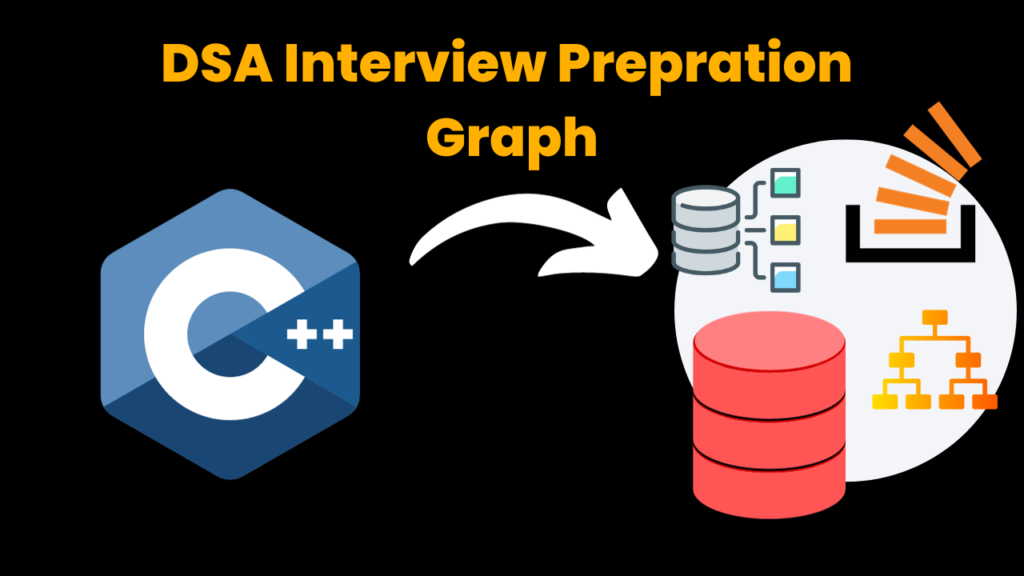
50 most frequently asked Graphs interview questions.
Graph Basics:
1. What is a graph in C++?
Answer: A graph is a data structure that consists of a set of nodes (vertices) connected by edges. It models relationships or connections between entities.
2. Explain the difference between a directed graph and an undirected graph in C++.
Answer: In a directed graph, edges have a direction, meaning they go from one vertex to another. In an undirected graph, edges have no direction and simply represent a connection between two vertices.
3. What is a weighted graph in C++?
Answer: A weighted graph is a graph in which each edge has an associated weight or cost. These weights represent the cost of traversing the edge and are used in algorithms like Dijkstra’s and Prim’s.
4. What is the difference between a sparse graph and a dense graph in C++?
Answer: A sparse graph has relatively few edges compared to the maximum possible number of edges. In contrast, a dense graph has a significant number of edges close to the maximum possible.
5. What is a bipartite graph in C++?
Answer: A bipartite graph is a graph whose vertices can be divided into two disjoint sets such that no two vertices within the same set are adjacent. It’s used to model relationships with no common connections.
Graph Representation:
6. Explain the adjacency matrix representation of a graph in C++.
Answer: An adjacency matrix is a 2D array where each cell at (i, j) represents whether there is an edge between vertex i and vertex j. It’s used for dense graphs.
7. What is the adjacency list representation of a graph in C++?
Answer: An adjacency list is a collection of lists or arrays, where each vertex has a list of its neighboring vertices. It’s used for sparse graphs.
8. Explain the concept of an edge list representation in C++.
Answer: An edge list is a list of pairs (u, v), where each pair represents an edge between vertex u and vertex v. It’s a simple representation that can be used for both sparse and dense graphs.
9. What is an incidence matrix in C++, and how is it used to represent graphs?
Answer: An incidence matrix is a 2D array where rows represent vertices, and columns represent edges. It indicates whether a vertex is incident to an edge. It’s commonly used for bipartite graphs and network flow problems.
10. How can you represent a graph with weighted edges in C++?
Answer: You can represent a graph with weighted edges by extending the adjacency list or adjacency matrix to include weights associated with each edge.
Graph Traversal:
11. What is depth-first search (DFS) in C++ for graph traversal, and how is it implemented?
Answer: DFS is a traversal algorithm that explores as far as possible along one branch before backtracking. It can be implemented using recursion or a stack.
12. Explain breadth-first search (BFS) in C++ for graph traversal and how it works.
Answer: BFS is a traversal algorithm that explores all nodes at the current level before moving to the next level. It is typically implemented using a queue.
