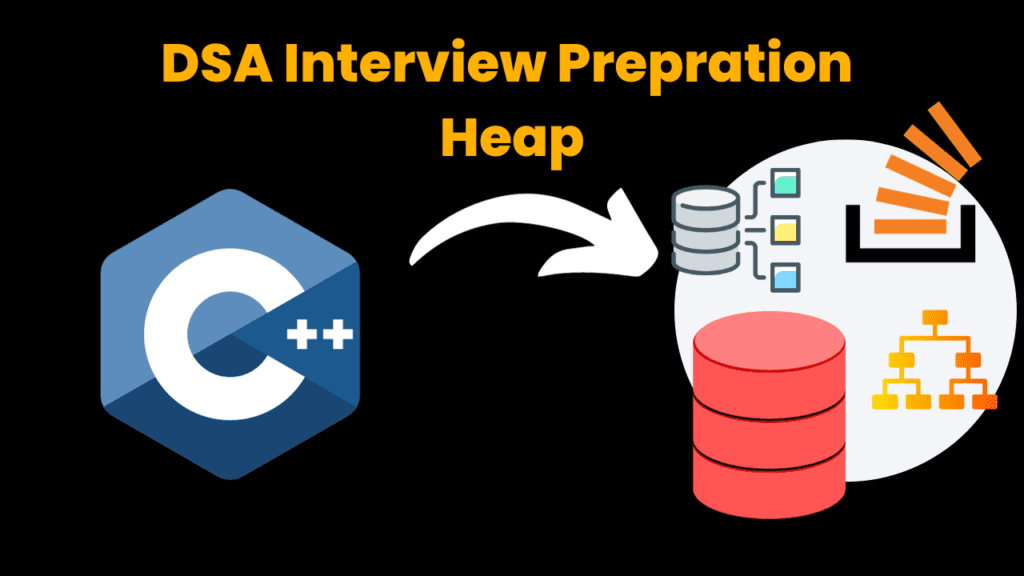
50 most frequently asked Heaps interview questions.
Basics of Heaps:
1.What is a heap data structure in C++?
Answer: A heap is a binary tree-based data structure that satisfies the heap property, where the value of each node is greater than or equal to (in a max-heap) or less than or equal to (in a min-heap) the values of its children.
2. Differentiate between a max-heap and a min-heap in C++.
Answer: In a max-heap, the value of each node is greater than or equal to the values of its children. In a min-heap, the value of each node is less than or equal to the values of its children.
3. How is a heap typically implemented in C++?
Answer: Heaps are commonly implemented as binary trees, with arrays used to represent the tree structure. The array elements are arranged so that the heap properties are maintained.
4. What is the root node of a heap in C++?
Answer: The root node is the topmost node of the heap, which contains the maximum value (in a max-heap) or the minimum value (in a min-heap).
5. What is the height of a heap in C++, and how is it related to the number of nodes?
Answer: The height of a heap is the maximum number of edges in a path from the root to a leaf node. It’s related to the number of nodes by the formula height = log2(n)
, where n
is the number of nodes.
Heap Operations:
6. How do you insert an element into a heap in C++ while maintaining the heap property?
Answer: To insert an element, add it to the next available position in the heap and then use a process called “heapify” (either up-heapify for min-heaps or down-heapify for max-heaps) to maintain the heap property.
7. Explain the process of removing the maximum (or minimum) element from a heap in C++.
Answer: To remove the maximum (or minimum) element, swap it with the last leaf node, remove the leaf node, and then use down-heapify (for max-heaps) or up-heapify (for min-heaps) to restore the heap property.
8. What is the time complexity of inserting an element into a heap in C++?
Answer: The time complexity of inserting an element into a heap is O(log n), where “n” is the number of elements in the heap.
9. What is the time complexity of extracting the maximum (or minimum) element from a heap in C++?
Answer: The time complexity of extracting the maximum (or minimum) element from a heap is O(log n), where “n” is the number of elements in the heap.
10. Explain the concept of heapify in C++ and its role in maintaining the heap property.
Answer: Heapify is a process used to maintain the heap property. It involves recursively swapping elements to ensure that the parent node’s value is greater than or equal to (in a max-heap) or less than or equal to (in a min-heap) its children’s values.
Heap Applications:
11. What are some common applications of heaps in C++?
Answer: Heaps are used in various applications, such as priority queues, heap sort, finding the kth largest (or smallest) element in an array, and graph algorithms like Dijkstra’s shortest path.
