Python Function Interview Questions
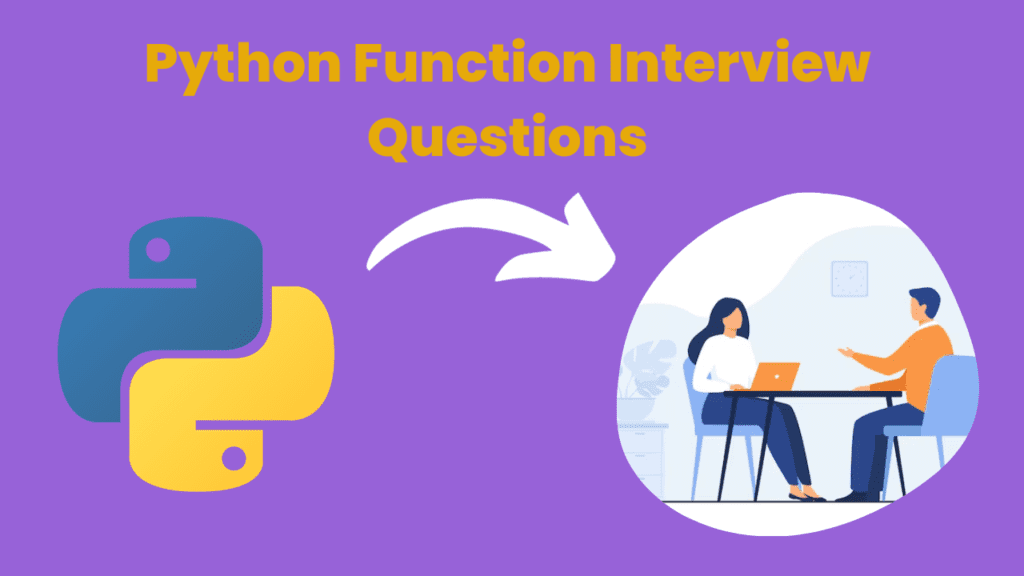
50 most frequently asked Python Function interview questions.
1. How do you define a function in Python?
Answer: You define a function in Python using the def
keyword followed by the function name and a set of parentheses containing parameters. The function body is indented below.
def my_function(parameter1, parameter2):
# Function body
# ...
2. What is a function signature in Python?
Answer: A function signature consists of the function name and the number and types of its parameters. It helps identify the function.
3. Explain the difference between return
and print
in a function.
Answer: return
is used to specify the value that a function should produce as its result. print
is used to display information on the console but does not affect the function’s return value.
def add(a, b):
result = a + b
return result # Returns the result
def greet(name):
print(f"Hello, {name}") # Prints a message but doesn't return a value
4. How do you call a function in Python?
Answer: You call a function by using its name followed by parentheses containing arguments (if any). For example, my_function(arg1, arg2)
.
def greet(name):
print(f"Hello, {name}")
greet("Alice") # Calling the greet function with an argument
5. What is a docstring, and why is it used in Python functions?
Answer: A docstring is a string literal used to provide documentation for functions, classes, or modules. It helps users understand the purpose and usage of the function.
def my_function(parameter):
"""
This is a docstring.
It provides documentation for the function.
"""
# Function body
# ...
6. What is the purpose of the None
keyword in Python functions?
Answer: None
is used to represent the absence of a value in functions. Functions without a return
statement implicitly return None
.
7. How can you define default values for function parameters in Python?
Answer: Default values for function parameters are specified in the function definition, like this: def my_function(param1=default_value)
.
def greet(name="Guest"):
print(f"Hello, {name}")
greet() # Calling greet with the default parameter value
greet("Alice") # Calling greet with a specified argument
8. Explain the concept of function overloading in Python.
Answer: Python does not support function overloading like some other languages. Instead, Python allows you to define multiple functions with the same name but different parameter counts using default arguments.
def add(a, b):
return a + b
def add(a, b, c):
return a + b + c
9. What is the purpose of the *args
and **kwargs
parameters in Python functions?
