Java Control Flow Interview Questions
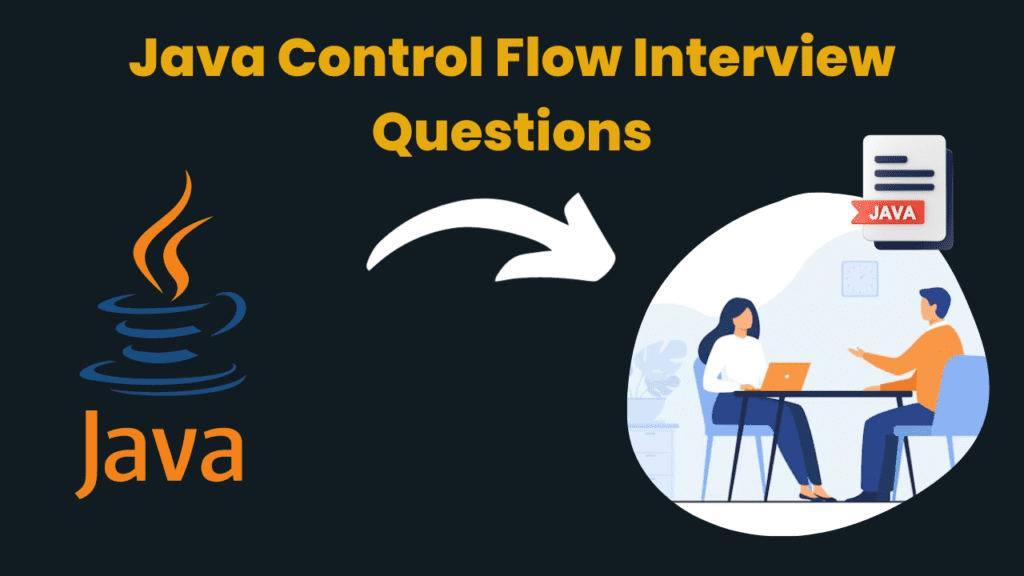
50 most frequently asked Java Control Flow Type Interview Questions.
1. What is control flow in Java, and why is it important in programming?
Answer: Control flow refers to the order in which statements in a program are executed. It’s essential for defining the logic and sequence of operations in a program.
2. What are the primary categories of control flow in Java?
Answer: The primary categories of control flow in Java are conditional statements, loops, and method calls.
3. Explain the ‘if’ statement in Java and its syntax.
Answer: The ‘if’ statement is used for conditional execution. Syntax:
if (condition) {
// Code to execute if condition is true
}
4. What is the purpose of the ‘else’ clause in an ‘if’ statement?
Answer: The ‘else’ clause is used to provide an alternative code block that executes when the ‘if’ condition is false.
5. What is the ‘else if’ statement in Java, and how is it used for multiple conditions?
Answer: The ‘else if’ statement allows you to specify multiple conditions in a chain, with each condition tested in sequence until one is true.
6. Explain the ‘switch’ statement in Java and its syntax.
Answer: The ‘switch’ statement is used to evaluate a variable against a list of values. Syntax:
switch (variable) {
case value1:
// Code for value1
break;
case value2:
// Code for value2
break;
default:
// Code for default case
}
7. What is the purpose of the ‘break’ statement in a ‘switch’ statement?
Answer: The ‘break’ statement is used to exit the ‘switch’ statement after a case is executed, preventing subsequent cases from being executed.
8. What is the ‘for’ loop in Java, and how is it used?
Answer: The ‘for’ loop is used for iterating a specific number of times. Syntax:
for (initialization; condition; update) {
// Code to repeat
}
9. Explain the ‘while’ loop in Java and its syntax.
Answer: The ‘while’ loop is used for repetitive execution as long as a condition is true. Syntax:
while (condition) {
// Code to repeat
}
10. What is the ‘do-while’ loop in Java, and how is it different from the ‘while’ loop?
Answer: The ‘do-while’ loop is similar to the ‘while’ loop, but it always executes the code block at least once, as the condition is checked after execution.
11. Explain the ‘break’ statement in Java and its role in control flow.
Answer: The ‘break’ statement is used to exit a loop or switch statement prematurely, transferring control to the statement following the loop or switch.
12. What is the ‘continue’ statement in Java, and how does it affect loop execution?
Answer: The ‘continue’ statement is used to skip the current iteration of a loop and proceed to the next iteration without executing the remaining code in the current iteration.
13. What are nested loops in Java, and how are they used?
Answer: Nested loops are loops within other loops. They are used for more complex repetition patterns, like iterating through 2D arrays or generating combinations.
14. Explain the ‘return’ statement in Java and its role in control flow within methods.
Answer: The ‘return’ statement is used to exit a method and provide a return value to the caller. It can also be used to control the flow within a method by terminating it prematurely.
15. What is the ‘break’ label in Java, and how is it used with nested loops?
Answer: The ‘break’ label is used to specify which loop to break out of when dealing with nested loops. It allows you to break out of a specific loop when multiple loops are nested.
16. What is a “labeled break” in Java, and how is it used to break out of nested loops?
Answer: A labeled break is used to exit from a specific loop when dealing with nested loops. It specifies which loop to break out of, allowing more precise control.
17. What is a “labeled continue” in Java, and how is it used with nested loops?
Answer: A labeled continue is used to skip the current iteration of a specific loop when dealing with nested loops. It allows you to continue or skip iterations of a particular loop.
18. What is the ‘for-each’ loop in Java, and how is it used to iterate over arrays or collections?
Answer: The ‘for-each’ loop (enhanced for loop) simplifies array and collection iteration by providing a concise syntax. It automatically iterates through all elements without needing an index variable.
19. What is the ‘switch’ statement fall-through behavior, and how is it controlled in Java?
Answer: By default, the ‘switch’ statement falls through to subsequent cases after a matching case is found. Fall-through can be controlled using ‘break’ statements to exit the ‘switch’ statement.
20. Explain the concept of “short-circuit evaluation” in logical expressions in Java.
Answer: Short-circuit evaluation is a feature in logical expressions where the second operand is not evaluated if the outcome can be determined based on the evaluation of the first operand.
21. What is the ‘assert’ statement in Java, and how is it used for debugging?
Answer: The ‘assert’ statement is used to test assumptions about program behavior. It is used for debugging and is typically enabled in development but disabled in production.
22. What is the ‘return’ statement’s role in recursion and how it affects the call stack.
Answer: In recursive methods, the ‘return’ statement is used to propagate results up the call stack. Each return “pops” a method call from the stack, eventually leading to the base case and unwinding the recursion.
23. What is a “block” or “compound statement,” and how is it used in control flow?
Answer: A block or compound statement is a group of statements enclosed in curly braces {}. It is used to create a single, cohesive unit of code in control flow structures like ‘if,’ ‘for,’ and ‘while’ statements.
24. What is the ‘break’ statement used for in a loop? Provide an example where it’s commonly used.
Answer: The ‘break’ statement is used to exit a loop prematurely. It’s often used in a loop that searches for a specific condition, like finding a target value in an array.
25. What is the purpose of a ‘return’ statement in a method with a ‘void’ return type?
Answer: In a method with a ‘void’ return type, the ‘return’ statement is used to exit the method without returning a value. It is often used for early method termination.
26. What is the ‘continue’ statement in Java, and how does it affect loop execution?
Answer: The ‘continue’ statement is used to skip the current iteration of a loop and proceed to the next iteration without executing the remaining code in the current iteration.
27. What are nested loops in Java, and how are they used?
Answer: Nested loops are loops within other loops. They are used for more complex repetition patterns, like iterating through 2D arrays or generating combinations.
28. Explain the concept of “infinite loops” and their impact on program execution.
Answer: Infinite loops are loops that continue indefinitely. They can lead to program crashes or unresponsiveness and are typically unintended.
29. What is the ‘break’ statement used for in a loop?
Answer: The ‘break’ statement is used to exit a loop prematurely, regardless of the loop’s condition.
30. What is the ‘continue’ statement used for in a loop?
Answer: The ‘continue’ statement is used to skip the remaining code in the current iteration of a loop and proceed to the next iteration.
31. Explain the ‘return’ statement in Java and its role in recursion.
Answer: In recursion, the ‘return’ statement is used to propagate results up the call stack. Each return “pops” a method call from the stack, eventually leading to the base case and unwinding the recursion.