12. Explain how heaps are used to implement a priority queue in C++.
Answer: A priority queue is implemented using a heap, where the element with the highest priority (minimum or maximum, depending on the type of heap) is always at the root. This allows for efficient insertion and removal of elements with the highest priority.
13. How can heaps be utilized to perform heap sort in C++?
Answer: Heap sort involves building a max-heap, repeatedly extracting the maximum element, and placing it in the sorted portion of the array. It uses the heap property to sort the elements efficiently.
14. Explain the role of heaps in finding the kth largest (or smallest) element in an array in C++.
Answer: Heaps can be used to find the kth largest (or smallest) element in an array by maintaining a heap of the k largest (or smallest) elements encountered while iterating through the array.
15. How are heaps used in graph algorithms like Dijkstra’s shortest path algorithm in C++?
Answer: In Dijkstra’s algorithm, a min-heap is used to efficiently select the vertex with the shortest distance as the next vertex to explore. This ensures that the shortest path is found.
Heap Variations:
16. Explain the concept of a binary heap in C++, and how does it differ from other types of heaps?
Answer: A binary heap is a type of heap where each parent has at most two children. It is commonly used due to its simplicity and efficiency.
17. What is a Fibonacci heap in C++,?
Answer: A Fibonacci heap is a type of heap that supports certain operations like decrease key and merge in constant or amortized constant time. It is used in advanced algorithms like Dijkstra’s algorithm with better time complexity.
18. What is a binomial heap in C++,?
Answer: A binomial heap is a type of heap that is a collection of binomial trees, each of which satisfies the heap property. Binomial heaps are used in some graph algorithms.
19. Explain the concept of a d-ary heap in C++, and how does it differ from a binary heap?
Answer: A d-ary heap is a type of heap where each parent has at most d children. It differs from a binary heap in that it has more children per node.
20. What is a leftist heap in C++,,, and what makes it “leftist”?
Answer: A leftist heap is a type of heap that satisfies the leftist property, where the right child’s rank is less than or equal to the left child’s rank. This property allows for efficient merging of leftist heaps.
Advanced Heap Concepts:
21. Explain the concept of a skew heap in C++, and how does it differ from other types of heaps?
Answer: A skew heap is a type of heap that is a self-adjusting binary tree. It differs from other heaps in that it does not guarantee the balance of subtrees but still maintains the heap property.
22. What is the concept of a pairing heap in C++,?
Answer: A pairing heap is a type of heap that uses a simplified structure to achieve good time complexity for most operations, including insertions, deletions, and merging.
23. What are some techniques for optimizing heap operations, especially in advanced heap variations in C++?
Answer: Techniques include lazy merging, decrease key optimization, and simplifications in heap variations like Fibonacci heaps and skew heaps.
24. Explain the concept of a Brodal queue in C++, and what makes it unique among heap variations.
Answer: A Brodal queue is a self-adjusting priority queue that uses a mix of different heap types to optimize various operations. It combines the strengths of different heap structures.
25. What are some potential drawbacks and trade-offs of using advanced heap variations like Fibonacci heaps or Brodal queues in C++?
Answer: Advanced heap variations often have complex implementations and may require more memory than traditional heaps. While they offer improved time complexity for certain operations, their practical advantages may vary depending on the specific use case.
Heap Operations and Use Cases:
26. What is the main advantage of using a max-heap over a sorted array for finding the maximum element in C++?
Answer: Max-heaps provide a faster way to find the maximum element compared to a sorted array, which requires linear time for searching.
27. Explain how heaps can be used to efficiently merge multiple sorted arrays in C++.
Answer: To merge multiple sorted arrays, elements can be inserted into a min-heap, and the minimum element can be repeatedly extracted, ensuring an efficient merge in O(n log k) time, where n is the total number of elements and k is the number of arrays.
28. What is the “heap property” in C++,,, and why is it important in maintaining the correctness of a heap?
Answer: The heap property ensures that the values of parent nodes are greater than or equal to (in a max-heap) or less than or equal to (in a min-heap) the values of their children. It’s crucial for maintaining the heap’s correct structure and ordering.
29. Explain how heaps can be used to efficiently implement a median of streaming integers in C++.
Answer: Two heaps (a max-heap for the lower half and a min-heap for the upper half) can be used to efficiently calculate the median as new elements arrive, ensuring O(log n) time complexity.
30. How do heaps contribute to efficient implementations of algorithms like Prim’s and Kruskal’s for finding minimum spanning trees in C++?
Answer: Heaps are used to select the next edge with the minimum weight in algorithms like Prim’s and Kruskal’s, making them efficient for finding minimum spanning trees.
