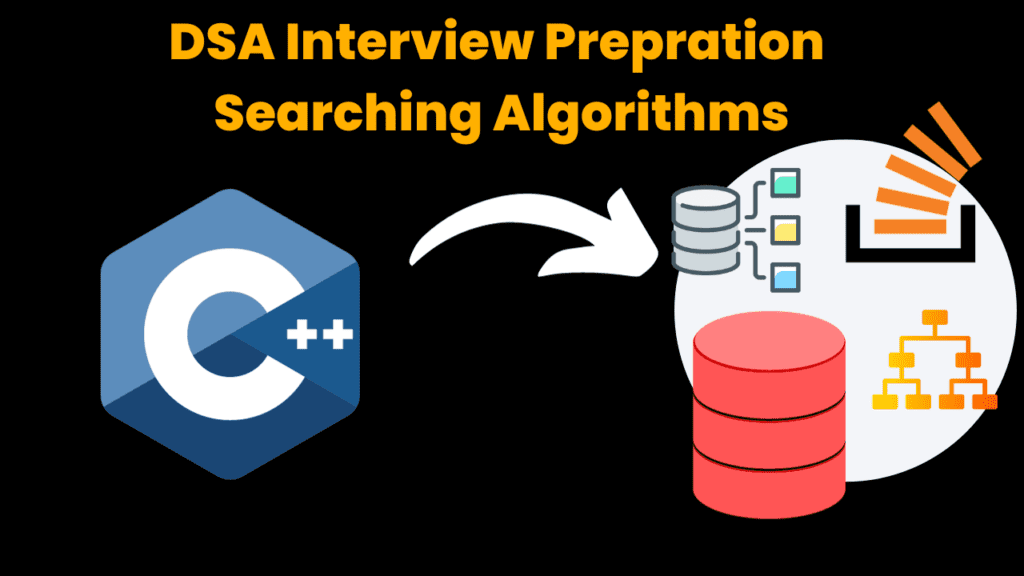
50 most frequently asked Searching Algorithms interview questions.
Basics of Searching Algorithms:
1.What is searching in C++?
Answer: Searching is the process of finding a specific target element within a collection of data, such as an array or a list.
2. Differentiate between linear search and binary search in C++.
Answer: Linear search involves checking each element in the collection one by one until the target element is found. Binary search, on the other hand, requires a sorted collection and repeatedly dividing it in half to narrow down the search.
3. Explain the concept of a search key in C++, and why is it important in searching algorithms?
Answer: A search key is the value being searched for in the data collection. It’s crucial because it defines what you are trying to find.
4. What is the significance of searching algorithms in C++, and where are they commonly used?
Answer: Searching algorithms are essential in various applications, including databases, information retrieval, sorting algorithms, and more, where efficient data retrieval is required.
5. What is the time complexity of linear search in C++ and binary search in the worst case?
Answer: The worst-case time complexity of linear search is O(n), while the worst-case time complexity of binary search is O(log n), where “n” is the number of elements in the collection.
Linear Searching Algorithms:
6. Explain how linear search works in C++, and provide an example of its implementation.
Answer: Linear search checks each element in the collection sequentially until the target element is found or the entire collection has been checked. Here’s a C++ example:
int linearSearch(int arr[], int n, int target) {
for (int i = 0; i < n; i++) {
if (arr[i] == target) {
return i; // Element found at index i
}
}
return -1; // Element not found
}
7. What are the advantages and disadvantages of linear search in C++?
Answer: Advantages of linear search include simplicity and applicability to unsorted data. Disadvantages include its O(n) worst-case time complexity, which can be inefficient for large datasets.
8. Explain the concept of sentinel linear search in C++, and how does it improve efficiency?
Answer: Sentinel linear search involves placing a sentinel value at the end of the array. It eliminates the need to check for the end of the array in each iteration, potentially improving search efficiency.
9. What is a self-organizing list in C++,, and how does it optimize linear searches over time?
Answer: A self-organizing list rearranges elements based on search frequency. Frequently accessed elements are moved toward the front, reducing search time for commonly used values.
10. Explain the concept of a move-to-front heuristic in C++, and how does it optimize linear searches?
Answer: The move-to-front heuristic places the accessed element at the front of the list. This optimizes linear searches by favoring recently accessed elements, reducing search time.
Binary Searching Algorithms:
11. How does binary search work in C++, and why is it efficient for sorted collections?
Answer: Binary search repeatedly divides the collection in half, reducing the search space logarithmically. It is efficient for sorted collections because it eliminates half of the remaining elements in each iteration.
12. Explain the recursive implementation of binary search in C++, and provide a code example.
Answer: Here’s a recursive C++ implementation of binary search:
int binarySearch(int arr[], int left, int right, int target) {
if (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid; // Element found at index mid
}
if (arr[mid] < target) {
return binarySearch(arr, mid + 1, right, target);
}
return binarySearch(arr, left, mid - 1, target);
}
return -1; // Element not found
}
13. What are the advantages and limitations of binary search in C++?
Answer: Advantages of binary search include its O(log n) worst-case time complexity for sorted data. Limitations include the requirement for sorted data and the overhead of maintaining the sort order.
