Java File Handling Interview Questions
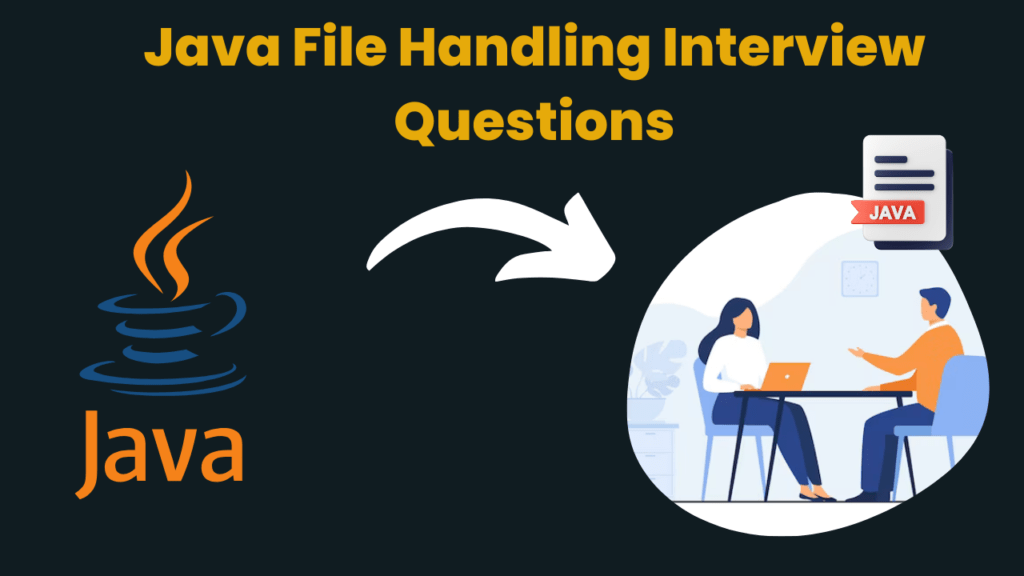
50 most frequently asked Java File Handling Interview Questions.
Get Discount on Top Educational Courses
1. What is file handling in Java, and why is it important in programming?
Answer: File handling in Java involves reading from and writing to files. It is essential for tasks like data storage, input/output, and configuration management.
2. Explain the difference between text files and binary files.
Answer: Text files store data as plain text, while binary files store data in a non-human-readable format, often for efficiency or to preserve data structure.
3. How do you open and read the contents of a text file in Java?
Answer: You can use classes like File
, FileReader
, and BufferedReader
to open and read text files in Java.
4. What is the purpose of the ‘File’ class in Java, and how is it used for file handling?
Answer: The ‘File’ class is used to represent file and directory paths and provides methods for file manipulation and information retrieval.
5. Explain how to write data to a text file in Java.
Answer: You can use classes like File
, FileWriter
, and BufferedWriter
to open a file for writing and then use the write
method to add data.
6. What is the ‘try-with-resources’ statement in Java, and how does it simplify file handling and resource cleanup?
Answer: The ‘try-with-resources’ statement simplifies file handling by automatically closing resources like files, ensuring proper cleanup and exception handling.
7. How do you handle file exceptions in Java, such as ‘FileNotFoundException’ or ‘IOException’?
Answer: File exceptions can be handled using try-catch blocks, where you catch specific exception types and provide appropriate error-handling code.
8. What is the ‘FileInputStream’ class in Java, and how is it used to read binary files?
Answer: ‘FileInputStream’ is used to read binary data from files. It reads bytes from a file into a byte array.
9. Explain the ‘FileOutputStream’ class in Java and its role in writing data to binary files.
Answer: ‘FileOutputStream’ is used to write binary data to files. It writes bytes from a byte array to a file.
10. What is the ‘RandomAccessFile’ class in Java, and how is it used for both reading and writing to a file at a specific position?
Answer: ‘RandomAccessFile’ allows you to read and write data at a specific position within a file, offering both reading and writing capabilities.
11. How do you check if a file exists in Java before attempting to read or write it?
Answer: You can use the ‘File’ class’s ‘exists()’ method to check if a file exists.
