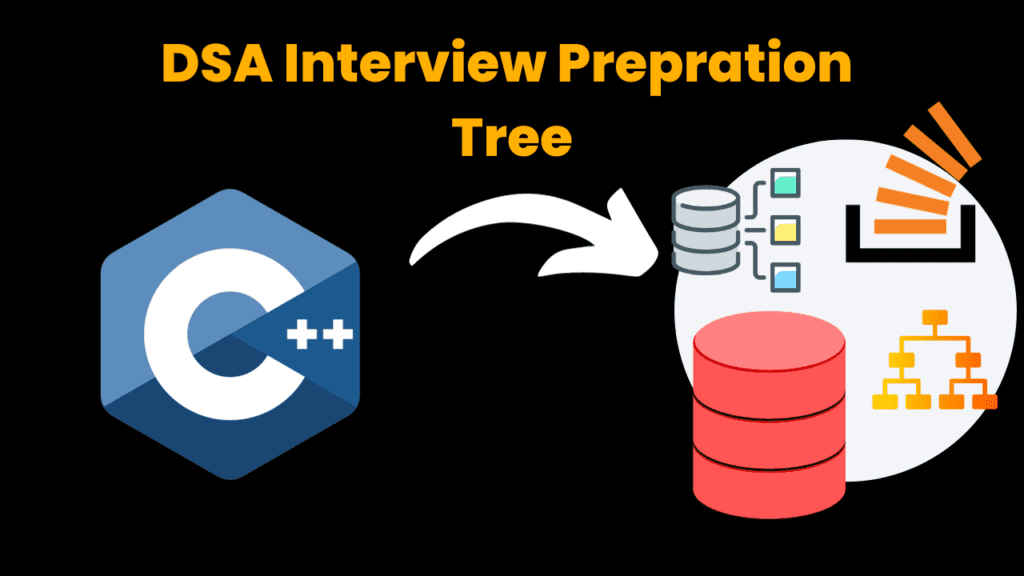
50 most frequently asked Tree interview questions.
Tree Basics:
1. What is a tree in C++?
Answer: A tree is a hierarchical data structure composed of nodes connected by edges. It consists of a root node, internal nodes, and leaf nodes.
2. Explain the difference between a tree and a graph in C++.
Answer: A tree is a special type of graph with no cycles, meaning that there are no closed loops or paths that visit the same node more than once.
3. How is a binary tree different from a general tree in C++?
Answer: In a binary tree, each node has at most two children, while in a general tree, a node can have any number of children.
4. What is a binary search tree (BST) in C++, and what properties should it satisfy?
Answer:A binary search tree is a binary tree where for each node:
- All nodes in its left subtree have values less than the node’s value.
- All nodes in its right subtree have values greater than the node’s value.
5. Explain the concept of a balanced tree in C++.
Answer: A balanced tree is a tree where the heights of the two subtrees of every node differ by at most one. It ensures that the tree remains relatively balanced, resulting in efficient operations.
Binary Tree Traversal:
6. What are the different types of binary tree traversals in C++?
Answer: The three main types of binary tree traversals are:
- Inorder traversal (left-root-right)
- Preorder traversal (root-left-right)
- Postorder traversal (left-right-root)
7. How can you implement an inorder traversal of a binary tree in C++ using recursion?
Answer: You can implement an inorder traversal using recursion as follows:
void inorderTraversal(TreeNode* root) {
if (!root) return;
inorderTraversal(root->left);
// Process the current node
inorderTraversal(root->right);
}
8. Explain the concept of level-order traversal in C++ and how it is implemented.
Answer: Level-order traversal visits nodes level by level, starting from the root and moving to the leftmost child of each level before visiting their siblings. It is typically implemented using a queue data structure.
Binary Search Tree Operations:
9. How do you insert a node into a binary search tree in C++?
Answer: To insert a node into a BST:
- If the tree is empty, create a new node and make it the root.
- Otherwise, start from the root and traverse the tree:
- If the value is less than the current node’s value, move to the left subtree.
- If the value is greater, move to the right subtree.
- Repeat until you find an empty spot to insert the new node.
10. What is the time complexity of searching for a node in a balanced binary search tree in C++?
Answer: In a balanced BST, the time complexity of searching for a node is O(log n), where n is the number of nodes in the tree.
