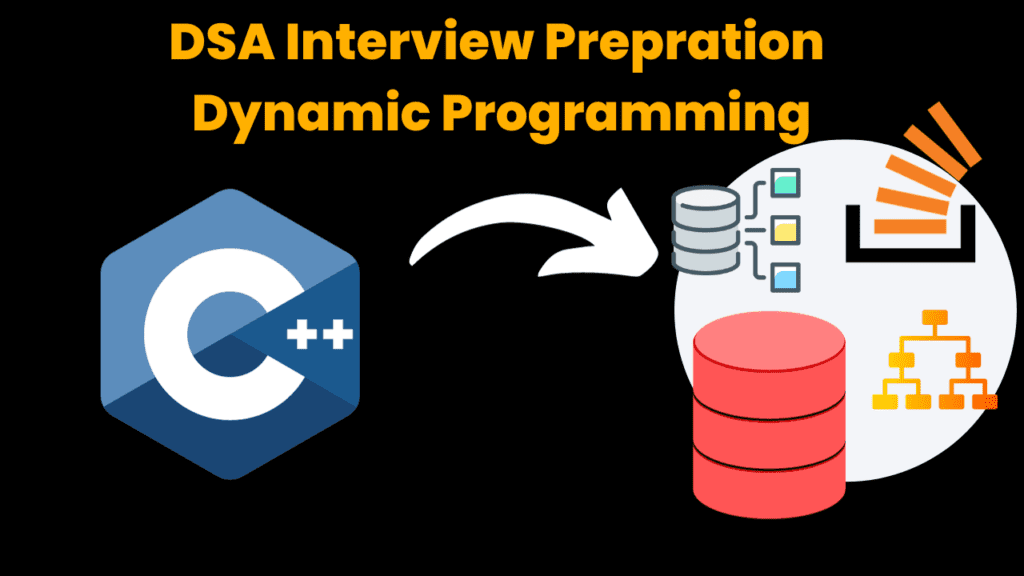
50 most frequently asked Dynamic Programming interview questions.
Basics of Dynamic Programming:
1. What is Dynamic Programming (DP) in C++ and how does it differ from traditional brute-force approaches?
Answer: Dynamic Programming is a technique used to solve problems by breaking them into smaller subproblems and storing their solutions to avoid redundant calculations. It optimizes time complexity compared to brute-force methods.
2. Explain the concept of memoization in C++ and its role in DP.
Answer: Memoization is a technique in DP where the results of subproblems are stored in a cache (usually an array or a map) to avoid redundant computations, improving efficiency.
3. What is the difference between top-down and bottom-up DP approaches in C++?
Answer: In the top-down approach, the problem is solved recursively from the top, often using memoization. In the bottom-up approach, subproblems are solved iteratively, starting from the base cases and building up to the final solution.
4. What is the “optimal substructure” property in C++,, and why is it important in DP problems?
Answer: Optimal substructure means that the optimal solution of a problem can be constructed from the optimal solutions of its subproblems. This property is essential in DP because it allows problems to be broken down into smaller, manageable parts.
5. Explain the concept of “overlapping subproblems” in C++ DP problems, and how does DP address this issue?
Answer: Overlapping subproblems occur when the same subproblem is solved multiple times in a recursive algorithm. DP addresses this issue by storing the results of subproblems in a cache, preventing redundant computations.
Fibonacci Sequence and Factorial:
6. Provide a C++ code example of calculating the nth Fibonacci number using the recursive approach.
Answer:
int fibonacciRecursive(int n) {
if (n <= 1) {
return n;
}
return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2);
}
7. What are the time and space complexities of the recursive Fibonacci calculation in C++?
Answer: The time complexity is O(2^n) due to redundant calculations, and the space complexity is O(n) due to the function call stack.
8. Explain how dynamic programming can optimize the calculation of Fibonacci numbers in C++.
Answer: DP can optimize Fibonacci calculations by storing previously computed values and reusing them, reducing time complexity to O(n) and space complexity to O(1).
9. Provide a C++ code example of calculating the factorial of a number using dynamic programming.
Answer:
int factorialDP(int n) {
int dp[n + 1];
dp[0] = 1;
for (int i = 1; i <= n; i++) {
dp[i] = i * dp[i - 1];
}
return dp[n];
}
10. What are the time and space complexities of the dynamic programming factorial calculation in C++?
Answer: The time complexity is O(n), and the space complexity is O(n) due to the array used for memoization.
