Java Exception Handling Interview Questions
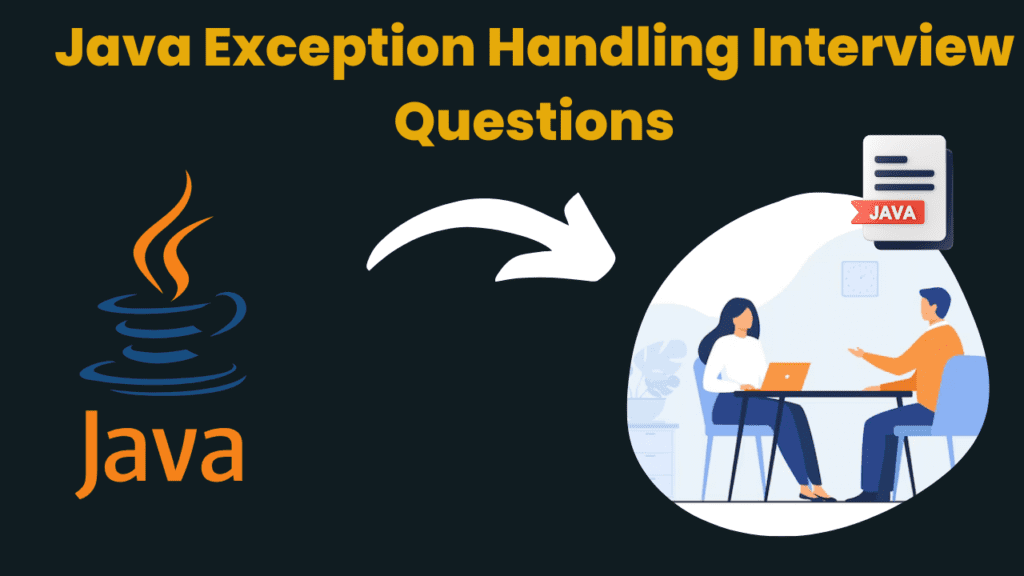
50 most frequently asked Java Exception Handling Interview Questions.
Get Discount on Top Educational Courses
1. What is an exception in Java, and why is it essential for error handling?
Answer: An exception is an event that occurs during the execution of a program, disrupting the normal flow. It is essential for handling errors and exceptional conditions gracefully.
2. Explain the difference between checked exceptions and unchecked exceptions in Java.
Answer: Checked exceptions are checked at compile time, while unchecked exceptions (RuntimeExceptions) are not checked at compile time.
3. What is the ‘try-catch’ block, and how is it used for handling exceptions in Java?
Answer: The ‘try-catch’ block is used to catch and handle exceptions. Code that may throw an exception is placed in the ‘try’ block, and exception handling code is placed in the ‘catch’ block.
4. What is the purpose of the ‘throw’ keyword in Java, and how is it used to raise custom exceptions?
Answer: The ‘throw’ keyword is used to explicitly throw an exception. It is useful for raising custom exceptions or propagating existing ones.
5. Explain the ‘finally’ block in Java exception handling and its role in resource cleanup.
Answer: The ‘finally’ block is used for code that must be executed regardless of whether an exception occurs or not. It ensures resource cleanup or finalization.
6. What is the ‘try-with-resources’ statement in Java, and how does it simplify resource management and exception handling?
Answer: The ‘try-with-resources’ statement simplifies resource management by automatically closing resources like streams, ensuring proper cleanup and exception handling.
7. Explain the ‘catch’ block without an exception type (generic catch) and its use in handling multiple exceptions.
Answer: A catch block without an exception type can catch multiple exceptions, making it useful for handling different exceptions in a similar way.
8. What is the ‘throws’ clause in method declarations, and how does it indicate that a method may throw exceptions?
Answer: The ‘throws’ clause in method declarations specifies the exceptions that a method may throw. It provides information to the calling code about potential exceptions.
9. Explain the ‘try-catch-finally’ block and its role in exception handling when resource cleanup is required.
Answer: The ‘try-catch-finally’ block is used to handle exceptions and ensures that resources are properly cleaned up in the ‘finally’ block.
10. What is the ‘try-with-resources’ statement, and how does it simplify handling resources like files and streams in Java?
Answer: The ‘try-with-resources’ statement simplifies resource management by automatically closing resources at the end of the block, reducing the need for explicit resource cleanup.
11. What is the ‘try-with-resources’ statement, and how does it handle exceptions that occur during resource closure?
Answer: The ‘try-with-resources’ statement automatically closes resources and handles exceptions that occur during closure by suppressing them or adding them to the list of suppressed exceptions.
12. Explain the ‘finally’ block’s behavior when an exception is thrown in the ‘catch’ block.
Answer: The ‘finally’ block is executed even if an exception is thrown in the ‘catch’ block. It ensures that cleanup code is executed regardless of the exception outcome.
