Java Data Type Interview Questions
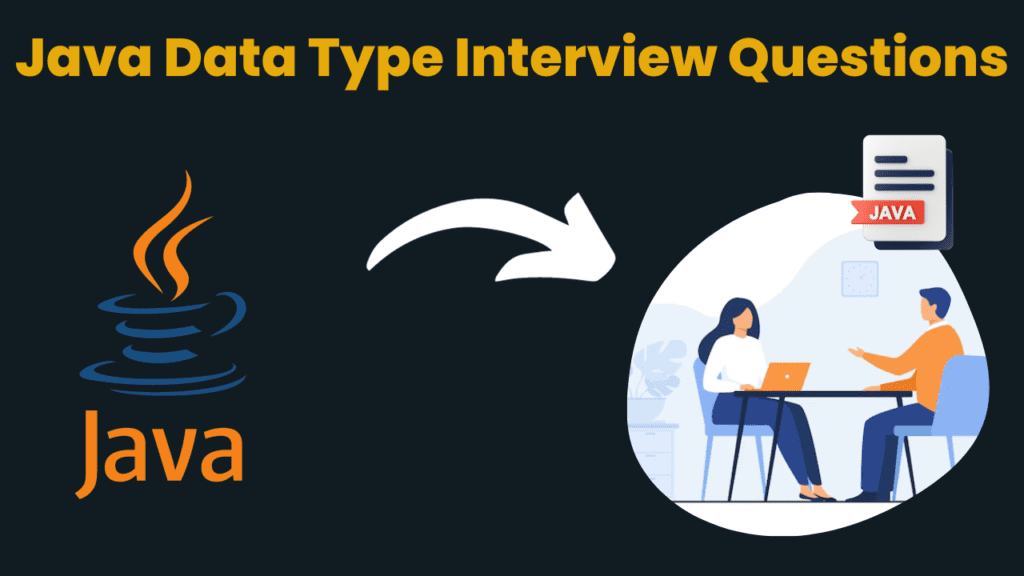
50 most frequently asked Java Data Type Interview Questions.
Get Discount on Top Educational Courses
1. What is a data type in Java?
Answer: A data type in Java is a classification that specifies which type of data a variable can hold.
2. What are the two main categories of data types in Java?
Answer: The two main categories of data types in Java are primitive data types and reference data types.
3. How are primitive data types different from reference data types in Java?
Answer: Primitive data types store simple values, while reference data types store references or addresses to objects.
4. What is the purpose of declaring a variable in Java?
Answer: Declaring a variable in Java reserves memory to store data and associates a name with the memory location.
5. What is the syntax for declaring a variable in Java?
Answer: The syntax for declaring a variable is: data_type variable_name;
6. Give an example of declaring an integer variable in Java.
Answer: Example: int myNumber;
7. What is the purpose of initializing a variable in Java?
Answer: Initializing a variable assigns an initial value to the variable, allowing it to be used in calculations and operations.
8. What is the syntax for initializing a variable in Java?
Answer: The syntax for initializing a variable is: data_type variable_name = initial_value;
9. Give an example of declaring and initializing a double variable in Java.
Answer: Example: double pi = 3.14159;
10. What is the difference between ‘int’ and ‘double’ data types in Java, and when would you use each?
Answer: ‘int’ is used for storing integer values, and ‘double’ is used for storing floating-point (decimal) values. Use ‘int’ for whole numbers and ‘double’ for numbers with fractions.
11. What are the eight primitive data types in Java?
Answer: The eight primitive data types in Java are byte, short, int, long, float, double, char, and boolean.
12. What is the maximum value that can be stored in a ‘byte’ variable in Java?
Answer: The maximum value for a ‘byte’ variable is 127.
13. What is the difference between ‘float’ and ‘double’ data types in Java, and when would you use each?
Answer: ‘float’ is a single-precision floating-point data type, and ‘double’ is a double-precision floating-point data type. Use ‘float’ when you need to save memory, and ‘double’ when you need higher precision.
