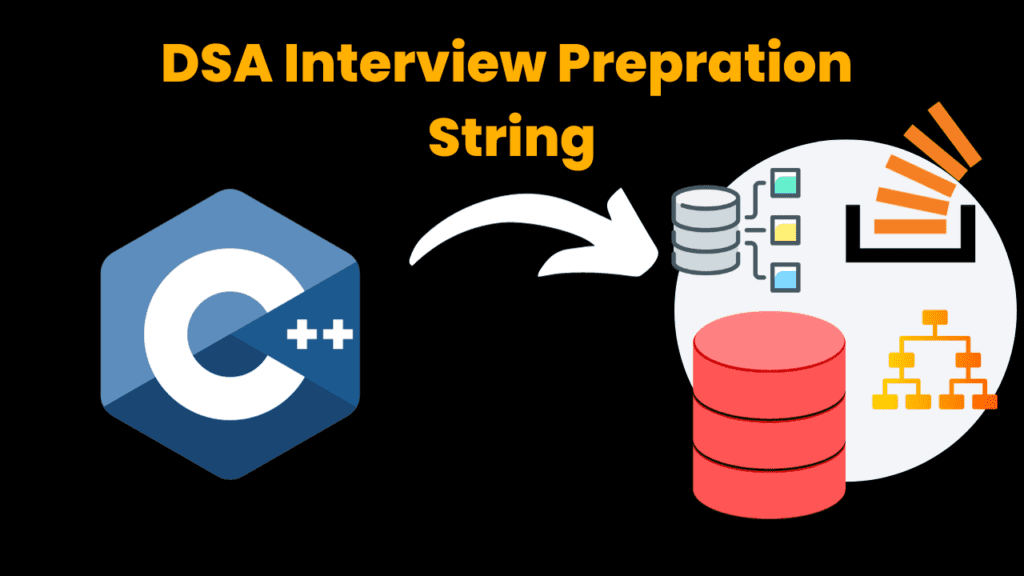
50 most frequently asked String interview questions.
1. What is a C++ string?
Answer: A C++ string is a sequence of characters represented as an object of the std::string
class, part of the C++ Standard Library.
2. How do you declare and initialize a C++ string?
Answer: You can declare and initialize a C++ string using the std::string
class constructor or by assigning a string literal.
std::string str1 = "Hello, World!";
std::string str2("C++ Strings");
3. What is the difference between C-style strings and C++ strings?
Answer: C-style strings are arrays of characters terminated by a null character ('\0'
), while C++ strings are objects that manage their own memory and provide various methods for string manipulation.
4. How do you find the length of a C++ string?
Answer: You can find the length of a C++ string using the length()
or size()
member functions.
std::string str = "C++";
int length = str.length(); // or str.size()
5. Explain string concatenation in C++.
Answer: String concatenation in C++ can be performed using the +
operator or the append()
or +=
methods.
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2; // or str1.append(str2);
6. How can you access individual characters of a C++ string?
Answer: You can access individual characters of a C++ string using the []
operator or the at()
member function.
std::string str = "C++";
char firstChar = str[0]; // or char firstChar = str.at(0);
7. What is the difference between []
and at()
for accessing characters in a C++ string?
Answer: The []
operator does not perform bounds checking, while at()
checks bounds and throws an exception if out of range.
8. How do you convert a C++ string to a C-style string and vice versa?
Answer: To convert a C++ string to a C-style string, you can use the c_str()
method. To convert a C-style string to a C++ string, you can use the std::string
constructor.
std::string cppString = "C++";
const char* cString = cppString.c_str();
const char* cString = "C";
std::string cppString(cString);
9. What is the purpose of the find()
method in C++ strings?
Answer: The find()
method is used to search for a substring within a C++ string and returns the position of the first occurrence.
10. How can you check if a C++ string starts or ends with a specific substring?
Answer: You can use the substr()
method along with find()
to check if a string starts or ends with a specific substring.
11. Explain the concept of string comparison in C++.
Answer: String comparison in C++ can be done using operators like ==
, !=
, <
, <=
, >
, and >=
. These operators compare strings lexicographically.