Answer: *args
is used to pass a variable number of non-keyword arguments to a function, while **kwargs
is used to pass a variable number of keyword arguments.
def my_function(arg1, *args, kwarg1="default", **kwargs):
# arg1 is a regular argument
# *args is a tuple of additional non-keyword arguments
# kwarg1 is a keyword argument with a default value
# **kwargs is a dictionary of additional keyword arguments
10. How do you pass values to a function using keyword arguments in Python?
Answer: You can pass values to a function using keyword arguments by specifying the argument name followed by the value, like this: my_function(param1=value)
.
def greet(name):
print(f"Hello, {name}")
greet(name="Alice") # Calling greet with a keyword argument
11. What is variable scope in Python functions, and how does it work? – Variable scope defines where a variable is accessible. In Python, variables defined within a function have local scope, while variables defined outside functions have global scope.
12. How can you access global variables within a function in Python? – You can access global variables within a function by using the global
keyword or by referencing them directly without reassignment.
13. Explain the purpose of the lambda
keyword in Python. – The lambda
keyword is used to create anonymous (nameless) functions, often for simple operations. It returns a function object.
14. What are recursive functions, and why are they used in Python? – Recursive functions are functions that call themselves. They are used to solve problems that can be broken down into smaller, similar subproblems.
15. How do you pass a function as an argument to another function in Python? – You can pass a function as an argument by using the function name without parentheses, like this: my_function(another_function)
.
16. Explain the purpose of the map()
function in Python. – The map()
function applies a specified function to each item in an iterable and returns an iterator of the results.
17. What is a closure in Python, and how is it created? – A closure is a function object that has access to variables in its enclosing lexical scope. It is created when a nested function references a variable from its containing function.
18. How can you define a recursive function in Python? – A recursive function is defined by calling itself within its own definition until a base case is reached to prevent infinite recursion.
19. What is the purpose of the nonlocal
keyword in Python functions? – The nonlocal
keyword is used to indicate that a variable is not local to the current function’s scope but belongs to an enclosing scope.
20. How can you return multiple values from a Python function? – You can return multiple values as a tuple, list, or other data structure, or you can use unpacking to return multiple values as separate variables.
21. What is the difference between print()
and return
in Python functions? – print()
is used to display information on the console, while return
is used to specify a value as the result of a function.
22. How do you define a default value for a function parameter in Python? – You can define a default value for a function parameter by including it in the function definition, like this: def my_function(param=default_value)
.
23. What is the purpose of the global
keyword in Python functions? – The global
keyword is used to indicate that a variable declared within a function should refer to a global variable instead of creating a new local variable.
24. Explain the concept of recursion depth in Python functions. – Recursion depth refers to the number of recursive function calls in a recursive chain. It affects memory usage and can lead to a “RecursionError” if it exceeds the system’s limit.
25. What is the purpose of the *args
parameter in Python functions, and how is it used? – *args
is used to pass a variable number of non-keyword arguments to a function. It allows functions to accept an arbitrary number of positional arguments.
26. How do you define an anonymous function in Python, and when might you use it? – You define an anonymous function (lambda function) using the lambda
keyword. Lambda functions are often used for short, simple operations, like sorting or filtering.
27. What is function composition in Python, and why is it useful? – Function composition is the practice of combining multiple functions to create a new function. It’s useful for creating complex operations by chaining simpler functions.
28. How can you define a generator function in Python? – A generator function is defined like a regular function but contains one or more yield
statements. It generates values lazily and is often used with large datasets.
29. What is a decorator in Python, and how is it used with functions? – A decorator is a higher-order function that can be used to modify or enhance the behavior of another function. It is applied using the @decorator_name
syntax.
30. What is function memoization, and why is it used in Python? – Function memoization is a technique used to cache and reuse the results of expensive function calls. It can significantly improve the performance of recursive functions.
