files to store records.
Explanation of Code :
The Hotel Management System is encapsulated in the class HOTEL. The class containsprivate and public members to control different aspects of the system.
class HOTEL {
private:
int room_no;
char name[30];
char address[50];
char phone[15];
long days;
long cost;
char rtype[30];
long pay;
void breakfast(int);
void lunch(int);
void dinner(int);
public:
void main_menu();
void add();
void display();
void rooms();
void edit();
int check(int);
void modify();
void delete_rec();
void restaurant();
void modify_name(int);
void modify_address(int);
void modify_phone(int);
void modify_days(int);
} h;
Private Members:
- room_no, name, address, phone, days, cost, rtype, pay: Variables to store customer information and booking details.
- breakfast(int), lunch(int), dinner(int): Functions to calculate and add meal costs to the customer’s bill.
Public Members:
- main_menu(): Displays the main menu and takes user input for further operations.
- add(): Prompts the user to book a room by inputting customer details and writes the data to a file.
- display(): Retrieves and displays information for a particular customer based on the room number.
- rooms(): Lists all allocated rooms along with customer details in a tabular format.
- edit(): Allows staff to modify customer details or check out a customer.
- check(int): Checks if a room is already booked or available.
- modify(): Calls specific functions to modify different details of a customer.
- delete_rec(): Manages customer checkout by deleting their records from the file.
- restaurant(): Facilitates ordering meals and updates the bill amount.
- modify_name(int), modify_address(int), modify_phone(int), modify_days(int): Functions to update specific customer information.
Key Functions:
add(): This function is for booking a new room.
void HOTEL::add() {
int r, flag;
ofstream fout("Record.DAT", ios::app | ios::binary);
cout << "\nENTER CUSTOMER DETAILS";
cout << "\nRoom Number: ";
cin >> r;
flag = check(r);
if (flag == 1)
cout << "\nSorry, Room is already booked.\n";
else {
room_no = r;
cout << "Name: ";
cin >> name;
cout << "Address: ";
cin >> address;
cout << "Phone Number: ";
cin >> phone;
cout << "Number of Days: ";
cin >> days;
// Assign room type and cost based on room number
if (room_no >= 1 && room_no <= 50) {
strcpy(rtype, "Deluxe");
cost = days * 10000;
} else if (room_no >= 51 && room_no <= 80) {
strcpy(rtype, "Executive");
cost = days * 12500;
} else if (room_no >= 81 && room_no <= 100) {
strcpy(rtype, "Presidential");
cost = days * 15000;
}
fout.write((char*)this, sizeof(HOTEL));
cout << "\nRoom has been booked.";
}
fout.close();
}
This function first checks if the room is available using the check() function. If so, it takes the details of the customer, assigns the type of the room and calculates the cost based on the room number, and finally writes the data to a binary file Record.DAT.
display(): Displays the details of a specific customer.
void HOTEL::display() {
ifstream fin("Record.DAT", ios::in | ios::binary);
int r, flag = 0;
cout << "\nEnter Room Number: ";
cin >> r;
while (fin.read((char*)this, sizeof(HOTEL))) {
if (room_no == r) {
cout << "\nCustomer Details";
cout << "\nRoom Number: " << room_no;
cout << "\nName: " << name;
cout << "\nAddress: " << address;
cout << "\nPhone Number: " << phone;
cout << "\nStaying for " << days << " days.";
cout << "\nRoom Type: " << rtype;
cout << "\nTotal cost of stay: " << cost;
flag = 1;
break;
}
}
if (flag == 0)
cout << "\nRoom is Vacant.";
fin.close();
}
The above function opens the Record.DAT file and then searches for the record matchingthe entered room number and displays all the information about the customer.
restaurant(): Allows customers to make a purchase of food items and adds the amount to their bill
void HOTEL::restaurant() {
int r, ch;
cout << "RESTAURANT MENU:";
cout << "\n1. Order Breakfast";
cout << "\n2. Order Lunch";
cout << "\n3. Order Dinner";
cout << "\nEnter your choice: ";
cin >> ch;
cout << "Enter Room Number: ";
cin >> r;
switch (ch) {
case 1: breakfast(r); break;
case 2: lunch(r); break;
case 3: dinner(r); break;
default: cout << "Invalid choice."; break;
}
}
This function calls, depending on the user’s choice, the corresponding meal function, breakfast(), lunch() or dinner(), that computes the price of the meal and updates the total bill
of the customer.
File Handling:
In this system, the binary files store and retrieve the customer records. The functions likeadd(), display(), modify(), and delete_rec() are used to read and write in the Record.DATfile for data persistence.
User Interaction:
It is the main_menu() function, which, interacting directly with the user, shows a variety of options and takes input from the user to enter different functionalities of the system. Each option transfers the user to different operations that are to be performed in a well-structured manner.