Longest Common Subsequence (LCS):
11. Explain the Longest Common Subsequence (LCS) problem in C++, and provide an example.
Answer: The LCS problem involves finding the longest subsequence common to two given sequences. For example, for sequences “ABCBDAB” and “BDCAB,” the LCS is “BCAB.”
12. What is the brute-force approach to solving the LCS problem in C++, and what are its limitations?
Answer: The brute-force approach involves generating all possible subsequences of both input sequences and finding their common subsequences. It is inefficient for long sequences due to its exponential time complexity.
13. Explain how dynamic programming can be used to solve the LCS problem in C++ efficiently.
Answer: DP stores the length of the LCS for various subproblems in a 2D array. By building the array iteratively, the final LCS can be reconstructed.
14. Provide a C++ code example of solving the LCS problem using dynamic programming.
Answer:
int longestCommonSubsequence(string text1, string text2) {
int m = text1.length();
int n = text2.length();
vector> dp(m + 1, vector(n + 1, 0));
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (text1[i - 1] == text2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
return dp[m][n];
}
15. What is the time and space complexity of the dynamic programming LCS algorithm in C++?
Answer: The time complexity is O(m * n), where m and n are the lengths of the input sequences, and the space complexity is O(m * n) due to the 2D array used for memoization.
Knapsack Problem:
16. Explain the 0/1 Knapsack problem in C++ and its applications in optimization.
Answer: The 0/1 Knapsack problem involves selecting a combination of items with given weights and values to maximize the total value while not exceeding a fixed weight capacity. It has applications in resource allocation, project scheduling, and more.
17. What is the brute-force approach to solving the 0/1 Knapsack problem in C++,, and why is it inefficient for large instances?
Answer: The brute-force approach involves trying all possible combinations of items. It is inefficient for large instances because the number of combinations grows exponentially with the number of items.
18. How can dynamic programming be applied to solve the 0/1 Knapsack problem in C++ efficiently?
Answer: DP stores the maximum value achievable for various subproblems in a 2D array. By iteratively filling the array, the solution can be obtained.
19. Provide a C++ code example of solving the 0/1 Knapsack problem using dynamic programming.
Answer:
int knapsackDP(int capacity, vector& weights, vector& values) {
int n = weights.size();
vector> dp(n + 1, vector(capacity + 1, 0));
for (int i = 1; i <= n; i++) {
for (int w = 1; w <= capacity; w++) {
if (weights[i - 1] <= w) {
dp[i][w] =
Knapsack Problem (Continued):
20.What is the time and space complexity of the dynamic programming solution to the 0/1 Knapsack problem in C++?
Answer: The time complexity is O(n * capacity), where “n” is the number of items, and “capacity” is the maximum weight capacity. The space complexity is O(n * capacity) due to the 2D array used for memoization.
Coin Change Problem:
21. Explain the Coin Change problem in C++ and its applications in making change with a minimum number of coins.
Answer: The Coin Change problem involves finding the minimum number of coins needed to make a given amount of money using a given set of coin denominations. It has applications in financial transactions and vending machines.
22. What is the brute-force approach to solving the Coin Change problem in C++,, and why is it inefficient for certain scenarios?
Answer: The brute-force approach involves trying all possible combinations of coins. It is inefficient for scenarios where there are many coin denominations or large target amounts due to the exponential growth in possibilities.
23. How can dynamic programming be used to efficiently solve the Coin Change problem in C++?
Answer: DP stores the minimum number of coins required for various subproblems in an array. By iteratively filling the array, the solution can be obtained efficiently.
24. Provide a C++ code example of solving the Coin Change problem using dynamic programming.
Answer:
int coinChangeDP(vector& coins, int amount) {
vector dp(amount + 1, INT_MAX);
dp[0] = 0;
for (int i = 1; i <= amount; i++) {
for (int coin : coins) {
if (coin <= i && dp[i - coin] != INT_MAX) {
dp[i] = min(dp[i], dp[i - coin] + 1);
}
}
}
return dp[amount] == INT_MAX ? -1 : dp[amount];
}
25. What is the time and space complexity of the dynamic programming solution to the Coin Change problem in C++?
Answer: The time complexity is O(amount * n), where “amount” is the target amount and “n” is the number of coin denominations. The space complexity is O(amount) due to the array used for memoization.