14. Explain the ‘char’ data type in Java, and how are characters represented?
Answer: The ‘char’ data type is used to store a single Unicode character. Characters are represented using single quotes, e.g., ‘A’.
15. What is the default value for a ‘boolean’ variable in Java?
Answer: The default value for a ‘boolean’ variable is ‘false’.
16. What is the ‘null’ value, and in which data types can it be used in Java?
Answer: ‘null’ represents the absence of a value and can be assigned to reference data types like objects and strings.
17. How do you declare a constant variable in Java, and what is the naming convention for constants?
Answer: Constants are declared using the ‘final’ keyword, and naming conventions typically use uppercase letters with underscores, e.g., final int MAX_VALUE = 100;
.
18. What is a variable’s scope in Java, and how does it affect where the variable can be used?
Answer: Variable scope defines where in the code the variable is accessible. Variables can have local, instance, or class scope.
19. What is the lifetime of a local variable in Java?
Answer: Local variables exist only within the block or method where they are declared and have a shorter lifetime compared to instance or class variables.
20. What is variable shadowing, and how does it occur in Java?
Answer: Variable shadowing occurs when a local variable has the same name as an instance or class variable, making the local variable take precedence in that scope.
21. What is type casting in Java, and when is it required?
Answer: Type casting is the process of converting one data type to another. It is required when you need to assign a value from one type to another, such as from a ‘double’ to an ‘int’.
22. What is an implicit type cast, and when does it occur in Java?
Answer: An implicit type cast is an automatic conversion of a smaller data type to a larger data type, e.g., from ‘int’ to ‘double’.
23. What is an explicit type cast, and how is it done in Java?
Answer: An explicit type cast is a manual conversion of a larger data type to a smaller data type. It is done by specifying the target data type in parentheses, e.g., (int) 3.14159
.
24. Explain the concept of widening and narrowing conversions in type casting.
Answer: Widening conversion occurs when a smaller data type is cast to a larger data type (e.g., ‘int’ to ‘double’). Narrowing conversion is the opposite, where a larger data type is cast to a smaller one, which may result in data loss.
25. What is variable initialization, and why is it important in Java?
Answer: Variable initialization is the process of giving a variable an initial value. It is essential to prevent using variables with unpredictable values.
26. What is the scope of instance variables in Java, and where are they declared?
Answer: Instance variables are declared within a class but outside of any method. They have class-wide scope and are accessible throughout the class.
27. What is the scope of class variables (static variables) in Java, and where are they declared?
Answer: Class variables, or static variables, are declared within a class and marked as ‘static.’ They have class-wide scope and are shared among all instances of the class.
28. What is the ‘this’ keyword in Java, and how is it used to refer to instance variables?
Answer: ‘this’ is a reference to the current object and can be used to distinguish between instance variables and method parameters with the same names.
29. How do you declare and initialize a character array (char[]) in Java?
Answer: You can declare and initialize a character array like this: char[] letters = {'a', 'b', 'c'};
.
30. What is the concept of “finalization” for objects in Java, and how is it related to variable cleanup?
Answer: Finalization is the process of releasing resources and cleaning up when an object is about to be garbage collected. It is related to variable cleanup because it allows for custom cleanup code in objects.
31. Explain the role of the ‘new’ keyword in creating objects in Java.
Answer: The ‘new’ keyword is used to create new instances of objects from class definitions. It allocates memory for the object and invokes the constructor to initialize it.
32. What is a local variable in Java, and how is it different from instance and class variables?
Answer: A local variable is declared within a method and has a limited scope. It differs from instance and class variables, which are declared within a class and have broader scopes.
33. What is a “literal” in Java, and how are they used with data types?
Answer: Literals are constant values in Java’s source code. They are used directly with data types to represent values, such as integer literals (e.g., 42) or string literals (e.g., “Hello”).