Get Discount on Top Educational Courses
Source Code :
Hotel.cpp
#include
#include
#include
#include
#include
#include
using namespace std;
class HOTEL
{
private:
int room_no;
char name[30];
char address[50];
char phone[15];
long days;
long cost;
char rtype[30];
long pay;
void breakfast(int); //to assign price of breakfast
void lunch(int); //to assign price of lunch
void dinner(int); //to assign price of dinner
public:
void main_menu(); //to dispay the main menu
void add(); //to book a room
void display(); //to display the specific customer information
void rooms(); //to display alloted rooms
void edit(); //to edit the customer by calling modify or delete
int check(int); //to check room status
void modify(); //to modify the customer information
void delete_rec(); //to check out the customer
void restaurant(); //to order food from restaurant
void modify_name(int); //to modify name of guest
void modify_address(int); //to modify address of guest
void modify_phone(int); //to modify phone number of guest
void modify_days(int); //to modify days of stay of guest
}h;
void HOTEL::main_menu()
{
int choice;
while(choice!=6)
{
system("clear");
cout<<"\n\t\t\t\t *************";
cout<<"\n\t\t\t\t **THE HOTEL**";
cout<<"\n\t\t\t\t *************";
cout<<"\n\t\t\t\t * MAIN MENU *";
cout<<"\n\t\t\t\t *************";
cout<<"\n\n\n\t\t\t\t1. Book A Room";
cout<<"\n\t\t\t\t2. Customer Information";
cout<<"\n\t\t\t\t3. Rooms Allotted";
cout<<"\n\t\t\t\t4. Edit Customer Details";
cout<<"\n\t\t\t\t5. Order Food from Restaurant";
cout<<"\n\t\t\t\t6. Exit";
cout<<"\n\n\t\t\t\tEnter Your Choice: ";
cin>>choice;
switch(choice)
{
case 1: add();
break;
case 2: display();
break;
case 3: rooms();
break;
case 4: edit();
break;
case 5: restaurant();
break;
case 6: return;
default:
{
cout<<"\n\n\t\t\tWrong choice.";
cout<<"\n\t\t\tPress any key to continue.";
getchar();
}
}
}
}
void HOTEL::add()
{
system("clear");
int r,flag;
ofstream fout("Record.DAT",ios::app|ios::binary);
cout<<"\n\t\t\t +-----------------------+";
cout<<"\n\t\t\t | Rooms | Room Type |";
cout<<"\n\t\t\t +-----------------------+";
cout<<"\n\t\t\t | 1-50 | Deluxe |";
cout<<"\n\t\t\t | 51-80 | Executive |";
cout<<"\n\t\t\t | 81-100 | Presidential |";
cout<<"\n\t\t\t +-----------------------+";
cout<<"\n\n ENTER CUSTOMER DETAILS";
cout<<"\n ----------------------";
cout<<"\n\n Room Number: ";
cin>>r;
flag=check(r);
if(flag==1)
cout<<"\n Sorry, Room is already booked.\n";
else
{
if(flag==2)
cout<<"\n Sorry, Room does not exist.\n";
else
{
room_no=r;
cout<<" Name: ";
cin>>name;
cout<<" Address: ";
cin>>address;
cout<<" Phone Number: ";
cin>>phone;
cout<<" Number of Days: ";
cin>>days;
if(room_no>=1&&room_no<=50)
{
strcpy(rtype,"Deluxe");
cost=days*10000;
}
else
{
if(room_no>=51&&room_no<=80)
{
strcpy(rtype,"Executive");
cost=days*12500;
}
else
{
if(room_no>=81&&room_no<=100)
{
strcpy(rtype,"Presidential");
cost=days*15000;
}
}
}
fout.write((char*)this, sizeof(HOTEL));
cout<<"\n Room has been booked.";
}
}
cout<<"\n Press any key to continue.";
getchar();
getchar();
fout.close();
}
void HOTEL::display()
{
