Java Threads and Concurrency Interview Questions
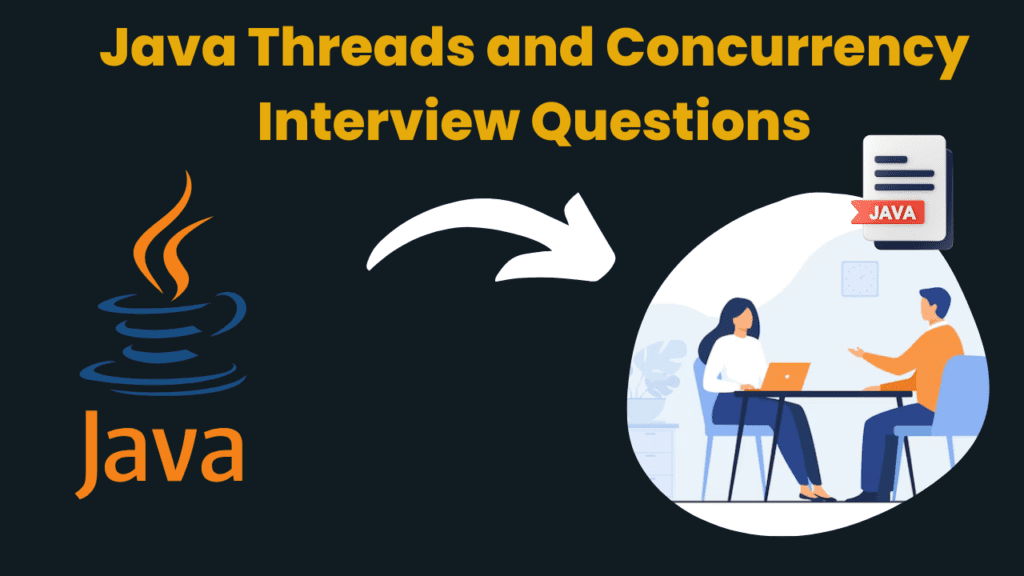
50 most frequently asked Java Threads and Concurrency Interview Questions.
Get Discount on Top Educational Courses
1. What is multithreading, and why is it important in Java programming?
Answer: Multithreading is the concurrent execution of multiple threads within a single program. It is essential for improving program efficiency and handling tasks concurrently.
2. Explain the difference between a thread and a process in Java.
Answer: A process is an independent program with its own memory space, while a thread is a unit of a process that shares the same memory space with other threads in the same process.
3. What is a thread in Java, and how is it created?
Answer: A thread in Java is a lightweight, executable unit of a program. Threads can be created by extending the Thread
class or implementing the Runnable
interface.
4. What is the ‘Runnable’ interface, and how does it relate to multithreading in Java?
Answer: The Runnable
interface defines a single method run()
. It is used to represent a task that can be executed concurrently within a thread.
5. Explain the difference between extending the ‘Thread’ class and implementing the ‘Runnable’ interface for thread creation.
Answer: Extending the ‘Thread’ class is a way to create a thread, while implementing the ‘Runnable’ interface allows better separation of concerns as the class can extend another class if needed.
6. What is the ‘Thread’ class in Java, and how is it used for multithreading?
Answer: The ‘Thread’ class is a fundamental class in Java for creating and managing threads. It provides methods for thread creation, start, and control.
7. Explain the lifecycle of a thread in Java, including its different states.
Answer: The thread lifecycle consists of the states: NEW, RUNNABLE, BLOCKED, WAITING, TIMED_WAITING, and TERMINATED. Threads transition through these states as they are created, run, and complete.
8. What is the ‘start’ method in the ‘Thread’ class, and why is it used to begin thread execution?
Answer: The ‘start’ method is used to initiate the execution of a thread. It schedules the thread to run its ‘run’ method concurrently.
9. What is thread priority, and how is it set in Java?
Answer: Thread priority is a value indicating the relative importance of a thread. It can be set using the setPriority()
method, with values ranging from 1 (lowest) to 10 (highest).
10. Explain the concept of thread synchronization and why it’s crucial in multithreaded applications.
Answer: Thread synchronization is the coordination of threads to ensure data consistency and prevent race conditions. It’s crucial in multithreaded applications to avoid unpredictable and erroneous behavior.
11. What are the differences between ‘synchronized’ methods and ‘synchronized’ blocks in Java?
Answer: ‘synchronized’ methods synchronize the entire method, while ‘synchronized’ blocks allow for more fine-grained control by specifying the synchronized block’s scope.
12. What is a race condition, and how does it occur in multithreaded programs?
Answer: A race condition occurs when multiple threads access shared data concurrently, leading to unpredictable and erroneous results due to the order of execution.
