Java Design Patterns Interview Questions
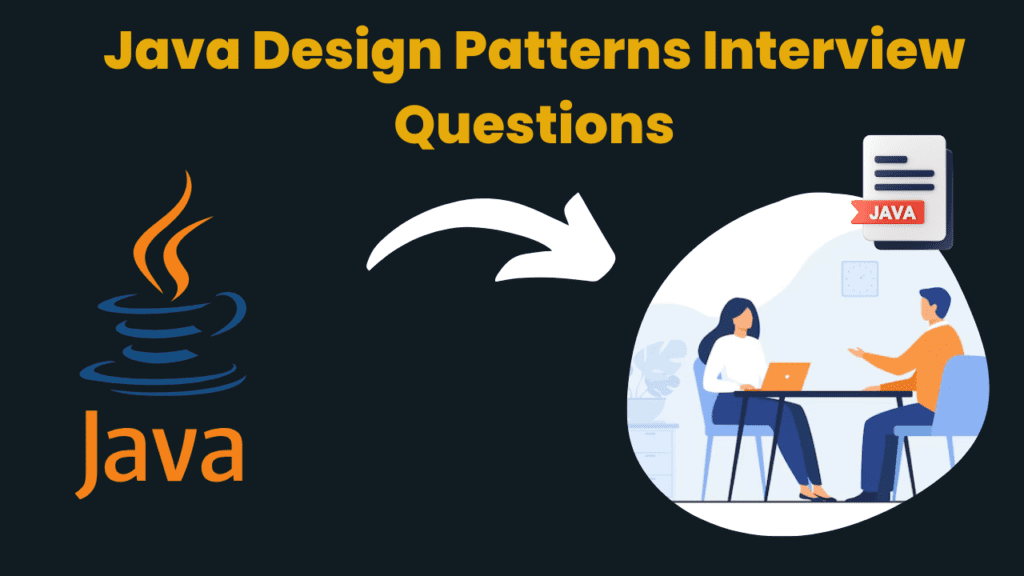
50 most frequently asked Java Design Patterns Interview Questions.
1. What are design patterns, and why are they important in software development?
Answer: Design patterns are reusable solutions to common software design problems. They are important because they help improve code maintainability, scalability, and reusability.
2. Explain the Singleton design pattern in Java and its use cases.
Answer: The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. Use cases include managing a single database connection or a configuration manager.
3. What is the Factory Method design pattern, and how does it provide an interface for creating objects in a superclass but allows subclasses to alter the type of objects created?
Answer: The Factory Method pattern defines an interface for creating objects, with subclasses implementing the creation method. It allows the creation of objects with varying types.
4. Explain the Builder design pattern in Java and its use for constructing complex objects step by step.
Answer: The Builder pattern separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
5. What is the Observer design pattern, and how is it used for establishing a one-to-many dependency between objects?
Answer: The Observer pattern defines a one-to-many relationship between objects, where one object (the subject) maintains a list of observers and notifies them of state changes.
6. Explain the Adapter design pattern in Java and its use for making incompatible interfaces work together.
Answer: The Adapter pattern allows the interface of an existing class to be used as another interface, making it compatible with clients that require the new interface.
7. What is the Decorator design pattern, and how does it allow behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class?
Answer: The Decorator pattern attaches additional responsibilities to objects dynamically. It is used for extending an object’s functionality without altering its structure.
8. Explain the Strategy design pattern in Java and its use for defining a family of algorithms, encapsulating each one, and making them interchangeable.
Answer: The Strategy pattern defines a set of algorithms, encapsulates them in separate classes, and allows the client to choose the algorithm to use at runtime.
9. What is the Command design pattern, and how is it used for encapsulating a request as an object, thereby allowing parameterization of clients with queues, requests, and operations?
Answer: The Command pattern encapsulates a request as an object, allowing the parameterization of clients with queues, requests, and operations. It supports undo and redo operations.
10. Explain the Template Method design pattern in Java and its use for defining the skeleton of an algorithm in the superclass but letting subclasses override specific steps of the algorithm.
Answer: The Template Method pattern defines the structure of an algorithm in a superclass, with specific steps delegated to subclasses. It enforces a common algorithm structure.
11. What is the Chain of Responsibility design pattern, and how does it pass a request along a chain of handlers, with each handler deciding either to process the request or to pass it to the next handler in the chain?
Answer: The Chain of Responsibility pattern allows you to pass a request along a chain of handlers, where each handler decides whether to process the request or pass it to the next handler.
12. Explain the Composite design pattern and how it composes objects into tree structures to represent part-whole hierarchies.
Answer: The Composite pattern composes objects into tree structures to represent part-whole hierarchies, allowing clients to treat individual objects and compositions of objects uniformly.
