Python Advanced Topics Interview Questions
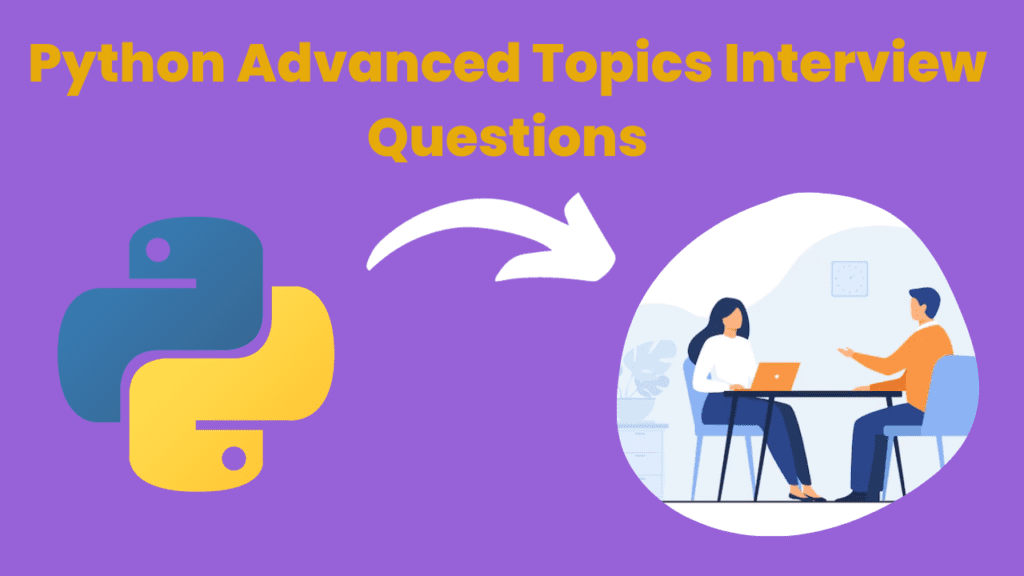
50 most frequently asked Python Python Advanced interview questions.
1. What is a decorator in Python?
Answer: A decorator is a design pattern in Python that allows you to modify or extend the behavior of functions or methods without changing their source code.2.
2. Explain the Global Interpreter Lock (GIL) in Python.
Answer: The GIL is a mutex in CPython (Python’s reference implementation) that allows only one thread to execute Python bytecode at a time. It prevents true multi-core execution in CPython but ensures thread safety.
3. Discuss multithreading and multiprocessing in Python.
Answer: Multithreading involves using multiple threads to perform concurrent tasks within a single process. Multiprocessing, on the other hand, uses multiple processes, allowing true parallelism. Python’s GIL limits the effectiveness of multithreading for CPU-bound tasks but not for I/O-bound tasks.
4. What is a generator, and how does it differ from a regular function?
Answer: A generator is a special type of iterable in Python that generates values lazily using the yield
keyword. It differs from regular functions in that it retains its state between calls and produces values one at a time.
5. How can you handle memory management in Python?
Answer: Python uses automatic memory management (garbage collection). You can manage memory by ensuring proper object reference management, closing file handles, and using tools like sys.getsizeof()
to analyze memory usage.
6. Explain the use of libraries like NumPy, pandas, and Matplotlib in data science.
Answer: NumPy provides support for large, multi-dimensional arrays and matrices along with mathematical functions. pandas offers data structures for data manipulation and analysis. Matplotlib is a data visualization library for creating static, animated, or interactive plots and graphs.
7. What is virtualenv, and why is it used?
Answer: virtualenv is a tool for creating isolated Python environments. It allows you to have separate Python installations and libraries for different projects, preventing conflicts and ensuring project-specific dependencies.
8. What is the purpose of the async
and await
keywords in Python?
Answer: async
and await
are used for asynchronous programming. async
defines a coroutine, and await
suspends the coroutine’s execution until the awaited asynchronous operation is complete.