13. Explain the ‘wait’ and ‘notify’ methods in Java and their role in thread communication.
Answer: The ‘wait’ method is used to make a thread wait for a condition to be met, and the ‘notify’ method is used to wake up a waiting thread when the condition is satisfied.
14. What is the ‘volatile’ keyword in Java, and how does it affect thread visibility and memory consistency?
Answer: The ‘volatile’ keyword ensures that changes to a variable are immediately visible to other threads and helps enforce memory consistency.
15. Explain the ‘join’ method in Java and its role in coordinating thread execution.
Answer: The ‘join’ method is used to make one thread wait for another thread to complete its execution. It is useful for coordinating the order of execution.
16. What is a thread pool, and how does it help manage and reuse threads efficiently?
Answer: A thread pool is a managed group of threads that can be reused for executing tasks. It helps avoid the overhead of creating new threads for each task.
17. What is the ‘Executor’ framework in Java, and how does it simplify thread management?
Answer: The ‘Executor’ framework provides a higher-level abstraction for managing and executing threads, making it easier to work with thread pools and execute tasks.
18. Explain the ‘Callable’ and ‘Future’ interfaces in Java and their use for executing tasks with return values.
Answer: The ‘Callable’ interface is used for tasks that return values. The ‘Future’ interface is used to obtain the result of a ‘Callable’ task and provides methods for checking if the task is complete.
19. What are daemon threads in Java, and how do they differ from user threads?
Answer: Daemon threads are background threads that do not prevent the JVM from exiting when all user threads have finished. They are typically used for tasks like garbage collection.
20. What is the Java Memory Model, and how does it ensure memory consistency in multithreaded programs?
Answer: The Java Memory Model defines the rules and guarantees for memory visibility and consistency in multithreaded programs. It ensures that changes to shared data are properly synchronized.
21. Explain the concept of thread interruption in Java and how it’s used for thread control.
Answer: Thread interruption is a mechanism for asking a thread to stop its execution gracefully. It is used for cooperative thread termination.
22. What is the ‘ReentrantLock’ class in Java, and how is it used for explicit thread synchronization?
Answer: ‘ReentrantLock’ is a class that provides a more flexible alternative to ‘synchronized’ blocks for explicit thread synchronization. It allows fine-grained control over locking and unlocking.
23. Explain the ‘Semaphore’ class in Java and its use for managing access to a limited number of resources.
Answer: The ‘Semaphore’ class is used for controlling access to a limited number of resources. It allows a fixed number of threads to acquire permits before blocking others.
24. What is the ‘CyclicBarrier’ class, and how does it facilitate synchronization among a group of threads?
Answer: The ‘CyclicBarrier’ class is used to coordinate a group of threads that must all reach a barrier point before continuing.
25. Explain the ‘CountDownLatch’ class and its use for waiting for a set of threads to complete their tasks.
Answer: ‘CountDownLatch’ is used for waiting until a set of threads has completed their tasks. It allows one or more threads to await the completion of multiple operations.
26. What is the ‘Phaser’ class in Java, and how does it provide more flexible synchronization than ‘CountDownLatch’?
Answer: ‘Phaser’ is a more flexible synchronization mechanism that allows for dynamic registration of threads and advanced synchronization control.
27. What is the ‘LockSupport’ class, and how is it used for low-level thread synchronization and parking threads?
Answer: ‘LockSupport’ is a low-level class for thread synchronization. It provides methods for parking and unparking threads, enabling more advanced synchronization mechanisms.
28. Explain the ‘Exchanger’ class in Java and its use for exchanging data between two threads.
Answer: ‘Exchanger’ is used for coordination between two threads. It allows them to exchange data at a predefined synchronization point.
29. What is the ‘ThreadLocal’ class, and how does it help manage thread-specific data?
Answer: ‘ThreadLocal’ is used to store data that is specific to each thread. It ensures that each thread has its own independent copy of the data.
30. What is the ‘CompletableFuture’ class, and how does it simplify asynchronous and parallel programming in Java?
Answer: ‘CompletableFuture’ is a class that simplifies asynchronous programming by providing a framework for composing and orchestrating asynchronous tasks.
31. Explain the concept of deadlock in multithreading, and what are some common causes of deadlocks?
Answer: Deadlock is a situation where two or more threads are unable to proceed because each is waiting for the other to release a resource. Common causes include circular waiting, resource contention, and lack of proper synchronization.
32. What is thread starvation, and how does it relate to thread priority and scheduling in Java?
Answer: Thread starvation occurs when a thread is not given a fair chance to execute due to lower priority or unfair scheduling. Thread priority influences the order in which threads are scheduled by the JVM.