12. Explain the ‘BufferedReader’ class in Java and its role in efficient text file reading.
Answer: ‘BufferedReader’ is used for efficient text file reading by buffering data, reducing the number of read operations and enhancing performance.
13. What is the ‘BufferedWriter’ class in Java, and how is it used for efficient text file writing?
Answer: ‘BufferedWriter’ is used for efficient text file writing by buffering data, reducing the number of write operations and enhancing performance.
14. How do you delete a file in Java using the ‘File’ class?
Answer: You can use the ‘delete()’ method of the ‘File’ class to delete a file.
15. What is the ‘FileWriter’ class in Java, and how is it used for text file writing?
Answer: ‘FileWriter’ is used to write character data to text files in Java. It allows the easy creation and writing of text files.
16. Explain the ‘File.separator’ and ‘File.pathSeparator’ in Java and their role in working with file paths.
Answer: ‘File.separator’ is the platform-specific file separator character, while ‘File.pathSeparator’ is the platform-specific path separator for multiple file paths.
17. What is the ‘Scanner’ class in Java, and how is it used for reading data from files and other input sources?
Answer: The ‘Scanner’ class is used to read data from various sources, including files, by tokenizing and parsing input.
18. How can you create a new directory in Java using the ‘File’ class?
Answer: You can use the ‘mkdir()’ method to create a new directory using the ‘File’ class.
19. Explain the ‘FileReader’ class in Java and its role in reading text files.
Answer: ‘FileReader’ is used to read character data from text files in Java. It provides character-based input for text files.
20. What is the ‘FileWriter’ class in Java, and how is it used for writing text files?
Answer: ‘FileWriter’ is used to write character data to text files. It provides character-based output for text files.
21. How can you check if a file is a directory in Java using the ‘File’ class?
Answer: You can use the ‘isDirectory()’ method of the ‘File’ class to check if a file represents a directory.
22. What is the ‘Files’ class in Java, and how does it simplify file operations, such as copying and moving files?
Answer: The ‘Files’ class provides methods for common file operations like copying, moving, deleting, and more, making file handling tasks more convenient.
23. Explain the ‘Path’ class in Java and its role in representing file and directory paths.
Answer: The ‘Path’ class represents file and directory paths in a platform-independent manner. It provides methods for working with paths and performing various operations.
24. How can you list the files and directories within a directory using Java?
Answer: You can use the ‘listFiles()’ method of the ‘File’ class to obtain an array of files and directories within a directory.
25. What is the ‘File.createTempFile()’ method in Java, and how is it used for creating temporary files?
Answer: The ‘File.createTempFile()’ method is used to create temporary files with a specified prefix and suffix.
26. Explain the concept of file permissions in Java and how to set them using the ‘File’ class.
Answer: File permissions control who can read, write, and execute a file. You can use the ‘setReadable()’, ‘setWritable()’, and ‘setExecutable()’ methods of the ‘File’ class to change permissions.
27. What is the ‘File.renameTo()’ method in Java, and how is it used for renaming files and directories?
Answer: The ‘File.renameTo()’ method is used to rename files and directories. It allows you to change the name or move them to a different location.
28. Explain the ‘FileFilter’ interface in Java and how it is used to filter files during directory traversal.
Answer: The ‘FileFilter’ interface is used to filter files and directories during directory traversal, allowing you to select specific files based on criteria.
29. How do you create a new file in Java using the ‘File’ class, and what happens if the file already exists?
Answer: You can use the ‘createNewFile()’ method of the ‘File’ class to create a new file. If the file already exists, the method returns ‘false.’
30. Explain the ‘FileWriter’ class in Java, its constructors, and how to append data to an existing file.
Answer: ‘FileWriter’ can be used with constructors that allow appending to an existing file by specifying ‘true’ as the second argument.
31. What is the purpose of the ‘FileWriter’ class in Java, and how does it handle character encoding when writing to text files?
Answer: ‘FileWriter’ is used to write character data to text files. It uses the default character encoding of the platform unless specified otherwise.
32. Explain the ‘BufferedOutputStream’ class in Java and how it improves the performance of writing binary files.
Answer: ‘BufferedOutputStream’ is used to improve the performance of writing binary files by buffering data, reducing the number of write operations, and enhancing speed.
