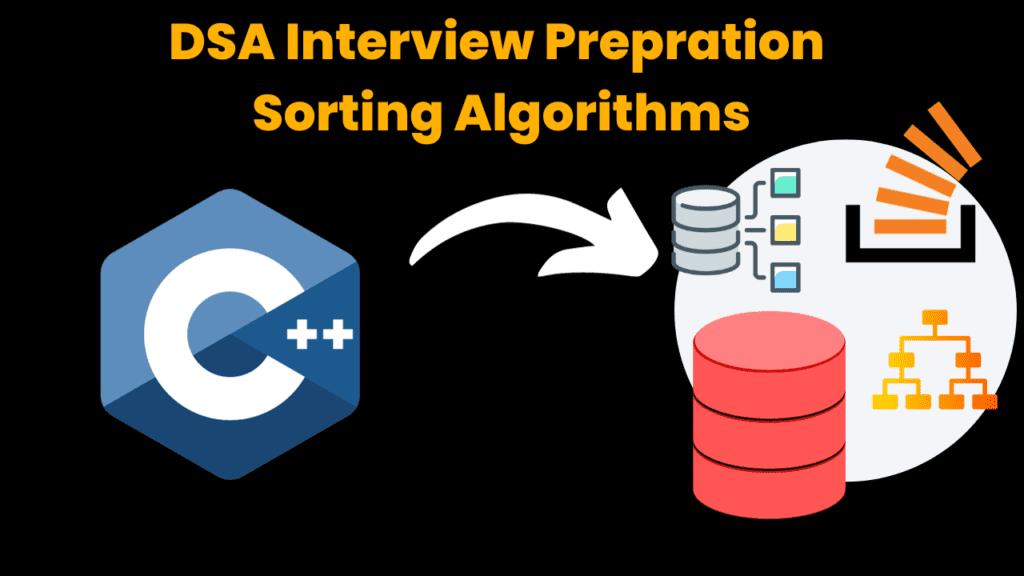
50 most frequently asked Sorting Algorithms interview questions.
Basics of Sorting Algorithms:
1. What is sorting in C++?
Answer: Sorting is the process of arranging a collection of data elements in a specific order, such as ascending or descending, based on certain criteria.
2. Differentiate between in-place and out-of-place sorting algorithms in C++.
Answer: In-place sorting algorithms modify the input data without requiring additional memory, while out-of-place algorithms create a separate copy of the data.
3. What is the significance of stable sorting algorithms in C++?
Answer: Stable sorting algorithms preserve the relative order of equal elements in the sorted output, which can be important in some applications.
4. Explain the time complexity notation O(n^2) in the context of sorting algorithms in C++.
Answer: O(n^2) indicates that the time complexity of an algorithm grows quadratically with the size of the input data. It is often associated with inefficient sorting algorithms like bubble sort and insertion sort.
5. What are comparison-based sorting algorithms in C++,, and what is their common time complexity lower bound?
6. Answer: Comparison-based sorting algorithms compare elements using only comparison operations (e.g., less than or equal to). Their common time complexity lower bound is O(n log n) for the worst-case scenario.
Sorting Algorithms Overview:
6. Name and briefly describe the bubble sort algorithm in C++.
Answer: Bubble sort repeatedly compares adjacent elements and swaps them if they are in the wrong order. It continues this process until no more swaps are needed.
7. Explain the concept of insertion sort in C++, and provide its time complexity.
Answer: Insertion sort builds the sorted array one element at a time by inserting each unsorted element into its correct position within the sorted part of the array. Its time complexity is O(n^2).
8. What is the selection sort algorithm in C++?
Answer: Selection sort repeatedly selects the minimum (or maximum) element from the unsorted part of the array and moves it to the sorted part.
9. Explain how merge sort works in C++, and provide its time complexity.
Answer: Merge sort divides the array into two halves, recursively sorts them, and then merges the sorted halves. Its time complexity is O(n log n).
10. What is the quicksort algorithm in C++?
Answer: Quicksort is a divide-and-conquer sorting algorithm that selects a pivot element and partitions the array into two subarrays: elements less than the pivot and elements greater than the pivot. It recursively sorts the subarrays.
Sorting Algorithms Continued:
11. Explain the concept of heap sort in C++, and provide its time complexity.
Answer: Heap sort converts the input array into a max-heap, repeatedly extracts the maximum element, and places it in the sorted portion of the array. Its time complexity is O(n log n).
12. What is the counting sort algorithm in C++, and when is it most suitable?
Answer: Counting sort is a non-comparison-based sorting algorithm that works well for sorting integers with a known range. Its time complexity is O(n + k), where k is the range of values.
13. Explain the radix sort algorithm in C++ and its application to sorting non-integer data.
Answer: Radix sort sorts data by processing digits or individual characters. It is suitable for sorting strings and non-integer data types.