Heap Variations and Implementations:
31. Explain the concept of a 2-3 heap in C++, and what distinguishes it from other heap types.
Answer: A 2-3 heap is a type of heap that allows nodes to have two or three children. It is a variation of d-ary heaps and has a more balanced structure.
32. What is a soft heap in C++?
Answer: A soft heap is a type of heap that allows for approximate solutions to some heap operations, sacrificing precision for improved performance.
33. Explain the concept of a leftist tree in C++, and what makes it “leftist.”
Answer: A leftist tree is a binary tree that satisfies the leftist property, where the right child’s rank is less than or equal to the left child’s rank. This property allows for efficient merging of leftist trees.
34. What is a binomial queue in C++,?
Answer: A binomial queue is a type of heap that is a collection of binomial trees, each of which satisfies the heap property. Binomial queues support efficient insertion, merging, and extraction of the minimum element.
35. What are skew heaps in C++, and how are they different from other heap structures?
Answer: Skew heaps are self-adjusting binary trees where no strict structural constraints are enforced. Their primary difference is their simplicity and the lack of strict balancing rules.
Advanced Heap Concepts:
36. Explain the concept of pairing heaps in C++, and how do they achieve improved efficiency?
Answer: Pairing heaps are self-adjusting heaps that use a more complex structure to achieve better average-case time complexity for most operations compared to traditional binary heaps.
37. What are some use cases where Fibonacci heaps in C++ can outperform other heap structures?
Answer: Fibonacci heaps are advantageous in algorithms that require efficient decrease key and merge operations, such as Dijkstra’s algorithm and certain graph algorithms.
38. What is the role of heap order statistics in C++ in finding the kth largest (or smallest) element in a heap efficiently?
Answer: Heap order statistics provide a way to efficiently find the kth largest (or smallest) element in a heap by maintaining additional information about the number of elements in subtrees.
39. How do Brodal queues in C++ differ from other heap structures, and what are their advantages?
Answer: Brodal queues combine different heap structures for optimized operations, making them suitable for various scenarios. They adapt dynamically to the data distribution, improving efficiency.
40. What are some practical considerations and trade-offs when choosing a heap structure for a specific application in C++?
Answer: Practical considerations include the complexity of implementation, memory usage, and the specific requirements of the application. Trade-offs involve balancing time and space efficiency.
41. Question: What is a binary heap?
Answer: A binary heap is a complete binary tree that satisfies the heap property. In a binary min-heap, the value of each node is less than or equal to the values of its children.
42. Question: How can you efficiently implement a priority queue in C++ using a binary heap?
Answer: A binary min-heap can be used to implement a priority queue efficiently. The minimum element is always at the root, allowing for constant-time retrieval and logarithmic time insertion and removal.
43. Question: What is the time complexity of building a binary heap from an array of elements in C++?
Answer: Building a binary heap from an array of n elements takes O(n) time using a bottom-up approach called “heapify.”
44. Question: Explain the concept of a d-ary heap in C++. How does it compare to a binary heap?
Answer: A d-ary heap is a heap where each parent has at most d children. It is a generalization of a binary heap, offering a trade-off between the number of children and tree height.
45. Question: What is a k-ary heap in C++?
Answer: A k-ary heap is a heap where each parent has exactly k children. It is a specific type of d-ary heap where d equals k.
46. Question: How can you efficiently sort an array using a binary heap in C++ (Heap Sort)?
Answer: Heap Sort involves building a max-heap from the array and repeatedly removing the maximum element (root) and placing it at the end of the array until the array is sorted. It has a time complexity of O(n log n).
47. Question: Explain the concept of a Fibonacci heap in C++. What is its main advantage over other heaps?
Answer: A Fibonacci heap is a type of heap that offers amortized constant-time operations for decrease key and efficient merging. Its main advantage is the improved time complexity for specific algorithms like Dijkstra’s.
48. Question: How does a skew heap differ from other heap structures in C++?
Answer: A skew heap is a self-adjusting binary tree that does not enforce strict structural rules. It differs from other heaps in its simplicity and lack of balancing constraints.
49. Question: What is lazy merging in the context of heap data structures in C++?
Answer: Lazy merging is a technique where merging of heaps is deferred until necessary. This can help improve the efficiency of some operations in certain heap variations.
50. Question: In a max-heap, what is the largest element after extracting the maximum element?
Answer: After extracting the maximum element from a max-heap, the next largest element becomes the new root of the heap, and the heap property is restored through down-heapify operations.