33. Explain the ‘synchronized’ keyword and its role in ensuring mutually exclusive access to shared resources.
Answer: The ‘synchronized’ keyword is used to mark a method or block as critical sections, ensuring that only one thread can execute that code at a time, preventing race conditions.
34. What is the ‘Atomic’ package in Java, and how does it provide thread safety for operations on primitive types and references?
Answer: The ‘Atomic’ package provides classes like AtomicInteger and AtomicReference that offer atomic operations, ensuring thread safety for incrementing, updating, or swapping values.
35. Explain the ‘ThreadGroup’ class in Java and its role in organizing and managing threads.
Answer: The ‘ThreadGroup’ class is used for organizing threads into a hierarchical structure. It allows you to manage and control a group of threads as a single entity.
36. What is the purpose of the ‘java.util.concurrent’ package in Java, and what are some key classes it provides for concurrency control?
Answer: The ‘java.util.concurrent’ package offers classes for advanced concurrency control, including Executors, Locks, ConcurrentCollections, and more.
37. Explain the ‘ForkJoinPool’ class in Java and how it is used for parallelism and efficient task execution.
Answer: ‘ForkJoinPool’ is a specialized Executor for parallelism, particularly for divide-and-conquer tasks. It efficiently manages and schedules tasks that can be split into subtasks.
38. What is a thread-local variable, and how is it different from a regular variable in Java?
Answer: A thread-local variable is specific to each thread and holds a separate value for each thread that accesses it. It is different from a regular variable, which is shared among all threads.
39. Explain the ‘ScheduledExecutorService’ interface in Java and its role in scheduling tasks for execution at a future time or at regular intervals.
Answer: The ‘ScheduledExecutorService’ interface is used for scheduling tasks to be executed at specific times or with fixed-rate or fixed-delay intervals.
40. What is the ‘ReadWriteLock’ interface in Java, and how does it provide efficient access to shared resources for read and write operations?
Answer: The ‘ReadWriteLock’ interface provides a mechanism for multiple threads to access shared resources concurrently for reading while allowing exclusive access for writing.
41. Explain the concept of thread local memory and how it relates to thread performance and cache management.
Answer: Thread local memory is memory allocated for each thread, optimizing data access and reducing contention, particularly in a multi-core processor environment.
42. What is the ‘ExecutorService’ interface, and how does it provide a higher-level way to manage and execute threads?
Answer: The ‘ExecutorService’ interface is an abstraction for managing and executing tasks in a thread pool. It simplifies thread management, task submission, and result retrieval.
43. What is the ‘CompletableFuture’ class, and how does it facilitate asynchronous and non-blocking programming in Java?
Answer: ‘CompletableFuture’ is a class that allows you to perform asynchronous and non-blocking operations, enabling efficient handling of concurrent tasks.
44. Explain the concept of thread affinity and how it impacts thread performance in a multicore environment.
Answer: Thread affinity refers to the association of a thread with a specific core in a multicore processor. It can improve performance by reducing cache contention and context switching.
45. What is the ‘Lock’ interface in Java, and how does it provide more flexibility and control over synchronization than intrinsic locks?
Answer: The ‘Lock’ interface provides a more flexible and explicit way to control synchronization, allowing for finer-grained control and advanced features like condition variables.
46. Explain the ‘CopyOnWriteArraySet’ class and how it provides thread safety for sets in Java.
Answer: ‘CopyOnWriteArraySet’ is a thread-safe Set implementation. It uses a copy-on-write strategy to ensure thread safety while allowing for efficient reads.
47. What is the ‘CountDownLatch’ class, and how is it used for synchronizing the execution of multiple threads?
Answer: ‘CountDownLatch’ is used to synchronize the execution of multiple threads by ensuring that a set of threads waits for a specified count to reach zero before continuing.
48. What is the ‘Phaser’ class in Java, and how does it offer more flexibility and functionality than ‘CountDownLatch’?
Answer: ‘Phaser’ provides advanced synchronization capabilities, allowing dynamic registration of threads and more flexible coordination than ‘CountDownLatch.’
49. Explain the concept of thread yield in Java and its impact on thread scheduling.
Answer: Thread yield is a hint to the JVM that the current thread is willing to give up its current execution to allow other threads to run. It can impact thread scheduling but is not guaranteed.
50. What is the ‘Exchanger’ class, and how does it facilitate data exchange between two threads in Java?
Answer: ‘Exchanger’ is used for data exchange between two threads. Each thread can exchange data at a synchronization point provided by the ‘Exchanger.’