12 .How do you convert a string to uppercase or lowercase in C++?
Answer: You can convert a string to uppercase using the toupper()
function from the <cctype>
header or loop through the string and use std::toupper()
. For lowercase, use tolower()
or std::tolower()
.
13. What is string slicing, and how is it done in C++?
Answer: String slicing involves extracting a portion of a string. In C++, you can use the substr()
method to achieve this.
std::string str = "Hello, World!";
std::string slice = str.substr(0, 5); // Extracts "Hello"
14.How do you replace a substring within a C++ string?
Answer: You can use the replace()
method to replace a substring with another string.
std::string str = "I like pizza.";
str.replace(7, 5, "hamburgers"); // Replaces "pizza" with "hamburgers"
15. What is the getline()
function, and how is it used in C++?
Answer: getline()
is used to read a line from an input stream (e.g., std::cin
) and store it in a C++ string.
std::string line;
std::getline(std::cin, line);
16. How can you check if a string contains a specific character or substring in C++?
Answer: You can use the find()
method to check if a string contains a specific substring or character and see if the returned position is valid.
17. Explain the concept of string splitting in C++.
Answer: String splitting involves breaking a string into multiple substrings based on a delimiter. You can use libraries like std::istringstream
or custom functions to achieve this.
18. What are string streams (istringstream
, ostringstream
) in C++ used for?
Answer: String streams are used for input and output operations on strings. istringstream
is used for reading from a string, while ostringstream
is used for writing to a string.
19. What is the std::stringstream
class in C++?
Answer: std::stringstream
is a C++ class that allows you to perform both input and output operations on a string stream. It’s useful for parsing and formatting string data.
20. How do you remove whitespace from the beginning and end of a C++ string?
Answer: You can use the std::string
member functions erase()
and find_first_not_of()
to remove leading and trailing whitespace.
21. What is the difference between push_back()
and pop_back()
for C++ strings?
Answer: push_back()
is used to append a character to the end of a string, while pop_back()
removes the last character from the string.
22. How can you check if a string is a palindrome in C++?
Answer: To check if a string is a palindrome, compare it with its reverse. If they are the same, the string is a palindrome.
23. Explain the concept of string hashing and its applications.
Answer: String hashing involves converting a string into a numeric hash value. It is used in data structures like hash tables for efficient string-based lookups.
24. What is string interpolation, and how can it be achieved in C++?
Answer: String interpolation is the process of embedding variables or expressions within a string. In C++, you can achieve it using the +
operator or using string streams (std::stringstream
).
25. How can you efficiently reverse words in a sentence using C++ strings?
Answer: To reverse words in a sentence, you can tokenize the sentence using a delimiter (e.g., space), reverse the order of the tokens, and then concatenate them back together.
26. Explain the concept of string encoding and character sets in C++.
Answer: String encoding refers to representing characters as binary data. Character sets define the mapping between characters and their binary representations (e.g., ASCII, UTF-8).
27. How do you count the occurrences of a substring within a C++ string?
Answer: You can count the occurrences of a substring in a C++ string using a loop and the find()
method repeatedly until it returns std::string::npos
.
28. What are the string-related functions available in the C++ Standard Library (STL)?
Answer: The C++ Standard Library provides functions for common string operations, including std::to_string()
, std::stoi()
, std::stof()
, and std::stod()
for string conversion, as well as std::getline()
for reading lines.
29. How can you efficiently remove duplicate characters from a C++ string?
Answer: To remove duplicate characters from a string, you can use a set or an array to keep track of seen characters and rebuild the string without duplicates.
30. What is the concept of string matching, and how can it be done in C++?
Answer: String matching involves finding the occurrence of one string (the pattern) within another string (the text). C++ provides various methods for string matching, including the find()
method, regular expressions, and algorithms like the Knuth-Morris-Pratt (KMP) algorithm.
31. Explain the concept of string tokenization in C++.
Answer: String tokenization is the process of splitting a string into smaller tokens based on a delimiter. You can use libraries like std::istringstream
or custom functions to tokenize a string.
