Basic Python Interview Questions
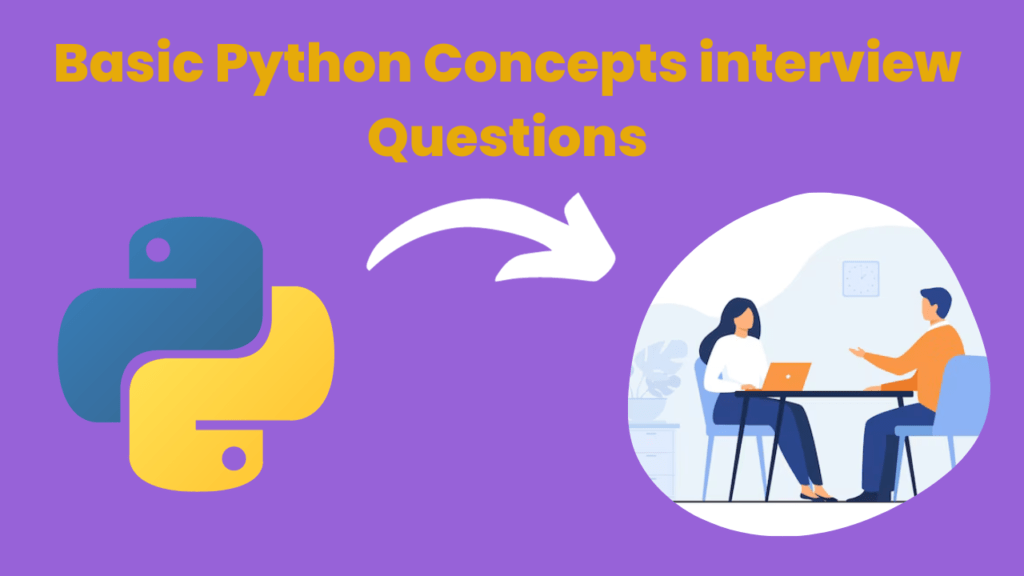
50 most frequently asked Basic Python interview questions.
1. What is Python, and why is it popular?
Answer: Python is a high-level programming language known for its simplicity and readability. It’s popular due to its versatility, extensive libraries, and large community support.
2. Explain the differences between Python 2 and Python 3.
Answer: Python 2 is legacy, while Python 3 is the present and future. Key differences include print statements, Unicode handling, and division behavior.
3. How do you install Python on your machine?
Answer: Download the Python installer from the official website (python.org) and run it. Follow the installation prompts.
4. What are Python data types? Give examples.
Answer: Python has various data types:
int
: Integer data type represents whole numbers.- Example:
5
- Example:
float
: Float data type represents numbers with a decimal point.- Example:
3.14
- Example:
str
: String data type represents text.- Example:
'hello'
- Example:
bool
: Boolean data type represents truth values, eitherTrue
orFalse
.- Example:
True
- Example:
None
: NoneType represents the absence of a value.- Example:
None
- Example:
5. Explain the concept of dynamic typing in Python.
Answer: In Python, variables are not statically typed; their types can change dynamically based on the assigned value.
6. What is the difference between a list and a tuple?
Answer: Lists are mutable (modifiable), and tuples are immutable (cannot be changed after creation).
7. How do you comment on a Python code line?
Answer: Use the #
symbol to add a single-line comment. Multi-line comments can be enclosed in triple-quotes ('''
or """
).
8. Explain the difference between local and global variables.
Answer: Local variables are defined within a specific function and have local scope. Global variables are defined outside functions and have global scope.
9. What is PEP 8, and why is it important?
Answer: PEP 8 is the Python Enhancement Proposal that provides coding style guidelines for Python. Following PEP 8 enhances code readability and consistency.
10. What is the purpose of indentation in Python code?
Answer: Indentation is used to define block structures and is essential for code readability. It replaces braces or brackets found in other languages.
11. How can you check the version of Python installed on your machine?
Answer: Use the python --version
or python -V
command to check the Python version in the command line.
12. Explain what a boolean data type represents in Python.
Answer: The boolean data type represents truth values, either True
or False
, and is often used for conditional statements.
13. What is a module in Python?
Answer: A module is a file containing Python code that can be imported and reused in other Python programs.
14. How do you create and use a virtual environment in Python?
Answer: Use python -m venv venv_name
to create a virtual environment and source venv_name/bin/activate
to activate it.
15. Describe the purpose of the elif
keyword in Python.
Answer: elif
is short for “else if” and is used to specify alternative conditions in an if-elif-else
statement.
16. What is the purpose of the len()
function in Python?
Answer: The len()
function is used to get the length (number of items) of an iterable, such as a string, list, or tuple.
17. Explain the use of the range()
function in Python.
Answer: The range()
function generates a sequence of numbers and is often used for looping.