14. What is the bucket sort algorithm in C++, and under what conditions is it efficient?
Answer: Bucket sort divides the input into buckets, sorts each bucket, and concatenates them. It is efficient when the data is uniformly distributed and falls within a known range.
15. Explain the concept of timSort in C++, and why is it used in modern programming languages?
Answer: TimSort is a hybrid sorting algorithm that combines elements of merge sort and insertion sort. It is used in modern programming languages because it performs well on real-world data and takes advantage of pre-existing order in data.
Sorting Algorithms Applications:
16. How is sorting used in C++ for implementing efficient searching algorithms like binary search?
Answer: Binary search relies on the input data being sorted, allowing for efficient searching by repeatedly dividing the search space in half.
17. Explain the use of sorting in C++ for duplicate detection and removal in a dataset.
Answer: Sorting data simplifies duplicate detection as identical elements are adjacent. Removing duplicates involves iterating through the sorted data and eliminating duplicates.
18. How is sorting used in C++ for data analysis and statistics, such as finding the median and quartiles of a dataset?
Answer: Sorting is used to arrange data in ascending or descending order, making it easier to find the median, quartiles, and other statistical measures.
19. Explain the role of sorting in C++ in optimizing cache usage and improving memory access patterns.
Answer: Sorting data can improve cache performance and memory access patterns by reducing cache misses and optimizing data locality.
20. How do sorting algorithms contribute to optimizing database query performance in C++?
Answer: Sorting indexed data in a database allows for efficient retrieval of sorted results, improving query performance for ordered queries.
Sorting Algorithms Properties:
21. Explain the stability property of sorting algorithms in C++. and provide an example.
Answer: Stable sorting algorithms preserve the relative order of equal elements. For example, if you sort a list of people by age using a stable sort, those with the same age will remain in the same order as they were before sorting.
22. What is an in-place sorting algorithm in C++, and why is it advantageous in certain scenarios?
Answer: An in-place sorting algorithm sorts the data without requiring additional memory. It is advantageous in scenarios with limited memory or where memory allocation is costly.
23. What is an unstable sorting algorithm in C++,, and when might its instability not matter?
Answer: An unstable sorting algorithm does not guarantee the preservation of the relative order of equal elements. Instability may not matter when the relative order of equal elements is irrelevant.
24. Explain the concept of an adaptive sorting algorithm in C++ and its ability to adapt to partially sorted data.
Answer: Adaptive sorting algorithms are efficient when the input data is partially sorted, making them well-suited for scenarios where the data is already partially ordered.
25. What is the worst-case time complexity of quicksort in C++,, and how can it be mitigated?
Answer: The worst-case time complexity of quicksort is O(n^2) when the pivot selection is poor. Mitigation strategies include selecting a good pivot and using hybrid algorithms like introsort.
Sorting Algorithm Techniques:
26. What is the concept of divide and conquer in the context of sorting algorithms in C++?
Answer: Divide and conquer is a technique used in sorting algorithms where the problem is divided into smaller subproblems, solved recursively, and then combined to obtain the final sorted result.
27. Explain the term “comparison-based sorting” in C++,, and why is it important in algorithm analysis?
Answer: Comparison-based sorting relies on comparing elements using comparison operations. It is important in algorithm analysis because most sorting algorithms fall into this category, and their performance is analyzed based on the number of comparisons.
28. What is the principle behind non-comparison-based sorting algorithms in C++,, and how do they achieve efficiency?
Answer: Non-comparison-based sorting algorithms, like counting sort and radix sort, sort elements without directly comparing them. They achieve efficiency by exploiting specific properties of the data, such as integer values.
29. Explain the concept of hybrid sorting algorithms in C++, and provide an example.
Answer: Hybrid sorting algorithms combine multiple sorting techniques to take advantage of their strengths. For example, introsort combines quicksort and heapsort to balance quicksort’s worst-case scenario.
30. What is the concept of adaptive sorting algorithms in C++?
Answer: Adaptive sorting algorithms are designed to perform efficiently when the input data is partially sorted or already in order. They adapt their behavior based on the characteristics of the data.
Sorting Algorithm Analysis:
31. What is the difference between the best-case, average-case, and worst-case time complexities of a sorting algorithm in C++?
Answer: The best-case time complexity represents the smallest number of operations for an input, the worst-case represents the largest, and the average-case is an average of all possible inputs.
32. Explain the concept of asymptotic notation (e.g., O, Θ, Ω) in analyzing sorting algorithms in C++.
Answer: Asymptotic notation is used to describe the upper bound (O notation), tight bound (Θ notation), and lower bound (Ω notation) of an algorithm’s time complexity, providing a simplified representation of its growth rate.
