Java Collections Framework Interview Questions
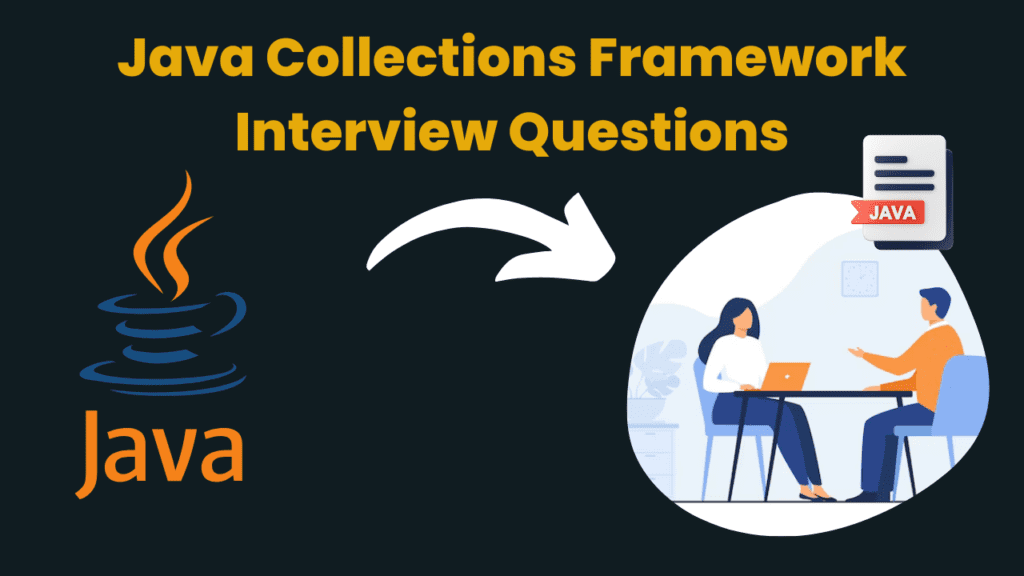
50 most frequently asked Java Collections Framework Interview Questions.
Get Discount on Top Educational Courses
1. What is the Java Collections Framework, and why is it essential in Java programming?
Answer: The Java Collections Framework is a set of classes and interfaces for working with collections of objects. It provides fundamental data structures and algorithms for organizing and manipulating data.
2. Name some core interfaces in the Java Collections Framework.
Answer: Core interfaces include List, Set, Map, Queue, and their respective subinterfaces.
3. Explain the difference between a List and a Set in the Collections Framework.
Answer: A List allows duplicate elements and maintains order, while a Set does not allow duplicates and does not guarantee order.
4. What is the purpose of the ‘Collection’ interface, and what methods does it define?
Answer: The Collection interface is the root interface in the Collections Framework. It defines methods for basic collection operations, like adding, removing, and iterating over elements.
Syntax for adding an element to a Collection:
collection.add(element);
5. Name a class that implements the ‘Set’ interface in the Collections Framework.
Answer: HashSet, TreeSet, and LinkedHashSet are classes that implement the Set interface.
Syntax for creating a HashSet:
Set set = new HashSet<>();
6. Explain the ‘Map’ interface and its key-value association.
Answer: The Map interface defines a collection that holds key-value pairs. Each key is associated with a value, and keys are unique within the map.
Syntax for putting a key-value pair in a Map:
map.put(key, value);
7. What is the difference between ‘HashMap’ and ‘Hashtable’ in the Collections Framework?
Answer: HashMap is not synchronized and allows null keys and values, while Hashtable is synchronized and does not allow null keys or values.
8. Explain the ‘List’ interface and name a few classes that implement it.
Answer: The List interface represents an ordered collection of elements with duplicates allowed. Classes that implement List include ArrayList, LinkedList, and Vector.
9. What is the ‘Queue’ interface in the Collections Framework, and how is it different from a List?
Answer: The Queue interface represents a collection that orders elements for processing, typically using a “first-in, first-out” (FIFO) order. It is different from a List, which can maintain arbitrary ordering.
Syntax for adding an element to a Queue:
queue.add(element);
10. What is the ‘Deque’ interface, and how does it differ from a Queue?
Answer: The Deque (Double-ended Queue) interface extends Queue and allows elements to be added or removed from both ends, making it more versatile than a standard Queue.
Syntax for adding an element to the front of a Deque:
deque.addFirst(element);
11. Explain the ‘Iterator’ interface and its role in traversing collections.
- Answer: The Iterator interface is used to traverse collections, providing methods like ‘next()’ and ‘hasNext()’ to access elements sequentially.
Syntax for using an Iterator to traverse a Collection:
Iterator iterator = collection.iterator();
while (iterator.hasNext()) {
Type element = iterator.next();
// Process element
}
12. What is an “unmodifiable” or “immutable” collection, and why is it useful?
Answer: An unmodifiable collection is one that cannot be modified after creation. It is useful for creating read-only views of collections, ensuring data integrity.
Syntax for creating an unmodifiable List:
List unmodifiableList = Collections.unmodifiableList(list);
13. Explain the ‘Comparable’ interface and its role in sorting elements.
Answer: The Comparable interface is used to define natural ordering for objects. Classes that implement Comparable can be sorted using methods like ‘Collections.sort()’ and ‘Arrays.sort()’.
Syntax for implementing Comparable in a class:
class MyClass implements Comparable {
@Override
public int compareTo(MyClass other) {
// Implement comparison logic
}
}
14. What is the purpose of the ‘Comparator’ interface in the Collections Framework?
- Answer: The Comparator interface allows for custom sorting of objects by providing a comparison mechanism that can be applied to classes that don’t implement Comparable.
Syntax for using a custom Comparator for sorting:
Collections.sort(list, customComparator);
15. What is the ‘Collections’ class in Java, and what utility methods does it offer for collections?
Answer: The Collections class is a utility class that provides various methods for working with collections, such as sorting, searching, and synchronization.
16. Explain the ‘hashCode’ and ‘equals’ methods in the context of using objects as keys in a Map.
Answer: The ‘hashCode’ method generates a hash code for an object, and the ‘equals’ method is used to compare objects for equality. Both are essential for correct key-value retrieval in a Map.
17. What is the ‘HashSet’ class in the Collections Framework, and how does it store elements?
Answer: HashSet is an implementation of the Set interface that uses a hash table for storing elements. It does not guarantee order and does not allow duplicate elements.
18. What is the ‘ArrayList’ class, and how does it differ from an array in Java?
Answer: ArrayList is a dynamic array implementation, which means it can grow or shrink dynamically, unlike a traditional array with a fixed size.
19. Explain the ‘TreeSet’ class in the Collections Framework and its characteristics.
Answer: TreeSet is an implementation of the Set interface that stores elements in sorted order. It uses a red-black tree to maintain the sorted structure.
20. What is the ‘LinkedList’ class in the Collections Framework, and how does it differ from an ArrayList?
Answer: LinkedList is a doubly-linked list implementation, which provides efficient insertions and deletions but slower random access compared to an ArrayList.
21. Explain the ‘HashMap’ class in the Collections Framework, and how does it store key-value pairs?
Answer: HashMap is an implementation of the Map interface that uses a hash table to store key-value pairs. It offers fast access and retrieval based on key hashes.
22. What is the ‘Hashtable’ class, and what are its main characteristics?
Answer: Hashtable is a synchronized implementation of the Map interface. It ensures thread-safety but is less efficient than HashMap.
23. What is the ‘Vector’ class, and how does it differ from an ArrayList?
Answer: Vector is a synchronized version of an ArrayList. It ensures thread-safety but may be slower due to synchronization.
24. Explain the ‘PriorityQueue’ class in the Collections Framework, and what ordering does it use?
Answer: PriorityQueue is an implementation of the Queue interface that orders elements based on their natural ordering (comparable) or a custom comparator.
25. What is the ‘ConcurrentHashMap’ class, and why is it useful for multithreaded applications?
Answer: ConcurrentHashMap is a concurrent implementation of the Map interface that allows multiple threads to access and modify it concurrently, with high performance and safety.
26. Explain the ‘Arrays’ class in Java and its utility methods for working with arrays.
Answer: The Arrays class provides various static methods for working with arrays, including sorting, searching, and converting arrays to collections.
27. What is the purpose of the ‘CopyOnWriteArrayList’ class, and how does it work in multithreaded environments?
Answer: CopyOnWriteArrayList is a list implementation that ensures thread-safety by creating a new copy of the list whenever an element is modified. It is useful when reads are much more frequent than writes.
28. Explain the ‘BlockingQueue’ interface in the context of concurrent programming.
Answer: The BlockingQueue interface represents a thread-safe queue that provides blocking operations for managing concurrent access to elements.
29. What is the ‘WeakHashMap’ class, and how does it handle weak references to keys?
Answer: WeakHashMap is an implementation of the Map interface that uses weak references for keys. Keys can be collected by the garbage collector if no strong references are held.
30. Explain the ‘EnumSet’ class in the Collections Framework and its use with enums.
Answer: EnumSet is a specialized Set implementation for use with enums. It is highly efficient and takes advantage of enum’s characteristics.
31. Explain the ‘WeakHashMap’ class, and how does it handle weak references to keys?
Answer: WeakHashMap is an implementation of the Map interface that uses weak references for keys. Keys can be collected by the garbage collector if no strong references are held.
32. What is the ‘EnumSet’ class in the Collections Framework, and how is it used with enums?
Answer: EnumSet is a specialized Set implementation for use with enums. It is highly efficient and takes advantage of enum’s characteristics.
33. Explain the ‘NavigableSet’ interface in the Java Collections Framework and its key features.
Answer: NavigableSet extends the Set interface and provides navigation methods for traversing elements in a sorted order. It offers methods for floor, ceiling, lower, and higher elements.
34. What is the ‘ConcurrentSkipListSet’ class, and how does it provide concurrent access to a sorted set?
Answer: ConcurrentSkipListSet is a concurrent implementation of the NavigableSet interface that allows multiple threads to access and modify it concurrently while maintaining a sorted order.
35. Explain the ‘BlockingQueue’ interface and its role in multithreaded programming.
Answer: The BlockingQueue interface represents a thread-safe queue with blocking operations for managing concurrent access to elements, making it useful in multithreaded environments.
36. What is the ‘LinkedBlockingQueue’ class, and how does it differ from other blocking queue implementations?
Answer: LinkedBlockingQueue is a concurrent implementation of the BlockingQueue interface that uses a linked node structure. It is unbounded, meaning it can hold an unlimited number of elements.
37. Explain the ‘CopyOnWriteArrayList’ class, its use in multithreaded scenarios, and its characteristics.
Answer: CopyOnWriteArrayList is a thread-safe list implementation that creates a new copy of the list whenever an element is modified. It is useful when reads are much more frequent than writes.
38. What is the ‘ConcurrentLinkedQueue’ class, and how does it handle concurrent access in a queue?
Answer: ConcurrentLinkedQueue is a concurrent implementation of the Queue interface that provides high-performance, non-blocking, and thread-safe operations for managing elements in a queue.
39. Explain the ‘ConcurrentHashMap’ class, its role in concurrent programming, and its key features.
Answer: ConcurrentHashMap is a concurrent implementation of the Map interface that allows multiple threads to access and modify it concurrently with high performance and safety.
40. What is the ‘EnumMap’ class, and how is it used to associate keys with enums in a Map?
Answer: EnumMap is a specialized Map implementation for use with enums as keys. It is highly efficient, and each key is associated with a specific enum.
41. Explain the ‘BitSet’ class in the Java Collections Framework and its use for managing sets of bits.
Answer: BitSet is a class for managing sets of bits. It provides methods for setting, clearing, and testing individual bits and supports various bitwise operations.
42. What is the ‘ArrayDeque’ class, and how does it implement a double-ended queue (deque)?
Answer: ArrayDeque is a resizable array-based implementation of the Deque (double-ended queue) interface. It allows elements to be added or removed from both ends, making it versatile.
43. What is the ‘IdentityHashMap’ class, and how does it differ from other Map implementations?
Answer: IdentityHashMap is a specialized Map implementation that uses reference equality for keys, making it distinct from other Map implementations that use object equality.
44. Explain the ‘LinkedHashSet’ class and its characteristics in maintaining a predictable order.
Answer: LinkedHashSet is an implementation of the Set interface that maintains order based on the order in which elements were inserted. It combines the features of HashSet and LinkedHashSet.
45. What is the ‘Properties’ class, and how is it used for managing key-value pairs in a Java properties file?
Answer: The Properties class is used to manage key-value pairs in a properties file. It is often used for configuration settings and can load and store properties to and from files.
46. Explain the ‘IdentityHashMap’ class, its use cases, and its distinctive behavior with key comparisons.
Answer: IdentityHashMap is a Map implementation that uses reference equality (==) for key comparisons rather than object equality (equals()). It is useful for cases where reference identity matters.
47. What is the ‘TreeMap’ class in the Java Collections Framework, and how does it provide sorted key-value mappings?
Answer: TreeMap is an implementation of the Map interface that stores key-value pairs in sorted order based on the keys. It uses a red-black tree for efficient maintenance.
48. Explain the ‘Spliterator’ interface and its role in iterating and partitioning elements in a collection.
Answer: The Spliterator interface provides a more flexible way to traverse and partition elements in a collection, allowing for parallel processing in addition to sequential iteration.
49. What is the ‘Collections.unmodifiable’ methods, and how are they used to create read-only views of collections?
Answer: The ‘Collections.unmodifiable’ methods create unmodifiable (read-only) views of collections, preventing modifications while allowing access to the original data.
50. Explain the ‘Collections.checked’ methods, their purpose, and how they help ensure type-safety in collections.
Answer: The ‘Collections.checked’ methods create type-safe views of collections. They are used to detect and prevent runtime class-cast exceptions when adding elements of the wrong type.
Get Discount on Top Educational Courses
These questions cover various aspects of the Java Collections Framework, including different collection types, concurrent collections, and specialized implementations.