Advanced Java Interview Questions
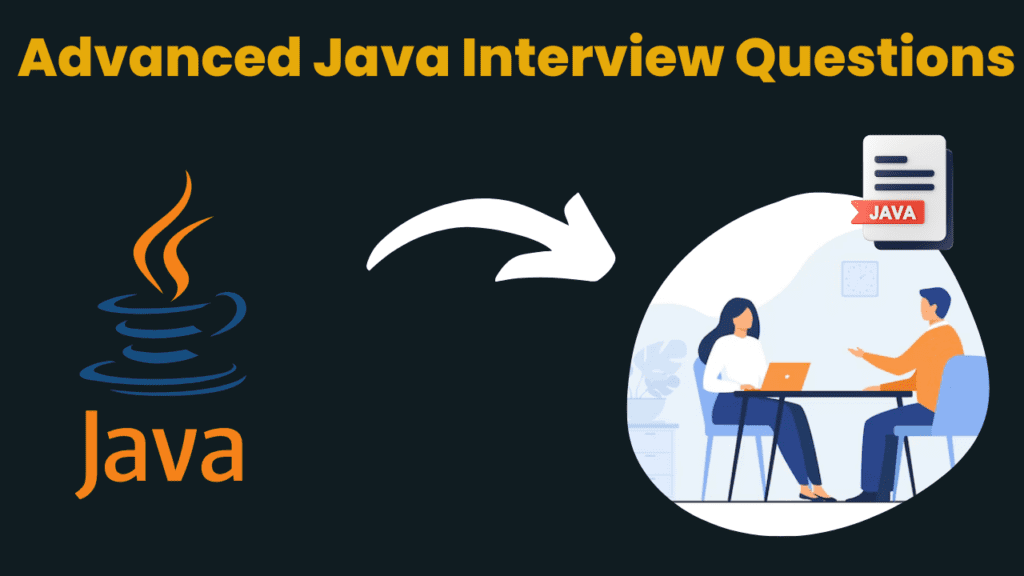
50 most frequently asked Advanced Java Interview Questions.
Get Discount on Top Educational Courses
1. What is the difference between Java and JavaScript?
Answer: Java is a statically-typed, compiled language for server-side and desktop applications, while JavaScript is a dynamically-typed, interpreted language mainly used for web development.
2. Explain the concept of Generics in Java and how they are used to make classes and methods type-safe.
Answer: Generics allow you to write classes and methods that can work with types yet to be specified, making them type-safe by providing compile-time checks.
3. What is Java Serialization, and why is it used?
Answer: Java Serialization is the process of converting objects into a byte stream, mainly for storing or transmitting objects. It is used for saving and restoring object states.
4. Explain the Java Virtual Machine (JVM) and its role in executing Java code.
Answer: The JVM is a crucial component in Java that interprets and executes Java bytecode. It provides platform independence by running Java code on any compatible system.
5. What is the purpose of the ‘transient’ keyword in Java, and how does it affect object serialization?
Answer: The ‘transient’ keyword is used to mark fields that should not be included in the object’s serialized form, making them non-persistent.
6. Explain the ‘volatile’ keyword in Java and its role in ensuring visibility and atomicity of variables in multi-threaded applications.
Answer: ‘volatile’ is used to declare variables as thread-safe, ensuring that their values are always up-to-date when accessed by multiple threads.
7. What are lambda expressions in Java, and how are they used to simplify code when working with functional interfaces?
Answer: Lambda expressions provide a concise way to define anonymous methods. They are used to implement functional interfaces, simplifying code for single-method interfaces.
8. Explain the ‘enum’ type in Java and its use for defining a fixed set of constants or enumeration values.
Answer: An ‘enum’ in Java is a special data type that represents a fixed set of constants. It is often used for defining a set of related values.
9. What is the purpose of the ‘finalize()’ method in Java, and when is it called during object lifecycle?
Answer: The ‘finalize()’ method is used for resource cleanup before an object is garbage-collected. It is called by the JVM before reclaiming the object’s memory.
10. Explain the concept of reflection in Java and its uses in inspecting and manipulating classes, methods, and fields at runtime.
Answer: Reflection allows you to examine and manipulate classes, methods, and fields at runtime. It is commonly used for tasks like dynamic loading and inspection.
11. What is the ‘ClassLoader’ in Java, and how does it load classes into memory dynamically?
Answer: The ‘ClassLoader’ is responsible for loading classes into memory dynamically at runtime. It follows a hierarchical structure and allows for dynamic class loading.
12. Explain the ‘assert’ statement in Java and its role in enforcing assumptions and program correctness during debugging.
Answer: The ‘assert’ statement is used to enforce assumptions and program correctness during debugging. It throws an exception if an assertion is false.
