GUI Calendar Project In Python
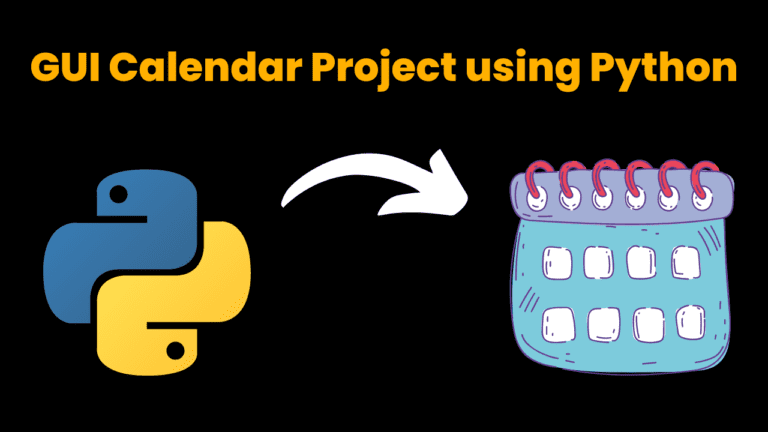
Introduction:
A calendar is used in our daily life. Here we will build a GUI(graphical user interface) Calendar project in python using a python module called Tkinter. Tkinter is a python module to create GUI applications. It is a great tool to build python applications.
This calendar is created with the calendar and the Tkinter module. you need to install both the calendar and the Tkinter module.
Requirements:
- For writing code, there must be a text editor on your pc (any text editor).
- There must be a python install in your system
- You must have python basic knowledge
That’s you’re ready to go
Let’s Start:
- Create a new file with any name you like
- Open that file with a text editor of your choice. We are using vs code.
- Now create a folder with calendar.py (you can use any name you like just add .py at the end)
- Now programming and programming logic begins (Final Code)
Source Code:
import calendar
from tkinter import *
# Codewithcurious.com/projects
def showCalender():
gui = Tk()
gui.config(background='white')
gui.title("Calender")
gui.geometry("550x600")
year = int(year_field.get())
gui_content = calendar.calendar(year)
calYear = Label(gui, text=gui_content, font="Consolas 10 bold")
calYear.grid(row=4, column=1, padx=20)
gui.mainloop()
if __name__ == '__main__':
new = Tk()
new.config(background='saddle brown')
new.title("Calender")
new.geometry("500x500")
cal = Label(new, text="Calender", bg="saddle brown",
fg="bisque", font=("times", 28, "bold"))
cal.grid(row=1, column=1)
year = Label(new, text="Enter Year", bg="saddle brown",
fg="bisque", font=("times", 20))
year.grid(row=2, column=1)
year_field = Entry(new, bg="bisque",)
year_field.grid(row=3, column=1)
button = Button(new, text="Show Calender", fg="saddle brown",
bg="bisque", command=showCalender)
button.grid(row=4, column=1)
new.mainloop()
Explanation:
- First, we import all the important libraries and modules
- Next, we have to use the Tkinter module to create our application GUI
- Then we created a showcalendar() function to take input to show the calendar of the entered year
- Then we created the next window to show our calendar by dates, days, and months
- Then we define the window size, font, and color.
That’s it your calendar is ready.
Keep visiting codewithcurious.com for more such projects.
Output:
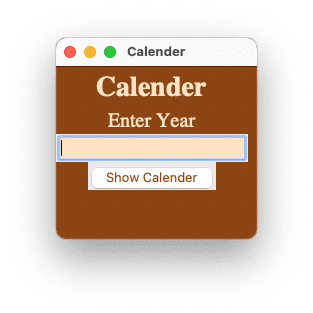
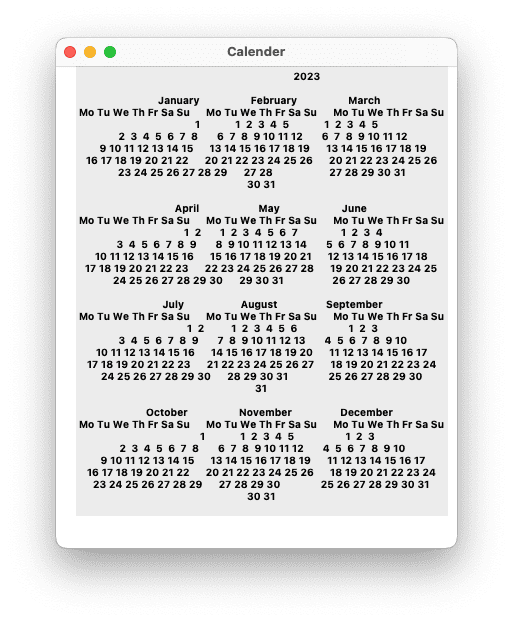
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …