Python Object-Oriented Programming Interview Questions
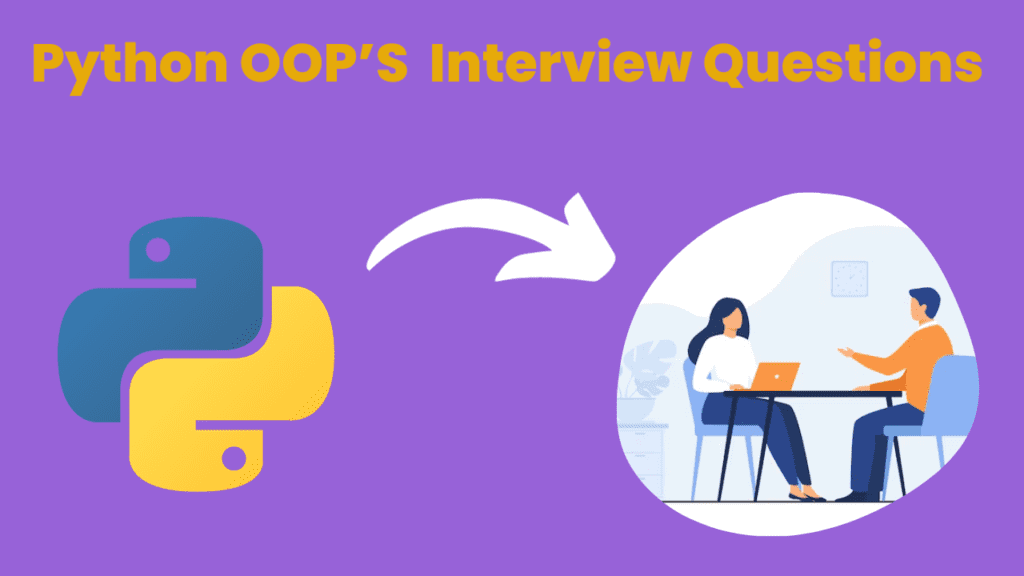
50 most frequently asked Python Object-Oriented Programming interview questions.
Get Discount on Top Educational Courses
1. What is OOP, and why is it important?
Answer: OOP (Object-Oriented Programming) is a programming paradigm that uses objects to structure and organize code. It is important because it promotes modularity, reusability, and a more intuitive way of representing real-world concepts in code.
2.Explain the concepts of classes and objects in Python.
Answer: A class in Python is a blueprint or template for creating objects. Objects are instances of classes and represent real-world entities. Classes define attributes (properties) and methods (functions) that objects of that class will have.
3.What is inheritance, and how is it implemented in Python?
Answer: Inheritance is a mechanism in OOP that allows a new class (subclass or derived class) to inherit properties and behaviors from an existing class (superclass or base class). In Python, you can implement inheritance by defining a subclass that inherits from a superclass using the class Subclass(Superclass):
syntax.
4.Discuss polymorphism and encapsulation in Python.
Answer: Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables method overriding and dynamic method dispatch. Encapsulation is the practice of hiding the internal implementation details of an object and providing a public interface to interact with it, achieved through private attributes and methods.
5.Explain the difference between instance and class variables.
Answer: Instance variables (also called instance attributes) are specific to each object created from a class. They are defined inside methods and can vary from object to object. Class variables (also called class attributes) are shared among all objects of the class and are defined at the class level.
6.What is a constructor in Python, and how is it defined?
Answer: A constructor is a special method in a class used to initialize its objects. In Python, the constructor is defined as __init__(self, parameters)
within the class. It is automatically called when an object is created.
7.How do you create an instance of a class in Python?
Answer: You create an instance of a class by calling the class as if it were a function. For example, to create an instance of class MyClass
, you write obj = MyClass()
.
8.What is a method in Python, and how is it different from a function?
Answer: A method is a function defined within a class and is associated with objects of that class. Functions are standalone and not tied to a specific class.
9.What is composition in OOP, and how is it implemented in Python?
Answer: Composition is a design principle where a class contains objects of other classes as parts. It is implemented by creating instances of other classes within a class and using them to provide functionality.
10.What is an abstract class in Python, and how is it defined?
Answer: An abstract class is a class that cannot be instantiated and is meant to be subclassed. It can have abstract methods (methods without implementation) that must be implemented by its subclasses. Abstract classes are defined using the abc
module and the @abstractmethod
decorator.
11.Explain the purpose of method overloading and method overriding in Python.
Answer: Method overloading allows a class to define multiple methods with the same name but different parameter lists. Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass.
12.What is multiple inheritance, and how is it implemented in Python?
Answer: Multiple inheritance is a feature that allows a class to inherit properties and behaviors from more than one superclass. In Python, you can implement multiple inheritance by specifying multiple base classes in the class definition, like class Subclass(Base1, Base2):
.
13.What are access modifiers (public, private, protected), and how are they used in Python?
Answer: Access modifiers control the visibility and accessibility of class members. In Python, there is no strict enforcement of access modifiers, but you can use naming conventions to indicate visibility. _
prefix indicates “protected,” and __
prefix indicates “private.”
14.What is a destructor in Python, and how is it defined?
Answer: A destructor is a special method used to clean up resources when an object is no longer needed. In Python, the destructor is defined as __del__(self)
within the class.
15.Explain the concept of method chaining in Python.
Answer: Method chaining is a technique where multiple methods are invoked on an object one after the other, and each method returns the object itself (self
). This allows for more concise and readable code.
16.What is a static method in Python, and how is it defined?
Answer: A static method is a method that is bound to the class and not to an instance of the class. It is defined using the @staticmethod
decorator and does not have access to instance-specific data.
17. What is the purpose of the super()
function in Python?
Answer: The super()
function is used to call a method from the superclass within a subclass. It is often used in method overriding to invoke the superclass’s implementation before adding additional functionality.
18. How can you prevent a class from being subclassed in Python?
Answer: You can prevent a class from being subclassed by defining it with the final
keyword or by using the __final__()
method.
19. What is method resolution order (MRO) in Python, and how is it determined?
Answer: MRO is the order in which Python looks for a method or attribute in a class hierarchy. It is determined using the C3 linearization algorithm and can be viewed using the mro()
method or the __mro__
attribute.
20. Explain the purpose of the isinstance()
and issubclass()
functions in Python.
Answer: isinstance()
is used to check if an object is an instance of a class or a tuple of classes. issubclass()
is used to check if a class is a subclass of a specified class or a tuple of classes.
21. What is method resolution order (MRO) in Python, and how is it determined?
Answer: MRO is the order in which Python looks for a method or attribute in a class hierarchy. It is determined using the C3 linearization algorithm and can be viewed using the mro()
method or the __mro__
attribute.
22. Explain the purpose of the isinstance()
and issubclass()
functions in Python.
Answer: isinstance()
is used to check if an object is an instance of a class or a tuple of classes. issubclass()
is used to check if a class is a subclass of a specified class or a tuple of classes.
23. What is a metaclass in Python, and how is it used?
Answer: A metaclass is a class that defines the behavior of other classes (class factories). It can be used to customize class creation and control class attributes and methods.
24. Explain the concept of method overloading in Python.
Answer: Method overloading refers to defining multiple methods in a class with the same name but different parameter lists. Python does not support method overloading by the number or type of arguments, but you can achieve similar behavior using default arguments and variable-length arguments.
25. What is a class attribute, and how is it different from an instance attribute?
Answer: A class attribute is a variable that is shared among all instances of a class. It is defined at the class level and can be accessed using the class name or any instance. An instance attribute is specific to a particular object and is defined within the constructor method (__init__()
).
26. How do you create a private method in Python, and what is its visibility?
Answer: In Python, there is no strict concept of private methods, but you can indicate a method as private by prefixing its name with a double underscore (__
). However, it is still accessible, but its name is “mangled” to include the class name, making it less visible.
27. What is method overloading in Python, and how is it achieved?
Answer: Method overloading refers to defining multiple methods with the same name in a class, but with different parameters. In Python, you can achieve method overloading by using default argument values or variable-length arguments (*args
and **kwargs
).
28. Explain the concept of method overriding in Python.
Answer: Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. The overridden method in the subclass has the same name and parameters as the method in the superclass.
29. What is the purpose of the @classmethod
decorator in Python?
Answer: The @classmethod
decorator is used to define a class method in Python. Class methods are bound to the class and not the instance, and they can be called on the class itself. They are often used to create alternative constructors for a class.
30. What is a factory method in Python, and how is it implemented?
Answer: A factory method is a method used to create and return instances of a class. It is often used to encapsulate the object creation process. Factory methods are typically defined as class methods and can be used to create objects of that class or its subclasses.
31. What is the purpose of the @property
decorator in Python, and how is it used?
Answer: The @property
decorator is used to define a method as a “getter” for a class attribute. It allows you to access the attribute as if it were an instance variable while providing control over its retrieval.