32. What is the ‘assert’ statement in Java, and how is it used for debugging?
Answer: The ‘assert’ statement is used to test assumptions about program behavior. It is used for debugging and is typically enabled in development but disabled in production.
33. What is a “block” or “compound statement,” and how is it used in control flow?
Answer: A block or compound statement is a group of statements enclosed in curly braces {}. It is used to create a single, cohesive unit of code in control flow structures like ‘if,’ ‘for,’ and ‘while’ statements.
34. Explain the ‘throw’ statement in Java and its use in exception handling.
Answer: The ‘throw’ statement is used to explicitly throw an exception. It is a key component of exception handling, allowing custom exceptions to be thrown when needed.
35. What is the ‘try-catch’ block in Java, and how is it used for handling exceptions?
Answer: The ‘try-catch’ block is used to catch and handle exceptions. Code that may throw an exception is placed in the ‘try’ block, and exception handling code is placed in the ‘catch’ block.
36. What is the ‘finally’ block in Java exception handling, and how is it different from ‘try’ and ‘catch’?
Answer: The ‘finally’ block is used for code that must be executed regardless of whether an exception occurs or not. It ensures resource cleanup or finalization.
37. What is the ‘throw’ statement’s role in custom exception handling in Java?
Answer: The ‘throw’ statement is used to explicitly throw a custom exception. It allows you to create and throw user-defined exceptions when specific conditions are met.
38. Explain the ‘try-with-resources’ statement in Java, and how does it simplify resource management?
Answer: The ‘try-with-resources’ statement is used for automatic resource management. It simplifies the code for working with resources like files or streams by automatically closing them after use.
39. What is the ‘break’ label used for in nested loops, and how is it applied in Java?
Answer: The ‘break’ label is used to specify which loop to break out of when dealing with nested loops. It allows you to break out of a specific loop when multiple loops are nested.
40. What is a “labeled continue” statement in Java, and how is it used in nested loops?
Answer: A labeled continue is used to skip the current iteration of a specific loop when dealing with nested loops. It allows you to continue or skip iterations of a particular loop.
41. Explain the ‘return’ statement’s role in recursion and how it affects the call stack.
Answer: In recursive methods, the ‘return’ statement is used to propagate results up the call stack. Each return “pops” a method call from the stack, eventually leading to the base case and unwinding the recursion.
42. What is a “block” or “compound statement,” and how is it used in control flow?
Answer: A block or compound statement is a group of statements enclosed in curly braces {}. It is used to create a single, cohesive unit of code in control flow structures like ‘if,’ ‘for,’ and ‘while’ statements.
43. What is the ‘break’ statement used for in a loop?
Answer: The ‘break’ statement is used to exit a loop prematurely, regardless of the loop’s condition.
44. What is the ‘continue’ statement used for in a loop?
Answer: The ‘continue’ statement is used to skip the remaining code in the current iteration of a loop and proceed to the next iteration.
45. Explain the ‘return’ statement in Java and its role in recursion.
Answer: In recursion, the ‘return’ statement is used to propagate results up the call stack. Each return “pops” a method call from the stack, eventually leading to the base case and unwinding the recursion.
46. What is the ‘assert’ statement in Java, and how is it used for debugging?
Answer: The ‘assert’ statement is used to test assumptions about program behavior. It is used for debugging and is typically enabled in development but disabled in production.
47. What is a “block” or “compound statement,” and how is it used in control flow?
Answer: A block or compound statement is a group of statements enclosed in curly braces {}. It is used to create a single, cohesive unit of code in control flow structures like ‘if,’ ‘for,’ and ‘while’ statements.
48. Explain the ‘throw’ statement in Java and its use in exception handling.
Answer: The ‘throw’ statement is used to explicitly throw an exception. It is a key component of exception handling, allowing custom exceptions to be thrown when needed.
49. What is the ‘try-catch’ block in Java, and how is it used for handling exceptions?
Answer: The ‘try-catch’ block is used to catch and handle exceptions. Code that may throw an exception is placed in the ‘try’ block, and exception handling code is placed in the ‘catch’ block.
50. What is the ‘finally’ block in Java exception handling, and how is it different from ‘try’ and ‘catch’?
Answer: The ‘finally’ block is used for code that must be executed regardless of whether an exception occurs or not. It ensures resource cleanup or finalization.