11. How do you delete a node from a binary search tree in C++?
Answer: Deleting a node from a BST involves three cases:
- If the node has no children, simply remove it.
- If the node has one child, replace it with its child.
- If the node has two children, find its in-order successor or predecessor, replace the node with it, and delete the successor/predecessor.
12. Explain the concept of balancing a binary search tree in C++ and why it’s important.
Answer: Balancing a binary search tree ensures that the tree remains relatively balanced, which is crucial for maintaining efficient search, insert, and delete operations. Common balancing techniques include AVL trees and Red-Black trees.
Binary Tree Properties:
13. What is the height of a binary tree in C++?
Answer: The height of a binary tree is the length of the longest path from the root to a leaf node. It measures the tree’s depth.
14. Explain the difference between a full binary tree and a complete binary tree in C++.
Answer: In a full binary tree, every node has either 0 or 2 children. In a complete binary tree, all levels are completely filled except possibly for the last level, which is filled from left to right.
15. What is a perfect binary tree in C++,?
Answer: A perfect binary tree is a binary tree in which all internal nodes have exactly two children, and all leaf nodes are at the same level.
16. What is the concept of a binary tree’s depth and height in C++?
Answer: The depth of a node in a binary tree is the number of edges from the root to that node. The height of a binary tree is the length of the longest path from the root to a leaf node.
Binary Tree Variations:
17. What is a binary min-heap in C++,?
Answer: A binary min-heap is a complete binary tree where the value of each node is less than or equal to the values of its children. It’s often used to implement priority queues.
18. What is a binary max-heap in C++,?
Answer: A binary max-heap is a complete binary tree where the value of each node is greater than or equal to the values of its children. It’s also used for priority queues but with maximum priority elements at the root.
19. Explain the concept of a threaded binary tree in C++.
Answer: A threaded binary tree is a binary tree with additional threads (pointers) that allow for efficient in-order traversal without recursion or a stack.
20. What is a binary tree with a parent pointer in C++,?
Answer: A binary tree with a parent pointer includes an additional pointer in each node that points to its parent node. This can simplify certain tree-related operations.
Binary Tree Balance and Rotation:
21. What is a self-balancing binary search tree in C++?
Answer: A self-balancing binary search tree is a binary search tree that automatically maintains balance after insertions and deletions. Examples include AVL trees and Red-Black trees.
22. Explain the concept of an AVL tree in C++ and how it ensures balance.
Answer: An AVL tree is a self-balancing binary search tree where the balance factor (the height difference between left and right subtrees) of every node is limited to -1, 0, or 1. Balancing is achieved through rotations.
23. What is a Red-Black tree in C++,?
Answer: A Red-Black tree is a self-balancing binary search tree where nodes are colored red or black. It maintains balance by adhering to a set of rules, including color-changing and tree rotations.
24. Explain the concept of a B-tree in C++ and its applications.
Answer: A B-tree is a self-balancing tree structure that maintains sorted data and is commonly used in databases and file systems for efficient data storage and retrieval.
Advanced Binary Tree Operations:
25. How do you find the lowest common ancestor of two nodes in a binary tree in C++?
Answer: To find the lowest common ancestor, start from the root and traverse the tree. If one node is in the left subtree, and the other is in the right subtree, the current node is the lowest common ancestor.
26. What is a binary tree with lazy deletion in C++, and why is it used?
Answer: A binary tree with lazy deletion marks nodes as deleted without immediately removing them. This is useful for efficient undo and redo operations.
27. How can you serialize and deserialize a binary tree in C++?
Answer: You can serialize a binary tree by traversing it and saving the nodes’ values and structure to a file or string. Deserialization involves reconstructing the tree from the serialized data.
28. Explain the concept of a binary tree with a custom balancing policy in C++.
Answer: A binary tree with a custom balancing policy allows you to specify rules for balancing the tree, which can be tailored to specific application requirements.
29. What is a binary tree with memoization in C++,, and how is it used to optimize recursive algorithms?
Answer: A binary tree with memoization is used to optimize recursive algorithms by caching and reusing previously computed results for specific inputs.
30. How do you find the lowest common ancestor of two nodes in a binary tree in C++?
Answer: To find the lowest common ancestor, start from the root and traverse the tree. If one node is in the left subtree, and the other is in the right subtree, the current node is the lowest common ancestor.