33. What is the ‘BufferedInputStream’ class in Java, and how does it enhance the performance of reading binary files?
Answer: ‘BufferedInputStream’ is used to enhance the performance of reading binary files by buffering data, reducing the number of read operations, and improving speed.
34. Explain the ‘DataInputStream’ class in Java and its role in reading primitive data types from binary files.
Answer: ‘DataInputStream’ is used to read primitive data types from binary files. It provides methods for reading specific data types like int, float, and double.
35. What is the ‘DataOutputStream’ class in Java, and how is it used for writing primitive data types to binary files?
Answer: ‘DataOutputStream’ is used to write primitive data types to binary files. It provides methods for writing specific data types like int, float, and double.
36. How do you handle end-of-file conditions when reading data from a file in Java?
Answer: You can check for end-of-file conditions by verifying the return value of file read methods (e.g., -1 for ‘read()’ method).
37. Explain the ‘PrintWriter’ class in Java and its use for writing formatted text to text files.
Answer: ‘PrintWriter’ is used to write formatted text to text files in a human-readable format, making it suitable for log files and configuration files.
38. What is the ‘FileChannel’ class in Java, and how does it provide advanced file operations like memory mapping and locking?
Answer: ‘FileChannel’ provides advanced file operations such as memory mapping and file locking, offering better control over file access and modification.
39. How do you read and write binary data to a file in Java using byte arrays?
Answer: You can use ‘FileInputStream’ and ‘FileOutputStream’ along with byte arrays to read and write binary data efficiently.
40. What is the ‘FileReader’ class in Java, and how does it handle character encoding when reading text files?
Answer: ‘FileReader’ is used to read character data from text files and relies on the platform’s default character encoding unless specified otherwise.
41. Explain the ‘Charset’ class in Java and its role in character encoding and decoding.
Answer: ‘Charset’ is used to represent character encodings and provides methods for encoding and decoding character data.
42. What is the ‘LineNumberReader’ class in Java, and how is it used to read text files while tracking line numbers?
Answer: ‘LineNumberReader’ is used to read text files and automatically track line numbers, making it useful for parsing structured text data.
43. Explain the ‘FileLock’ class in Java and its role in file locking for concurrent access control.
Answer: ‘FileLock’ is used for file locking, allowing you to control concurrent access to files by preventing multiple processes from modifying the same file simultaneously.
44. How do you read and write character data to a text file in Java using the ‘FileReader’ and ‘FileWriter’ classes?
Answer: You can use ‘FileReader’ to read character data and ‘FileWriter’ to write character data to text files in Java.
45. What is the ‘PrintStream’ class in Java, and how is it used for writing formatted text to text files and other output streams?
Answer: ‘PrintStream’ is used for writing formatted text to text files and other output streams, providing convenient methods for printing various data types.
46. Explain the ‘Paths’ class in Java and its role in creating ‘Path’ objects for file and directory operations.
Answer: ‘Paths’ provides methods for creating ‘Path’ objects, which represent file and directory paths and are used in various file operations.
47. What is the ‘File.deleteOnExit()’ method in Java, and how does it schedule a file for deletion when the JVM exits?
Answer: ‘File.deleteOnExit()’ schedules a file for deletion when the JVM exits, ensuring that temporary files are cleaned up.
48. How do you handle exceptions related to file operations, and what are some best practices for error handling?
Answer: File operation exceptions can be handled using try-catch blocks, and best practices include providing meaningful error messages and taking appropriate actions.
49. Explain the ‘File.toPath()’ method in Java and how it converts a ‘File’ object to a ‘Path’ object for enhanced file handling.
Answer: ‘File.toPath()’ converts a ‘File’ object to a ‘Path’ object, enabling better compatibility with modern file handling operations.
50. What is the ‘File.list()’ method in Java, and how is it used to obtain the names of files and directories within a directory?
Answer: ‘File.list()’ returns an array of file and directory names within a directory, allowing you to enumerate the contents of a directory.
Get Discount on Top Educational Courses
These questions cover various aspects of file handling in Java, including reading and writing text and binary files, file encoding, file locking, and the use of classes like ‘FileWriter,’ ‘FileReader,’ ‘FileChannel,’ and others.