9. Explain the concept of metaclasses in Python.
Answer: Metaclasses are classes that define the behavior of other classes (class factories). They can be used to customize class creation, attribute access, and more.
10. What is the purpose of the __slots__
attribute in Python classes?
Answer: __slots__
allows you to specify a fixed set of attributes for a class, reducing memory usage and preventing the creation of new attributes dynamically.
11. How do you create a context manager in Python using the with
statement?
Answer: You can create a context manager by defining a class with __enter__
and __exit__
methods or using the @contextlib.contextmanager
decorator with a generator function.
12. Explain the purpose of the pickle
module in Python.
Answer: The pickle
module is used for serializing and deserializing Python objects. It allows objects to be saved to a file and loaded back into memory.
13. What is the purpose of the os
module in Python?
Answer: The os
module provides a way to interact with the operating system, including functions for file and directory manipulation, environment variables, and process management.
14. Discuss the differences between list comprehensions and generator expressions in Python.
Answer: List comprehensions create lists and evaluate all elements at once, while generator expressions create generators and yield elements lazily, conserving memory.
15. Explain the concept of monkey patching in Python.
Answer: Monkey patching is the practice of dynamically modifying or extending classes and modules at runtime. It’s often used to fix bugs or add features to existing code.
16. What is a closure in Python, and how is it created?
Answer: A closure is a function that retains access to variables from its containing (enclosing) function’s scope even after the outer function has finished execution. Closures are created when a nested function references a variable from its containing function.
17. How can you measure the execution time of a Python program or function?
Answer: You can use the time
module to measure execution time by recording the start and end times and calculating the difference.
18. What are the key differences between Python 2.x and Python 3.x?
Answer: Python 3 introduced several significant changes, including print as a function, improved Unicode support, and various syntax updates. Python 2 is no longer maintained, so Python 3 is the recommended version.
19. Explain the purpose of the functools
module in Python.
Answer: The functools
module provides higher-order functions and operations on callable objects, such as memoization using functools.lru_cache()
and function partial application using functools.partial()
.
20. What is the purpose of the asyncio
module in Python?
Answer: The asyncio
module provides support for asynchronous I/O, event loops, and coroutines, making it suitable for building asynchronous and concurrent applications.
21. Explain the purpose of the collections.namedtuple
in Python.
Answer: collections.namedtuple
is a factory function that creates a named tuple class with named fields, providing a more readable alternative to regular tuples.
22. What is the Global Interpreter Lock (GIL) in Python, and how does it impact multi-threading?
Answer: The GIL is a mutex that prevents multiple native threads from executing Python bytecode concurrently in CPython. It can limit the effectiveness of multi-threading for CPU-bound tasks but not for I/O-bound tasks.
23. How can you handle exceptions using the try
, except
, and finally
blocks in Python?
Answer: try
is used to enclose code that may raise an exception. except
is used to catch and handle exceptions. finally
contains code that runs regardless of whether an exception occurred or not.
24. Explain the purpose of the raise
statement in Python.
Answer: The raise
statement is used to manually raise exceptions in Python. It allows you to signal exceptional conditions in your code.
25. What is the except clause without an exception type used for in Python?
Answer: An except
clause without an exception type catches and handles any exception raised in the try
block. It is a catch-all for exceptions.
26. How do you open and close files in Python?
Answer: Use the open()
function to open files and the .close()
method or the with
statement to close them.
27. Explain the modes for file opening in Python.
Answer: File modes include 'r'
for reading, 'w'
for writing (creates a new file or truncates an existing one), 'a'
for appending, 'b'
for binary mode, and 'x'
for exclusive creation.
28. What is the purpose of the with
statement in file handling?
Answer: The with
statement ensures proper resource management, such as automatically closing files when the block exits.
29. How do you read and write text files in Python?
Answer: You can use open()
with 'r'
mode to read text files and 'w'
mode to write text to files.