31. How can you define a function with keyword-only arguments in Python? – You can define a function with keyword-only arguments by using the *
symbol in the function signature, like this: def my_function(*, kwarg1, kwarg2)
.
32. Explain the purpose of the assert
statement in Python functions. – The assert
statement is used for debugging and testing. It raises an AssertionError
if a specified condition is not True
.
33. What are first-class functions in Python, and why are they important? – First-class functions treat functions as first-class citizens, allowing them to be assigned to variables, passed as arguments, and returned from other functions. They are important for functional programming and creating higher-order functions.
34. What is a decorator in Python, and how do you use it to modify a function’s behavior? – A decorator is a function that takes another function as input and returns a modified version of that function. It is often used to add functionality to functions without changing their code.
35. How can you create a closure in Python, and what are common use cases for closures? – Closures are created when a nested function references a variable from its containing function. Common use cases include data encapsulation, function factories, and callback functions.
36. Explain the purpose of the staticmethod
decorator in Python. – The staticmethod
decorator defines a static method within a class that is not bound to instances and can be called on the class itself.
37. How do you define a generator function in Python, and why might you use one? – A generator function is defined like a regular function but contains one or more yield
statements. It is used to generate values lazily, conserving memory and improving performance.
38. What is the difference between a regular function and a lambda function in Python? – A regular function is defined with the def
keyword, has a name, and can contain multiple statements. A lambda function is defined with the lambda
keyword, is nameless, and typically contains a single expression.
39. How can you raise an exception in a Python function, and why might you do so? – You can raise an exception using the raise
keyword, typically to indicate errors or exceptional conditions within a function.
40. What is a recursive function, and what are some common examples of problems solved using recursion? – A recursive function is a function that calls itself. Common examples include calculating factorials, Fibonacci sequences, and traversing trees and graphs.
41. How do you pass a function as an argument to another function in Python, and what is this concept called? – You can pass a function as an argument to another function, and this concept is called “higher-order functions” or “function passing.”
42. What is the purpose of the global
keyword in Python functions, and when should you use it? – The global
keyword is used to indicate that a variable declared within a function should refer to a global variable instead of creating a new local variable. It should be used sparingly to modify global variables within a function.
43. How can you define a function with keyword-only arguments in Python? – You can define a function with keyword-only arguments by using the *
symbol in the function signature, followed by the keyword-only arguments.
44. What is function memoization, and how does it work in Python? – Function memoization is a technique of caching and reusing the results of expensive function calls. It works by storing previously computed results in a cache and returning them when the same inputs are encountered again.
45. How can you pass a variable number of arguments to a Python function using *args
and **kwargs
? – *args
is used to pass a variable number of non-keyword arguments, and **kwargs
is used to pass a variable number of keyword arguments to a function.
46. Explain the purpose of the assert
statement in Python functions. – The assert
statement is used to test conditions within functions and raise an AssertionError
if the condition is not met. It is often used for debugging and testing.
47. What are first-class functions, and why are they important in Python? – First-class functions are functions that can be treated as first-class citizens, allowing them to be assigned to variables, passed as arguments, and returned from other functions. They are important for functional programming and creating higher-order functions.
48. What is a decorator in Python, and how is it used to modify the behavior of functions? – A decorator is a function that takes another function as input and returns a modified version of that function. It is used to add functionality to functions without changing their code.
49. How do you define a generator function in Python, and what are its advantages? – A generator function is defined like a regular function but contains one or more yield
statements. It generates values lazily, conserving memory and improving performance.
50. What is the difference between a regular function and a lambda function in Python? – A regular function is defined using the def
keyword, has a name, and can contain multiple statements. A lambda function is defined using the lambda
keyword, is nameless, and typically contains a single expression.
These questions and answers cover various aspects of functions in Python, including definitions, usage, scope, decorators, memoization, and more.