Control Structures Interview Questions
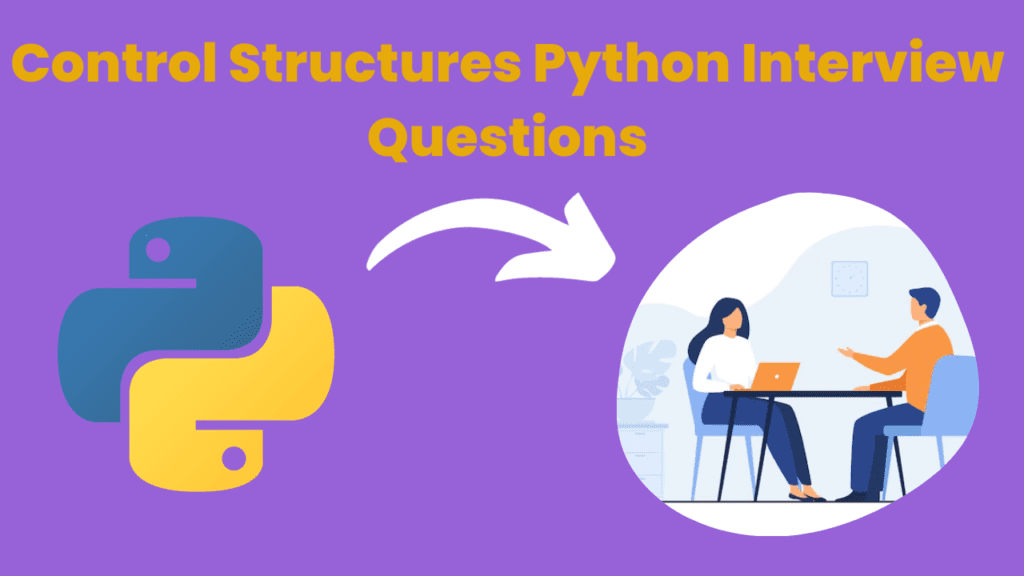
50 most frequently asked Control Structures interview questions.
Get Discount on Top Educational Courses
1. Describe Python’s if-elif-else statement.
Answer: The if-elif-else
statement is used for conditional branching in Python. It allows you to specify multiple conditions and execute different code blocks based on the first condition that evaluates to True
.
if condition1:
# Code block to execute if condition1 is True
elif condition2:
# Code block to execute if condition2 is True
else:
# Code block to execute if none of the conditions are True
2. What is a for loop, and how does it work in Python?
Answer: A for
loop in Python is used for iterating over a sequence (such as a list, tuple, or string) or any iterable object. It executes a block of code for each item in the sequence.
for item in iterable:
# Code block to execute for each item in the iterable
3. Explain the while loop and give an example of its usage.
Answer: A while
loop in Python is used for repeated execution of a block of code as long as a specified condition is True
. Here’s an example:
count = 0
while count < 5:
print(count)
count += 1
4. How do you write an if statement with multiple conditions in Python?
Answer: You can use if
, elif
, and else
to handle multiple conditions in an if-elif-else statement.
if condition1:
# Code block to execute if condition1 is True
elif condition2:
# Code block to execute if condition2 is True
else:
# Code block to execute if none of the conditions are True
5. What is the purpose of the else
clause in an if-elif-else statement?
Answer: The else
clause is executed when none of the preceding conditions in an if-elif-else statement is True
.
if condition1:
# Code block to execute if condition1 is True
elif condition2:
# Code block to execute if condition2 is True
else:
# Code block to execute if none of the conditions are True
6. Explain the concept of nested loops in Python.
Answer: Nested loops are loops within loops. They are used for more complex iterations, such as iterating over elements in a 2D list.
for i in range(3):
for j in range(3):
# Code block to execute for each combination of i and j
7. How can you exit a loop prematurely in Python?
Answer: You can use the break
statement to exit a loop prematurely when a specific condition is met.