30. What is a decorator in Python, and how is it used?
Answer: A decorator is a function that modifies the behavior of another function. It is used by placing the @decorator_name
line above the function definition.
31. Explain the purpose of the async
and await
keywords in Python.
Answer: async
is used to define asynchronous functions (coroutines), and await
is used within async functions to pause execution until an asynchronous operation is complete.
32. What is memoization, and how can you implement it in Python?
Answer: Memoization is a technique of storing the results of expensive function calls and returning the cached result when the same inputs occur again. You can use decorators or dictionaries to implement memoization.
33. How does garbage collection work in Python, and what is its purpose?
Answer: Python’s garbage collector automatically reclaims memory used by objects that are no longer reachable or referenced, preventing memory leaks.
34. Explain the purpose of the os
module in Python.
Answer: The os
module provides functions for interacting with the operating system, including file and directory operations, process management, and environment variables.
35. What is the purpose of the multiprocessing
module in Python?
Answer: The multiprocessing
module allows you to create and manage multiple processes, enabling true parallelism and avoiding the GIL limitations of multi-threading.
36. What is a metaclass in Python, and how is it different from a regular class?
Answer: A metaclass is a class that defines the behavior of other classes (class factories). It differs from regular classes as it controls class creation and attributes.
37. Explain the use of list comprehensions and generator expressions in Python.
Answer: List comprehensions create lists by applying an expression to each item in an iterable. Generator expressions create generators and yield values lazily, conserving memory.
38. What is monkey patching in Python, and when might it be used?
Answer: Monkey patching is the practice of dynamically modifying or extending classes and modules at runtime. It’s used to fix bugs or add features to existing code without modifying the source.
39. What is the purpose of the functools
module in Python?
Answer: The functools
module provides higher-order functions and operations on callable objects, including memoization, partial function application, and more.
40. Explain the concept of closures in Python.
Answer: Closures are functions that retain access to variables from their containing (enclosing) function’s scope, even after the outer function has finished execution.
41. What is the purpose of the contextlib
module in Python?
Answer: The contextlib
module provides utilities for working with context managers, including the @contextmanager
decorator for creating custom context managers.
42. Explain the concept of metaprogramming in Python.
Answer: Metaprogramming is the practice of writing code that generates or modifies other code during runtime. It allows you to create flexible and dynamic programs.
43. What is the Global Interpreter Lock (GIL) in Python, and how does it impact multi-threading?
Answer: The GIL is a mutex that prevents multiple native threads from executing Python bytecode concurrently in CPython. It can limit the effectiveness of multi-threading for CPU-bound tasks but not for I/O-bound tasks.
44. How do you handle exceptions using the try
, except
, and finally
blocks in Python?
Answer: try
is used to enclose code that may raise an exception. except
is used to catch and handle exceptions. finally
contains code that runs regardless of whether an exception occurred or not.
45. Explain the purpose of the raise
statement in Python.
Answer: The raise
statement is used to manually raise exceptions in Python. It allows you to signal exceptional conditions in your code.
46. What is the except clause without an exception type used for in Python?
Answer: An except
clause without an exception type catches and handles any exception raised in the try
block. It is a catch-all for exceptions.
47. How do you open and close files in Python?
Answer: Use the open()
function to open files and the .close()
method or the with
statement to close them.
48. Explain the modes for file opening in Python.
Answer: File modes include 'r'
for reading, 'w'
for writing (creates a new file or truncates an existing one), 'a'
for appending, 'b'
for binary mode, and 'x'
for exclusive creation.
49. What is the purpose of the with
statement in file handling?
Answer: The with
statement ensures proper resource management, such as automatically closing files when the block exits.
50. How do you read and write text files in Python?
Answer: You can use open()
with 'r'
mode to read text files and 'w'
mode to write text to files.
These questions cover a range of advanced Python topics, including metaprogramming, exceptions, and file handling, to help you prepare for interviews.