13. What is the difference between DFS and BFS in C++ for graph traversal?
Answer: DFS explores as deeply as possible before backtracking, while BFS explores all nodes at the current level before moving to the next level. DFS often uses less memory.
14. How can you find the shortest path between two vertices in an unweighted graph in C++?
Answer: You can find the shortest path between two vertices in an unweighted graph using BFS, starting from the source vertex and stopping when you reach the target vertex.
15. Explain the concept of topological sorting in C++ and its application.
Answer: Topological sorting is a linear ordering of vertices in a directed acyclic graph (DAG) such that for every directed edge (u, v), vertex u comes before v in the ordering. It’s used in scheduling and task ordering problems.
Graph Algorithms:
16. What is Dijkstra’s algorithm in C++, for finding the shortest path in a weighted graph, and how does it work?
Answer: Dijkstra’s algorithm finds the shortest path from a source vertex to all other vertices in a weighted graph. It uses a priority queue or a min-heap to select the closest vertex at each step.
17. Explain the concept of the Bellman-Ford algorithm in C++ for finding the shortest path in a weighted graph with negative weights.
Answer: The Bellman-Ford algorithm finds the shortest path from a source vertex to all other vertices in a weighted graph, even if it contains negative-weight edges. It uses relaxation to update distance estimates iteratively.
18. What is Kruskal’s algorithm in C++ for finding the minimum spanning tree (MST) of a weighted graph, and how does it work?
Answer: Kruskal’s algorithm finds the MST by repeatedly selecting the smallest edge that doesn’t form a cycle in the growing MST. It uses a disjoint-set data structure to efficiently detect cycles.
19. Explain the concept of Prim’s algorithm in C++ for finding the minimum spanning tree (MST) of a weighted graph.
20. Answer: Prim’s algorithm starts with an arbitrary vertex and grows the MST by repeatedly selecting the smallest edge that connects a vertex in the MST to a vertex outside the MST.
21. What is the Floyd-Warshall algorithm in C++ for finding all-pairs shortest paths in a weighted graph, and how does it work?
Answer: The Floyd-Warshall algorithm finds the shortest paths between all pairs of vertices in a weighted graph. It uses dynamic programming and considers all possible paths.
Advanced Graph Concepts:
22. Explain the concept of a directed acyclic graph (DAG) in C++ and its significance.
Answer: A DAG is a directed graph with no cycles. It’s used in applications where dependencies must be represented without circular references, such as task scheduling.
23. What is the concept of a strongly connected component (SCC) in C++ within a directed graph?
Answer: A strongly connected component is a subgraph where every pair of vertices can reach each other. Finding SCCs is useful for analyzing the connectivity of directed graphs.
24. Explain the concept of a bipartite graph in C++ and how to check if a graph is bipartite.
Answer: A bipartite graph is one that can be divided into two disjoint sets such that no two vertices within the same set are adjacent. You can check bipartiteness using BFS or DFS.
25. What is a minimum cut in C++, in a flow network, and how is it found using algorithms like Ford-Fulkerson?
Answer: A minimum cut in a flow network is the smallest total capacity that, if removed, disconnects the source from the sink. Algorithms like Ford-Fulkerson find the maximum flow and, as a result, the minimum cut.
26. Explain the concept of a traveling salesman problem (TSP) in C++ and its significance in optimization.
Answer: The TSP is a classic optimization problem where a salesman seeks to find the shortest route that visits a set of cities exactly once and returns to the starting city. It has applications in logistics and route planning.
Graph Variations:
27. What is the concept of a directed graph with cycles in C++?
Answer: A directed graph with cycles contains one or more directed paths that form closed loops. These cycles can affect reachability and connectivity.
28. Explain the concept of a multigraph in C++, and how is it different from a simple graph?
Answer: A multigraph is a graph that can have multiple edges between the same pair of vertices, possibly with different weights. In contrast, a simple graph has at most one edge between any two vertices.
29. What is a planar graph in C++,?
Answer: A planar graph is a graph that can be drawn in the plane without any edges crossing each other. Planarity is important in circuit design and map theory.
30. Explain the concept of a hypergraph in C++, and how is it used to represent relationships with more than two entities?
Answer: A hypergraph is a generalization of a graph where edges can connect more than two vertices. It’s used to represent relationships involving multiple entities simultaneously.
31. What is a directed acyclic word graph (DAWG) in C++, and how is it used in string processing?
Answer: A DAWG is a data structure used to represent a set of strings efficiently, such as in dictionaries and spell-checkers. It minimizes redundancy by merging common prefixes.