system("clear");
ifstream fin("Record.DAT",ios::in|ios::binary);
int r,flag;
cout<<"\n Enter Room Number: ";
cin>>r;
while(fin.read((char*)this,sizeof(HOTEL)))
{
if(room_no==r)
{
system("clear");
cout<<"\n Customer Details";
cout<<"\n ----------------";
cout<<"\n\n Room Number: "<>choice;
system("clear");
switch(choice)
{
case 1: modify();
break;
case 2: delete_rec();
break;
default: cout<<"\n Wrong Choice.";
break;
}
cout<<"\n Press any key to continue.";
getchar();
getchar();
}
int HOTEL::check(int r)
{
int flag=0;
ifstream fin("Record.DAT",ios::in|ios::binary);
while(fin.read((char*)this,sizeof(HOTEL)))
{
if(room_no==r)
{
flag=1;
break;
}
else
{
if(r>100)
{
flag=2;
break;
}
}
}
fin.close();
return(flag);
}
void HOTEL::modify()
{
system("clear");
int ch,r;
cout<<"\n MODIFY MENU";
cout<<"\n -----------";
cout<<"\n\n\n 1. Modify Name";
cout<<"\n 2. Modify Address";
cout<<"\n 3. Modify Phone Number";
cout<<"\n 4. Modify Number of Days of Stay";
cout<<"\n Enter Your Choice: ";
cin>>ch;
system("clear");
cout<<"\n Enter Room Number: ";
cin>>r;
switch(ch)
{
case 1: modify_name(r);
break;
case 2: modify_address(r);
break;
case 3: modify_phone(r);
break;
case 4: modify_days(r);
break;
default: cout<<"\n Wrong Choice";
getchar();
getchar();
break;
}
}
void HOTEL::modify_name(int r)
{
long pos,flag=0;
fstream file("Record.DAT",ios::in|ios::out|ios::binary);
while(!file.eof())
{
pos=file.tellg();
file.read((char*)this,sizeof(HOTEL));
if(room_no==r)
{
cout<<"\n Enter New Name: ";
cin>>name;
file.seekg(pos);
file.write((char*)this,sizeof(HOTEL));
cout<<"\n Customer Name has been modified.";
flag=1;
break;
}
}
if(flag==0)
cout<<"\n Sorry, Room is vacant.";
getchar();
getchar();
file.close();
}
void HOTEL::modify_address(int r)
{
long pos,flag=0;
fstream file("Record.DAT",ios::in|ios::out|ios::binary);
while(!file.eof())
{
pos=file.tellg();
file.read((char*)this,sizeof(HOTEL));
if(room_no==r)
{
cout<<"\n Enter New Address: ";
cin>>address;
file.seekg(pos);
file.write((char*)this,sizeof(HOTEL));
cout<<"\n Customer Address has been modified.";
flag=1;
break;
}
}
if(flag==0)
cout<<"\n Sorry, Room is vacant.";
getchar();
getchar();
file.close();
}
void HOTEL::modify_phone(int r)
{
long pos,flag=0;
fstream file("Record.DAT",ios::in|ios::out|ios::binary);
while(!file.eof())
{
pos=file.tellg();
file.read((char*)this,sizeof(HOTEL));
if(room_no==r)
{
cout<<"\n Enter New Phone Number: ";
cin>>phone;
file.seekg(pos);
file.write((char*)this,sizeof(HOTEL));
cout<<"\n Customer Phone Number has been modified.";
flag=1;
break;
}
}
if(flag==0)
cout<<"\n Sorry, Room is vacant.";
getchar();
getchar();
file.close();
}
void HOTEL::modify_days(int r)
{
long pos,flag=0;
fstream file("Record.DAT",ios::in|ios::out|ios::binary);
while(!file.eof())
{
pos=file.tellg();
file.read((char*)this,sizeof(HOTEL));
if(room_no==r)
{
cout<<" Enter New Number of Days of Stay: ";
cin>>days;
if(room_no>=1&&room_no<=50)
{
strcpy(rtype,"Deluxe");
cost=days*10000;
}
else
{
if(room_no>=51&&room_no<=80)
{
strcpy(rtype,"Executive");
cost=days*12500;
}
else
{
if(room_no>=81&&room_no<=100)
{