33. What is the time complexity of the bubble sort algorithm in C++ in the worst-case scenario?
Answer: The worst-case time complexity of bubble sort is O(n^2), where “n” is the number of elements to be sorted.
34. What is the time complexity of the selection sort algorithm in C++ in the best-case scenario?
Answer: The best-case time complexity of selection sort is also O(n^2), as it always makes the same number of comparisons.
35. How does the choice of pivot element impact the time complexity of quicksort in C++?
Answer: The choice of pivot element can significantly impact the time complexity of quicksort. A good pivot selection reduces the number of comparisons and leads to better performance.
Sorting Algorithm Optimizations:
36. Explain the concept of stable partitioning in C++ and its role in stable sorting algorithms.
Answer: Stable partitioning ensures that elements with equal keys remain in their original relative order after sorting, which is essential for maintaining stability in sorting algorithms.
37. What is the concept of three-way partitioning in C++ sorting algorithms, and when is it useful?
Answer: Three-way partitioning is used in some sorting algorithms, like quicksort, to efficiently handle duplicate elements by partitioning the array into three parts: elements less than the pivot, equal to the pivot, and greater than the pivot.
38. Explain the concept of in-place merge in C++ sorting algorithms and its advantages.
Answer: In-place merge is a technique that merges two sorted subarrays without requiring additional memory. It is advantageous in reducing memory overhead for merge operations.
39. What is the concept of adaptive merge sort in C++, and how does it adapt to the data distribution?
Answer: Adaptive merge sort is a variation of merge sort that adapts to the data distribution. It switches to a more efficient algorithm, like insertion sort, for small subarrays to minimize overhead.
40. Explain the concept of block sort in C++ sorting algorithms and its use in external sorting.
Answer: Block sort is a sorting technique used in external sorting, where data does not fit entirely in memory. It operates on blocks of data, minimizing the need for extensive disk I/O operations.
Sorting Algorithm Variations:
41. What is the concept of stable sorting in C++,, and how does it differ from unstable sorting?
Answer: Stable sorting algorithms maintain the relative order of equal elements in the sorted output, while unstable sorting algorithms do not guarantee this property.
42. Explain the concept of in-place sorting in C++, and provide an example of an in-place sorting algorithm.
Answer: In-place sorting algorithms sort the data without using additional memory proportional to the input size. An example is the Quicksort algorithm, which sorts data in-place by swapping elements.
43. What are the advantages of using non-comparison-based sorting algorithms like counting sort and radix sort in C++ for specific types of data?
Answer: Non-comparison-based sorting algorithms have linear time complexity and are advantageous when sorting data with a known range, such as integers or strings with fixed lengths.
44. Explain the concept of external sorting in C++ and its relevance in scenarios where data does not fit entirely in memory.
Answer: External sorting is used when the data to be sorted is too large to fit in memory. It involves dividing the data into blocks, sorting each block in memory, and then merging the sorted blocks to produce the final sorted result.
45. What are the key characteristics and advantages of hybrid sorting algorithms like introsort in C++?
Answer: Hybrid sorting algorithms combine multiple sorting techniques to take advantage of their strengths. Introsort, for example, uses quicksort and heapsort to achieve good average-case performance with worst-case guarantees.
Sorting Algorithm Properties:
46. What are the key factors to consider when choosing a sorting algorithm in C++ for a specific task or dataset?
Answer: Factors to consider include the size of the dataset, memory constraints, data distribution, stability requirements, and desired time complexity.
47. Explain the concept of a stable sorting algorithm in C++ and why it may be important in certain applications.
Answer: A stable sorting algorithm preserves the relative order of equal elements in the sorted output. This property is important when maintaining the order of elements based on multiple criteria or when stability is a requirement.
48. What is the significance of in-place sorting algorithms in C++, and how can they impact memory usage and performance?
Answer: In-place sorting algorithms sort data without using additional memory, making them memory-efficient. They can also have lower overhead and better cache performance.
49. What is the time complexity of quicksort in C++ on average, and how does it compare to other sorting algorithms like merge sort and heapsort?
Answer: Quicksort has an average-case time complexity of O(n log n), which is the same as merge sort and heapsort. However, quicksort often performs better in practice due to its low constant factors.
50. Explain the role of sorting algorithms in the context of real-world applications and scenarios where sorted data is essential.
Answer: Sorting algorithms are fundamental to various real-world applications, such as databases, search engines, financial systems, and data analysis, where sorted data enables efficient querying, analysis, and reporting.
These questions and answers cover a wide range of topics related to sorting algorithms in C++, including basics, algorithms, properties, applications, and more. Understanding these concepts will help you prepare for interviews and gain a deeper knowledge of sorting algorithms and their use in programming.