13. What is reflection in Java, and how is it used to obtain class information, access methods, and modify fields at runtime?
Answer: Reflection allows you to inspect and modify class information, access methods, and manipulate fields at runtime, making it useful for frameworks and tools.
14. What are annotations in Java, and how are they used for adding metadata to classes, methods, and fields?
Answer: Annotations are used to add metadata to classes, methods, fields, and other program elements. They provide information that can be processed at compile-time or runtime.
15. Explain the ‘java.util.concurrent’ package in Java and its role in supporting multithreaded and concurrent programming.
Answer: The ‘java.util.concurrent’ package provides classes and interfaces for concurrent programming, including thread management, synchronization, and task scheduling.
16. What is the purpose of the ‘Executor’ framework in Java, and how does it simplify the management of concurrent tasks and threads?
Answer: The ‘Executor’ framework simplifies the management of concurrent tasks and threads by providing a high-level API for thread management, scheduling, and execution.
17. Explain the ‘Fork-Join Framework’ in Java and its use for parallelizing tasks by dividing them into smaller subtasks and merging results.
Answer: The Fork-Join Framework is used for parallelizing tasks by breaking them into smaller subtasks and merging results, making it suitable for recursive divide-and-conquer algorithms.
18. What are Java Beans, and how are they used for creating reusable, customizable software components?
Answer: Java Beans are reusable software components that follow conventions for customization and property access. They are used for building extensible and configurable applications.
19. Explain the ‘SecurityManager’ class in Java and its role in controlling access to resources and enforcing security policies.
Answer: The ‘SecurityManager’ class is used to control access to resources and enforce security policies. It provides a security sandbox for Java applications.
20. What is the purpose of the ‘Classpath’ in Java, and how is it used to locate classes and resources during program execution?
Answer: The ‘Classpath’ is a system variable that specifies the location of classes and resources. It is used by the JVM to locate and load classes during program execution.
21. Explain the concept of inner classes in Java and their use in creating nested classes with various access levels.
Answer: Inner classes are classes defined within other classes. They are used for encapsulation and organizing code, and they can have different access levels.
22. What is the ‘strictfp’ modifier in Java, and how does it ensure consistent floating-point arithmetic across different platforms?
Answer: The ‘strictfp’ modifier is used to ensure consistent floating-point arithmetic by restricting floating-point calculations to follow the IEEE 754 standard.
23. Explain the ‘native’ keyword in Java and its use for invoking platform-specific native code through the Java Native Interface (JNI).
Answer: The ‘native’ keyword is used to declare methods that are implemented in platform-specific native code through the Java Native Interface (JNI).
24. What are ‘WeakReferences’ in Java, and how are they used for creating references that do not prevent objects from being garbage collected?
Answer: ‘WeakReferences’ are references that do not prevent objects from being garbage collected. They are used for creating soft associations with objects.
25. Explain the ‘java.util.concurrent’ package in Java and its role in supporting multithreaded and concurrent programming.
Answer: The ‘java.util.concurrent’ package provides classes and interfaces for concurrent programming, including thread management, synchronization, and task scheduling.
26. What is the ‘Executor’ framework in Java, and how does it simplify the management of concurrent tasks and threads?
Answer: The ‘Executor’ framework simplifies the management of concurrent tasks and threads by providing a high-level API for thread management, scheduling, and execution.
27. Explain the ‘Fork-Join Framework’ in Java and its use for parallelizing tasks by dividing them into smaller subtasks and merging results.
Answer: The Fork-Join Framework is used for parallelizing tasks by breaking them into smaller subtasks and merging results, making it suitable for recursive divide-and-conquer algorithms.
28. What are Java Beans, and how are they used for creating reusable, customizable software components?
Answer: Java Beans are reusable software components that follow conventions for customization and property access. They are used for building extensible and configurable applications.
29. Explain the ‘SecurityManager’ class in Java and its role in controlling access to resources and enforcing security policies.
Answer: The ‘SecurityManager’ class is used to control access to resources and enforce security policies. It provides a security sandbox for Java applications.
30. What is the purpose of the ‘Classpath’ in Java, and how is it used to locate classes and resources during program execution?
Answer: The ‘Classpath’ is a system variable that specifies the location of classes and resources. It is used by the JVM to locate and load classes during program execution.
31. Explain the concept of inner classes in Java and their use in creating nested classes with various access levels.
Answer: Inner classes are classes defined within other classes. They are used for encapsulation and organizing code, and they can have different access levels.
32. What is the ‘strictfp’ modifier in Java, and how does it ensure consistent floating-point arithmetic across different platforms?
Answer: The ‘strictfp’ modifier is used to ensure consistent floating-point arithmetic by restricting floating-point calculations to follow the IEEE 754 standard.