Graph Operations:
32. Explain the concept of a Hamiltonian cycle in C++ within a graph.
Answer: A Hamiltonian cycle is a cycle that visits every vertex in a graph exactly once and returns to the starting vertex. Finding a Hamiltonian cycle is an NP-complete problem.
33. What is the Eulerian path and Eulerian circuit in C++ within a graph, and how are they different?
Answer: An Eulerian path is a path that traverses every edge in a graph exactly once, but it may not return to the starting vertex. An Eulerian circuit is an Eulerian path that starts and ends at the same vertex.
34. Explain the concept of a graph with a custom edge type in C++, and why it’s useful.
Answer: A graph with a custom edge type allows you to associate additional information (e.g., labels, capacities, or costs) with edges, making it more versatile for various applications.
35. What is the concept of a graph with attributes in C++?
Answer: A graph with attributes includes attributes or properties associated with vertices and edges. These attributes can be used for additional information or metadata.
36. Explain the concept of a graph with dynamic resizing in C++ for handling graph growth.
Answer: A graph with dynamic resizing automatically resizes its data structures (e.g., adjacency lists or matrices) as vertices and edges are added or removed, ensuring efficient memory usage.
Advanced Graph Algorithms:
37. What is the concept of the A algorithm in C++ for finding the shortest path in a graph, and how does it work?*
Answer: The A* algorithm is an informed search algorithm that uses heuristics to efficiently find the shortest path in a graph. It combines the cost to reach a node and a heuristic estimate of the cost to reach the goal.
38. Explain the concept of the Kruskal-Katona algorithm in C++ for generating combinatorial structures like graphs.
Answer: The Kruskal-Katona algorithm generates combinatorial structures, such as graphs with specific properties or structures with certain constraints. It’s used in combinatorics and discrete mathematics.
39. What is a graph with multi-objective optimization in C++?
Answer: A graph with multi-objective optimization involves optimizing multiple objectives (e.g., cost and time) simultaneously, often using techniques like Pareto optimization.
40. Explain the concept of a graph with time-dependent edges in C++ and its applications.
Answer: A graph with time-dependent edges has edges with variable weights that change over time. It’s used in modeling dynamic networks and transportation systems.
41. What is the concept of a graph with custom routing algorithms in C++ for optimizing network traffic?
Answer: A graph with custom routing algorithms allows for the implementation of specialized routing strategies, such as Quality of Service (QoS) routing, in network applications.
Graph Variations:
42. Explain the concept of a random graph in C++ and its applications in modeling probabilistic systems.
Answer: A random graph is a graph generated based on a probability distribution. It’s used in modeling and analyzing probabilistic systems, social networks, and random phenomena.
43. What is a planar embedding of a graph in C++,?
Answer: A planar embedding of a graph is a drawing of the graph on a plane such that no edges intersect except at their endpoints. Planar embeddings are important in graph theory and map-related problems.
44. Explain the concept of a graph with node and edge capacities in C++, and how is it used in flow networks.
Answer: A graph with node and edge capacities includes capacities assigned to both vertices and edges. It’s used in flow networks to model constraints on flow.
45. What is a self-loop in a graph in C++,?
Answer: A self-loop is an edge in a graph that connects a vertex to itself. Self-loops can represent self-referencing relationships or loops in certain applications.
46. Explain the concept of a complete graph in C++, and what is its significance in graph theory.
Answer: A complete graph is a graph where there is an edge between every pair of distinct vertices. It’s often used as a theoretical construct in graph theory and combinatorics.
Graph Theory Applications:
47. What is the concept of a graph with probabilistic edges in C++ and its use in modeling uncertain networks?
Answer: A graph with probabilistic edges includes edges with associated probabilities. It’s used to model networks where edge existence or reliability is uncertain, such as communication networks.
48. Explain the concept of a graph with edge capacities and demands in C++ for solving network flow problems.
Answer: A graph with edge capacities and demands involves assigning both capacity limits and flow demands to edges. It’s used in network flow problems, such as the maximum flow problem.
49. What is the concept of a graph with time-varying weights in C++, and how is it used in scheduling and optimization?
Answer: A graph with time-varying weights assigns changing weights to edges over time. It’s used in scheduling, resource allocation, and optimization problems that account for changing costs or constraints.
50. Explain the concept of a hierarchical graph in C++ and its applications in representing complex systems.
Answer: A hierarchical graph is a graph structure that contains nested subgraphs or layers, representing different levels of abstraction in complex systems, such as organizational structures or software architectures.
These questions and answers provide a comprehensive overview of various graph-related topics in C++, covering basics, representations, traversal, algorithms, advanced concepts, and variations. Understanding these topics will help you prepare for interviews and deepen your knowledge of graph data structures and algorithms.