34. What is variable naming convention in Java, and why is it important to follow it?
Answer: Variable naming conventions in Java recommend using meaningful names with camelCase for variables. Following conventions improves code readability and maintainability.
35. What are the rules for naming variables in Java?
Answer: Variable names in Java must begin with a letter, dollar sign, or underscore, followed by letters, digits, underscores, or dollar signs. They are case-sensitive and should not be Java keywords.
36. Explain the concept of “final variables” in Java, and how are they different from regular variables?
Answer: Final variables, marked with the ‘final’ keyword, cannot be reassigned after their initial value is assigned. They act as constants, and any attempt to modify them will result in a compilation error.
37. What is the difference between “local variables” and “instance variables” in terms of their declaration and scope?
Answer: Local variables are declared within methods and have method-level scope, while instance variables are declared within a class but outside methods and have object-level scope.
38. What is the purpose of variable data types, and how do they affect the storage of values?
Answer: Variable data types specify the kind of data that can be stored in a variable and the amount of memory allocated. They determine the range and precision of values that can be stored.
39. Explain the concept of “automatic type promotion” in Java.
Answer: Automatic type promotion occurs when a smaller data type is automatically promoted to a larger data type in expressions, to prevent data loss and maintain precision.
40. What is the “declaration statement” for variables in Java, and how does it relate to variable initialization?
Answer: The declaration statement for variables in Java declares a variable’s name and data type. It can also include variable initialization, where the variable is given an initial value.
41. What is the “stack” and “heap” in memory management in Java, and how are variables stored in these areas?
Answer: In Java, the “stack” is used for local variables and method calls, while the “heap” is used for objects and dynamically allocated memory. Local variables are stored on the stack, while objects and instance variables are stored in the heap.
42. What is the difference between “copy by value” and “copy by reference” when passing variables to methods?
Answer: In “copy by value,” a copy of the variable’s value is passed to the method, so changes to the parameter do not affect the original variable. In “copy by reference,” the method receives a reference to the original variable, and changes to the parameter affect the original.
43. What is a “constant pool” in Java, and how is it related to string literals and variable initialization?
Answer: The constant pool is a special area in memory where string literals and constants are stored. String literals and constant variables are usually stored in the constant pool, saving memory and improving performance.
44. Explain the concept of “scope resolution” for variables in Java, and how does it determine which variable is accessed?
Answer: Scope resolution refers to the process of determining which variable is being accessed when multiple variables with the same name exist in different scopes. The innermost variable takes precedence.
45. What are “local constants” in Java, and how do they differ from regular variables?
Answer: Local constants are final variables declared within a method. They cannot be modified after being assigned a value, and they act as read-only variables within the method.
46. How is memory allocated for variables with dynamic data types in Java, and what are some examples of such variables?
Answer: Variables with dynamic data types are typically reference data types. Memory is allocated for them on the heap, and they can refer to objects of different classes. Examples include variables of type ‘Object’ or ‘String’.
47. Explain the “instanceof” operator in Java and how it is used to check variable types.
Answer: The “instanceof” operator is used to determine if an object is an instance of a particular class or interface. It is often used to check the type of an object before performing type-specific operations.
48. What is the “ternary operator” in Java, and how is it used to assign values to variables conditionally?
Answer: The ternary operator (?:) is a shorthand way of assigning values to variables based on a condition. It has the format: variable = (condition) ? value_if_true : value_if_false;
.
49. Explain “variable hoisting” in JavaScript and its difference from Java’s variable declaration.
Answer: Variable hoisting in JavaScript involves moving variable declarations to the top of their respective scopes during execution. In Java, variables must be declared before use, and there is no hoisting.
50. What is the significance of variable initialization in control structures like loops and conditionals in Java?
Answer: Variable initialization is important in control structures as it determines the starting values for loop counters or condition variables. Proper initialization can affect the behavior and outcome of control structures.