Tree Traversal:
31. Explain the concept of Morris traversal in C++ for binary trees.
Answer: Morris traversal is an in-order traversal of a binary tree without using additional data structures. It modifies the tree’s structure temporarily to traverse it efficiently.
32. What is the difference between depth-first search (DFS) and breadth-first search (BFS) for tree traversal in C++?
Answer: DFS explores as far as possible along one branch before backtracking, while BFS explores all nodes at the current depth before moving to the next level.
33. How can you implement a preorder traversal of a binary tree iteratively in C++ without using recursion?
34. Answer: You can use a stack to implement iterative preorder traversal. Push the root onto the stack, then repeatedly pop nodes, process them, and push their right and left children onto the stack.
35. Explain the concept of in-order predecessor and successor in a binary search tree (BST) in C++.
Answer: The in-order predecessor is the node with the largest value smaller than a given node’s value, and the in-order successor is the node with the smallest value greater than the given node’s value.
Binary Search Trees (BST):
36. How can you determine if a binary tree is a binary search tree (BST) in C++?
Answer: To check if a binary tree is a BST, perform an in-order traversal and ensure that the values are in ascending order.
37. What is the concept of a self-balancing binary search tree (BST) in C++,?
Answer: A self-balancing BST automatically maintains balance during insertions and deletions to ensure that the tree remains relatively balanced, leading to efficient operations.
38. Explain the difference between an AVL tree and a Red-Black tree in C++ and when to use each.
Answer: Both AVL and Red-Black trees are self-balancing BSTs, but AVL trees provide faster lookups while Red-Black trees have faster insertions and deletions. Use AVL trees when lookups are more frequent and Red-Black trees when insertions and deletions are common.
39. What is a binary tree with a lazy deletion policy in C++,?
Answer: A binary tree with lazy deletion marks nodes as deleted but doesn’t remove them immediately. Deletions are performed only when necessary to optimize performance.
Tree Operations:
40. How do you find the diameter of a binary tree in C++ (the longest path between any two nodes)?
Answer: To find the diameter of a binary tree, find the longest path between two nodes. It can be the diameter of the left subtree, the diameter of the right subtree, or the path that passes through the root.
41. Explain the concept of a binary tree with a custom comparator in C++ for determining the order of elements.
42. Answer: A binary tree with a custom comparator allows you to define custom rules for comparing elements, enabling you to build trees with different orderings.
43. What is a binary search tree (BST) iterator in C++, and how is it implemented?
Answer: A BST iterator is an object that iterates through the nodes of a BST in ascending order. It’s implemented using in-order traversal, either with recursion or a stack.
Advanced Tree Concepts:
44. Explain the concept of a binary indexed tree (BIT) or Fenwick tree in C++, and what are its applications?
Answer: A BIT or Fenwick tree is a data structure used for efficient updates and queries on an array of values. It has applications in solving problems related to cumulative frequency and range queries.
45. What is the concept of a trie (prefix tree) in C++?
Answer: A trie is a tree-like data structure used to store a dynamic set of strings. It’s often used in dictionary searches and string-related problems.
46. Explain the concept of a Cartesian tree in C++, and how is it constructed?
Answer: A Cartesian tree is a binary tree derived from a sequence of numbers. It is constructed using a stack to efficiently find the parent of each node.
47. What is a tree with an LRU (Least Recently Used) eviction policy in C++, used for in caching?
Answer: A tree with an LRU eviction policy ensures that the least recently used items are removed from the tree when it reaches its capacity, making it useful for caching and maintaining frequently accessed data.
Tree Variations:
48. Explain the concept of a binary heap in C++ and its two main variations (min-heap and max-heap).
Answer: A binary heap is a complete binary tree with two main variations:
- Min-heap: The parent node has a value less than or equal to its children.
- Max-heap: The parent node has a value greater than or equal to its children.
49. What is a quadtree in C++,?
Answer: A quadtree is a tree data structure in which each internal node has exactly four children. It’s often used for spatial indexing and efficient retrieval of multidimensional data.
50. Explain the concept of an octree in C++ and its applications.
Answer: An octree is a tree structure in which each internal node has eight children. It’s used for 3D space partitioning and efficient storage and retrieval of volumetric data.
These questions and answers cover various aspects of trees in C++, from basic concepts to more advanced data structures and algorithms that use trees. Understanding these topics will help you prepare for interviews and deepen your knowledge of tree-based data structures.