Longest Increasing Subsequence (LIS):
26. Explain the Longest Increasing Subsequence (LIS) problem in C++ and its applications in sequence analysis and optimization.
Answer: The LIS problem involves finding the longest subsequence of a given sequence such that elements are in increasing order. It has applications in data analysis, stock trading, and more.
27. What is the brute-force approach to solving the LIS problem in C++,, and why is it inefficient for long sequences?
Answer: The brute-force approach involves generating all possible subsequences and finding the longest increasing one. It is inefficient for long sequences due to exponential time complexity.
28. How can dynamic programming be applied to solve the LIS problem in C++ efficiently?
Answer: DP stores the length of the LIS ending at each position in the sequence. By iteratively filling the array, the maximum length can be determined.
29. Provide a C++ code example of solving the LIS problem using dynamic programming.
Answer:
int lengthOfLIS(vector& nums) {
int n = nums.size();
vector dp(n, 1);
for (int i = 1; i < n; i++) {
for (int j = 0; j < i; j++) {
if (nums[i] > nums[j]) {
dp[i] = max(dp[i], dp[j] + 1);
}
}
}
return *max_element(dp.begin(), dp.end());
}
30. What is the time and space complexity of the dynamic programming solution to the LIS problem in C++?
Answer: The time complexity is O(n^2), where “n” is the length of the input sequence. The space complexity is O(n) due to the array used for memoization.
Edit Distance (Levenshtein Distance):
31. Explain the Edit Distance (Levenshtein Distance) problem in C++, and its applications in spell checking and string similarity measurement.
Answer: The Edit Distance problem involves finding the minimum number of edit operations (insertions, deletions, substitutions) required to transform one string into another. It is used in spell checking, DNA sequence alignment, and text correction.
32. What is the brute-force approach to solving the Edit Distance problem in C++, and why is it inefficient for long strings?
Answer: The brute-force approach involves trying all possible edit operations recursively. It is inefficient for long strings due to its exponential time complexity.
33. How can dynamic programming be used to efficiently solve the Edit Distance problem in C++?
Answer: DP stores the minimum number of edit operations required for various subproblems in a 2D array. By iteratively filling the array, the minimum edit distance can be determined.
34. Provide a C++ code example of solving the Edit Distance problem using dynamic programming.
Answer:
int minDistance(string word1, string word2) {
int m = word1.length();
int n = word2.length();
vector> dp(m + 1, vector(n + 1, 0));
for (int i = 0; i <= m; i++) {
for (int j = 0; j <= n; j++) {
if (i == 0) {
dp[i][j] = j;
} else if (j == 0) {
dp[i][j] = i;
} else if (word1[i - 1] == word2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1];
} else {
dp[i][j] = 1 + min({dp[i - 1][j], dp[i][j - 1], dp[i - 1][j - 1]});
}
}
}
return dp[m][n];
}
35. What is the time and space complexity of the dynamic programming solution to the Edit Distance problem in C++?
Answer: The time complexity is O(m * n), where “m” and “n” are the lengths of the input strings. The space complexity is O(m * n) due to the 2D array used for memoization.
Matrix Chain Multiplication:
36. Explain the Matrix Chain Multiplication problem in C++, and its applications in optimization and algorithm design.
Answer: The Matrix Chain Multiplication problem involves finding the most efficient way to multiply a sequence of matrices to minimize the total number of multiplications. It is used in computer graphics, numerical simulations, and algorithm design.
37. What is the brute-force approach to solving the Matrix Chain Multiplication problem in C++, and why is it inefficient for a large number of matrices?
Answer: The brute-force approach involves trying all possible parenthetical arrangements. It is inefficient for a large number of matrices due to its factorial time complexity.
38. How can dynamic programming be used to efficiently solve the Matrix Chain Multiplication problem in C++?
Answer: DP stores the minimum number of scalar multiplications required for various subproblems in a 2D array. By iteratively filling the array, the optimal arrangement can be determined.