13. What is the State design pattern, and how does it allow an object to change its behavior when its internal state changes?
Answer: The State pattern allows an object to change its behavior when its internal state changes. It involves encapsulating state-specific behavior in separate state classes.
14. Explain the Proxy design pattern in Java and its use for providing a surrogate or placeholder for another object to control access to it.
Answer: The Proxy pattern provides a surrogate or placeholder for an object to control access to it. It is used for adding an extra level of control over object access.
15. What is the Flyweight design pattern, and how does it minimize memory or computational overhead by sharing as much as possible with related objects?
Answer: The Flyweight pattern minimizes memory or computational overhead by sharing as much as possible with related objects. It is used for managing a large number of similar objects efficiently.
16. Explain the Visitor design pattern in Java and its use for representing an operation to be performed on elements of an object structure.
Answer: The Visitor pattern represents an operation to be performed on elements of an object structure. It allows you to add new operations without modifying the objects being visited.
17. What is the Memento design pattern, and how is it used for capturing and externalizing an object’s internal state so that the object can be restored to this state later?
Answer: The Memento pattern captures an object’s internal state and allows it to be restored later. It is used for implementing undo mechanisms or saving and restoring states.
18. Explain the Mediator design pattern and its use for defining an object that encapsulates how a set of objects interact with each other, promoting loose coupling between them.
Answer: The Mediator pattern defines an object that encapsulates how a set of objects interact, reducing direct dependencies between them and promoting loose coupling.
19. What is the Interpreter design pattern, and how is it used for defining a language for parsing expressions and interpreting them?
Answer: The Interpreter pattern defines a language for parsing expressions and interpreting them. It is used for implementing custom languages or rule-based systems.
20. Explain the Prototype design pattern in Java and its use for creating new objects by copying an existing object, known as the prototype.
Answer: The Prototype pattern creates new objects by copying an existing object (the prototype). It is used to avoid the cost of initializing objects from scratch.
21. What is the Dependency Injection design pattern, and how does it promote the use of interfaces and decouple the creation and use of objects in a system?
Answer: The Dependency Injection pattern promotes the use of interfaces and decouples object creation and use by injecting dependencies into classes rather than having them create their own dependencies.
22. Explain the Abstract Factory design pattern in Java and its use for providing an interface to create families of related or dependent objects without specifying their concrete classes.
Answer: The Abstract Factory pattern provides an interface for creating families of related objects without specifying their concrete classes. It ensures the compatibility of created objects.
23. What is the Bridge design pattern, and how does it separate an object’s abstraction from its implementation, allowing both to vary independently?
Answer: The Bridge pattern separates an object’s abstraction from its implementation, enabling them to change independently. It promotes loose coupling between abstractions and implementations.
24. Explain the Factory Method design pattern in Java and its use for creating objects based on a defined interface while allowing subclasses to provide the actual implementations.
Answer: The Factory Method pattern defines an interface for creating objects but lets subclasses provide the actual implementations. It is useful for creating families of related objects.
25. What is the Composite design pattern in Java, and how does it allow clients to treat individual objects and compositions of objects uniformly in a tree structure?
Answer: The Composite pattern composes objects into tree structures, enabling clients to treat individual objects and compositions of objects uniformly.
26. Explain the Strategy design pattern and its use for defining a family of algorithms, encapsulating them, and making them interchangeable.
Answer: The Strategy pattern defines a family of algorithms, encapsulates them, and allows clients to choose and switch between algorithms at runtime.
27. What is the Chain of Responsibility design pattern, and how does it pass a request along a chain of handlers, each deciding to process the request or pass it to the next handler?
Answer: The Chain of Responsibility pattern passes a request along a chain of handlers, where each handler decides whether to process the request or pass it to the next handler in the chain.
28. Explain the State design pattern in Java and how it allows an object to change its behavior when its internal state changes.
Answer: The State pattern allows an object to change its behavior when its internal state changes. It involves encapsulating state-specific behavior in separate state classes.
29. What is the Visitor design pattern, and how is it used for representing an operation to be performed on elements of an object structure?
Answer: The Visitor pattern represents an operation to be performed on elements of an object structure, allowing you to add new operations without modifying the objects being visited.
30. Explain the Observer design pattern and its use for establishing a one-to-many dependency between objects, where one object (the subject) notifies its observers of state changes.
Answer: The Observer pattern establishes a one-to-many dependency between objects, where one object (the subject) notifies its observers of state changes.
31. What is the Command design pattern, and how does it encapsulate a request as an object, allowing for parameterization of clients with commands and operations?
Answer: The Command pattern encapsulates a request as an object, enabling parameterization of clients with commands and operations. It supports undo and redo operations.