strcpy(rtype,"Presidential");
cost=days*15000;
}
}
}
file.seekg(pos);
file.write((char*)this,sizeof(HOTEL));
cout<<"\n Customer information is modified.";
flag=1;
break;
}
}
if(flag==0)
cout<<"\n Sorry, Room is Vacant.";
getchar();
getchar();
file.close();
}
void HOTEL::delete_rec()
{
int r,flag=0;
char ch;
cout<<"\n Enter Room Number: ";
cin>>r;
ifstream fin("Record.DAT",ios::in|ios::binary);
ofstream fout("temp.DAT",ios::out|ios::binary);
while(fin.read((char*)this,sizeof(HOTEL)))
{
if(room_no==r)
{
cout<<"\n Name: "<>ch;
if(ch=='n')
fout.write((char*)this,sizeof(HOTEL));
else
cout<<"\n Customer Checked Out.";
flag=1;
}
else
fout.write((char*)this,sizeof(HOTEL));
}
fin.close();
fout.close();
if(flag==0)
cout<<"\n Sorry, Room is Vacant.";
else
{
remove("Record.dat");
rename("temp.dat","Record.dat");
}
getchar();
getchar();
}
void HOTEL::restaurant()
{
system("clear");
int r,ch,num;
cout<<"\n RESTAURANT MENU:";
cout<<"\n --------------- ";
cout<<"\n\n 1. Order Breakfast\n 2. Order Lunch\n 3. Order Dinner";
cout<<"\n\n Enter your choice: ";
cin>>ch;
system("clear");
cout<<" Enter Room Number: ";
cin>>r;
switch(ch)
{
case 1: breakfast(r);
break;
case 2: lunch(r);
break;
case 3: dinner(r);
break;
}
cout<<"\n\n Press any key to continue.";
getchar();
getchar();
return;
}
void HOTEL::breakfast(int r)
{
int num,flag=0;
long pos;
cout<<" Enter number of people: ";
cin>>num;
fstream file("Record.DAT",ios::in|ios::out|ios::binary);
while(!file.eof())
{
pos=file.tellg();
file.read((char*)this,sizeof(HOTEL));
if(room_no==r)
{
pay=500*num;
cost=cost+pay;
file.seekg(pos);
file.write((char*)this,sizeof(HOTEL));
cout<<"\n Amount added to the bill: Rs. "<>num;
fstream file("Record.DAT",ios::in|ios::out|ios::binary);
while(!file.eof())
{
pos=file.tellg();
file.read((char*)this,sizeof(HOTEL));
if(room_no==r)
{
pay=1000*num;
cost=cost+pay;
file.seekg(pos);
file.write((char*)this,sizeof(HOTEL));
cout<<"\n Amount added to the bill: Rs. "<>num;
fstream file("Record.DAT",ios::in|ios::out|ios::binary);
while(!file.eof())
{
pos=file.tellg();
file.read((char*)this,sizeof(HOTEL));
if(room_no==r)
{
pay=1200*num;
cost=cost+pay;
file.seekg(pos);
file.write((char*)this,sizeof(HOTEL));
cout<<"\n Amount added to the bill: Rs. "<
Output :
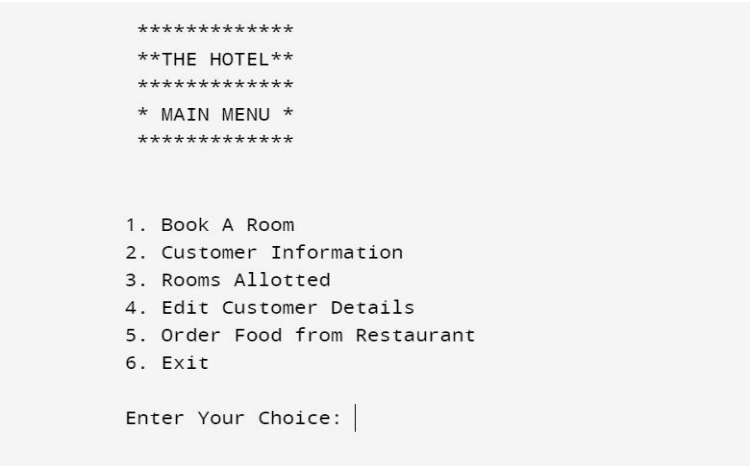
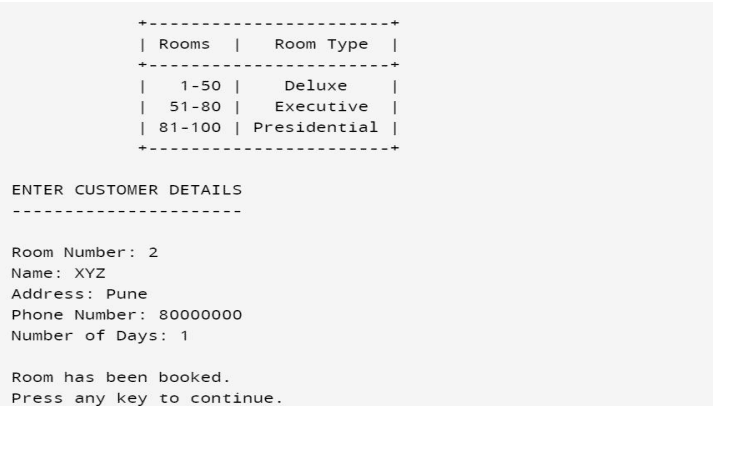
Find More Projects
Whack a Mole Game with HTML CSS And JavaScript Introduction Hello friends, you all are welcome to today’s beautiful and unique project. Today …
3D Car Driving Game Using Html CSS And JavaScript Introduction Hello friends, welcome to today’s new blog post. Today we have created …
Dice Rolling Game Using HTML CSS And JavaScript Introduction Hey coders, welcome to another new blog. In this article we’ll build a …
Crossey Road Game Clone Using HTML CSS And JavaScript Introduction This is our HTML code which sets up the basic structure of …
Memory Card Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
sudoku game using html CSS and JavaScript Introduction Hello friends, you all are welcome to today’s beautiful project. Today we have made …