39. Provide a C++ code example of solving the Matrix Chain Multiplication problem using dynamic programming.
Answer:
int matrixChainOrder(vector& dimensions) {
int n = dimensions.size() - 1;
vector> dp(n, vector(n, 0));
for (int len = 2; len <= n; len++) {
for (int i = 0; i <= n - len + 1; i++) {
int j = i + len - 1;
dp[i][j] = INT_MAX;
for (int k = i; k < j; k++) {
int cost = dp[i][k] + dp[k + 1][j] + dimensions[i] * dimensions[k + 1] * dimensions[j + 1];
dp[i][j] = min(dp[i][j], cost);
}
}
}
return dp[0][n - 1];
}
40. What is the time and space complexity of the dynamic programming solution to the Matrix Chain Multiplication problem in C++?
Answer: The time complexity is O(n^3), where “n” is the number of matrices. The space complexity is O(n^2) due to the 2D array used for memoization.
Longest Palindromic Subsequence (LPS):
41. Explain the Longest Palindromic Subsequence (LPS) problem in C++, and its applications in text processing and genetics.
Answer: The LPS problem involves finding the longest subsequence of a given sequence that is a palindrome. It has applications in text compression, DNA sequence analysis, and error correction.
42. What is the brute-force approach to solving the LPS problem in C++, and why is it inefficient for long sequences?
Answer: The brute-force approach involves generating all possible subsequences and checking if they are palindromes. It is inefficient for long sequences due to its exponential time complexity.
43. How can dynamic programming be applied to efficiently solve the LPS problem in C++?
Answer: DP stores the length of the LPS for various subproblems in a 2D array. By iteratively filling the array, the maximum length can be determined.
44. Provide a C++ code example of solving the LPS problem using dynamic programming.
Answer:
int longestPalindromeSubseq(string s) {
int n = s.length();
vector> dp(n, vector(n, 0));
for (int i = n - 1; i >= 0; i--) {
dp[i][i] = 1;
for (int j = i + 1; j < n; j++) {
if (s[i] == s[j]) {
dp[i][j] = 2 + dp[i + 1][j - 1];
} else {
dp[i][j] = max(dp[i + 1][j], dp[i][j - 1]);
}
}
}
return dp[0][n - 1];
}
45. What is the time and space complexity of the dynamic programming solution to the LPS problem in C++?
Answer: The time complexity is O(n^2), where “n” is the length of the input sequence. The space complexity is O(n^2) due to the 2D array used for memoization.
Subset Sum:
46. Explain the Subset Sum problem in C++, and its applications in resource allocation and optimization.
Answer: The Subset Sum problem involves finding whether there exists a subset of a given set of numbers that adds up to a specific target sum. It has applications in resource allocation, budget planning, and decision-making.
47. What is the brute-force approach to solving the Subset Sum problem in C++, and why is it inefficient for large sets of numbers?
Answer: The brute-force approach involves trying all possible subsets. It is inefficient for large sets of numbers due to the exponential number of subsets to consider.
48. How can dynamic programming be used to efficiently solve the Subset Sum problem in C++?
Answer: DP stores whether a given target sum can be achieved using a subset of numbers. By iteratively filling the array, the solution can be determined.
49. Provide a C++ code example of solving the Subset Sum problem using dynamic programming.
Answer:
bool canPartition(vector& nums) {
int sum = accumulate(nums.begin(), nums.end(), 0);
if (sum % 2 != 0) {
return false; // If the sum is odd, cannot partition into equal subsets.
}
int target = sum / 2;
vector dp(target + 1, false);
dp[0] = true;
for (int num : nums) {
for (int j = target; j >= num; j--) {
dp[j] = dp[j] || dp[j - num];
}
}
return dp[target];
}
50. What is the time and space complexity of the dynamic programming solution to the Subset Sum problem in C++?
Answer: The time complexity is O(n * target), where “n” is the number of elements in the input set, and “target” is the target sum. The space complexity is O(target) due to the array used for memoization.
These questions and answers provide a comprehensive understanding of Dynamic Programming in C++, covering fundamental concepts, common problems, and their efficient solutions. Understanding these concepts will help you excel in interviews and gain proficiency in using DP to solve complex problems.