14. Explain the concept of interpolation search in C++, and how does it improve efficiency for uniformly distributed data?
Answer: Interpolation search uses interpolation formulae to estimate the position of the target element based on its value. It is efficient for uniformly distributed data but may perform poorly for unevenly distributed data.
15. What is exponential search in C++, and in what scenarios is it useful?
Answer: Exponential search involves doubling the search range until a range containing the target element is found. It is useful when the location of the target is unknown in a large collection.
Searching Algorithm Variations:
16. Explain the concept of binary search tree (BST) in C++,,, and how is it used for searching and data organization?
Answer: A binary search tree is a data structure where each node has at most two children, and the left subtree contains elements less than the node, while the right subtree contains elements greater than the node. It is used for efficient searching and data organization.
17. What is a self-balancing binary search tree (BST) in C++,, and why is it important for maintaining search efficiency?
Answer: A self-balancing BST automatically maintains its balance, ensuring that the tree remains reasonably balanced during insertions and deletions. This is crucial for maintaining efficient search times.
18. Explain the concept of a hash table in C++ and how it is used for efficient searching.
Answer: A hash table is a data structure that uses a hash function to map keys to indexes in an array. It enables fast search, insert, and delete operations, making it efficient for searching.
19. What is the concept of a search algorithm’s complexity in C++,,, and how is it determined?
Answer: A search algorithm’s complexity refers to the amount of time and/or memory it requires. It is determined by analyzing the number of basic operations (comparisons, assignments, etc.) performed by the algorithm.
20. Explain the concept of heuristic search algorithms in C++ and their application in solving complex problems.
Answer: Heuristic search algorithms use heuristic functions to guide the search towards solutions in complex problem-solving scenarios. They make educated guesses to find approximate solutions efficiently.
Advanced Searching Algorithms:
21. What is the A algorithm in C++ and how does it work in pathfinding and graph traversal?*
Answer: A* (A-star) is an informed search algorithm used for finding the shortest path in graphs. It combines the advantages of Dijkstra’s algorithm and greedy best-first search by using heuristics to estimate the cost to reach the target.
22. Explain the concept of breadth-first search (BFS) in C++ and its applications in graph traversal.
Answer: BFS is a graph traversal algorithm that explores all nodes at the current depth level before moving to the next level. It is used for tasks like finding the shortest path in unweighted graphs.
23. What is the depth-first search (DFS) algorithm in C++,, and how does it differ from BFS?
Answer: DFS is a graph traversal algorithm that explores as far as possible along a branch before backtracking. Unlike BFS, it does not necessarily find the shortest path.
24. Explain how the binary search tree (BST) data structure in C++ enables efficient searching, insertion, and deletion operations.
Answer: In a BST, data is organized such that elements smaller than the root are in the left subtree, and elements greater than the root are in the right subtree. This structure allows for efficient searching, insertion, and deletion operations.
25. What is the concept of a balanced BST in C++ and how does it ensure that the tree remains balanced?
Answer: A balanced BST, such as an AVL tree or a Red-Black tree, automatically maintains balance by performing rotations and color adjustments during insertions and deletions, ensuring that the tree remains relatively balanced.
26. Explain how hash tables in C++ handle collisions and why they are crucial for efficient searching.
Answer: Hash tables use techniques like chaining or open addressing to handle collisions when two keys hash to the same index. This ensures that elements can be efficiently searched and retrieved.
27. What is a trie (prefix tree) in C++,, and how is it used for efficient searching of strings or words?
Answer: A trie is a tree-like data structure used for storing a dynamic set of strings. It allows for efficient string searching, prefix matching, and autocomplete suggestions.
28. Explain the concept of skip lists in C++ and how they enable efficient searching in linked lists.
Answer: Skip lists are data structures that maintain multiple layers of linked lists. They allow for efficient searching in linked lists by “skipping” ahead to reduce the number of comparisons.
29. What is the Boyer-Moore string searching algorithm in C++ and how does it improve the efficiency of substring searches?
Answer: Boyer-Moore is a string searching algorithm that uses character comparisons and heuristics to skip portions of the text, leading to significant improvements in efficiency for substring searches.
30. Explain the concept of KMP (Knuth-Morris-Pratt) string searching algorithm in C++ and its advantages in substring searching.
Answer: The KMP algorithm uses a partial match table to avoid unnecessary character comparisons during substring searches, making it more efficient than simple linear approaches.
Time and Space Complexity Analysis:
31. What is the time complexity of linear search in C++ for an unsorted collection of “n” elements in the worst case?
Answer: The worst-case time complexity of linear search for an unsorted collection is O(n), where “n” is the number of elements.
32. What is the time complexity of binary search in C++ for a sorted collection of “n” elements in the worst case?
Answer: The worst-case time complexity of binary search for a sorted collection is O(log n), where “n” is the number of elements.