7. How can you exit a loop prematurely in Python?
Answer: You can use the break statement to exit a loop prematurely when a specific condition is met.
8. What is the continue
statement used for in Python loops?
Answer: The continue
statement is used to skip the current iteration of a loop and continue with the next iteration.
for item in iterable:
if condition:
continue # Skip the current iteration and continue with the next one
9. Describe the range()
function in Python and its usage in for loops.
Answer: range()
generates a sequence of numbers. It’s often used in for
loops to specify the number of iterations.
for i in range(start, stop, step):
# Code block to execute for each value of i in the range
10. How do you iterate over the elements and their indices in a list using a for loop?
Answer: You can use the enumerate()
function to iterate over elements and their indices in a list using a for
loop.
for index, item in enumerate(iterable):
# Code block to execute for each item and its index
11. What is an infinite loop in Python, and how can you break out of it? – An infinite loop is a loop that continues to execute without stopping. You can break out of it using the break
statement.
12. Explain the use of the pass
statement in Python loops. – The pass
statement is a no-op and is often used as a placeholder when syntactically some code is required but no action is needed in a loop.
13. How do you iterate over the keys and values in a dictionary using a for loop? – You can use the .items()
method to iterate over key-value pairs in a dictionary using a for
loop.
14. What is the purpose of the else
block in a while loop in Python? – The else
block in a while
loop is executed when the loop’s condition becomes False
.
15. How can you simulate a do-while loop in Python? – You can simulate a do-while loop using a while
loop with a conditional check at the end.
16. Explain the concept of a loop control variable. – A loop control variable is a variable used to control the flow and behavior of a loop.
17. What is the role of the in
keyword in Python loops? – The in
keyword is used to iterate over elements in an iterable, such as a list or string.
18. How do you find the maximum and minimum values in a list using loops in Python? – You can use a for
loop to iterate over the list and keep track of the maximum and minimum values.
19. What are nested loops, and when are they commonly used? – Nested loops are loops within loops. They are used when you need to iterate over multiple dimensions, like rows and columns of a 2D array.
20. Explain the use of the range()
function with for
loops in Python. – range()
generates a sequence of numbers, often used as an iterable in for
loops to specify the number of iterations.
21. How can you avoid an infinite loop while waiting for user input in a Python program? – You can include a conditional check for user input and use the break
statement to exit the loop when a specific input is received.
22. Describe the difference between for
and while
loops, and when should each be used? – for
loops are used when you know the number of iterations in advance, while while
loops are used when you don’t know the exact number of iterations and want to loop until a condition is met.
23. How do you use the enumerate()
function in a for
loop in Python? – enumerate()
returns an iterable of tuples containing the index and value of each element, which can be used in a for
loop to iterate over elements and their indices.
24. Explain the purpose of the break
statement in Python loops. – The break
statement is used to exit a loop prematurely when a specific condition is met.
25. How do you create a loop that iterates over a list in reverse order in Python? – You can use slicing with a negative step size in a for
loop to iterate over a list in reverse order.
26. What is the purpose of the continue
statement in Python loops? – The continue
statement is used to skip the current iteration of a loop and continue with the next iteration.
27. How can you iterate over characters in a string using a for
loop in Python? – You can use a for
loop to iterate over characters in a string as if it were a list of characters.
28. Explain the difference between a loop and a recursive function in Python. – A loop is an iterative construct, while a recursive function calls itself to solve a problem.
29. What is an infinite loop, and how can you avoid it in Python? – An infinite loop is a loop that runs indefinitely. You can avoid it by ensuring that there’s a condition or a way to exit the loop.
30. How do you iterate over the elements of a list in reverse order using a for
loop in Python? – You can use the reversed()
function in a for
loop to iterate over the elements of a list in reverse order.
my_list = [1, 2, 3, 4, 5]
for item in reversed(my_list):
# Code block to iterate over elements in reverse order
print(item)
31. Explain the concept of an iterator and give an example in Python.
Answer: An iterator is an object that can be iterated (looped) over. An example is the use of the iter()
and next()
functions to create and iterate over an iterator.
my_list = [1, 2, 3, 4, 5]
my_iterator = iter(my_list) # Create an iterator from a list
while True:
try:
item = next(my_iterator)
# Code block to process each item in the iterator
print(item)
except StopIteration:
break # Exit the loop when the iterator is exhausted
32. How can you skip a specific iteration in a for loop without terminating the loop in Python?
Answer: You can use the continue
statement to skip a specific iteration and continue with the next one in a for
loop.
my_list = [1, 2, 3, 4, 5]
for item in my_list:
if item == 3:
continue # Skip iteration when item is 3
# Code block to process items (except when item is 3)
print(item)
33. Describe the range()
function with three arguments in Python.
Answer: The range()
function with three arguments allows you to specify the start, stop, and step values for generating a sequence of numbers.
for i in range(start, stop, step):
# Code block to execute for each value of i in the range
34. What is the purpose of the pass
statement in Python loops?
Answer: The pass
statement is used as a placeholder when syntactically some code is required, but no action is needed in a loop.
for item in iterable:
if condition:
pass # Placeholder for future code
# Code block to process items
35. How can you use a for loop to iterate over a dictionary’s keys and values in Python?
Answer: You can use the .items()
method to iterate over both keys and values in a dictionary within a for
loop.
my_dict = {'a': 1, 'b': 2, 'c': 3}
for key, value in my_dict.items():
# Code block to process each key and value in the dictionary
print(f"Key: {key}, Value: {value}")
36. What is the role of an iterable in a for
loop, and how can you create a custom iterable in Python? – An iterable is an object that can be looped over in a for
loop. You can create a custom iterable by implementing the __iter__()
and __next__()
methods in a class.
37. Explain the purpose of the else
block in a for
loop in Python. – The else
block in a for
loop is executed after the loop completes its iterations and is not interrupted by a break
statement.
38. How can you use the zip()
function in Python to iterate over multiple iterables simultaneously? – You can use the zip()
function to combine multiple iterables and iterate over them simultaneously in a for
loop.
39. Describe the range()
function with one argument in Python. – The range()
function with one argument generates a sequence of numbers starting from 0 up to, but not including, the specified value.
40. How do you create an infinite loop in Python, and when might you use one? – You can create an infinite loop using while True:
. Infinite loops are used in situations where you need continuous execution, such as server programs.
41. What is the purpose of the for-else
construct in Python? – The for-else
construct allows you to specify code to execute if a for
loop completes without encountering a break
statement.
42. How can you control the flow of a loop using the continue
statement in Python? – The continue
statement allows you to skip the current iteration and continue with the next iteration in a loop.
43. What is the difference between a loop and a conditional statement in Python? – A loop is used for repetitive tasks, while a conditional statement is used for making decisions based on conditions.
44. Explain the purpose of the break
statement in Python loops. – The break
statement is used to exit a loop prematurely when a specific condition is met.
45. How can you use the while
loop to iterate over elements in a list in Python? – You can use a while
loop with an index variable to iterate over elements in a list.
46. What is the enumerate()
function in Python, and how is it used in loops? – The enumerate()
function adds an index to an iterable and is commonly used in for
loops to iterate over elements and their indices.
47. Explain the use of the in
operator in Python loops. – The in
operator is used to check if an element is present in an iterable and is commonly used in loops for membership testing.
48. How do you find the sum of elements in a list using a for
loop in Python? – You can use a for
loop to iterate over the elements of a list and accumulate their values to find the sum.
49. Describe the range()
function with two arguments in Python. – The range()
function with two arguments generates a sequence of numbers starting from the first argument and up to, but not including, the second argument.
50. How do you create an infinite loop that terminates on user input in Python? – You can create an infinite loop that terminates when specific user input is received by using the break
statement to exit the loop.