32. What are string literals, and how are they used in C++?
Answer: String literals are sequences of characters enclosed in double quotes. They are used to represent constant strings in C++ code.
const char* str = "Hello, World!";
33. How can you efficiently reverse the characters within words of a sentence in C++?
Answer: To reverse characters within words of a sentence, you can tokenize the sentence, reverse each word, and then concatenate them back together.
34. What are the differences between string
and wstring
in C++?
Answer: string
is used for ASCII character strings, while wstring
is used for wide-character strings (e.g., Unicode). wstring
uses a larger character size to accommodate a broader range of characters.
35. How do you efficiently check if two strings are anagrams in C++?
Answer: To check if two strings are anagrams, compare the sorted versions of both strings. If they are equal, the strings are anagrams.
36. Explain the concept of string encoding conversion in C++.
Answer: String encoding conversion involves converting a string from one character encoding to another (e.g., from UTF-8 to UTF-16). C++ provides libraries like std::wstring_convert
and iconv
for encoding conversions.
37. What are the differences between string
and stringstream
in C++?
Answer: string
is used to store and manipulate strings, while stringstream
is used for formatted input and output operations on strings. stringstream
allows you to treat a string as a stream for parsing and formatting.
38. How do you check if a C++ string is empty?
Answer: You can check if a C++ string is empty using the empty()
member function or by comparing it to an empty string (""
).
std::string str = "Hello";
bool isEmpty = str.empty(); // false
std::string emptyStr = "";
bool isEmpty2 = (emptyStr == ""); // true
39. Explain the concept of string hashing collisions and how they are resolved.
Answer: String hashing collisions occur when two different strings produce the same hash value. Collisions are typically resolved by using techniques such as chaining (using linked lists at each hash bucket) or open addressing (finding the next available slot).
40. How can you efficiently count the number of words in a sentence using C++ strings?
Answer: To count the number of words in a sentence, tokenize the sentence and count the tokens.
41. What are string views (std::string_view
) in C++ used for?
Answer: String views are lightweight non-owning references to strings, allowing efficient access to substrings without copying. They are used for read-only operations and are part of C++17 and later.
42. Explain the concept of string manipulation in C++.
Answer: String manipulation involves performing various operations on strings, such as concatenation, trimming, searching, and modifying.
43. How do you find the first non-repeating character in a C++ string efficiently?
Answer: You can find the first non-repeating character in a string by using a hash table to count character frequencies and then iterating through the string to find the first character with a frequency of 1.
44. What are the different ways to iterate through the characters of a C++ string?
Answer: You can iterate through a C++ string using a range-based for loop, a regular for loop with an index, or an iterator.
45. Explain the concept of string formatting in C++ and its common tools.
Answer: String formatting involves creating strings with placeholders that are filled with values at runtime. Common tools for string formatting in C++ include printf
-style formatting, sprintf
, and libraries like fmtlib
.
46. How can you efficiently check if a C++ string is a valid number (integer or floating-point)?
Answer: You can check if a string is a valid number by attempting to convert it using functions like std::stoi()
(for integers) and std::stof()
or std::stod()
(for floating-point numbers) and handling exceptions if the conversion fails.
47. What are regular expressions, and how are they used for string matching in C++?
Answer: Regular expressions are patterns used for string matching. C++ provides the <regex>
library, allowing you to use regular expressions for powerful string manipulation.
48. Explain the concept of string hashing functions and their importance in C++.
Answer: String hashing functions convert a string into a numeric hash value. They are important for fast data retrieval in data structures.
49. How can you efficiently rotate the characters in a C++ string to the left or right?
Answer: You can efficiently rotate characters in a string by using a combination of substrings and concatenation based on the desired rotation direction.
50. What are string copy constructors and assignment operators in C++?
Answer: String copy constructors and assignment operators are used to create copies of strings. The copy constructor creates a new string that is a copy of an existing one, while the assignment operator assigns the value of one string to another.
These 50 string-related topics and questions cover a wide range of concepts and are valuable for interview preparation in C++. Make sure to practice solving problems and implementing these concepts to solidify your understanding.