33. How does the efficiency of searching algorithms in C++ vary with different data structures, such as arrays, linked lists, and hash tables?
Answer: The efficiency of searching algorithms can vary based on the data structure. Arrays and sorted arrays are suitable for binary search, while hash tables excel in constant time retrieval. Linked lists may require linear search unless sorted.
34. What is the space complexity of various searching algorithms in C++ and how does it impact memory usage?
Answer: The space complexity of searching algorithms varies. Linear search and binary search have constant space complexity. Data structures like hash tables and tries have space complexity that depends on the number of elements and the structure.
35. Explain the trade-offs between time complexity and space complexity in searching algorithms in C++ and when to consider each aspect in practice.
Answer: In practice, the trade-offs between time and space complexity depend on factors such as available memory, dataset size, and retrieval frequency. Consider the specific requirements of the application to choose the most suitable searching algorithm.
Search Algorithm Applications:
36. How are searching algorithms in C++ used in information retrieval systems and search engines?
Answer: Searching algorithms play a vital role in information retrieval systems and search engines by quickly locating relevant documents or web pages based on user queries.
37. Explain how searching algorithms can be applied to solving the problem of spell checking and autocorrection in natural language processing (NLP) tasks.
Answer: Searching algorithms can be used to find the closest matching words in a dictionary for spell checking and autocorrection in NLP, improving text accuracy.
38. What is the role of searching algorithms in databases, and how are they used for efficient data retrieval?
Answer: Searching algorithms are used in databases to efficiently locate records that match specific criteria, improving data retrieval speed and query performance.
39. How do searching algorithms facilitate efficient searching in software applications, such as search bars in web applications or file search utilities?
Answer: Searching algorithms power search functionality in software applications, enabling users to quickly find relevant content or files based on search queries.
40. Explain the concept of pattern matching in searching algorithms in C++ and its applications in text processing and data analysis.
Answer: Pattern matching involves finding occurrences of a specified pattern within a text or dataset. It is used in text processing, data analysis, and regular expressions for tasks like string searching and data extraction.
Advanced Search Algorithms:
41. What is the concept of Ternary Search in C++, and how does it differ from binary search?
Answer: Ternary Search is a divide-and-conquer algorithm that splits the search space into three parts instead of two in binary search. While binary search divides the space in half, ternary search divides it into thirds.
42. Explain the concept of the Rabin-Karp algorithm in C++ for string searching, and what are its advantages?
Answer: The Rabin-Karp algorithm uses hashing to efficiently search for a substring in a text. Its advantage is that it can achieve linear time complexity on average when using a good hash function.
43. What is the concept of a bloom filter in C++ and its role in probabilistic searching?
Answer: A bloom filter is a data structure used to test whether an element is a member of a set. It may return false positives but never false negatives, making it useful for efficient membership testing.
44. Explain the concept of binary search on a rotated sorted array in C++ and how it handles the rotated scenario.
Answer: Binary search on a rotated sorted array first identifies the pivot point (the point where rotation occurs) and then performs binary search in the appropriate half. It handles the rotated scenario by adjusting the search boundaries.
45. What is the Levenshtein distance in C++,,, and how is it used in searching algorithms, particularly in spell checking and string comparison?
Answer: The Levenshtein distance measures the minimum number of operations (insertions, deletions, substitutions) required to transform one string into another. It is used in spell checking, DNA sequence alignment, and similarity measurement.
Time and Space Complexity Analysis:
46. Explain the trade-off between time complexity and space complexity in searching algorithms in C++ and how it influences algorithm selection.
Answer: The trade-off involves choosing algorithms that strike a balance between time and space complexity based on available memory and dataset size. Algorithms that use more memory may be faster but require additional space.
47. What is the time complexity of the Boyer-Moore string searching algorithm in C++ and how does it compare to other string searching algorithms?
Answer: The Boyer-Moore algorithm typically has an average-case time complexity of O(n/m) for searching a pattern of length “m” in a text of length “n,” making it one of the most efficient string searching algorithms.
48. How does the efficiency of searching algorithms in C++ vary when searching for exact matches compared to approximate or fuzzy matches?
Answer: Searching for exact matches is typically more efficient, as it relies on exact comparisons. Approximate or fuzzy matching algorithms, such as edit distance-based searches, involve more complex computations.
49. Explain the space complexity considerations when implementing search algorithms in C++ for resource-constrained environments or embedded systems.
Answer: In resource-constrained environments, it is essential to choose algorithms that minimize memory usage, potentially favoring linear search or optimized data structures with lower space overhead.
50. What are some practical scenarios where multiple search algorithms in C++ might be used in combination to achieve better search performance?
Answer: Multiple search algorithms can be combined in scenarios where different algorithms excel in different aspects, such as using a hash table for quick lookups and a trie for prefix matching in a search engine.
These questions and answers provide a comprehensive overview of searching algorithms in C++, covering various algorithms, data structures, complexities, and applications. Understanding these concepts will help you prepare for interviews and gain a deeper knowledge of searching algorithms and their use in programming and problem-solving.