32. Explain the Adapter design pattern in Java and its use for making incompatible interfaces work together.
Answer: The Adapter pattern allows you to make incompatible interfaces work together by providing a wrapper that converts one interface into another.
33. What is the Decorator design pattern, and how is it used to add behavior to objects dynamically without altering their structure?
Answer: The Decorator pattern is used to add behavior to objects dynamically without changing their structure. It involves creating a series of decorator classes that wrap the original class.
34. Explain the Proxy design pattern in Java and its use for providing a surrogate or placeholder for another object to control access to it.
Answer: The Proxy pattern provides a surrogate or placeholder for an object, controlling access to it. It can be used for various purposes, such as lazy initialization or access control.
35. What is the Flyweight design pattern, and how does it minimize memory or computational overhead by sharing as much as possible with related objects?
Answer: The Flyweight pattern minimizes memory or computational overhead by sharing as much as possible with related objects. It is used for efficient resource management.
36. Explain the Template Method design pattern in Java and how it defines the skeleton of an algorithm in the superclass but allows subclasses to override specific steps.
Answer: The Template Method pattern defines the structure of an algorithm in the superclass, with specific steps delegated to subclasses. It enforces a common algorithm structure.
37. What is the Memento design pattern, and how does it capture and externalize an object’s internal state to allow the object to be restored to that state later?
Answer: The Memento pattern captures an object’s internal state and allows the object to be restored to that state later. It is commonly used for undo and redo functionality.
38. Explain the Mediator design pattern and its use for defining an object that encapsulates how a set of objects interact with each other, promoting loose coupling between them.
Answer: The Mediator pattern defines an object that encapsulates how a set of objects interact, promoting loose coupling and reducing direct dependencies between the objects.
39. What is the Interpreter design pattern, and how is it used for defining a language for parsing expressions and interpreting them?
Answer: The Interpreter pattern is used to define a language for parsing expressions and interpreting them. It is often used for building custom languages or rule-based systems.
40. Explain the Prototype design pattern in Java and how it allows new objects to be created by copying an existing object, known as the prototype.
Answer: The Prototype pattern allows new objects to be created by copying an existing object (the prototype). It is useful for scenarios where object creation is costly.
41. What is the Dependency Injection design pattern, and how does it promote the use of interfaces and decouple object creation and use in a system?
Answer: The Dependency Injection pattern promotes the use of interfaces and decouples object creation and use by injecting dependencies into classes, making them more modular and testable.
42. Explain the Abstract Factory design pattern in Java and its use for providing an interface to create families of related or dependent objects without specifying their concrete classes.
Answer: The Abstract Factory pattern provides an interface to create families of related objects without specifying their concrete classes. It ensures the compatibility of created objects.
43. What is the Bridge design pattern, and how does it separate an object’s abstraction from its implementation, allowing both to vary independently?
Answer: The Bridge pattern separates an object’s abstraction from its implementation, enabling both to vary independently. It promotes loose coupling between abstractions and implementations.
44. Explain the Factory Method design pattern and its use for creating objects based on a defined interface while allowing subclasses to provide the actual implementations.
Answer: The Factory Method pattern defines an interface for creating objects but lets subclasses provide the actual implementations. It is useful for creating families of related objects.
45. What is the Composite design pattern in Java, and how does it allow clients to treat individual objects and compositions of objects uniformly in a tree structure?
Answer: The Composite pattern composes objects into tree structures, enabling clients to treat individual objects and compositions of objects uniformly.
46. Explain the Strategy design pattern and its use for defining a family of algorithms, encapsulating them, and making them interchangeable.
Answer: The Strategy pattern defines a family of algorithms, encapsulates them, and allows clients to choose and switch between algorithms at runtime.
47. What is the Chain of Responsibility design pattern, and how does it pass a request along a chain of handlers, each deciding to process the request or pass it to the next handler?
Answer: The Chain of Responsibility pattern passes a request along a chain of handlers, where each handler decides whether to process the request or pass it to the next handler in the chain.
48. Explain the State design pattern in Java and how it allows an object to change its behavior when its internal state changes.
Answer: The State pattern allows an object to change its behavior when its internal state changes. It involves encapsulating state-specific behavior in separate state classes.
49. What is the Visitor design pattern, and how is it used for representing an operation to be performed on elements of an object structure?
Answer: The Visitor pattern represents an operation to be performed on elements of an object structure, allowing you to add new operations without modifying the objects being visited.
50. Explain the Observer design pattern and its use for establishing a one-to-many dependency between objects, where one object (the subject) notifies its observers of state changes.
Answer: The Observer pattern establishes a one-to-many dependency between objects, where one object (the subject) notifies its observers of state changes.
These questions cover a wide range of design patterns in Java, their use cases, and the principles behind each pattern.