13. What is the purpose of the ‘catch’ block with multiple exception types, and how is it used to handle different exceptions differently?
Answer: A ‘catch’ block with multiple exception types is used to handle different exceptions in distinct ways, allowing specific exception handling for each type.
14. What is the ‘try-catch’ block with a ‘finally’ block used for, and how does it affect resource management?
Answer: A ‘try-catch’ block with a ‘finally’ block is used for exception handling and ensures that resources are properly cleaned up in the ‘finally’ block, even in the presence of exceptions.
15. What is the ‘try-with-resources’ statement, and how does it relate to resource management in Java?
Answer: The ‘try-with-resources’ statement simplifies resource management by automatically closing resources, making it easier to handle resources like files, streams, or sockets.
16. What is the ‘try-catch’ block with multiple ‘catch’ blocks, and how is it used for handling different exceptions?
Answer: A ‘try-catch’ block with multiple ‘catch’ blocks is used to handle different exceptions based on their types, providing distinct exception handling for each case.
17. What is the ‘try-catch’ block, and how does it prevent the propagation of exceptions to the calling code?
Answer: The ‘try-catch’ block catches exceptions and handles them within the block, preventing the exceptions from propagating to the calling code.
18. What is the ‘try-with-resources’ statement, and what interface must resources implement to be used with it?
Answer: The ‘try-with-resources’ statement is used for automatic resource management. Resources must implement the AutoCloseable interface to be used with it.
19 . Explain the ‘throw’ keyword in Java and how it is used for custom exception throwing.
Answer: The ‘throw’ keyword is used to explicitly throw an exception. It is useful for raising custom exceptions or propagating existing ones.
20. What is the ‘NullPointerException’ in Java, and how can it be avoided when working with object references?
Answer: ‘NullPointerException’ occurs when a program attempts to access or invoke a method on an object reference that is null. It can be avoided by checking for null references before using them.
21. What is the ‘ArrayIndexOutOfBoundsException’ in Java, and how can it be avoided when working with arrays?
Answer: ‘ArrayIndexOutOfBoundsException’ occurs when trying to access an array element with an index that is out of bounds. It can be avoided by ensuring that array indices are within the valid range.
22. Explain the ‘ArithmeticException’ in Java, and how can it be avoided when performing arithmetic operations?
Answer: ‘ArithmeticException’ occurs when performing arithmetic operations with invalid operands, such as division by zero. It can be avoided by validating inputs before performing operations.
23. What is the ‘NumberFormatException’ in Java, and how can it be avoided when parsing strings to numbers?
Answer: ‘NumberFormatException’ occurs when trying to convert a string to a numeric value that is not a valid number format. It can be avoided by validating input strings before parsing.
24. Explain the ‘ClassCastException’ in Java and how it can be avoided when working with typecasting.
Answer: ‘ClassCastException’ occurs when an invalid typecast is attempted. It can be avoided by using type-checking or ‘instanceof’ checks before typecasting.
25. What is the ‘FileNotFoundException’ in Java, and how can it be handled when working with file operations?
Answer: ‘FileNotFoundException’ occurs when trying to access a file that does not exist. It can be handled by checking for file existence and using proper error handling.
26. Explain the ‘IOException’ and its subclasses in Java, and how they are used for handling input and output errors.
Answer: ‘IOException’ and its subclasses are used to handle input and output errors. They provide a mechanism for handling errors during file or network operations.
27. What is the ‘IllegalArgumentException’ in Java, and how can it be avoided when validating method arguments?
Answer: ‘IllegalArgumentException’ occurs when an invalid argument is passed to a method. It can be avoided by validating method arguments and providing appropriate error messages.
28. Explain the ‘IllegalStateException’ in Java and its use in indicating that an object is in an inappropriate state for a given operation.
Answer: ‘IllegalStateException’ is thrown to indicate that an object is in an inappropriate state for a particular operation. It is often used to prevent invalid method calls.
29. What is the ‘NoSuchElementException’ in Java, and how is it used to indicate the absence of elements in collections?
Answer: ‘NoSuchElementException’ is thrown to indicate that no more elements are available in a collection. It is commonly used with iterators.
30. Explain the ‘NullPointerException’ in Java, its causes, and how it can be handled gracefully in code.
Answer: ‘NullPointerException’ occurs when attempting to access members or methods of a null object reference. It can be handled by checking for null references and providing appropriate error messages.
31. What is the ‘StackOverflowError’ in Java, and when does it typically occur?
Answer: ‘StackOverflowError’ occurs when the call stack exceeds its maximum depth. It typically happens when there is excessive recursion in a method.
32. Explain the ‘OutOfMemoryError’ in Java and its different subtypes, such as ‘Heap Space’ and ‘PermGen Space’ errors.
Answer: ‘OutOfMemoryError’ occurs when the Java Virtual Machine (JVM) runs out of memory. Subtypes like ‘Heap Space’ and ‘PermGen Space’ indicate the specific memory area that is exhausted.
