Python Exception Handling Interview Questions
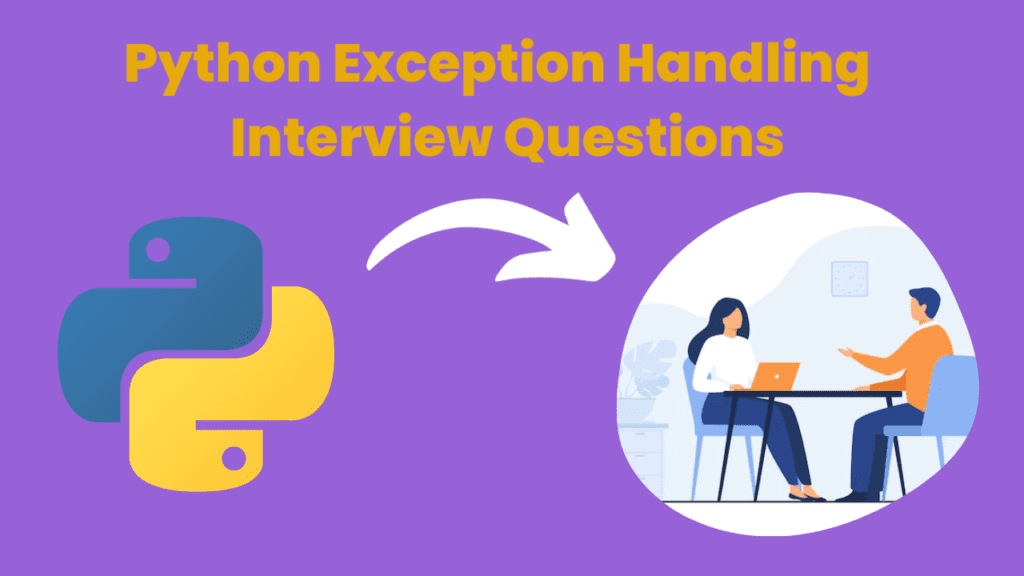
50 most frequently asked Python Exception Handling interview questions.
Get Discount on Top Educational Courses
1. What are exceptions in Python?
Answer: Exceptions are events that occur during the execution of a program, disrupting the normal flow of instructions. They are used to handle errors and unexpected situations.
2. How do you handle exceptions using try, except, and finally blocks?
Answer: You use a try
block to enclose code that may raise an exception. If an exception occurs, the except
block is executed to handle it. The finally
block, if present, is executed afterward regardless of whether an exception occurred.
3. Explain the purpose of the raise
statement in Python.
Answer: The raise
statement is used to manually raise an exception. You can raise built-in exceptions or create custom exceptions by inheriting from the Exception
class.
4. What is the except
clause without an exception type used for?
Answer: An except
clause without an exception type is used as a catch-all to handle any exception that may occur. It is often used as a final exception handler when specific exception types are not known.
5. What is the difference between the except
and else
blocks in exception handling?
Answer: The except
block is executed when an exception occurs, and you specify the exception type to catch. The else
block is executed if no exceptions are raised in the try
block.
6. How can you handle multiple exceptions in a single except
block?
Answer: You can handle multiple exceptions by specifying them as a tuple in a single except
block, like this: except (ExceptionType1, ExceptionType2) as e:
.
7. What is the purpose of the finally
block in exception handling?
Answer: The finally
block is used to define code that must be executed, regardless of whether an exception occurred or not. It’s often used for cleanup tasks, such as closing files or releasing resources.
8.Explain the concept of custom exceptions in Python.
Answer: Custom exceptions are user-defined exception classes that inherit from the Exception
class. They allow you to create specialized exceptions for your applications.
9. What is the difference between the try...except
and try...except...else
blocks?
Answer: The try...except
block is used to catch and handle exceptions. The try...except...else
block also includes an else
block that is executed when no exceptions occur.
10. How can you handle exceptions raised in a function that you call within a try
block?