18. How can you swap the values of two variables in Python without using a temporary variable?
Answer: You can swap values using tuple unpacking: a, b = b, a
.
19. What is the purpose of the break
statement in Python loops?
Answer: break
is used to exit a loop prematurely when a specific condition is met.
20. What is the purpose of the continue
statement in Python loops?
Answer: continue
is used to skip the current iteration and move to the next one in a loop.
21. Explain the difference between and
and or
logical operators in Python.
Answer: and
returns True
if both operands are True
, while or
returns True
if at least one operand is True
.
22. How do you convert a string to uppercase or lowercase in Python?
Answer: Use the upper()
method for uppercase and lower()
method for lowercase conversion.
23. What is a docstring, and how is it used in Python?
Answer: A docstring is a string literal used for documenting functions, classes, or modules. It’s often placed at the beginning of these entities.
24. What are the arithmetic operators in Python?
Answer: Arithmetic operators include +
(addition), -
(subtraction), *
(multiplication), /
(division), and %
(modulo).
25. Explain the purpose of the if __name__ == "__main__":
statement in Python scripts.
Answer: It allows you to run code only if the Python script is executed directly, not when it’s imported as a module.
26. How do you round a floating-point number to a specific number of decimal places in Python?
Answer: Use the round()
function to round a floating-point number to a specified number of decimal places.
27. What is the purpose of the with
statement in Python?
Answer: The with
statement is used for context management, ensuring resources are properly acquired and released.
28. Explain the purpose of the ord()
and chr()
functions in Python.
Answer: ord()
returns the Unicode code point of a character, while chr()
returns the character from a Unicode code point.
29. How do you check if a value exists in a list in Python?
Answer: Use the in
keyword to check if a value is present in a list.
30. What is a generator expression in Python, and how is it different from a list comprehension?
Answer: A generator expression is similar to a list comprehension but generates values lazily, conserving memory.
31. Explain the purpose of the map()
function in Python.
Answer: The map()
function applies a specified function to each item in an iterable and returns an iterator of the results.
32. How do you remove leading and trailing whitespace from a string in Python?
Answer: Use the strip()
method to remove both leading and trailing whitespace.
33. What is the purpose of the pop()
method for lists in Python?
Answer: The pop()
method removes and returns the last element from a list.
34. Explain the purpose of a set in Python.
Answer: A set is an unordered collection of unique elements, often used for operations like set intersection or union.
35. What is an exception in Python?
Answer: An exception is an event that occurs during the execution of a program and disrupts the normal flow of instructions.
36. How do you handle exceptions in Python using try and except blocks?
Answer: Use a try
block to enclose code that may raise an exception and an except
block to handle that exception.
37. What is a lambda function, and why is it used?
Answer: A lambda function is a small, anonymous function defined using the lambda
keyword. It’s often used for short, simple operations.
38. How do you open a file in Python, and what modes can you use for file opening?
Answer: Use the open()
function with the desired file name and mode (e.g., ‘r’ for read, ‘w’ for write).
39. What is the purpose of the zip()
function in Python?
Answer: zip()
is used to combine two or more iterables (e.g., lists) into a single iterable of tuples.
40. Explain the purpose of list slicing in Python.
Answer: List slicing allows you to extract a portion of a list by specifying start, end, and step values.
41. How do you check if a key exists in a dictionary in Python?
Answer: Use the in
keyword to check if a key is present in a dictionary.
42. What is the difference between shallow copy and deep copy in Python?
Answer: A shallow copy duplicates the outer object but references the same internal objects, while a deep copy duplicates both the outer and inner objects.
43. What is an iterator in Python, and how is it different from an iterable?
Answer: An iterable is an object capable of returning an iterator, which can be used to iterate over its elements.
44. How do you concatenate strings and variables in Python?
Answer: You can use the +
operator or f-strings (formatted strings) to concatenate strings and variables.
45. Explain the purpose of the all()
and any()
functions in Python.
Answer: all()
returns True
if all elements in an iterable are True
. any()
returns True
if any element in the iterable is True
.
46. How do you convert a list into a string in Python?
Answer: Use the join()
method to concatenate the elements of a list into a single string.
47. What is the purpose of the del
statement in Python?
Answer: del
is used to remove an item from a list or delete a variable or object.
48. How do you reverse a list in Python?
Answer: Use the reverse()
method to reverse the order of elements in a list.
49. Explain the purpose of the staticmethod
decorator in Python.
Answer: The staticmethod
decorator defines a static method within a class that is not bound to instances and can be called on the class itself.
50. How can you convert a number from one base to another in Python?
Answer: Use the int() function with the base parameter to convert a number from one base to another.
These questions and answers cover various fundamental aspects of Python basics, making them useful for interviews and assessments.