33. What is the ‘NumberFormatException’ in Java, and how can it be handled when parsing strings to numbers?
Answer: ‘NumberFormatException’ is thrown when trying to convert a string to a number that is not a valid numeric format. It can be handled by validating input strings and using try-catch blocks for parsing.
34. Explain the ‘IllegalArgumentException’ in Java and its use in indicating that a method’s argument is inappropriate.
Answer: ‘IllegalArgumentException’ is thrown to indicate that an argument passed to a method is inappropriate or outside valid bounds. It is often used to validate method inputs.
35. What is the ‘ArithmeticException’ in Java, and how can it be avoided when performing arithmetic operations?
Answer: ‘ArithmeticException’ occurs when performing arithmetic operations with invalid operands, such as division by zero. It can be avoided by validating input values before performing operations.
36. What is the ‘IndexOutOfBoundsException’ in Java, and how can it be handled when accessing arrays and collections?
Answer: ‘IndexOutOfBoundsException’ occurs when trying to access an index that is outside the valid range of arrays or collections. It can be avoided by validating index values before access.
37. Explain the ‘ConcurrentModificationException’ in Java and its use in indicating concurrent modification of a collection during iteration.
Answer: ‘ConcurrentModificationException’ is thrown to indicate concurrent modification of a collection while it is being iterated. It is used to maintain collection integrity during iteration.
38. What is the ‘SecurityException’ in Java, and how is it used to indicate security violations or access restrictions?
Answer: ‘SecurityException’ is thrown to indicate security violations or access restrictions. It is used to prevent unauthorized access to certain resources.
39. Explain the ‘ClassNotFoundException’ in Java and its use in indicating the absence of a class during dynamic class loading.
Answer: ‘ClassNotFoundException’ is thrown when attempting to load a class that does not exist. It is used to handle situations where dynamic class loading fails.
40. What is the ‘UnsatisfiedLinkError’ in Java, and how is it used to indicate native method linkage issues?
Answer: ‘UnsatisfiedLinkError’ is thrown when there are issues with linking native methods to their implementations. It is used to handle problems related to native code libraries.
41. Explain the ‘AssertionError’ in Java and its use for programmatic assertions.
Answer: ‘AssertionError’ is thrown when an assertion fails. It is used for programmatic assertions, allowing developers to check conditions and react to unexpected states.
42. What is the ‘IllegalStateException’ in Java, and how does it indicate that an object is in an inappropriate state for a given operation?
Answer: ‘IllegalStateException’ is thrown to indicate that an object is in an inappropriate state for a particular operation. It is often used to prevent invalid method calls.
43. Explain the ‘FileNotFound’ exception in Java and its use in handling file-related errors.
Answer: ‘FileNotFound’ is thrown to indicate that a requested file or directory does not exist. It is used for handling errors when working with files.
44. What is the ‘NoSuchElementException’ in Java, and how is it used to indicate the absence of elements in collections?
Answer: ‘NoSuchElementException’ is thrown when attempting to access an element that does not exist in a collection. It is commonly used with iterators to indicate the end of a collection.
45. Explain the ‘UnsupportedOperationException’ in Java and its use for indicating that an operation is not supported by a particular collection or object.
Answer: ‘UnsupportedOperationException’ is thrown to indicate that a specific operation is not supported by a collection or object. It is often used for read-only collections.
46. What is the ‘NumberFormatException’ in Java, and how can it be avoided when parsing strings to numbers?
Answer: ‘NumberFormatException’ is thrown when trying to convert a string to a number that is not a valid numeric format. It can be avoided by validating input strings and using try-catch blocks for parsing.
47. Explain the ‘ClassCastException’ in Java and how it can be avoided when working with typecasting.
Answer: ‘ClassCastException’ occurs when an invalid typecast is attempted. It can be avoided by using type-checking or ‘instanceof’ checks before typecasting.
48. What is the ‘UnsupportedOperationException’ in Java, and how is it used to indicate that an operation is not supported by a collection?
Answer: ‘UnsupportedOperationException’ is thrown to indicate that a particular operation is not supported by a collection. It is often used with read-only or unmodifiable collections.
49. Explain the ‘ConcurrentModificationException’ in Java and how it can be avoided when modifying a collection during iteration.
Answer: ‘ConcurrentModificationException’ is thrown when a collection is modified while it is being iterated. It can be avoided by using concurrent collections or explicit synchronization.
50. What is the ‘ArrayIndexOutOfBoundsException’ in Java, and how can it be avoided when working with arrays?
Answer: ‘ArrayIndexOutOfBoundsException’ occurs when trying to access an array element with an index that is out of bounds. It can be avoided by ensuring that array indices are within the valid range.
Get Discount on Top Educational Courses
These questions cover various aspects of exception handling in Java, including exception types, exception propagation, try-catch blocks, and best practices for handling exceptions.