Answer: You can catch exceptions raised in a function called within a try
block by adding an inner try...except
block within the outer one.
11. What is the purpose of the assert
statement in Python?
Answer: The assert
statement is used to check if a given condition is True
. If the condition is False
, an AssertionError
exception is raised.
12. Explain the difference between a built-in exception and a custom exception.
Answer: Built-in exceptions are provided by Python and cover a wide range of common error conditions. Custom exceptions are user-defined and are tailored to specific application requirements.
13. How do you re-raise an exception in Python?
Answer: You can re-raise an exception by using the raise
statement without any arguments within an except
block. This re-raises the currently caught exception.
14. What is the purpose of the try...finally
block in exception handling?
Answer: The try...finally
block ensures that code within the finally
block is executed regardless of whether an exception occurs or not. It’s commonly used for resource cleanup.
15. Explain the difference between the assert
statement and exception handling.
Answer: The assert
statement is used for debugging and testing to check conditions that should always be True
. Exception handling is used for handling runtime errors and exceptions that may occur during normal program execution.
16. How do you handle exceptions with multiple except
blocks in Python?
Answer: You can handle exceptions with multiple except
blocks by specifying different exception types for each block. Python will execute the first except
block that matches the raised exception.
17. What is the purpose of the try...except...finally
block in exception handling?
Answer: The try...except...finally
block combines error handling (try...except
) with cleanup (finally
) to ensure that resources are properly released even in the presence of exceptions.
18. How can you customize error messages when raising exceptions in Python?
Answer: You can include a message as an argument when raising an exception, like this: raise ExceptionType("Custom error message")
.
19. What is the purpose of the sys.exc_info()
function in Python?
Answer: The sys.exc_info()
function returns information about the current exception being handled, including the exception type, value, and traceback.
20. How do you handle exceptions raised by external libraries in Python?
Answer: You can handle exceptions raised by external libraries by using try...except
blocks and specifying the expected exception types. Additionally, you can check the library’s documentation for specific exceptions.
21. Explain the purpose of the with
statement in Python for exception handling.
Answer: The with
statement is used for context management, ensuring that resources are properly acquired and released, even in the presence of exceptions.
22. What is the finally
block used for in exception handling?
Answer: The finally
block is used to define code that must be executed, regardless of whether an exception occurred or not. It’s often used for cleanup tasks.
23. How can you handle exceptions of unknown types in Python?
Answer: You can handle exceptions of unknown types using a catch-all except
block without specifying an exception type.
24. What is the purpose of the try...except...else...finally
block in exception handling?
Answer: The try...except...else...finally
block combines exception handling (try...except
) with code that runs when no exceptions occur (else
) and code that always runs (finally
).
25. What is the difference between the except
and finally
blocks in exception handling?
Answer: The except
block is used to handle exceptions. The finally
block is used for cleanup code that always runs, regardless of whether an exception occurred or not.
26. How do you raise a custom exception with a specific error message in Python?
Answer: To raise a custom exception with a specific error message, create an instance of the custom exception class and pass the message as an argument to the exception constructor.
27. What is the purpose of the try...except...else
block in exception handling?
Answer: The try...except...else
block allows you to specify code that runs when no exceptions are raised in the try
block. It’s used for code that should only execute when there are no exceptions.
28. Explain the difference between the except
block with multiple exception types and multiple except
blocks.
Answer: The except
block with multiple exception types catches any of the specified exceptions. Multiple except
blocks allow you to handle each exception type differently.
29. What is the purpose of the try...except...else...finally
block in Python exception handling?
Answer: The try...except...else...finally
block combines error handling (try...except
), code that runs when no exceptions occur (else
), and cleanup code (finally
).
30. How can you handle exceptions in a specific order when multiple except
blocks are used?
Answer: When multiple except
blocks are used, Python will execute the first except
block that matches the raised exception. You can order the except
blocks based on the most specific exceptions first.