32. Explain the concept of multiple inheritance in Python.
Answer: Multiple inheritance is a feature that allows a class to inherit properties and behaviors from more than one superclass. In Python, it is implemented by specifying multiple base classes in the class definition using parentheses.
33. What is the diamond problem in the context of multiple inheritance, and how is it resolved in Python?
Answer: The diamond problem occurs when a class inherits from two classes that have a common ancestor. In Python, the C3 linearization algorithm is used to determine the method resolution order (MRO) and avoid ambiguity.
34. What is a mixin class in Python, and how is it used?
Answer: A mixin class is a class that provides additional functionality to other classes through multiple inheritance. It is not meant to be instantiated on its own but is intended to be combined with other classes to extend their behavior.
35. Explain the concept of operator overloading in Python.
Answer: Operator overloading allows you to define how operators (e.g., +
, -
, *
) behave when applied to instances of a class. It is achieved by defining special methods (e.g., __add__()
, __sub__()
) within the class.
36. What is method chaining in Python, and how is it useful?
Answer: Method chaining is a technique where multiple methods are invoked on an object one after the other, and each method returns the object itself (self
). It allows for more concise and readable code.
37. How can you create an abstract method in Python, and what is its purpose?
Answer: An abstract method is a method defined in an abstract class but without an implementation. It is meant to be overridden by concrete subclasses, ensuring that certain methods are implemented consistently across subclasses. Abstract methods are defined using the @abstractmethod
decorator.
38. Explain the purpose of the __slots__
attribute in Python classes.
Answer: The __slots__
attribute is used to restrict the set of attributes that instances of a class can have. It can be used to optimize memory usage and prevent the creation of new attributes dynamically.
39. What is a data descriptor in Python, and how does it differ from a non-data descriptor?
Answer: A data descriptor is an object attribute that defines both __get__()
and __set__()
methods, allowing it to control both attribute access and assignment. A non-data descriptor only defines __get__()
, making it read-only.
40. What is the purpose of the @staticmethod
decorator in Python, and how is it used?
Answer: The @staticmethod
decorator is used to define a static method in a class. Static methods are not bound to instances and can be called on the class itself. They are often used for utility functions that do not depend on instance-specific data.
41. What is a metaclass in Python, and how is it used?
Answer: A metaclass is a class that defines the behavior of other classes (class factories). It can be used to customize class creation and control class attributes and methods.
42. Explain the concept of method overloading in Python.
Answer: Method overloading refers to defining multiple methods in a class with the same name but different parameter lists. Python does not support method overloading by the number or type of arguments, but you can achieve similar behavior using default arguments and variable-length arguments.
43. What is a class attribute, and how is it different from an instance attribute?
Answer: A class attribute is a variable that is shared among all instances of a class. It is defined at the class level and can be accessed using the class name or any instance. An instance attribute is specific to a particular object and is defined within the constructor method (__init__()
).
44. How do you create a private method in Python, and what is its visibility?
Answer: In Python, there is no strict concept of private methods, but you can indicate a method as private by prefixing its name with a double underscore (__
). However, it is still accessible, but its name is “mangled” to include the class name, making it less visible.
45. What is method overloading in Python, and how is it achieved?
Answer: Method overloading refers to defining multiple methods with the same name in a class, but with different parameters. In Python, you can achieve method overloading by using default argument values or variable-length arguments (*args
and **kwargs
).
46. Explain the concept of method overriding in Python.
Answer: Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. The overridden method in the subclass has the same name and parameters as the method in the superclass.
47. What is the purpose of the @classmethod
decorator in Python?
Answer: The @classmethod
decorator is used to define a class method in Python. Class methods are bound to the class and not the instance, and they can be called on the class itself. They are often used to create alternative constructors for a class.
48. What is a factory method in Python, and how is it implemented?
Answer: A factory method is a method used to create and return instances of a class. It is often used to encapsulate the object creation process. Factory methods are typically defined as class methods and can be used to create objects of that class or its subclasses.
49. What is the purpose of the @property
decorator in Python, and how is it used?
Answer: The @property
decorator is used to define a method as a “getter” for a class attribute. It allows you to access the attribute as if it were an instance variable while providing control over its retrieval.
50. Explain the concept of multiple inheritance in Python.
Answer: Multiple inheritance is a feature that allows a class to inherit properties and behaviors from more than one superclass. In Python, it is implemented by specifying multiple base classes in the class definition using parentheses.