33. Explain the ‘native’ keyword in Java and its use for invoking platform-specific native code through the Java Native Interface (JNI).
Answer: The ‘native’ keyword is used to declare methods that are implemented in platform-specific native code through the Java Native Interface (JNI).
34. What are ‘WeakReferences’ in Java, and how are they used for creating references that do not prevent objects from being garbage collected?
Answer: ‘WeakReferences’ are references that do not prevent objects from being garbage collected. They are used for creating soft associations with objects.
35. What is the ‘AutoCloseable’ interface in Java, and how does it relate to the ‘try-with-resources’ statement for resource management?
Answer: The ‘AutoCloseable’ interface is used for resource management and is the basis for the ‘try-with-resources’ statement, which ensures proper resource cleanup.
36. Explain the ‘Class’ class in Java and its role in representing class metadata and allowing dynamic class loading.
Answer: The ‘Class’ class represents class metadata and provides methods for examining class information and dynamically loading classes.
37. What is the ‘PermGen’ space in Java, and how does it differ from the ‘Metaspace’ introduced in Java 8?
Answer: ‘PermGen’ (Permanent Generation) was a memory space for class metadata in Java prior to Java 8. In Java 8 and later, it was replaced by ‘Metaspace.’
38. Explain the ‘java.util.Optional’ class in Java and its use for representing an optional value that may be present or absent.
Answer: ‘java.util.Optional’ is a class that represents an optional value that may or may not be present, preventing null references and simplifying error handling.
39. What is the ‘ProcessBuilder’ class in Java, and how is it used for launching external processes from within a Java application?
Answer: The ‘ProcessBuilder’ class is used for launching external processes from within a Java application, providing control over process creation and execution.
40. Explain the ‘assert’ statement in Java and its role in enforcing program correctness during debugging and testing.
Answer: The ‘assert’ statement is used to enforce program correctness during debugging and testing by throwing an exception if an assertion is false.
41. What is the ‘MethodHandle’ class in Java, and how does it provide a more flexible alternative to Java reflection for invoking methods at runtime?
Answer: The ‘MethodHandle’ class is a more flexible alternative to Java reflection, allowing you to invoke methods at runtime with better performance and type safety.
42. Explain the ‘ResourceBundle’ class in Java and its use for internationalization and localization of applications.
Answer: The ‘ResourceBundle’ class is used for internationalization and localization of applications, providing access to locale-specific resources like text and images.
43. What is the ‘ClassLoader’ in Java, and how does it load classes into memory dynamically?
Answer: The ‘ClassLoader’ is responsible for loading classes into memory dynamically at runtime. It follows a hierarchical structure and allows for dynamic class loading.
44. Explain the ‘Unsafe’ class in Java and its role in performing low-level, unsafe operations not typically available to Java developers.
Answer: The ‘Unsafe’ class provides access to low-level, unsafe operations not typically available to Java developers. It is often used in core libraries and JVM implementations.
45. What is the Java Naming and Directory Interface (JNDI), and how is it used for accessing directory services in a networked environment?
Answer: JNDI is used to access directory services in a networked environment, providing a standard interface for connecting to and managing directories.
46. Explain the ‘MethodHandles.Lookup’ class in Java and its use for obtaining method handles with different access permissions.
Answer: ‘MethodHandles.Lookup’ is used to obtain method handles with different access permissions, allowing access to methods and fields that would otherwise be inaccessible.
47. What are ‘try-with-resources’ statements in Java, and how do they simplify resource management and ensure proper resource cleanup?
Answer: ‘try-with-resources’ statements simplify resource management by automatically closing resources like files, streams, and sockets, ensuring proper cleanup.
48. What is the purpose of the ‘Dynamic Proxy’ in Java, and how is it used to create dynamic implementations of interfaces at runtime?
Answer: Dynamic Proxies are used to create dynamic implementations of interfaces at runtime. They are often used in frameworks and AOP for cross-cutting concerns.
49. Explain the ‘MemoryModel’ in Java and its role in defining the memory consistency guarantees provided by the JVM.
Answer: The ‘MemoryModel’ defines the memory consistency guarantees provided by the JVM, ensuring predictable behavior in multithreaded applications.
50. What is the ‘java.util.ServiceLoader’ class in Java, and how is it used for loading service providers at runtime through the Service Provider Interface (SPI)?
Answer: The ‘java.util.ServiceLoader’ class is used for loading service providers at runtime through the Service Provider Interface (SPI), providing a mechanism for plugin-like functionality.
Get Discount on Top Educational Courses
These questions cover various advanced Java topics, including Java memory management, concurrency, reflection, and additional features introduced in the language.