31. What happens if an exception is raised in the finally
block in Python?
Answer: If an exception is raised in the finally
block, it will replace any exception that may have been raised in the try
or except
blocks. The new exception will propagate unless caught.
32. What is the purpose of the try...except...else...finally
block in Python?
Answer: The try...except...else...finally
block provides a comprehensive structure for handling exceptions, executing code when no exceptions occur (else
), and ensuring cleanup (finally
).
33. How do you create and raise a custom exception class in Python?
Answer: To create and raise a custom exception class, define a new class that inherits from the Exception
class or one of its subclasses. You can then raise instances of this custom exception class.
34. What is the purpose of the try...except...else...finally
block in Python exception handling?
Answer: The try...except...else...finally
block combines error handling (try...except
), code that runs when no exceptions occur (else
), and cleanup code (finally
).
35. How do you handle exceptions in a specific order when using multiple except
blocks?
Answer: When using multiple except
blocks, Python executes the first except
block that matches the raised exception. You can order the except
blocks based on the most specific exceptions first.
36. What happens if an exception is raised in the finally
block in Python?
Answer: If an exception is raised in the finally
block, it will replace any exception that may have been raised in the try
or except
blocks. The new exception will propagate unless caught.
37. What is the purpose of the try...except...else...finally
block in Python?
Answer: The try...except...else...finally
block provides a comprehensive structure for handling exceptions, executing code when no exceptions occur (else
), and ensuring cleanup (finally
).
38. How do you create and raise a custom exception class in Python?
Answer: To create and raise a custom exception class, define a new class that inherits from the Exception
class or one of its subclasses. You can then raise instances of this custom exception class.
39. What is the difference between the assert
statement and exception handling in Python?
Answer: The assert
statement is used for debugging and testing, while exception handling is used to handle errors and exceptional conditions during program execution.
40. What is the purpose of the try...except
block in Python exception handling?
Answer: The try...except
block is used to catch and handle exceptions that may occur within the try
block.
41. How can you specify an action when an exception is not caught in Python?
Answer: You can specify an action when an exception is not caught by using an except
block without an exception type, known as a catch-all or generic except
block.
42. What is the use of the finally
block in exception handling?
Answer: The finally
block is used to define code that must be executed, whether an exception occurs or not. It’s often used for cleanup tasks.
43. How do you raise a custom exception in Python?
Answer: You can raise a custom exception by creating an instance of the custom exception class and using the raise
statement.
44. What is the purpose of the try...except...else
block in Python?
Answer: The try...except...else
block allows you to specify code that runs when no exceptions are raised in the try
block. It’s used for code that should only execute when there are no exceptions.
45. Explain the difference between the except
block with multiple exception types and multiple except
blocks.
Answer: The except
block with multiple exception types catches any of the specified exceptions. Multiple except
blocks allow you to handle each exception type differently.
46. What is the purpose of the try...except...else...finally
block in Python exception handling?
Answer: The try...except...else...finally
block provides a comprehensive structure for handling exceptions, executing code when no exceptions occur (else
), and ensuring cleanup (finally
).
47. How can you handle exceptions in a specific order when multiple except
blocks are used?
Answer: When multiple except
blocks are used, Python will execute the first except
block that matches the raised exception. You can order the except
blocks based on the most specific exceptions first.
48. What happens if an exception is raised in the finally
block in Python?
Answer: If an exception is raised in the finally
block, it will replace any exception that may have been raised in the try
or except
blocks. The new exception will propagate unless caught.
49. What is the purpose of the try...except...else...finally
block in Python?
Answer: The try...except...else...finally
block provides a comprehensive structure for handling exceptions, executing code when no exceptions occur (else
), and ensuring cleanup (finally
).
50. How do you create and raise a custom exception class in Python?
Answer: To create and raise a custom exception class, define a new class that inherits from the Exception
class or one of its subclasses. You can then raise instances of this custom exception class.